


Exception handling in C++ technology: How to use exception handling for error handling and recovery?
Answer: Exception handling in C can be used to handle and recover from run-time errors. Exception handling mechanism: Exception throwing: Use the throw keyword to throw an exception object. Exception catching: The catch block catches the thrown exception. Exception handling: Try-catch blocks surround code that may throw exceptions. Best practice: Use exception handling only when needed. Throw specific and meaningful exceptions. Properly handle all thrown exceptions. Use noexcept to specify functions that will not throw exceptions.
Exception Handling in C Technology: A Guide to Error Handling and Recovery
Introduction
Exception Handling is C A powerful mechanism for handling and recovering from runtime errors. By detecting and handling exceptions, programmers can ensure that applications remain stable and predictable when unexpected conditions arise.
Exception handling mechanism
The exception handling mechanism mainly includes the following steps:
-
Exception throwing (throw): When an exception is detected, an exception object is thrown through the
throw
keyword. Exception objects contain specific information about the error. -
Exception catching (catch): The
catch
block is used to catch thrown exceptions. Eachcatch
block specifies one or more exception types that it can handle. -
Exception handling (try-catch):
try-catch
blocks surround code in areas where exceptions may be thrown. When an exception is thrown in this code, control goes to the correspondingcatch
block to handle the exception.
Practical Case
Consider the following example that demonstrates how to use exception handling to handle file open errors:
#include <iostream> #include <fstream> using namespace std; int main() { try { ifstream file("myfile.txt"); if (!file.is_open()) { throw runtime_error("无法打开文件"); } // 对文件流进行操作 } catch (const runtime_error& e) { cout << "文件打开错误:" << e.what() << endl; } return 0; }
In this example, ifstream::is_open()
Function checks whether the file has been opened successfully. If the file is not open, a runtime_error
exception will be thrown. catch
block catches this exception and prints an error message.
Termination vs. Non-termination exception
Exceptions can be divided into termination exceptions and non-termination exceptions:
-
Termination exceptionWill terminate the program immediately upon detection. Examples of termination exceptions include
bad_alloc
andbad_cast
. -
Non-terminating exceptionAllows the program to continue execution after handling the exception. Examples of non-terminating exceptions include
runtime_error
andlogic_error
.
Best Practices
There are some best practices to follow when using exception handling:
- Only when needed Use exception handling when doing so.
- Throw specific and meaningful exceptions.
- Properly handle all thrown exceptions.
- Use
noexcept
to specify functions that will not throw exceptions.
The above is the detailed content of Exception handling in C++ technology: How to use exception handling for error handling and recovery?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


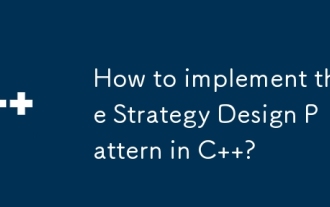
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
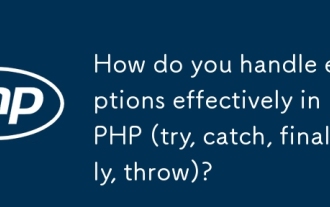
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
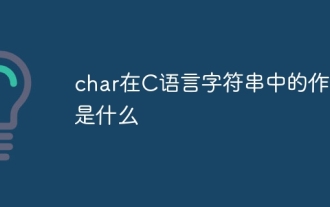
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
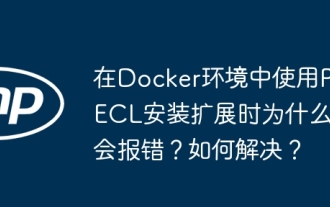
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
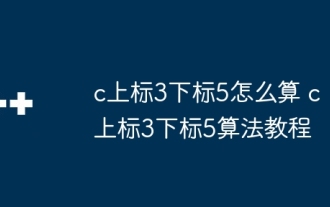
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
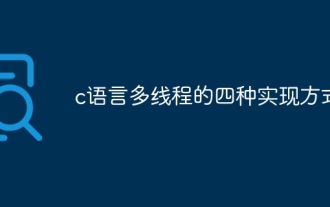
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
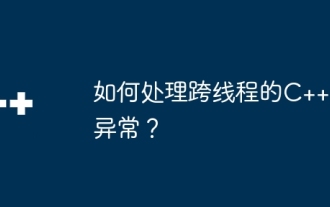
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
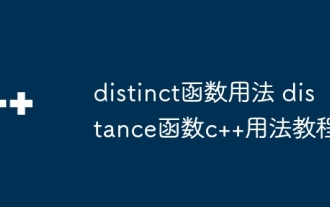
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
