


JavaScript method to delete elements with specified value from array_javascript tips
The example in this article describes how to delete elements with specified values from an array using JavaScript. Share it with everyone for your reference. The specific analysis is as follows:
The following code uses two methods to delete elements of the array. The first one defines a separate function, and the second one defines a removeByValue method for the Array object. The call is very simple
Define function removeByValue to delete elements
function removeByValue(arr, val) { for(var i=0; i<arr.length; i++) { if(arr[i] == val) { arr.splice(i, 1); break; } } } var somearray = ["mon", "tue", "wed", "thur"] removeByValue(somearray, "tue"); //somearray will now have "mon", "wed", "thur"
Add the corresponding method to the array object, and the call becomes easier. Directly call the removeByValue method of the array to delete the specified element
Array.prototype.removeByValue = function(val) { for(var i=0; i<this.length; i++) { if(this[i] == val) { this.splice(i, 1); break; } } } var somearray = ["mon", "tue", "wed", "thur"] somearray.removeByValue("tue"); //somearray will now have "mon", "wed", "thur"
I hope this article will be helpful to everyone’s JavaScript programming design.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


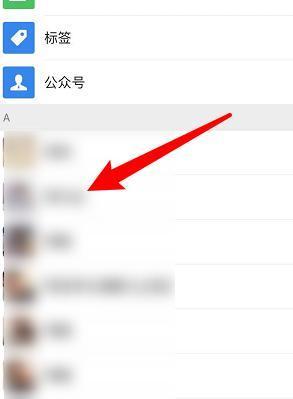
Unfortunately, people often delete certain contacts accidentally for some reasons. WeChat is a widely used social software. To help users solve this problem, this article will introduce how to retrieve deleted contacts in a simple way. 1. Understand the WeChat contact deletion mechanism. This provides us with the possibility to retrieve deleted contacts. The contact deletion mechanism in WeChat removes them from the address book, but does not delete them completely. 2. Use WeChat’s built-in “Contact Book Recovery” function. WeChat provides “Contact Book Recovery” to save time and energy. Users can quickly retrieve previously deleted contacts through this function. 3. Enter the WeChat settings page and click the lower right corner, open the WeChat application "Me" and click the settings icon in the upper right corner to enter the settings page.
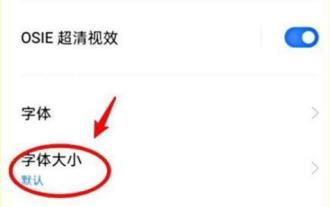
Setting font size has become an important personalization requirement as mobile phones become an important tool in people's daily lives. In order to meet the needs of different users, this article will introduce how to improve the mobile phone use experience and adjust the font size of the mobile phone through simple operations. Why do you need to adjust the font size of your mobile phone - Adjusting the font size can make the text clearer and easier to read - Suitable for the reading needs of users of different ages - Convenient for users with poor vision to use the font size setting function of the mobile phone system - How to enter the system settings interface - In Find and enter the "Display" option in the settings interface - find the "Font Size" option and adjust it. Adjust the font size with a third-party application - download and install an application that supports font size adjustment - open the application and enter the relevant settings interface - according to the individual
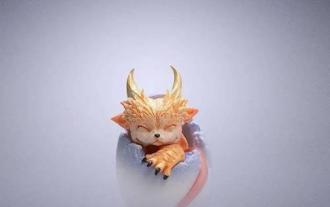
Mobile games have become an integral part of people's lives with the development of technology. It has attracted the attention of many players with its cute dragon egg image and interesting hatching process, and one of the games that has attracted much attention is the mobile version of Dragon Egg. To help players better cultivate and grow their own dragons in the game, this article will introduce to you how to hatch dragon eggs in the mobile version. 1. Choose the appropriate type of dragon egg. Players need to carefully choose the type of dragon egg that they like and suit themselves, based on the different types of dragon egg attributes and abilities provided in the game. 2. Upgrade the level of the incubation machine. Players need to improve the level of the incubation machine by completing tasks and collecting props. The level of the incubation machine determines the hatching speed and hatching success rate. 3. Collect the resources required for hatching. Players need to be in the game
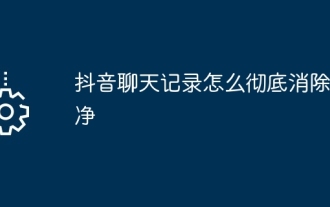
1. Open the Douyin app, click [Message] at the bottom of the interface, and click the chat conversation entry that needs to be deleted. 2. Long press any chat record, click [Multiple Select], and check the chat records you want to delete. 3. Click the [Delete] button in the lower right corner and select [Confirm deletion] in the pop-up window to permanently delete these records.
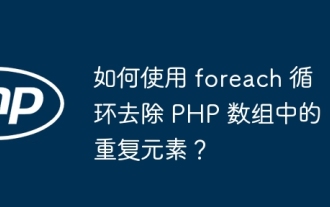
The method of using a foreach loop to remove duplicate elements from a PHP array is as follows: traverse the array, and if the element already exists and the current position is not the first occurrence, delete it. For example, if there are duplicate records in the database query results, you can use this method to remove them and obtain results without duplicate records.
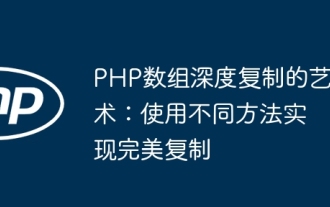
Methods for deep copying arrays in PHP include: JSON encoding and decoding using json_decode and json_encode. Use array_map and clone to make deep copies of keys and values. Use serialize and unserialize for serialization and deserialization.

The performance comparison of PHP array key value flipping methods shows that the array_flip() function performs better than the for loop in large arrays (more than 1 million elements) and takes less time. The for loop method of manually flipping key values takes a relatively long time.
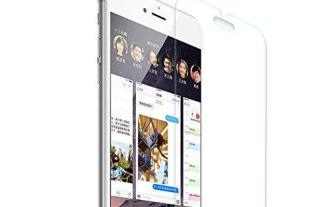
Mobile phone film has become one of the indispensable accessories with the popularity of smartphones. To extend its service life, choose a suitable mobile phone film to protect the mobile phone screen. To help readers choose the most suitable mobile phone film for themselves, this article will introduce several key points and techniques for purchasing mobile phone film. Understand the materials and types of mobile phone films: PET film, TPU, etc. Mobile phone films are made of a variety of materials, including tempered glass. PET film is relatively soft, tempered glass film has good scratch resistance, and TPU has good shock-proof performance. It can be decided based on personal preference and needs when choosing. Consider the degree of screen protection. Different types of mobile phone films have different degrees of screen protection. PET film mainly plays an anti-scratch role, while tempered glass film has better drop resistance. You can choose to have better
