


Using Golang to develop blockchain decentralized financial applications
The Go programming language is ideal for building DeFi applications as it allows users to write smart contracts, interact with the blockchain, and create tokens. Smart contracts can be used to define rules and logic, libraries and tools can be used to interact with the blockchain, and practical examples provide examples of creating tokens that allow users to mint, transfer and check balances.
Using Go to build blockchain decentralized finance (DeFi) applications
Introduction
The development of blockchain technology has promoted the rise of the field of decentralized finance (DeFi). DeFi applications allow users to conduct various financial transactions without relying on traditional financial institutions. Go is a powerful, efficient, and easy-to-use programming language that is ideal for developing DeFi applications.
Creating Smart Contracts
Smart contracts are self-executing pieces of code stored on the blockchain. They can be used to define the rules and logic of DeFi applications. Here is a simple smart contract example written in Go:
package main import ( "fmt" ) type Asset struct { Name string Value float64 } func main() { asset := Asset{ Name: "MyAsset", Value: 100.0, } fmt.Printf("Asset Name: %s\n", asset.Name) fmt.Printf("Asset Value: %f\n", asset.Value) }
Interacting with the Blockchain
Once the smart contract is deployed, you can use libraries in Go and Tools interact with the blockchain. For example, you can use the Ethereum client library to connect to the Ethereum network and interact with contracts:
package main import ( "context" "fmt" "github.com/ethereum/go-ethereum/client" "github.com/ethereum/go-ethereum/common" "github.com/ethereum/go-ethereum/ethclient" ) func main() { // 连接到以太坊网络 client, err := client.Dial("ws://localhost:8546") if err != nil { panic(err) } defer client.Close() // 加载智能合约 ABI contractAddress := common.HexToAddress("0x1234...") bytecode, err := client.CodeAt(context.Background(), contractAddress, nil) // 从链上获取已部署的合约bytecode if err != nil { panic(err) } // 创建与合约交互的函数 contract, err := ethclient.NewContract(contractAddress, bytecode) if err != nil { panic(err) } // 调用智能合约函数 result, err := contract.Call(context.Background(), "balanceOf", common.HexToAddress("0xabcd...")) // 注意此处传入函数名及其参数 if err != nil { panic(err) } fmt.Println(result) }
Practical case: Token creation
The following is an example created using Go Practical example of tokens:
// 定义代币合约 type Token struct { Name string Symbol string TotalSupply int64 Balances map[string]int64 } // 创建代币 func NewToken(name, symbol string, totalSupply int64) *Token { return &Token{ Name: name, Symbol: symbol, TotalSupply: totalSupply, Balances: make(map[string]int64), } } // 分发代币 func (token *Token) Distribute(recipient string, amount int64) { token.Balances[recipient] += amount } // 转移代币 func (token *Token) Transfer(sender, recipient string, amount int64) { token.Balances[sender] -= amount token.Balances[recipient] += amount } // 主函数 func main() { // 创建代币 token := NewToken("MyToken", "MTK", 1000000) // 分发代币 token.Distribute("Alice", 500000) token.Distribute("Bob", 300000) token.Distribute("Carol", 200000) // 转移代币 token.Transfer("Alice", "Bob", 100000) // 输出余额 fmt.Println("Alice's Balance:", token.Balances["Alice"]) fmt.Println("Bob's Balance:", token.Balances["Bob"]) fmt.Println("Carol's Balance:", token.Balances["Carol"]) }
This Go program creates an ERC-20 compatible token named "MyToken". It allows users to mint, transfer and check balances.
The above is the detailed content of Using Golang to develop blockchain decentralized financial applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
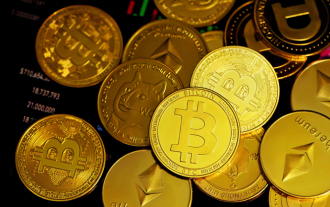
OKX is a global digital asset trading platform. Its main functions include: 1. Buying and selling digital assets (spot trading), 2. Trading between digital assets, 3. Providing market conditions and data, 4. Providing diversified trading products (such as derivatives), 5. Providing asset value-added services, 6. Convenient asset management.
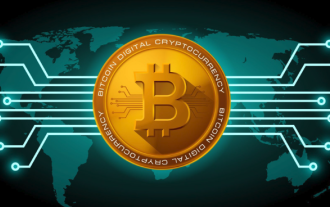
This article recommends ten well-known virtual currency-related APP recommendation websites, including Binance Academy, OKX Learn, CoinGecko, CryptoSlate, CoinDesk, Investopedia, CoinMarketCap, Huobi University, Coinbase Learn and CryptoCompare. These websites not only provide information such as virtual currency market data, price trend analysis, etc., but also provide rich learning resources, including basic blockchain knowledge, trading strategies, and tutorials and reviews of various trading platform APPs, helping users better understand and make use of them
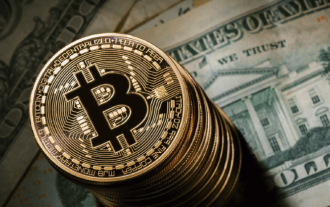
This article lists the top ten well-known Web3 trading platforms, including Binance, OKX, Gate.io, Kraken, Bybit, Coinbase, KuCoin, Bitget, Gemini and Bitstamp. The article compares the characteristics of each platform in detail, such as the number of currencies, trading types (spot, futures, options, NFT, etc.), handling fees, security, compliance, user groups, etc., aiming to help investors choose the most suitable trading platform. Whether it is high-frequency traders, contract trading enthusiasts, or investors who focus on compliance and security, they can find reference information from it.

The handling fees of the Gate.io trading platform vary according to factors such as transaction type, transaction pair, and user VIP level. The default fee rate for spot trading is 0.15% (VIP0 level, Maker and Taker), but the VIP level will be adjusted based on the user's 30-day trading volume and GT position. The higher the level, the lower the fee rate will be. It supports GT platform coin deduction, and you can enjoy a minimum discount of 55% off. The default rate for contract transactions is Maker 0.02%, Taker 0.05% (VIP0 level), which is also affected by VIP level, and different contract types and leverages
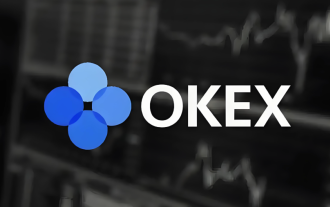
This article introduces in detail the steps and preparations for withdrawing OKX digital currency. First of all, it is necessary to ensure that account registration, real-name authentication has been completed, and sufficient withdrawal balance has been established. Secondly, be sure to prepare an accurate external storage address. The article then gradually explained the operation steps such as logging in to the account, entering the withdrawal page, selecting currency, filling in withdrawal information (including address, quantity, handling fee), confirming withdrawal and viewing withdrawal records, and emphasized the necessity of checking the information to avoid asset losses.
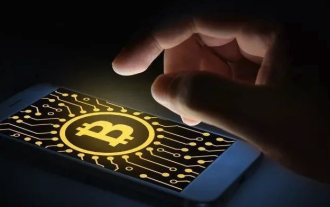
This article has compiled the official website links of the top ten popular exchanges in the Web3 field to facilitate users to quickly access. It includes well-known platforms such as Binance, OKX, Gate.io, Coinbase, Huobi (now HTX), KuCoin, Kraken, Bitget, MEXC and Bybit. The article lists the official website addresses of each exchange and specifically reminds that the information on the MEXC official website is temporarily missing. Users can search their APP through the app store or download platform. If you want to know about Web3 exchange information, this article will be a convenient reference for you.
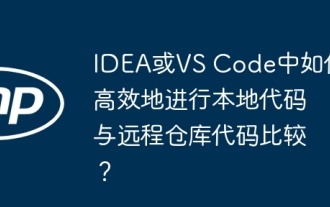
In IDEA or VS...
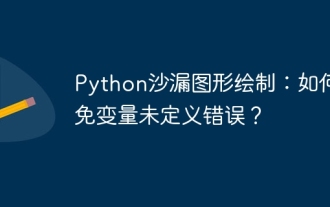
Getting started with Python: Hourglass Graphic Drawing and Input Verification This article will solve the variable definition problem encountered by a Python novice in the hourglass Graphic Drawing Program. Code...
