


Example of jQuery plug-in that limits the size and format of uploaded files_jquery
The example in this article describes the jQuery plug-in that limits the size and format of uploaded files. Share it with everyone for your reference. The specific analysis is as follows:
When uploading files on the client, it is usually necessary to limit the size and format of the file. The most common method is to use a certain plug-in. Some mature plug-ins do have beautiful interfaces and powerful functions, but the only drawback is: sometimes you encounter Browser compatibility issues. In this article, we will write an "original" jQuery plug-in that can limit the size and format of uploaded files.
First, write a plug-in named checkFileTypeAndSize.js. Limit the file format by judging whether the suffix name of the current file is included in the suffix name array allowed by the preset; by judging whether the size of the current file under IE and other browsers is greater than the maximum file size allowed by the preset, to limit the file size; and provide callback functions for format errors, exceeding the limit size, and meeting conditions.
$.fn.checkFileTypeAndSize = function (options) {
//Default settings
var defaults = {
allowedExtensions: [],
maxSize: 1024, //The unit is KB, the default maximum file size is 1MB=1024KB
Success: function () { },
extensionerror: function () { },
sizeerror: function () { }
};
//Merge settings
options = $.extend(defaults, options);
//Traverse elements
return this.each(function () {
$(this).on('change', function () {
//Get file path
var filePath = $(this).val();
//File path in lower case letters
var fileLowerPath = filePath.toLowerCase();
//Get the suffix name of the file
var extension = fileLowerPath.substring(fileLowerPath.lastIndexOf('.') 1);
//Determine whether the suffix name is included in the preset and allowed suffix name array
If ($.inArray(extension, options.allowedExtensions) == -1) {
options.extensionerror();
$(this).focus();
} else {
try {
var size = 0;
If ($.browser.msie) {//ie old version of browser
var fileMgr = new ActiveXObject("Scripting.FileSystemObject");
var fileObj = fileMgr.getFile(filePath);
size = fileObj.size; //byte
size = size / 1024;//kb
//size = size / 1024;//mb
} else {//Other browsers
size = $(this)[0].files[0].size;//byte
size = size / 1024;//kb
//size = size / 1024;//mb
} If (size > options.maxSize) {
options.sizeerror();
} else {
options.success();
} } catch (e) {
alert("Error: "e);
}
}
});
});
};
})(jQuery);
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


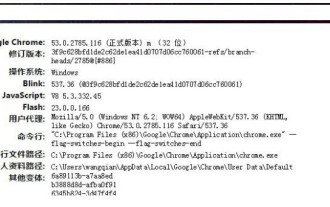
What is the Chrome plug-in extension installation directory? Under normal circumstances, the default installation directory of Chrome plug-in extensions is as follows: 1. The default installation directory location of chrome plug-ins in windowsxp: C:\DocumentsandSettings\username\LocalSettings\ApplicationData\Google\Chrome\UserData\Default\Extensions2. chrome in windows7 The default installation directory location of the plug-in: C:\Users\username\AppData\Local\Google\Chrome\User
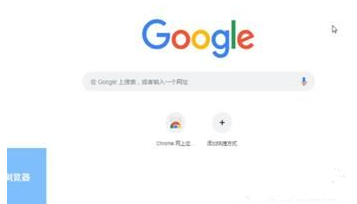
When users use the Edge browser, they may add some plug-ins to meet more of their needs. But when adding a plug-in, it shows that this plug-in is not supported. How to solve this problem? Today, the editor will share with you three solutions. Come and try it. Method 1: Try using another browser. Method 2: The Flash Player on the browser may be out of date or missing, causing the plug-in to be unsupported. You can download the latest version from the official website. Method 3: Press the "Ctrl+Shift+Delete" keys at the same time. Click "Clear Data" and reopen the browser.
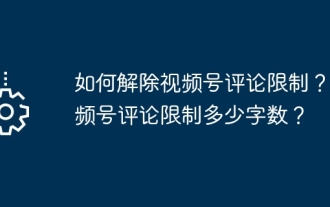
With the popularity of video accounts on social media, more and more people are beginning to use video accounts to share their daily lives, insights and stories. However, some users may experience comments being restricted, which can leave them confused and dissatisfied. 1. How to remove comment restrictions on video accounts? To lift the restriction on commenting on a video account, you must first ensure that the account has been properly registered and real-name authentication has been completed. Video accounts have requirements for comments. Only accounts that have completed real-name authentication can lift comment restrictions. If there are any abnormalities in the account, these issues need to be resolved before comment restrictions can be lifted. 2. Comply with the community standards of the video account. Video accounts have certain standards for comment content. If the comment involves illegal content, you will be restricted from speaking. To lift comment restrictions, you need to abide by the community of the video account
![How to increase disk size in VirtualBox [Guide]](https://img.php.cn/upload/article/000/887/227/171064142025068.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
We often encounter situations where the predefined disk size has no room for more data? If you need more virtual machine hard disk space at a later stage, you must expand the virtual hard disk and partitions. In this post, we will see how to increase disk size in VirtualBox. Increasing the disk size in VirtualBox It is important to note that you may want to back up your virtual hard disk files before performing these operations, as there is always the possibility of something going wrong. It is always a good practice to have backups. However, the process usually works fine, just make sure to shut down your machine before continuing. There are two ways to increase disk size in VirtualBox. Expand VirtualBox disk size using GUI using CL
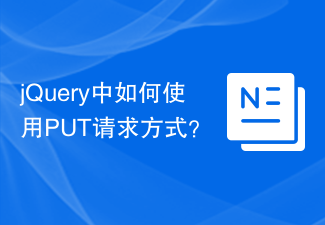
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
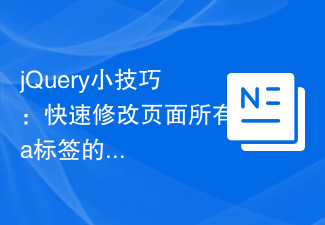
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
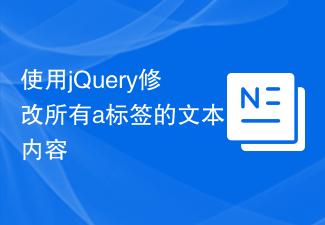
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
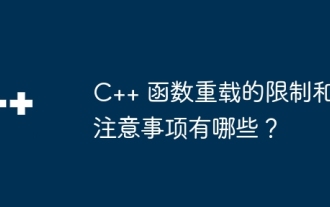
Restrictions on function overloading include: parameter types and orders must be different (when the number of parameters is the same), and default parameters cannot be used to distinguish overloading. In addition, template functions and non-template functions cannot be overloaded, and template functions with different template specifications can be overloaded. It's worth noting that excessive use of function overloading can affect readability and debugging, the compiler searches from the most specific to the least specific function to resolve conflicts.
