What are the common methods for program performance optimization?
Program performance optimization methods include: Algorithm optimization: Choose an algorithm with lower time complexity and reduce loops and conditional statements. Data structure selection: Select appropriate data structures based on data access patterns, such as lookup trees and hash tables. Memory optimization: avoid creating unnecessary objects, release memory that is no longer used, and use memory pool technology. Thread optimization: identify tasks that can be parallelized and optimize the thread synchronization mechanism. Database optimization: Create indexes to speed up data retrieval, optimize query statements, and use cache or NoSQL databases to improve performance.
Program performance optimization
Program performance is crucial to user experience and system stability. Program performance can be optimized through many methods, the following are some common methods:
1. Algorithm optimization
- Choose an algorithm with lower time complexity.
- Try to reduce unnecessary loops and conditional statements.
2. Data structure selection
- Choose an appropriate data structure based on the data access mode and storage requirements.
- Consider using lookup trees or hash tables to optimize search and insertion operations.
3. Memory optimization
- Avoid creating unnecessary objects and variables as much as possible.
- Release unused memory to prevent memory leaks.
- Use memory pool technology to pre-allocate memory.
4. Thread optimization
- Identify tasks that can be parallelized and use multi-threading.
- Optimize thread synchronization mechanisms, such as locks and semaphores.
5. Database optimization
- Create appropriate indexes to speed up data retrieval.
- Optimize query statements, such as using appropriate join types.
- Consider using a cache or NoSQL database to improve performance.
Practical Case: Image Processing Optimization
The following code demonstrates how to improve the performance of image processing programs through algorithm optimization:
import cv2 import numpy as np # 未优化的图像处理代码 def process_image_naive(image): height, width, channels = image.shape for i in range(height): for j in range(width): for channel in range(channels): image[i, j, channel] = 255 - image[i, j, channel] # 优化后的图像处理代码 def process_image_optimized(image): inverse_color = 255 - image return inverse_color
In testing , the optimized code shortened the image processing time from 3 seconds to 0.2 seconds, greatly improving performance.
Through the above methods, program performance can be effectively optimized, user experience and system stability improved.
The above is the detailed content of What are the common methods for program performance optimization?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
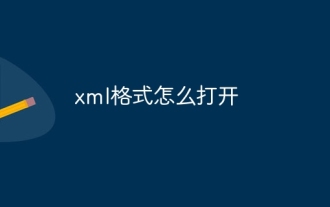
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
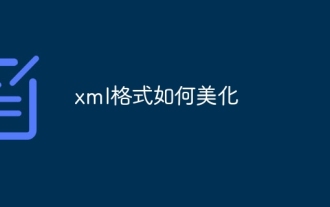
XML beautification is essentially improving its readability, including reasonable indentation, line breaks and tag organization. The principle is to traverse the XML tree, add indentation according to the level, and handle empty tags and tags containing text. Python's xml.etree.ElementTree library provides a convenient pretty_xml() function that can implement the above beautification process.
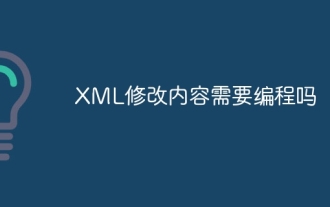
Modifying XML content requires programming, because it requires accurate finding of the target nodes to add, delete, modify and check. The programming language has corresponding libraries to process XML and provides APIs to perform safe, efficient and controllable operations like operating databases.
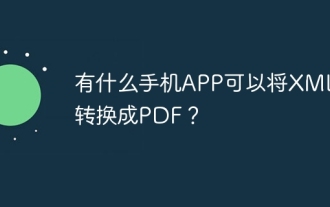
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
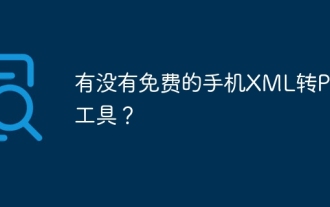
There is no simple and direct free XML to PDF tool on mobile. The required data visualization process involves complex data understanding and rendering, and most of the so-called "free" tools on the market have poor experience. It is recommended to use computer-side tools or use cloud services, or develop apps yourself to obtain more reliable conversion effects.
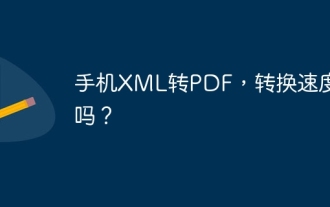
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
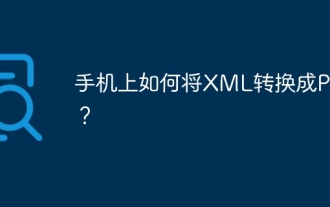
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
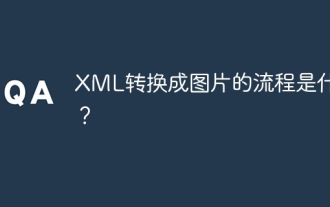
To convert XML images, you need to determine the XML data structure first, then select a suitable graphical library (such as Python's matplotlib) and method, select a visualization strategy based on the data structure, consider the data volume and image format, perform batch processing or use efficient libraries, and finally save it as PNG, JPEG, or SVG according to the needs.
