How design patterns deal with code maintenance challenges
Design patterns solve code maintenance challenges by providing reusable and extensible solutions: Observer pattern: allows objects to subscribe to events and receive notifications when events occur. Factory Pattern: Provides a centralized way to create objects without relying on concrete classes. Singleton pattern: ensures that a class has only one instance, which is used to create globally accessible objects.
How design patterns deal with code maintenance challenges
Code maintenance is a difficult task, especially for large projects. Design patterns can help solve this problem by providing reusable and scalable solutions.
Observer Pattern
The Observer pattern allows objects to subscribe to events and receive notifications when events occur. This avoids hard-coded dependencies, making your code more readable and maintainable.
public class Subject { private List<Observer> observers = new ArrayList<>(); public void addObserver(Observer observer) { observers.add(observer); } public void removeObserver(Observer observer) { observers.remove(observer); } public void notifyObservers() { for (Observer observer : observers) { observer.update(); } } } public class Observer { public void update() { // Implement logic to respond to event } }
Practical case: In GUI applications, controller objects can act as Subjects, and buttons, text boxes, and labels can act as Observers. When the user interacts with the control, the controller notifies all Observers to make corresponding updates.
Factory Pattern
The factory pattern provides a centralized way to create objects without relying on concrete classes. This eliminates hard-coded dependencies on class hierarchies, making the code easier to modify and extend.
public interface Shape { void draw(); } public class Circle implements Shape { @Override public void draw() { // Draw circle } } public class Square implements Shape { @Override public void draw() { // Draw square } } public class ShapeFactory { public static Shape getShape(String shapeType) { switch (shapeType) { case "CIRCLE": return new Circle(); case "SQUARE": return new Square(); default: throw new IllegalArgumentException("Invalid shape type"); } } }
Practical case: In the graphics editor, ShapeFactory can create specific shape objects based on user selections. This eliminates the need to directly instantiate different shape classes.
Singleton pattern
The singleton pattern ensures that there is only one instance of a class. This is useful for creating globally accessible objects, such as logging objects or database connection objects.
public class Singleton { private static Singleton instance; private Singleton() {} public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
Practical case: In server applications, the singleton pattern can be used to create unique data access objects to ensure data consistency.
The above is the detailed content of How design patterns deal with code maintenance challenges. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


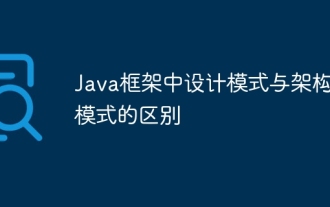
In the Java framework, the difference between design patterns and architectural patterns is that design patterns define abstract solutions to common problems in software design, focusing on the interaction between classes and objects, such as factory patterns. Architectural patterns define the relationship between system structures and modules, focusing on the organization and interaction of system components, such as layered architecture.

.NET 4.0 is used to create a variety of applications and it provides application developers with rich features including: object-oriented programming, flexibility, powerful architecture, cloud computing integration, performance optimization, extensive libraries, security, Scalability, data access, and mobile development support.
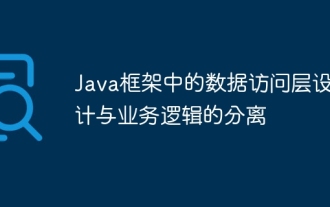
Answer: The separation of data access layer (DAL) from business logic is crucial for Java applications as it enhances reusability, maintainability, and testability. DAL manages the interaction with the database (read, update, delete), while business logic contains business rules and algorithms. SpringDataJPA provides a simplified data access interface that can be extended by implementing custom methods or query methods. Business logic services rely on the DAL but must not interact with the database directly, this can be tested using a mock or in-memory database. Separating DAL and business logic is key to designing maintainable and testable Java applications.
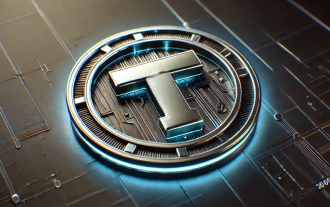
Original title: Bittensor=AIBitcoin? Original author: S4mmyEth, Decentralized AI Research Original translation: zhouzhou, BlockBeats Editor's note: This article discusses Bittensor, a decentralized AI platform, hoping to break the monopoly of centralized AI companies through blockchain technology and promote an open and collaborative AI ecosystem. Bittensor adopts a subnet model that allows the emergence of different AI solutions and inspires innovation through TAO tokens. Although the AI market is mature, Bittensor faces competitive risks and may be subject to other open source
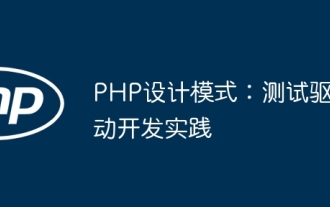
TDD is used to write high-quality PHP code. The steps include: writing test cases, describing the expected functionality and making them fail. Write code so that only the test cases pass without excessive optimization or detailed design. After the test cases pass, optimize and refactor the code to improve readability, maintainability, and scalability.
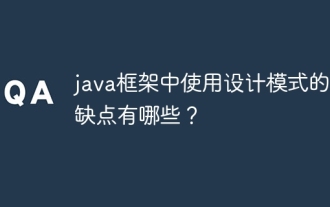
The advantages of using design patterns in Java frameworks include: enhanced code readability, maintainability, and scalability. Disadvantages include complexity, performance overhead, and steep learning curve due to overuse. Practical case: Proxy mode is used to lazy load objects. Use design patterns wisely to take advantage of their advantages and minimize their disadvantages.
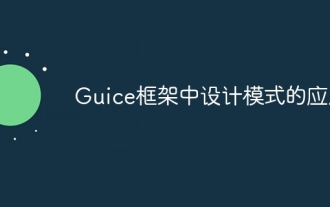
The Guice framework applies a number of design patterns, including: Singleton pattern: ensuring that a class has only one instance through the @Singleton annotation. Factory method pattern: Create a factory method through the @Provides annotation and obtain the object instance during dependency injection. Strategy mode: Encapsulate the algorithm into different strategy classes and specify the specific strategy through the @Named annotation.
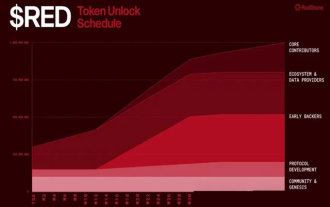
In-depth analysis of the 64th issue of Launchpool project RED: Modular oracle prospects and currency price predictions This article deeply analyzes the 64th issue of Launchpool project RED - a multi-chain oracle project across EVM and non-EVM chains, and makes reasonable estimates of the project fundamentals and currency price. The RED project was launched for only 2 days, with the total volume of Launchpool being 40,000,000RED (accounting for 4% of the maximum supply of tokens), and the initial circulation was 280,000,000RED (accounting for 28% of the total supply of tokens). Project Overview: RedStone is a modular blockchain oracle founded in 2020 and incubated by Arweave Chain with the team from Estonia. Currently supports 70 chains
