


Simple voting system and js vote brushing ideas and methods_javascript skills
May 16, 2016 pm 04:05 PMI have long heard that there are some scripts for swiping votes, and some people use them to swipe votes in Weibo voting and other related polls.
Give it a try, maybe you can also swipe votes? After tinkering for a few hours, I finally got something.
(1) Voting system
To vote, you must first have a voting interface.
Of course, you can go directly to various voting websites, but it is better to create your own voting page here for your convenience.
The page is roughly as follows or View the demo
Normally, the interface is very simple, but it also basically has the basic functions of voting.
The original rule is: you can only vote once, then it prompts success, and then the button is unavailable.
They are all native JS. Those who are not flexible in DOM operations can use this to practice their skills. Of course, using jq will be very convenient.
html/css part
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"/> <head> <title>投票系统 & js脚本简单刷票</title> <style type="text/css"> *{padding: 0;margin: 0;} #wrap{margin: 0 auto; width:600px; text-align: center;} .person{position: relative; margin: 20px; float: left;} .person h4, .person p, .person button{margin-bottom: 5px;} .person h4{color: blue;} .person span{color: red;} .person button:hover{cursor: pointer; font-weight: bold;} .clear{clear: both;} </style> </head> <body> <div id="wrap"> <h3>给你的小伙伴投上一票吧</h3> <div class="person"> <h4>one</h4> <p>总票数: <span>0</span> 票</p> <button>给它投票</button> </div> <div class="person"> <h4>two</h4> <p>总票数: <span>0</span> 票</p> <button>给它投票</button> </div> <div class="person"> <h4>three</h4> <p>总票数: <span>0</span> 票</p> <button>给它投票</button> </div> <div class="person"> <h4>four</h4> <p>总票数: <span>0</span> 票</p> <button>给它投票</button> </div> <div class="clear"></div> </div>
js part
<script type="text/javascript"> function getElemensByClassName(className){ // 通过class获取 var classArr = new Array(); var tags = document.getElementsByTagName("*"); //获取所有节点 for(var item in tags){ if(tags[item].nodeType == 1){ if(tags[item].getAttribute("class") == className){ classArr.push(tags[item]); //收集class匹配的节点 } } } return classArr; } function delete_FF(element){ // 在FireFox中删除子节点为空的元素 var childs = element.childNodes; for(var i=0;i<childs.length;i++){ var pattern = /\s/; //模式匹配,内容为空 if(childs[i].nodeName == "#text" && pattern.test(childs[i].nodeValue)){ //处理 //alert(childs[i].nodeName); element.removeChild(childs[i]); //删除FF中获取的空节点 } } } window.onload = function(){ var persons = getElemensByClassName("person"); // alert(persons); for(var item in persons){ //遍历所有person,为它们绑定投票事件 (function(_item){ //匿名函数传入item, 防止因作用域问题导致item总为最后一个 delete_FF(persons[_item]); //出去FF中空行代表的子节点 persons[_item].setAttribute("id","person"+(parseInt(_item)+1)); //赋上id var childs = persons[_item].childNodes; for(var i = 0;i<childs.length;i++){ //alert(childs[i].nodeName); if(childs[i].nodeName == "BUTTON"){ //点击按钮投票 var oButton = childs[i]; } if(childs[i].nodeName == "P"){ //投票结果更新 var oP = childs[i]; var oSpan = oP.getElementsByTagName("span")[0]; } } if(oButton != null){ oButton.onclick = function(){ //事件绑定 var num = oSpan.innerHTML; //获取票数 oSpan.innerHTML = (++num); //票数更新 // 这时一般我们可能就需要把这个票数num传送给服务器保存,更新时也是和服务器中的num同步 this.setAttribute("disabled","true"); // 一般只能投票一次的吧 alert("投票成功,谢谢您的支持"); }; } })(item); // 传入各项person } }; </script>
The comments should be clearer, a simple voting page.
(2) Implementation of ticket brushing script
The vote brushing script means voting is implemented through the script. How to achieve voting?
From the above code, we know that general voting is to click "Vote", so that the data is processed.
The front-end has a vote statistics num, and the back-end also has a vote statistics num. They are synchronized. We do not need to pay attention to the back-end num because the front-end and back-end are synchronized.
When the click event is triggered, js will naturally synchronize num. If we want to swipe tickets, we just need to trigger the click event.
Furthermore, the voting system is someone else’s page, and we have no right to modify it. What we can do is actually simulate the occurrence of events through js.
Now that you have written the script, how should you use it?
Usually, you use the console mode, such as the FireFox Chrome console, put your own script into it, and it will parse and execute it and process the page data.
For example, in the FireBug console here, the information is displayed on the left, and js code can be entered on the right.
Or you can also use the chrome console, just enter js and press Enter to execute
If you haven’t used these things in children’s shoes, you can search for relevant knowledge.
Then write a simple ticket brushing script
First of all, we follow the formal method, assuming that the voting page is not written by us, how do we brush the votes?
We must find out the key points of voting.
Use the review element to find it, usually the voting button.
Click that and then move the mouse to the button on the voting page. Try it? Search for other tag information in the page, such as id class, etc., which will be used later.
Okay, determine the relevant information, id tag type, etc.
Now, I want to vote for two and vote for him every two seconds. My goal is to keep the total number of votes for two greater than three (of course, whatever you think)
Then start writing code. If you are used to jquery, you can also use it directly in the console.
Or if the older version does not support jquery, just add:
in the codejavascript:(function(url) { var s = document.createElement('script'); s.src = url; (document.getElementsByTagName('head')[0] || document.getElementsByTagName('body')[0]).appendChild(s); })('http://code.jquery.com/jquery-2.1.3.js');
Officially begins
1. Write a general ticket brushing function
function brushVotes(){ //刷票函数 var t = setInterval(function(){ var three_num = $("#person3>p>span").text(); //three票数 var two_num = $("#person2>p>span").text(); // two票数 console.info(two_num+" "+three_num); if(two_num - three_num < 5){ //要保持领先5票的优势 $("#person2>button").click().attr("disabled",false); //触发投票的事件click,投完后记得把投票权限拿回来 } if(two_num - three_num == 5){ //5票领先了就此打住 clearInterval(t); } },2000); }
Use a timer to execute the voting event every two seconds. It will be suspended after leading by 5 votes.
2. Call the ticket brushing function
The initial call is once, and when you click Run, the script will be executed naturally.
Then monitor the change of three votes and perform binding processing.
Ordinary change events can only be supported by those form-related tag elements. Of course we can change the span in the votes to the input tag and let it have the onchange event.
But the page belongs to someone else and we cannot change it.
So I searched and searched, and finally found a way to detect content changes in other tags such as div span and so on. If you want to understand this method in depth welcome
brushVotes(); // 刷票 $("#person3>p>span").bind('DOMNodeInserted', function(e) { //three改变则 触发 brushVotes(); //继续刷票 });
In this way, if the number of three votes changes, it will automatically be triggered to continue to vote.
Full script
javascript:(function(url) { var s = document.createElement('script'); s.src = url; (document.getElementsByTagName('head')[0] || document.getElementsByTagName('body')[0]).appendChild(s); })('http://code.jquery.com/jquery-2.1.3.js'); brushVotes(); // 刷票 $("#person3>p>span").bind('DOMNodeInserted', function(e) { //three改变则 触发 brushVotes(); //继续刷票 }); function brushVotes(){ //刷票函数 var t = setInterval(function(){ var three_num = $("#person3>p>span").text(); //three票数 var two_num = $("#person2>p>span").text(); // two票数 console.info(two_num+" "+three_num); if(two_num - three_num < 5){ //要保持领先5票的优势 $("#person2>button").click().attr("disabled",false); //触发投票的事件click,投完后记得把投票权限拿回来 } if(two_num - three_num == 5){ //5票领先了就此打住 clearInterval(t); } },2000); }
Finally, simulate it
1. Enter the voting page, call up Firebug, and type the complete code in the code input area on the right side of the console
2. Then click Run in the upper left corner first, and let two start from zero to 5. It is 5 votes ahead of three
Alert until 5 times
3. Then, someone simulated voting for three and clicked three’s button
4. It is detected that the number of three votes has changed, and two continues to vote
5. Finally, after reaching 6 votes, it was suspended again
-------------------------------------------------- -------------------------------------------------- -----
This is a simple ticket brushing script implementation.
The most important thing through this is to learn how to use your own scripts to operate other people's pages. Of course, this is not the same thing as so-called script injection..
All we do is simulate normal page events and trigger them manually.
Through this mechanism, you can not only swipe votes in the voting system, but also perform violent verification and login... But you will lose a lot when encountering the verification code. You can also use the so-called train ticket grabbing script... But that It should involve more knowledge.

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
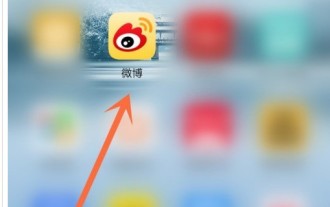
How to vote on Weibo Night_How to vote on Weibo Night
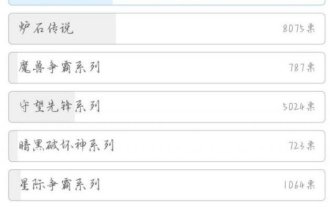
World of Warcraft: Multiple media voting results, player return rate is amazing, even if the point card increase is 10 times, you still want to play
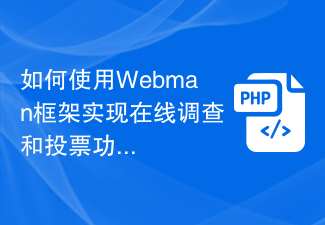
How to use Webman framework to implement online survey and voting functions?
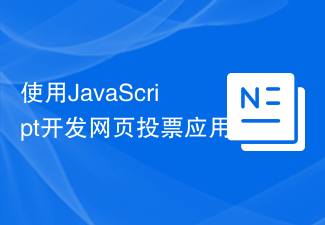
Developing a web voting application using JavaScript
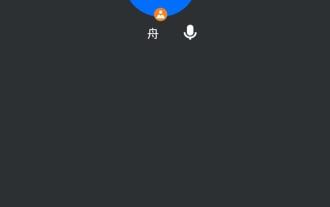
A simple tutorial for voting in Tencent Conference
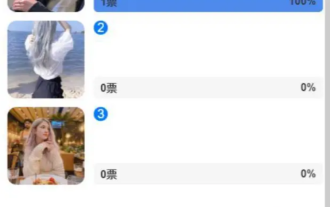
How does Voting Helper see people who voted?
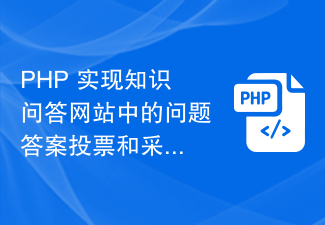
PHP implements the voting and adoption functions of question answers in the knowledge question and answer website.
