Design and implementation of PHP microservice architecture
PHP microservice architecture follows the principles of single responsibility, loose coupling, scalability, and fault tolerance, and creates user management microservices through case demonstrations. Microservices are typically deployed in Docker or Kubernetes, and monitoring performance, availability, errors, and dependencies is critical to achieve scalable, fault-tolerant, maintainable applications.
Design and Implementation of PHP Microservice Architecture
Introduction
Microservice architecture is a software design A pattern that decomposes an application into a set of loosely coupled, independently deployed microservices. This architectural style provides many benefits, including scalability, fault tolerance, and maintainability.
Design principles
The design of PHP microservice architecture should follow the following principles:
- Single responsibility principle: Each microservice should only be responsible for one specific task.
- Loose coupling: Microservices should be as loosely coupled as possible to reduce dependencies.
- Scalability: The architecture should support the expansion of the application as needed.
- Fault Tolerance: The architecture should be able to cope with microservice failures and outages.
Practical case: User management microservice
As a practical case, we create a user management microservice. This microservice will be responsible for user registration, login and management.
Code Implementation
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
Deployment
Microservices are typically deployed in Docker containers or Kubernetes clusters. This provides the benefits of scalability, fault tolerance, and ease of deployment.
Monitoring
Monitoring of microservice architecture is crucial. Microservice performance, availability, errors, and dependencies should be monitored. This helps detect problems early and take corrective action.
Conclusion
PHP microservices architecture provides a powerful solution for building scalable, fault-tolerant and maintainable applications. By following design principles and illustrating with practical examples, we show how to design and implement a PHP microservices architecture.
The above is the detailed content of Design and implementation of PHP microservice architecture. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
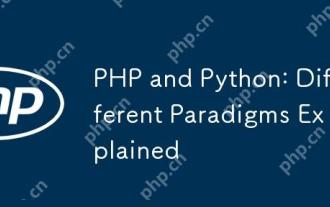
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
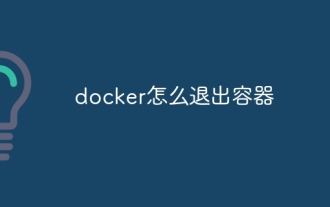
Four ways to exit Docker container: Use Ctrl D in the container terminal Enter exit command in the container terminal Use docker stop <container_name> Command Use docker kill <container_name> command in the host terminal (force exit)
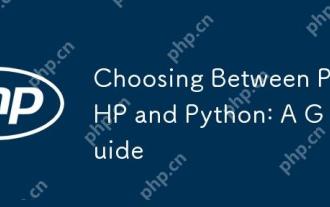
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
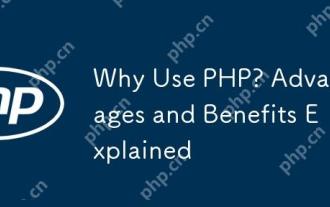
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
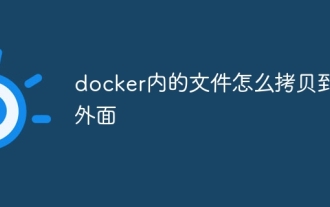
Methods for copying files to external hosts in Docker: Use the docker cp command: Execute docker cp [Options] <Container Path> <Host Path>. Using data volumes: Create a directory on the host, and use the -v parameter to mount the directory into the container when creating the container to achieve bidirectional file synchronization.
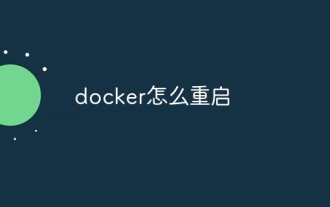
How to restart the Docker container: get the container ID (docker ps); stop the container (docker stop <container_id>); start the container (docker start <container_id>); verify that the restart is successful (docker ps). Other methods: Docker Compose (docker-compose restart) or Docker API (see Docker documentation).
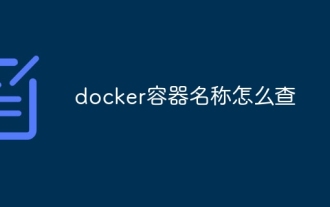
You can query the Docker container name by following the steps: List all containers (docker ps). Filter the container list (using the grep command). Gets the container name (located in the "NAMES" column).
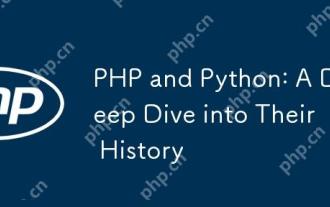
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
