


Java data structure and algorithm: practical optimization of microservice architecture
Java Data Structures and Algorithms: How to Optimize in Microservice Architecture
Introduction
In a microservices architecture, optimizing data structures and algorithms is crucial to improve system performance and scalability. This article explores how to use appropriate data structures to optimize common microservices architecture patterns and provides real-world examples.
Data structure
- Arrays and linked lists: Used to store and access linear data. Arrays provide fast access, while linked lists have advantages in inserting and deleting elements.
- Stacks and queues: Last-in-first-out (LIFO) and first-in-first-out (FIFO) structures are used to temporarily store data.
- Hash table: Use key-value pairs to store data and provide fast retrieval.
- Trees and graphs: Used to store and navigate complex data structures.
Real example
Scenario 1: Storing authentication information in the gateway microservice
Problem : Highly concurrent requests require fast access to authentication information.
Solution: Use a hash table to store user ID and token pairs. This structure allows fast lookups with O(1) time complexity.
Scenario 2: Storing pending tasks in the message queue
Problem: It is necessary to ensure that tasks are executed in FIFO order.
Solution: Use a queue to store tasks. The first-in-first-out mechanism ensures that tasks are processed in order.
Scenario 3: Storing popular data in a cache service
Problem: Frequently accessed data needs to be retrieved as quickly as possible.
Solution: Use an array or linked list to store popular data. These structures provide fast sequential access.
Algorithm
- Sort algorithm: Used to sort data, such as merge sort and quick sort.
- Search algorithm: Used to find specific elements in a data structure, such as binary search.
- Graph algorithms: Used to process graph structures, such as breadth-first search and depth-first search.
Real example
Scenario 4: Searching for text in a search service
Question: Need to search large amounts of text efficiently.
Solution: Use the trie data structure. This structure supports prefix searches and fast matching.
Scenario 5: Calculating similarity in a recommendation system
Problem: Need to calculate the similarity between users to recommend content to them .
Solution: Use cosine similarity or Jaccard similarity algorithm. These algorithms measure the similarity of two vectors.
Scenario 6: Selecting the best service instance in the routing service
Problem: It is necessary to select the best performing service instance from a set of service instances Example.
Solution: Use Dijkstra's algorithm or A* algorithm. These algorithms find the shortest path in a weight graph that represents the latency between service instances.
Conclusion
Using appropriate data structures and algorithms is crucial to optimizing microservices architecture. By carefully considering the performance requirements of different use cases, developers can significantly improve system performance, scalability, and reliability.
The above is the detailed content of Java data structure and algorithm: practical optimization of microservice architecture. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
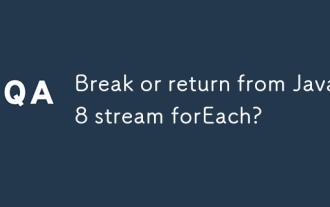
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
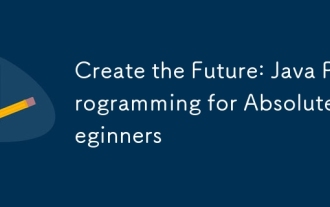
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
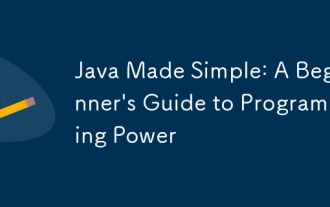
Java Made Simple: A Beginner's Guide to Programming Power Introduction Java is a powerful programming language used in everything from mobile applications to enterprise-level systems. For beginners, Java's syntax is simple and easy to understand, making it an ideal choice for learning programming. Basic Syntax Java uses a class-based object-oriented programming paradigm. Classes are templates that organize related data and behavior together. Here is a simple Java class example: publicclassPerson{privateStringname;privateintage;
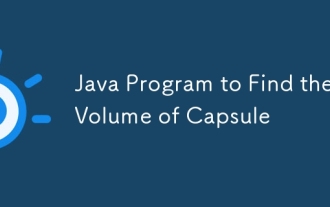
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
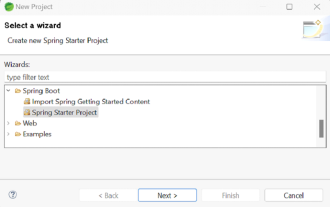
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
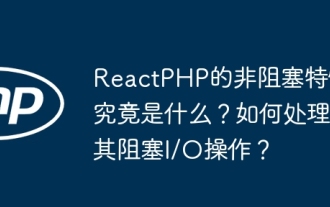
An official introduction to the non-blocking feature of ReactPHP in-depth interpretation of ReactPHP's non-blocking feature has aroused many developers' questions: "ReactPHPisnon-blockingbydefault...
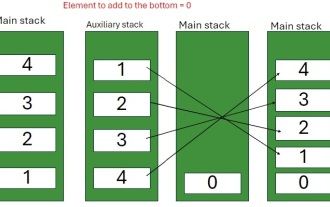
A stack is a data structure that follows the LIFO (Last In, First Out) principle. In other words, The last element we add to a stack is the first one to be removed. When we add (or push) elements to a stack, they are placed on top; i.e. above all the
