Python实现的二维码生成小软件
前几天,我估摸着做一个能生成QR Code小程序,并能用wxPython在屏幕上显示出来。当然,我想用纯Python实现,观望了一会后,我找到了三个候选:
github 上的 python-qrcode
sourceforge上的 pyqrcode
Goolge code 上的 pyqrnative
我尝试了python-qrcode以及pyqrnative,因为它们能够运行在Windows/Mac/Linux。也不需要依赖额外的其他库除了Python图像库。pyqrcode项目需要其他一些先决条件,并且不能在Windows上运行,所以我不想与之纠缠了。我最后使用了一些以前写过的一个Photo Viewer程序的代码,然后稍微地修改了一下,就成了QRCode的查看器了。
开始
正如我上面提到的,你只需要Python图像库,GUI部分我们将使用wxPython。python-qrcode相比pyqrnative生成图片更快,并包含了你见过的大多数QR码类型。
生成 QR Codes
当你准备好所有需要的以后,你可以运行以下代码,看看Python做了些啥:
import os import wx try: import qrcode except ImportError: qrcode = None try: import PyQRNative except ImportError: PyQRNative = None ######################################################################## class QRPanel(wx.Panel): """""" #---------------------------------------------------------------------- def __init__(self, parent): """Constructor""" wx.Panel.__init__(self, parent=parent) self.photo_max_size = 240 sp = wx.StandardPaths.Get() self.defaultLocation = sp.GetDocumentsDir() img = wx.EmptyImage(240,240) self.imageCtrl = wx.StaticBitmap(self, wx.ID_ANY, wx.BitmapFromImage(img)) qrDataLbl = wx.StaticText(self, label="Text to turn into QR Code:") self.qrDataTxt = wx.TextCtrl(self, value="http://www.mousevspython.com", size=(200,-1)) instructions = "Name QR image file" instructLbl = wx.StaticText(self, label=instructions) self.qrPhotoTxt = wx.TextCtrl(self, size=(200,-1)) browseBtn = wx.Button(self, label='Change Save Location') browseBtn.Bind(wx.EVT_BUTTON, self.onBrowse) defLbl = "Default save location: " + self.defaultLocation self.defaultLocationLbl = wx.StaticText(self, label=defLbl) qrcodeBtn = wx.Button(self, label="Create QR with qrcode") qrcodeBtn.Bind(wx.EVT_BUTTON, self.onUseQrcode) pyQRNativeBtn = wx.Button(self, label="Create QR with PyQRNative") pyQRNativeBtn.Bind(wx.EVT_BUTTON, self.onUsePyQR) # Create sizer self.mainSizer = wx.BoxSizer(wx.VERTICAL) qrDataSizer = wx.BoxSizer(wx.HORIZONTAL) locationSizer = wx.BoxSizer(wx.HORIZONTAL) qrBtnSizer = wx.BoxSizer(wx.VERTICAL) qrDataSizer.Add(qrDataLbl, 0, wx.ALL, 5) qrDataSizer.Add(self.qrDataTxt, 1, wx.ALL|wx.EXPAND, 5) self.mainSizer.Add(wx.StaticLine(self, wx.ID_ANY), 0, wx.ALL|wx.EXPAND, 5) self.mainSizer.Add(qrDataSizer, 0, wx.EXPAND) self.mainSizer.Add(self.imageCtrl, 0, wx.ALL, 5) locationSizer.Add(instructLbl, 0, wx.ALL, 5) locationSizer.Add(self.qrPhotoTxt, 0, wx.ALL, 5) locationSizer.Add(browseBtn, 0, wx.ALL, 5) self.mainSizer.Add(locationSizer, 0, wx.ALL, 5) self.mainSizer.Add(self.defaultLocationLbl, 0, wx.ALL, 5) qrBtnSizer.Add(qrcodeBtn, 0, wx.ALL, 5) qrBtnSizer.Add(pyQRNativeBtn, 0, wx.ALL, 5) self.mainSizer.Add(qrBtnSizer, 0, wx.ALL|wx.CENTER, 10) self.SetSizer(self.mainSizer) self.Layout() #---------------------------------------------------------------------- def onBrowse(self, event): """""" dlg = wx.DirDialog(self, "Choose a directory:", style=wx.DD_DEFAULT_STYLE) if dlg.ShowModal() == wx.ID_OK: path = dlg.GetPath() self.defaultLocation = path self.defaultLocationLbl.SetLabel("Save location: %s" % path) dlg.Destroy() #---------------------------------------------------------------------- def onUseQrcode(self, event): """ https://github.com/lincolnloop/python-qrcode """ qr = qrcode.QRCode(version=1, box_size=10, border=4) qr.add_data(self.qrDataTxt.GetValue()) qr.make(fit=True) x = qr.make_image() qr_file = os.path.join(self.defaultLocation, self.qrPhotoTxt.GetValue() + ".jpg") img_file = open(qr_file, 'wb') x.save(img_file, 'JPEG') img_file.close() self.showQRCode(qr_file) #---------------------------------------------------------------------- def onUsePyQR(self, event): """ http://code.google.com/p/pyqrnative/ """ qr = PyQRNative.QRCode(20, PyQRNative.QRErrorCorrectLevel.L) qr.addData(self.qrDataTxt.GetValue()) qr.make() im = qr.makeImage() qr_file = os.path.join(self.defaultLocation, self.qrPhotoTxt.GetValue() + ".jpg") img_file = open(qr_file, 'wb') im.save(img_file, 'JPEG') img_file.close() self.showQRCode(qr_file) #---------------------------------------------------------------------- def showQRCode(self, filepath): """""" img = wx.Image(filepath, wx.BITMAP_TYPE_ANY) # scale the image, preserving the aspect ratio W = img.GetWidth() H = img.GetHeight() if W > H: NewW = self.photo_max_size NewH = self.photo_max_size * H / W else: NewH = self.photo_max_size NewW = self.photo_max_size * W / H img = img.Scale(NewW,NewH) self.imageCtrl.SetBitmap(wx.BitmapFromImage(img)) self.Refresh() ######################################################################## class QRFrame(wx.Frame): """""" #---------------------------------------------------------------------- def __init__(self): """Constructor""" wx.Frame.__init__(self, None, title="QR Code Viewer", size=(550,500)) panel = QRPanel(self) if __name__ == "__main__": app = wx.App(False) frame = QRFrame() frame.Show() app.MainLoop()
python-qrcode生成效果图:
PyQRNative生成效果图:

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


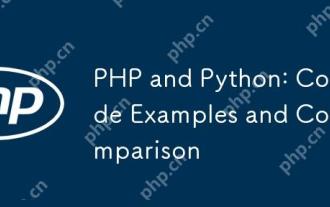
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
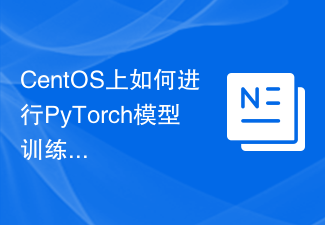
Efficient training of PyTorch models on CentOS systems requires steps, and this article will provide detailed guides. 1. Environment preparation: Python and dependency installation: CentOS system usually preinstalls Python, but the version may be older. It is recommended to use yum or dnf to install Python 3 and upgrade pip: sudoyumupdatepython3 (or sudodnfupdatepython3), pip3install--upgradepip. CUDA and cuDNN (GPU acceleration): If you use NVIDIAGPU, you need to install CUDATool
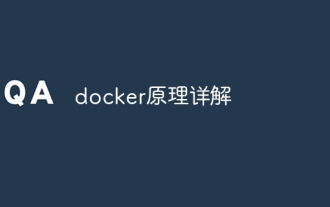
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
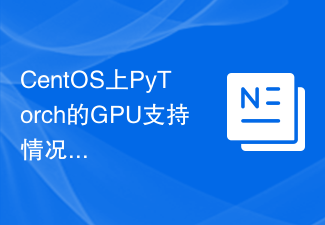
Enable PyTorch GPU acceleration on CentOS system requires the installation of CUDA, cuDNN and GPU versions of PyTorch. The following steps will guide you through the process: CUDA and cuDNN installation determine CUDA version compatibility: Use the nvidia-smi command to view the CUDA version supported by your NVIDIA graphics card. For example, your MX450 graphics card may support CUDA11.1 or higher. Download and install CUDAToolkit: Visit the official website of NVIDIACUDAToolkit and download and install the corresponding version according to the highest CUDA version supported by your graphics card. Install cuDNN library:
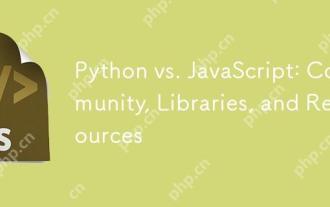
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.

When selecting a PyTorch version under CentOS, the following key factors need to be considered: 1. CUDA version compatibility GPU support: If you have NVIDIA GPU and want to utilize GPU acceleration, you need to choose PyTorch that supports the corresponding CUDA version. You can view the CUDA version supported by running the nvidia-smi command. CPU version: If you don't have a GPU or don't want to use a GPU, you can choose a CPU version of PyTorch. 2. Python version PyTorch

MinIO Object Storage: High-performance deployment under CentOS system MinIO is a high-performance, distributed object storage system developed based on the Go language, compatible with AmazonS3. It supports a variety of client languages, including Java, Python, JavaScript, and Go. This article will briefly introduce the installation and compatibility of MinIO on CentOS systems. CentOS version compatibility MinIO has been verified on multiple CentOS versions, including but not limited to: CentOS7.9: Provides a complete installation guide covering cluster configuration, environment preparation, configuration file settings, disk partitioning, and MinI
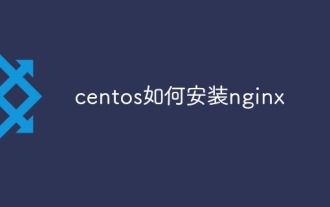
CentOS Installing Nginx requires following the following steps: Installing dependencies such as development tools, pcre-devel, and openssl-devel. Download the Nginx source code package, unzip it and compile and install it, and specify the installation path as /usr/local/nginx. Create Nginx users and user groups and set permissions. Modify the configuration file nginx.conf, and configure the listening port and domain name/IP address. Start the Nginx service. Common errors need to be paid attention to, such as dependency issues, port conflicts, and configuration file errors. Performance optimization needs to be adjusted according to the specific situation, such as turning on cache and adjusting the number of worker processes.
