


Example analysis of cookie object usage in javascript_javascript skills
The examples in this article describe the usage of cookie objects in JavaScript. Share it with everyone for your reference. The details are as follows:
Attributes
name The only attribute that must be set, indicating the name of the cookie
expires Specifies the lifetime of the cookie. If it is not set, it will automatically expire when the browser is closed
path determines the availability of the cookie to the server for other web pages. Generally, the cookie is available to all pages in the same directory. When the path attribute is set, the cookie is only valid for all web pages under the specified path and sub-path
domain Many servers are composed of multiple servers. The domain attribute mainly sets multiple servers in the same domain to share a cookie. If web server a needs to share cookies with web server b, the domain attribute of a's cookie needs to be set to b, so that a The created cookie can be shared by a and b
secure Generally, websites that support SSL start with HTTPS. The secure attribute can set cookies that can only be accessed through HTTPS or other security protocols
Cookies are essentially strings
Generally speaking, cookies cannot contain special characters such as semicolons, commas, and spaces. However, these characters can be transmitted using encoding, that is, the special characters in the text string are converted into corresponding hexadecimal ASCII values. Use the encodeURI() function to convert text characters into a valid URI, and use the decodeURI() function to decode
Write cookie
1 2 3 4 5 6 |
|
Read cookie
1 2 3 4 5 6 7 |
|
Delete cookies
1 2 3 4 |
|
I hope this article will be helpful to everyone’s JavaScript programming design.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
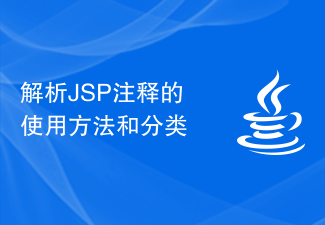
Classification and Usage Analysis of JSP Comments JSP comments are divided into two types: single-line comments: ending with, only a single line of code can be commented. Multi-line comments: starting with /* and ending with */, you can comment multiple lines of code. Single-line comment example Multi-line comment example/**This is a multi-line comment*Can comment on multiple lines of code*/Usage of JSP comments JSP comments can be used to comment JSP code to make it easier to read
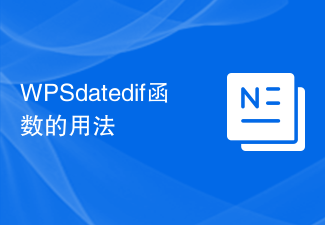
WPS is a commonly used office software suite, and the WPS table function is widely used for data processing and calculations. In the WPS table, there is a very useful function, the DATEDIF function, which is used to calculate the time difference between two dates. The DATEDIF function is the abbreviation of the English word DateDifference. Its syntax is as follows: DATEDIF(start_date,end_date,unit) where start_date represents the starting date.
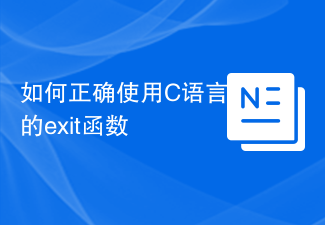
How to use the exit function in C language requires specific code examples. In C language, we often need to terminate the execution of the program early in the program, or exit the program under certain conditions. C language provides the exit() function to implement this function. This article will introduce the usage of exit() function and provide corresponding code examples. The exit() function is a standard library function in C language and is included in the header file. Its function is to terminate the execution of the program, and can take an integer
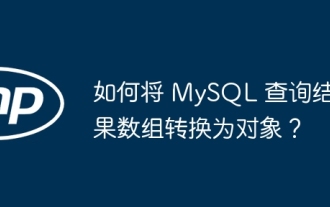
Here's how to convert a MySQL query result array into an object: Create an empty object array. Loop through the resulting array and create a new object for each row. Use a foreach loop to assign the key-value pairs of each row to the corresponding properties of the new object. Adds a new object to the object array. Close the database connection.
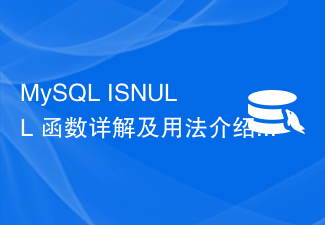
The ISNULL() function in MySQL is a function used to determine whether a specified expression or column is NULL. It returns a Boolean value, 1 if the expression is NULL, 0 otherwise. The ISNULL() function can be used in the SELECT statement or for conditional judgment in the WHERE clause. 1. The basic syntax of the ISNULL() function: ISNULL(expression) where expression is the expression to determine whether it is NULL or
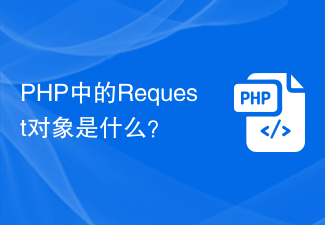
The Request object in PHP is an object used to handle HTTP requests sent by the client to the server. Through the Request object, we can obtain the client's request information, such as request method, request header information, request parameters, etc., so as to process and respond to the request. In PHP, you can use global variables such as $_REQUEST, $_GET, $_POST, etc. to obtain requested information, but these variables are not objects, but arrays. In order to process request information more flexibly and conveniently, you can
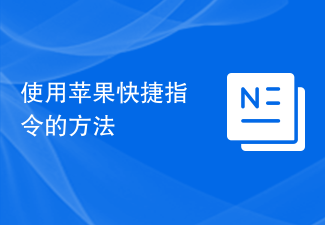
How to use Apple shortcut commands With the continuous development of technology, mobile phones have become an indispensable part of people's lives. Among many mobile phone brands, Apple mobile phones have always been loved by users for their stable systems and powerful functions. Among them, the Apple shortcut command function makes users’ mobile phone experience more convenient and efficient. Apple Shortcuts is a feature launched by Apple for iOS12 and later versions. It helps users simplify their mobile phone operations by creating and executing custom commands to achieve more efficient work and
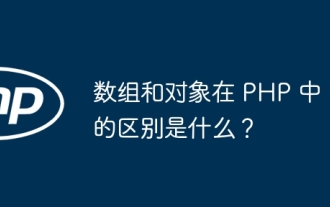
In PHP, an array is an ordered sequence, and elements are accessed by index; an object is an entity with properties and methods, created through the new keyword. Array access is via index, object access is via properties/methods. Array values are passed and object references are passed.
