How to implement the power function in C language
In C language, there are two ways to implement exponentiation operation: use the pow() function to calculate the power of the second parameter of the first parameter. Define a custom power function, which can be implemented recursively or iteratively: the recursive method continues to double the power until it is 0. The iterative method uses a loop to multiply the base one by one.
C language exponentiation function implementation
Introduction
In C language , to implement the exponentiation operation you need to use the pow() function or a custom function.
pow() function The
- pow() function is provided by the
<math.h>
header file. - It calculates and returns the first parameter raised to the power of the second parameter.
- Syntax:
double pow(double base, double exponent);
Example:
#include <math.h> int main() { double base = 2.0; double exponent = 3.0; double result = pow(base, exponent); printf("结果:%.2f\n", result); // 输出:8.00 return 0; }
Customized exponentiation function
You can also define your own exponentiation function, which is achieved through recursion or iteration.
Recursive method
- The function keeps reducing the power by one until it reaches 0.
- Syntax:
int my_pow(int base, int exponent);
Example:
int my_pow(int base, int exponent) { if (exponent == 0) { return 1; } else if (exponent > 0) { return base * my_pow(base, exponent - 1); } else { return 1.0 / my_pow(base, -exponent); } }
Iteration method
- The function uses a loop to multiply the base one by one.
- Syntax:
int my_pow_iterative(int base, int exponent);
Example:
int my_pow_iterative(int base, int exponent) { int result = 1; int i; for (i = 0; i < exponent; i++) { result *= base; } return result; }
The above is the detailed content of How to implement the power function in C language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
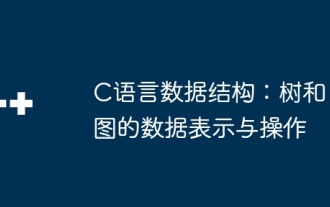
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
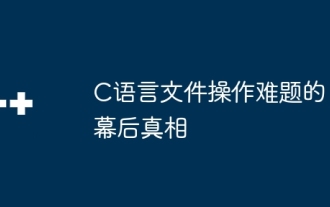
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
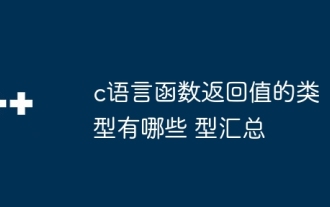
The return value types of C language function include int, float, double, char, void and pointer types. int is used to return integers, float and double are used to return floats, and char returns characters. void means that the function does not return any value. The pointer type returns the memory address, be careful to avoid memory leakage.结构体或联合体可返回多个相关数据。
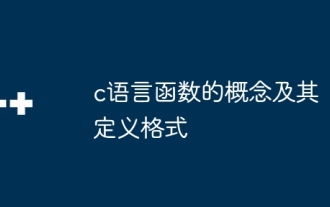
C language functions are reusable code blocks, receive parameters for processing, and return results. It is similar to the Swiss Army Knife, powerful and requires careful use. Functions include elements such as defining formats, parameters, return values, and function bodies. Advanced usage includes function pointers, recursive functions, and callback functions. Common errors are type mismatch and forgetting to declare prototypes. Debugging skills include printing variables and using a debugger. Performance optimization uses inline functions. Function design should follow the principle of single responsibility. Proficiency in C language functions can significantly improve programming efficiency and code quality.
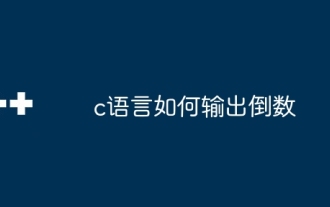
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
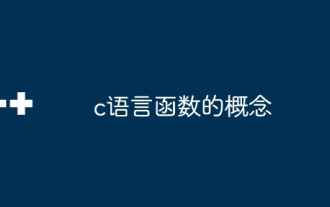
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
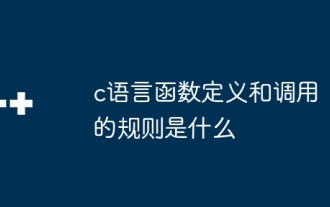
A C language function consists of a parameter list, function body, return value type and function name. When a function is called, the parameters are copied to the function through the value transfer mechanism, and will not affect external variables. Pointer passes directly to the memory address, modifying the pointer will affect external variables. Function prototype declaration is used to inform the compiler of function signatures to avoid compilation errors. Stack space is used to store function local variables and parameters. Too much recursion or too much space can cause stack overflow.
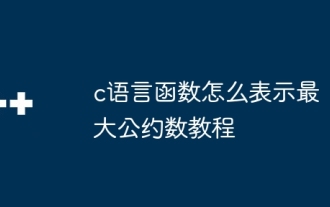
Methods to efficiently and elegantly find the greatest common divisor in C language: use phase division to solve by constantly dividing the remainder until the remainder is 0. Two implementation methods are provided: recursion and iteration are concise and clear, and the iterative implementation is higher and more stable. Pay attention to handling negative numbers and 0s, and consider performance optimization, but the phase division itself is efficient enough.
