Method to create mouseover effect based on jQuery_jquery
The example in this article describes how to create a mouse hover effect based on jQuery. Share it with everyone for your reference. The specific implementation method is as follows:
1. Create HTML:
<ul> <li><a href="/tv"><img src="images/tv_off.gif" class="mainnav"></a></li> </ul>
2. Select the class of .mainnav:
$(".mainnav").hover( function () { }, function () { } );
3. Create variable imgPath and specify image SRC:
$(".mainnav").hover( function () { // Grab the source var imgPath = $(this).attr("src"); }, function () { // Grab the source var imgPath = $(this).attr("src"); } );
4. Find the string off and replace it with on:
$(".mainnav").hover( function () { // Grab the source var imgPath = $(this).attr("src"); // Replace the path in the source $(this).attr("src",imgPath.replace("off", "on")); }, function () { // Grab the source var imgPath = $(this).attr("src"); // Replace the path in the source $(this).attr("src",imgPath.replace("on", "off")); } );
I hope this article will be helpful to everyone’s jQuery programming.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
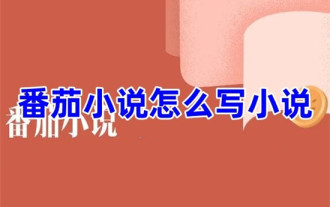
Tomato Novel is a very popular novel reading software. We often have new novels and comics to read in Tomato Novel. Every novel and comic is very interesting. Many friends also want to write novels. Earn pocket money and edit the content of the novel you want to write into text. So how do we write the novel in it? My friends don’t know, so let’s go to this site together. Let’s take some time to look at an introduction to how to write a novel. Share the Tomato novel tutorial on how to write a novel. 1. First open the Tomato free novel app on your mobile phone and click on Personal Center - Writer Center. 2. Jump to the Tomato Writer Assistant page - click on Create a new book at the end of the novel.
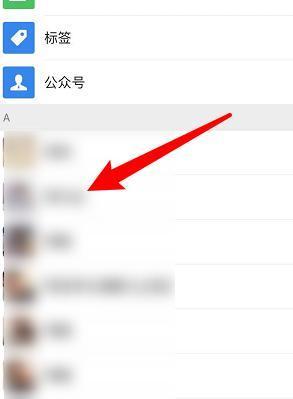
Unfortunately, people often delete certain contacts accidentally for some reasons. WeChat is a widely used social software. To help users solve this problem, this article will introduce how to retrieve deleted contacts in a simple way. 1. Understand the WeChat contact deletion mechanism. This provides us with the possibility to retrieve deleted contacts. The contact deletion mechanism in WeChat removes them from the address book, but does not delete them completely. 2. Use WeChat’s built-in “Contact Book Recovery” function. WeChat provides “Contact Book Recovery” to save time and energy. Users can quickly retrieve previously deleted contacts through this function. 3. Enter the WeChat settings page and click the lower right corner, open the WeChat application "Me" and click the settings icon in the upper right corner to enter the settings page.
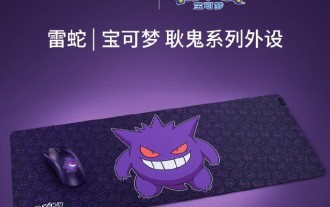
According to news from this site on July 12, Razer today announced the launch of the Razer|Pokémon Gengar wireless mouse and mouse pad. The single product prices are 1,299 yuan and 299 yuan respectively, and the package price including the two products is 1,549 yuan. This is not the first time that Razer has launched Gengar co-branded peripheral products. In 2023, Razer launched the Gengar-style Yamata Orochi V2 gaming mouse. The two new products launched this time all use a dark purple background similar to the appearance of the Ghost, Ghost, and Gengar families. They are printed with the outlines of these three Pokémon and Poké Balls, with the character Gengar in the middle. A large, colorful image of a classic ghost-type Pokémon. This site found that the Razer|Pokémon Gengar wireless mouse is based on the previously released Viper V3 Professional Edition. Its overall weight is 55g and equipped with Razer’s second-generation FOC
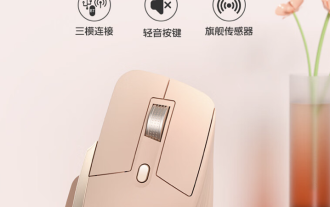
According to news from this website on March 31, HP recently launched a Professor1 three-mode Bluetooth mouse on JD.com, available in black and white milk tea colors, with an initial price of 99 yuan, and a deposit of 10 yuan is required. According to reports, this mouse weighs 106 grams, adopts ergonomic design, measures 127.02x79.59x51.15mm, has seven optional 4000DPI levels, is equipped with a Blue Shadow RAW3220 sensor, and uses a 650 mAh battery. It is said that it can be used on a single charge. 2 months. The mouse parameter information attached to this site is as follows:
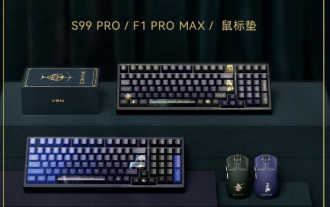
According to news from this site on August 12, VGN launched the co-branded "Elden Ring" keyboard and mouse series on August 6, including keyboards, mice and mouse pads, designed with a customized theme of Lani/Faded One. The current series of products It has been put on JD.com, priced from 99 yuan. The co-branded new product information attached to this site is as follows: VGN丨Elden Law Ring S99PRO Keyboard This keyboard uses a pure aluminum alloy shell, supplemented by a five-layer silencer structure, uses a GASKET leaf spring structure, has a single-key slotted PCB, and the original height PBT material Keycaps, aluminum alloy personalized backplane; supports three-mode connection and SMARTSPEEDX low-latency technology; connected to VHUB, it can manage multiple devices in one stop, starting at 549 yuan. VGN丨Elden French Ring F1PROMAX wireless mouse the mouse
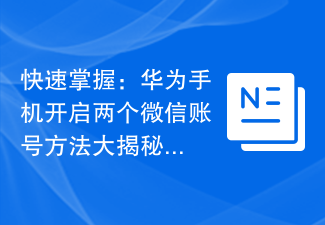
In today's society, mobile phones have become an indispensable part of our lives. As an important tool for our daily communication, work, and life, WeChat is often used. However, it may be necessary to separate two WeChat accounts when handling different transactions, which requires the mobile phone to support logging in to two WeChat accounts at the same time. As a well-known domestic brand, Huawei mobile phones are used by many people. So what is the method to open two WeChat accounts on Huawei mobile phones? Let’s reveal the secret of this method. First of all, you need to use two WeChat accounts at the same time on your Huawei mobile phone. The easiest way is to
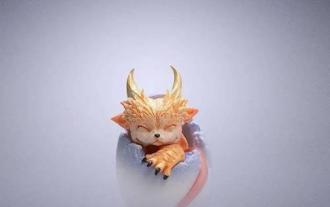
Mobile games have become an integral part of people's lives with the development of technology. It has attracted the attention of many players with its cute dragon egg image and interesting hatching process, and one of the games that has attracted much attention is the mobile version of Dragon Egg. To help players better cultivate and grow their own dragons in the game, this article will introduce to you how to hatch dragon eggs in the mobile version. 1. Choose the appropriate type of dragon egg. Players need to carefully choose the type of dragon egg that they like and suit themselves, based on the different types of dragon egg attributes and abilities provided in the game. 2. Upgrade the level of the incubation machine. Players need to improve the level of the incubation machine by completing tasks and collecting props. The level of the incubation machine determines the hatching speed and hatching success rate. 3. Collect the resources required for hatching. Players need to be in the game
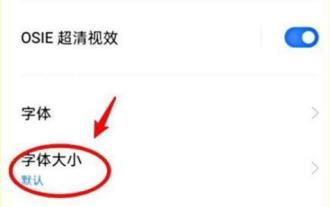
Setting font size has become an important personalization requirement as mobile phones become an important tool in people's daily lives. In order to meet the needs of different users, this article will introduce how to improve the mobile phone use experience and adjust the font size of the mobile phone through simple operations. Why do you need to adjust the font size of your mobile phone - Adjusting the font size can make the text clearer and easier to read - Suitable for the reading needs of users of different ages - Convenient for users with poor vision to use the font size setting function of the mobile phone system - How to enter the system settings interface - In Find and enter the "Display" option in the settings interface - find the "Font Size" option and adjust it. Adjust the font size with a third-party application - download and install an application that supports font size adjustment - open the application and enter the relevant settings interface - according to the individual
