


Simple registration module form verification made with javascript_javascript skills
一个注册框 进行表单验证处理
如图
有简单的验证提示功能
代码思路也比较简单
输入框失去焦点时便检测,并进行处理
表单具有 onsubmit = "return check()"行为,处理验证情况
点击提交表单按钮时,进行最终的验证,达到是否通过表单提交的请求。
先是最基本的html+css部分
<style type="text/css"> body{margin:0;padding: 0;} .login{position:relative;margin:100px auto;padding:50px 20px;width: 350px;height: 200px;border:1px solid #333;} .login legend{font-weight: bold;color: green;text-align: center;} .login label{display:inline-block;width:130px;text-align: right;} .btn{height: 30px;width:100px;padding: 5px;border:0;background-color: #00dddd;font-weight: bold;cursor: pointer;float: right;} input{height: 20px;width: 170px;} .borderRed{border: 2px solid red;} img{display: none;} </style> </head> <body> <div class="login"> <form name="form" method="post" action="register.php" onsubmit="return check()"> <legend>【Register】</legend> <p><label for="name">UserName: </label> <input type="text" id="name" > <img src="./img/gou.png" width="20px" height="20px"></p> <p><label for="password">Password: </label> <input type="password" id="password" > <img src="./img/gantan.png" width="20px" height="20px"></p> <p><label for="R_password">Password Again: </label> <input type="password" id="R_password" > <img src="./img/gou.png" width="20px" height="20px"></p> <p><label for="email">Email: </label> <input type="text" id="email" > <img src="./img/gou.png" width="20px" height="20px"></p> <p><input type="submit" value="Register" class="btn"></p> </form> </div>
然后是js的class相关处理函数
function hasClass(obj,cls){ // 判断obj是否有此class return obj.className.match(new RegExp('(\\s|^)' + cls + '(\\s|$)')); } function addClass(obj,cls){ //给 obj添加class if(!this.hasClass(obj,cls)){ obj.className += " "+cls; } } function removeClass(obj,cls){ //移除obj对应的class if(hasClass(obj,cls)){ var reg = new RegExp('(\\s|^)' + cls + '(\\s|$)'); obj.className = obj.className.replace(reg," "); } }
然后是验证各个输入框的值
function checkName(name){ //验证name if(name != ""){ //不为空则正确,当然也可以ajax异步获取服务器判断用户名不重复则正确 removeClass(ele.name,"borderRed"); //移除class document.images[0].setAttribute("src","./img/gou.png"); //对应图标 document.images[0].style.display = "inline"; //显示 return true; }else{ //name不符合 addClass(ele.name,"borderRed"); //添加class document.images[0].setAttribute("src","./img/gantan.png"); //对应图标 document.images[0].style.display = "inline"; //显示 return false; } } function checkPassw(passw1,passw2){ //验证密码 if(passw1 == "" || passw2 == "" || passw1 !== passw2){ //两次密码输入不为空且不等 不符合 addClass(ele.password,"borderRed"); addClass(ele.R_password,"borderRed"); document.images[1].setAttribute("src","./img/gantan.png"); document.images[1].style.display = "inline"; document.images[2].setAttribute("src","./img/gantan.png"); document.images[2].style.display = "inline"; return false; }else{ //密码输入正确 removeClass(ele.password,"borderRed"); removeClass(ele.R_password,"borderRed"); document.images[1].setAttribute("src","./img/gou.png"); document.images[1].style.display = "inline"; document.images[2].setAttribute("src","./img/gou.png"); document.images[2].style.display = "inline"; return true; } } function checkEmail(email){ //验证邮箱 var pattern = /^([\.a-zA-Z0-9_-])+@([a-zA-Z0-9_-])+(\.[a-zA-Z0-9_-])+/; if(!pattern.test(email)){ //email格式不正确 addClass(ele.email,"borderRed"); document.images[3].setAttribute("src","./img/gantan.png"); document.images[3].style.display = "inline"; ele.email.select(); return false; }else{ //格式正确 removeClass(ele.email,"borderRed"); document.images[3].setAttribute("src","./img/gou.png"); document.images[3].style.display = "inline"; return true; } }
然后为各个输入框添加监听事件:
var ele = { //存放各个input字段obj name: document.getElementById("name"), password: document.getElementById("password"), R_password: document.getElementById("R_password"), email: document.getElementById("email") }; ele.name.onblur = function(){ //name失去焦点则检测 checkName(ele.name.value); } ele.password.onblur = function(){ //password失去焦点则检测 checkPassw(ele.password.value,ele.R_password.value); } ele.R_password.onblur = function(){ //R_password失去焦点则检测 checkPassw(ele.password.value,ele.R_password.value); } ele.email.onblur = function(){ //email失去焦点则检测 checkEmail(ele.email.value); }
最后就是点击提交注册时调用的check()函数了
function check(){ //表单提交则验证开始 var ok = false; var nameOk = false; var emailOk = false; var passwOk = false; if(checkName(ele.name.value)){ nameOk = true; } //验证name if(checkPassw(ele.password.value,ele.R_password.value)){ passwOk = true; } //验证password if(checkEmail(ele.email.value)){ emailOk = true; } //验证email if(nameOk && passwOk && emailOk){ alert("Tip: Register Success .."); //注册成功 //return true; } return false; //有误,注册失败 }
完整代码:
Register
以上所述就是本文的全部内容了,希望能够对大家学习javascript表单验证有所帮助。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
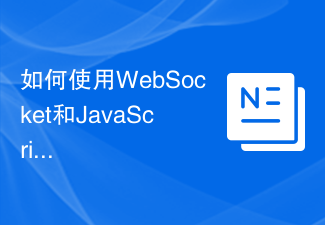
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
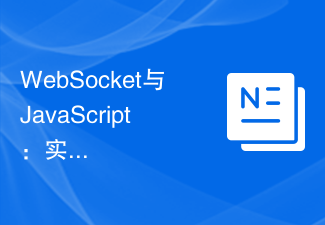
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
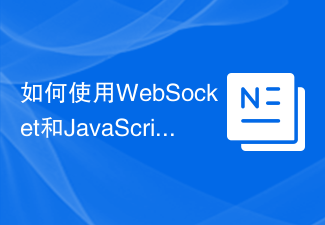
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
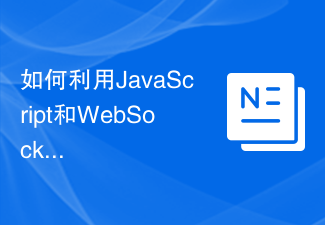
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
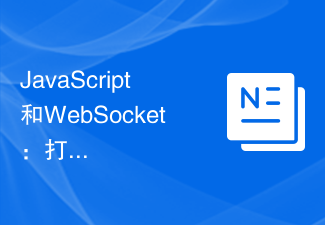
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
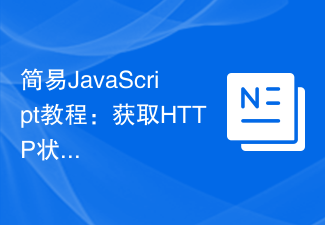
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
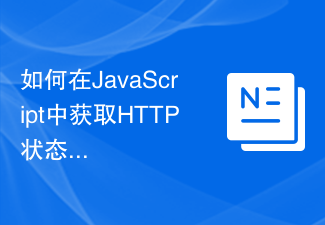
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
