Detailed explanation of inheritance in JavaScript_javascript skills
May 16, 2016 pm 04:14 PMThe concept of js inheritance
The following two inheritance methods are commonly used in js:
Prototype chain inheritance (inheritance between objects)
Class inheritance (inheritance between constructors)
Since js is not a truly object-oriented language like java, js is based on objects and has no concept of classes. Therefore, if you want to implement inheritance, you can use the prototype mechanism of js or the apply and call methods
In object-oriented languages, we use classes to create a custom object. However, everything in js is an object, so how to create a custom object? This requires the use of js prototype:
We can simply think of prototype as a template. The newly created custom objects are all copies of this template (prototype) (actually not copies but links, but this link is invisible. New There is an invisible __Proto__ pointer inside the instantiated object, pointing to the prototype object).
JS can simulate the functions of classes through constructors and prototypes. In addition, the implementation of js class inheritance also relies on the prototype chain.
Prototypal inheritance and class inheritance
Classic inheritance is calling the constructor of the supertype inside the constructor of the subtype.
Strict class inheritance is not very common, and is usually used in combination:
function Super(){
This.colors=["red","blue"];
}
function Sub(){
Super.call(this);
}
Prototypal inheritance is to create new objects with the help of existing objects, and point the prototype of the subclass to the parent class, which is equivalent to joining the prototype chain of the parent class
Prototype chain inheritance
In order for the subclass to inherit the attributes (including methods) of the parent class, you first need to define a constructor. Then, assign the new instance of the parent class to the constructor's prototype. The code is as follows:
<script>
Function Parent(){
This.name = 'mike';
}
function Child(){
This.age = 12;
}
Child.prototype = new Parent();//Child inherits Parent and forms a chain through the prototype
var test = new Child();
alert(test.age);
alert(test.name);//Get the inherited attributes
//Continue prototype chain inheritance
Function Brother(){ //brother construction
This.weight = 60;
}
Brother.prototype = new Child();//Continue prototype chain inheritance
var brother = new Brother();
alert(brother.name);//Inherits Parent and Child, pops up mike
alert(brother.age);//pop-up 12
</script>
The above prototype chain inheritance is missing a link, that is Object. All constructors inherit from Object. Inheriting Object is done automatically and does not require us to inherit manually. So what is their affiliation?
Determine the relationship between prototype and instance
The relationship between prototypes and instances can be determined in two ways. Operator instanceof and isPrototypeof() methods:
alert(brother instanceof Object)//true
alert(test instanceof Brother);//false, test is the super class of brother
alert(brother instanceof Child);//true
alert(brother instanceof Parent);//true
As long as it is a prototype that appears in the prototype chain, it can be said to be the prototype of the instance derived from the prototype chain. Therefore, the isPrototypeof() method will also return true
In js, the inherited function is called the super type (parent class, base class is also acceptable), and the inherited function is called the subtype (subclass, derived class). There are two main problems with using prototypal inheritance:
First, literal overriding of the prototype will break the relationship and use the prototype of the reference type, and the subtype cannot pass parameters to the supertype.
Pseudo classes solve the problem of reference sharing and the inability to pass parameters of super types. We can use the "borrowed constructor" technology
Borrow constructor (class inheritance)
<script>
Function Parent(age){
This.name = ['mike','jack','smith'];
This.age = age;
}
function Child(age){
Parent.call(this,age);
}
var test = new Child(21);
alert(test.age);//21
alert(test.name);//mike,jack,smith
Test.name.push('bill');
alert(test.name);//mike,jack,smith,bill
</script>
Although borrowing constructors solves the two problems just mentioned, without a prototype, reuse is impossible, so we need a prototype chain to borrow constructors. This pattern is called combined inheritance
Combined inheritance
<script>
Function Parent(age){
This.name = ['mike','jack','smith'];
This.age = age;
}
Parent.prototype.run = function () {
return this.name ' are both' this.age;
};
Function Child(age){
Parent.call(this,age);//Object impersonation, passing parameters to the super type
}
Child.prototype = new Parent();//Prototype chain inheritance
var test = new Child(21);//You can also write new Parent(21)
alert(test.run());//mike,jack,smith are both21
</script>
Combined inheritance is a commonly used inheritance method. The idea behind it is to use the prototype chain to inherit prototype properties and methods, and to borrow constructors to inherit instance properties. In this way, function reuse is achieved by defining methods on the prototype, and each instance is guaranteed to have its own attributes.
Usage of call(): Call a method of an object and replace the current object with another object.
call([thisObj[,arg1[, arg2[, [,.argN]]]]])
Prototypal inheritance
This kind of inheritance uses prototypes to create new objects based on existing objects without creating custom types. It is called prototypal inheritance
<script>
Function obj(o){
function F(){}
F.prototype = o;
return new F();
}
var box = {
name : 'trigkit4',
arr : ['brother','sister','baba']
};
var b1 = obj(box);
alert(b1.name);//trigkit4
b1.name = 'mike';
alert(b1.name);//mike
alert(b1.arr);//brother,sister,baba
b1.arr.push('parents');
alert(b1.arr);//brother,sister,baba,parents
var b2 = obj(box);
alert(b2.name);//trigkit4
alert(b2.arr);//brother,sister,baba,parents
</script>
Prototypal inheritance first creates a temporary constructor inside the obj() function, then uses the incoming object as the prototype of this constructor, and finally returns a new instance of this temporary type.
Parasitic inheritance
This inheritance method combines the prototype factory pattern with the purpose of encapsulating the creation process.
<script>
Function create(o){
var f= obj(o);
f.run = function () {
return this.arr;//Similarly, the reference will be shared
};
return f;
}
</script>
Small problems with combined inheritance
Combined inheritance is the most commonly used inheritance pattern in js, but the supertype of combined inheritance will be called twice during use; once when creating the subtype, and the other time inside the subtype constructor
<script>
Function Parent(name){
This.name = name;
This.arr = ['brother','sister','parents'];
}
Parent.prototype.run = function () {
return this.name;
};
function Child(name,age){
Parent.call(this,age);//Second call
This.age = age;
}
Child.prototype = new Parent();//First call
</script>
The above code is the previous combination inheritance, so the parasitic combination inheritance solves the problem of two calls.
Parasitic Combinatorial Inheritance
<script>
Function obj(o){
function F(){}
F.prototype = o;
return new F();
}
Function create(parent,test){
var f = obj(parent.prototype);//Create object
f.constructor = test;//Enhancement object
}
function Parent(name){
This.name = name;
This.arr = ['brother','sister','parents'];
}
Parent.prototype.run = function () {
return this.name;
};
function Child(name,age){
Parent.call(this,name);
This.age =age;
}
inheritPrototype(Parent,Child);//Inheritance is achieved through here
var test = new Child('trigkit4',21);
Test.arr.push('nephew');
alert(test.arr);//
alert(test.run());//Only the method is shared
var test2 = new Child('jack',22);
alert(test2.arr);//Quotation problem resolution
</script>
call and apply
The global functions apply and call can be used to change the pointer of this in the function, as follows:
//Define a global function
Function foo() {
console.log(this.fruit);
}
// Define a global variable
var fruit = "apple";
// Customize an object
var pack = {
fruit: "orange"
};
// Equivalent to window.foo();
foo.apply(window); // "apple", at this time this is equal to window
//This in foo at this time === pack
foo.apply(pack); // "orange"

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
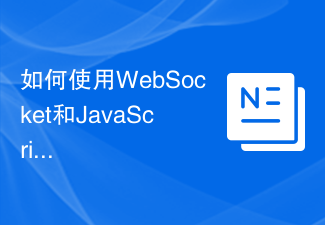
How to implement an online speech recognition system using WebSocket and JavaScript
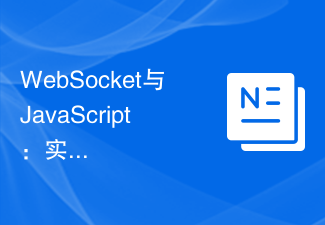
WebSocket and JavaScript: key technologies for implementing real-time monitoring systems
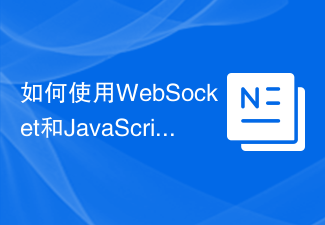
How to implement an online reservation system using WebSocket and JavaScript
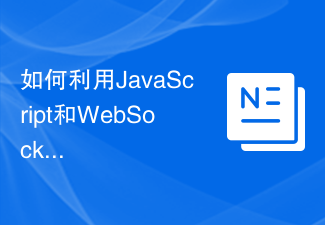
How to use JavaScript and WebSocket to implement a real-time online ordering system
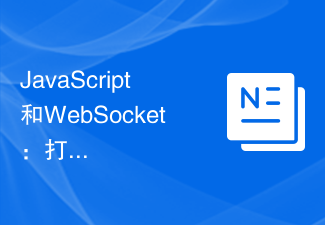
JavaScript and WebSocket: Building an efficient real-time weather forecasting system
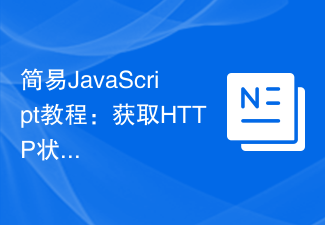
Simple JavaScript Tutorial: How to Get HTTP Status Code
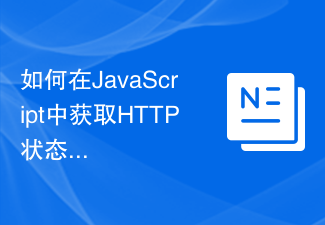
How to get HTTP status code in JavaScript the easy way

How to use insertBefore in javascript
