Examples of typical problems solved with templated programming?
Template programming can solve common programming problems: container types: easily create containers such as linked lists, stacks, and queues; function functors: create objects that can be called as functions, simplifying algorithm comparison; generic algorithms: in various data Run common algorithms on types without special implementation; Container adapter: Modify existing container behavior without creating new copies; Enumeration class: Create enumerations with strong type verification at compile time.
Examples of common problems with template programming
Template programming is a powerful technique that can make code more versatile, Reusable. It can solve many typical problems in the following ways:
1. Container type
Templated programming can easily create your own container types, such as linked lists, stacks and queues, No need to reimplement common functionality such as iteration and resizing.
template<class T> class Stack { vector<T> data; int top; public: Stack() { top = -1; } void push(const T& value) { data.push_back(value); top++; } T pop() { if (top < 0) throw exception(); return data.back(); } };
2. Function functor
Template programming can help create function functors, that is, objects that can be called like functions. This is useful in algorithms, which often require the use of function pointers or anonymous functions to specify comparisons or other operations.
template<class T> struct Comparator { bool operator()(const T& a, const T& b) { return a < b; } }; // 使用方式 sort(data.begin(), data.end(), Comparator<int>());
3. Generic Algorithms
Template-based programming can create generic algorithms that work on a variety of data types without the need for each type Implement them specifically.
template<class T> void find(vector<T>& data, T value) { for (auto it = data.begin(); it != data.end(); it++) { if (*it == value) return; } throw exception(); }
4. Container Adapters
Templated programming allows you to create container adapters that modify the behavior of existing containers without creating new copies of the containers.
template<class Container> class IndexedContainer { Container& container; size_t index; public: IndexedContainer(Container& c) : container(c), index(0) {} T& operator*() { return container[index]; } void operator++() { index++; } }; // 使用方式 for (auto& item : IndexedContainer(data)) { // ... }
5. Enumeration classes
Template-based programming makes it easy to create enumeration classes with strong type validation that is checked at compile time.
enum class Color { Red, Green, Blue }; template<Color C> struct ColorName { static const char* name() { switch (C) { case Color::Red: return "Red"; case Color::Green: return "Green"; case Color::Blue: return "Blue"; } } };
The above is the detailed content of Examples of typical problems solved with templated programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
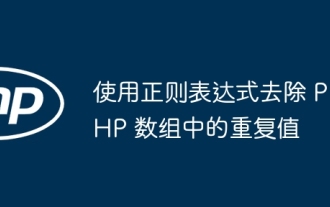
How to remove duplicate values from PHP array using regular expressions: Use regular expression /(.*)(.+)/i to match and replace duplicates. Iterate through the array elements and check for matches using preg_match. If it matches, skip the value; otherwise, add it to a new array with no duplicate values.
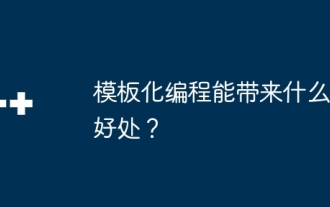
Templated programming improves code quality because it: Enhances readability: Encapsulates repetitive code, making it easier to understand. Improved maintainability: Just change the template to accommodate data type changes. Optimization efficiency: The compiler generates optimized code for specific data types. Promote code reuse: Create common algorithms and data structures that can be reused.
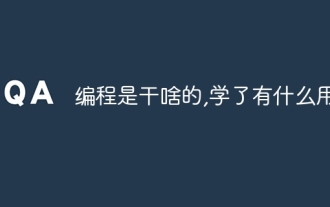
1. Programming can be used to develop various software and applications, including websites, mobile applications, games, and data analysis tools. Its application fields are very wide, covering almost all industries, including scientific research, health care, finance, education, entertainment, etc. 2. Learning programming can help us improve our problem-solving skills and logical thinking skills. During programming, we need to analyze and understand problems, find solutions, and translate them into code. This way of thinking can cultivate our analytical and abstract abilities and improve our ability to solve practical problems.
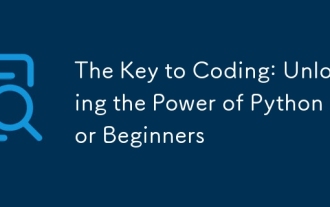
Python is an ideal programming introduction language for beginners through its ease of learning and powerful features. Its basics include: Variables: used to store data (numbers, strings, lists, etc.). Data type: Defines the type of data in the variable (integer, floating point, etc.). Operators: used for mathematical operations and comparisons. Control flow: Control the flow of code execution (conditional statements, loops).
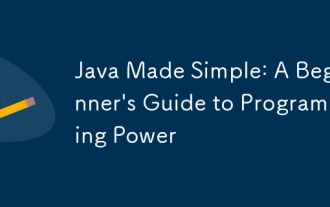
Java Made Simple: A Beginner's Guide to Programming Power Introduction Java is a powerful programming language used in everything from mobile applications to enterprise-level systems. For beginners, Java's syntax is simple and easy to understand, making it an ideal choice for learning programming. Basic Syntax Java uses a class-based object-oriented programming paradigm. Classes are templates that organize related data and behavior together. Here is a simple Java class example: publicclassPerson{privateStringname;privateintage;
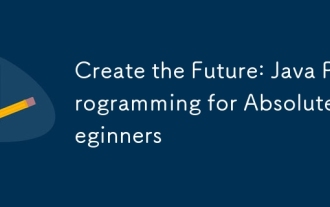
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
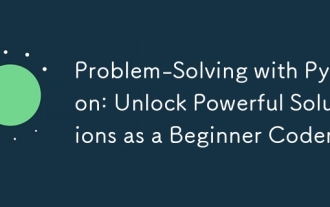
Pythonempowersbeginnersinproblem-solving.Itsuser-friendlysyntax,extensivelibrary,andfeaturessuchasvariables,conditionalstatements,andloopsenableefficientcodedevelopment.Frommanagingdatatocontrollingprogramflowandperformingrepetitivetasks,Pythonprovid
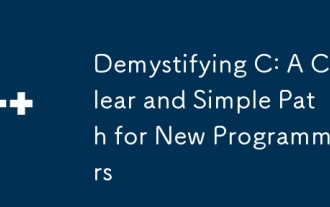
C is an ideal choice for beginners to learn system programming. It contains the following components: header files, functions and main functions. A simple C program that can print "HelloWorld" needs a header file containing the standard input/output function declaration and uses the printf function in the main function to print. C programs can be compiled and run by using the GCC compiler. After you master the basics, you can move on to topics such as data types, functions, arrays, and file handling to become a proficient C programmer.
