Backbone.js’ collection is just a simple ordered set model. By adapting models and collections, we can avoid placing data processing logic in our view layer. In addition, models and collections provide convenient ways to work with the backend to automatically mark Backbone.js views when data changes. In this way, it can be used in the following situations:
Model: Animal, Collection: Zoo
Typically your collection will only fit one model, but the model itself is not limited to the type of collection.
Model: person, Collection: Office
Model: person, Collection: Home
Here are examples of common models/collections:
var Music = Backbone.Model.extend({
initialize: function(){
console.log("Welcome to the music world");
}
});
var Album = Backbone.Collection.extend({
Model: Music
});
The above code tells us how to create a collection. But it doesn't tell us the process of manipulating collections with data. So, let’s explore the process:
var Music = Backbone.Model.extend({
defaults: {
name: "Not specified",
artist: "Not specified"
},
initialize: function(){
console.log("Welcome to the music world "); }
});
var Album = Backbone.Collection.extend({
model: Music
});
var music1 = new Music ({ id: 1 ,name: "How Bizarre", artist: "OMC" });
var music 2 = new Music ({id: 2, name: "What Hurts the Most", artist: "Rascal Flatts" });
var myAlbum = new Album([music 1, music 2]);
console.log( myAlbum.models );
Let’s take a look at the relationship between Backbone.js collections and other components:
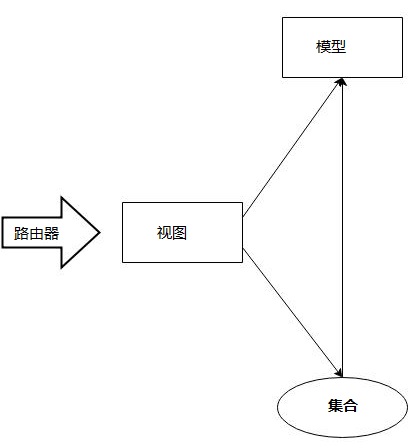
1. Add models to the collection
As we know, a collection is a collection of models. Therefore, we can add models on the collection. To add a model to the collection we can use the add method. We can also add models to the beginning of the collection - by using the unshift method.
var music3 = new Music({ id: 3, name: "Yes I Do", artist: "Rascal Flatts" });
Music.add(music3);
console.log('New Song Added');
console.log(JSON.stringify(Music));
2. Remove the model from the collection
Many times, we have the need to remove some specified data from the collection. To remove a model from the collection, we need to provide the model's id. If we want to replace the original collection with a complete new data set, we can use the reset method.
Music.remove(1);
console.log('How Bizarre removed...');
console.log(JSON.stringify(Music));
3. Get and Set
If we need to get a value from a collection elsewhere in the code, we can use the get method directly. At this point, we pass the ID value to the model with retrieval.
console.log(JSON.stringify(Music.get(2)));
The set method of collections has an interesting implementation. The set method performs "intelligent" updates of the collection by passing a list of models. If the model in the list is not already in the collection, it will be added to the collection. If the model is already in the collection, its properties will be merged. If the collection contains any model that is not part of the list, that model will be removed.
var Music = Backbone.Model.extend({
// This attribute should be set as a default
defaults: {
Name: ''
},
// Set the id attribute so that the collection
idAttribute: 'id'
});
var song = Backbone.Collection.extend({
model: Music
});
var models = [{
Name: 'OMC',
id: 1
}, {
Name: 'Flatts',
id: 2
}];
var collection = new song(models);
Collection.bind('add', function (model) {
alert('addb')
});
Collection.bind('remove', function () {
alert('add')
});
Models = [{
Name: 'OMC',
id:1
}, {
Name: 'Flatts',
id: 2
}, {
Name: ' Jackson ',
id: 3
}];
Collection.add(models);
});
As we can see above, beforehand we already had 2 models and when we added the 3rd model, the earlier model remained unchanged.
4. Constructor and initialization
When we create a collection, we can pass the model’s initialization array. Collection comparators can be added as an option. If the comparator option passed is false, then sorting is prevented. If we define an initialization function, this function will be called when the collection is created. Several options are described below and, if provided, are added directly to the collection: models and comparators.
var tabs = new TabSet([tab1, tab2, tab3]);
var spaces = new Backbone.Collection([], {
Model: Space
});
5. toJSON
The toJSO method returns an array containing the hash properties of each model in the collection. This method is usually used to serialize and persist the entire collection.
var song = new Backbone.Collection([
{name: "Flatts"},
{name: "OMC"},
{name: "Jackson"}
]);
alert(JSON.stringify(song));
6. Comparator
By default, collections do not have comparators. If we define a comparator, it can be used to maintain a certain ordering on the collection. This means that when a model is added, it is inserted into the collection at the appropriate location. The comparator can be defined using sortBy, or a string indicating the sorting attribute.
The sortBy comparator function gets a model and returns a number or string.
The sort comparator function gets two models. If the first model is before the second model, then it returns -1; if the two models are of the same level, then it returns 0; if the second model is before the first one model, then 1 is returned.
Let’s take a look at an example:
var student = Backbone.Model;
var students = new Backbone.Collection;
students.comparator = 'name';
students.add(new student({name: "name1", roll: 9}));
students.add(new student({name: "name2", roll: 5}));
students.add(new student({name: "name3", roll: 1}));
alert(students.pluck('roll'));
The collection of comparators is not automatically reordered, even if we modify the model's properties. Therefore, we should call sorting when we estimate that the sorting will be affected after modifying the model attributes.
7. Sorting
Should force the collection to be reordered when a model is added to the collection. To disable sorting when adding models to the collection, pass the {sort: false} parameter. The trigger that calls the sort checks this parameter.
sortByType: function(type) {
this.sortKey = type;
this.sort();
}
And view functions:
sortThingsByColumn: function(event) {
var type = event.currentTarget.classList[0]
this.collections.things.sortByType(type)
this.render()
}
8. Picking
Pluck: Picks an attribute from each model in the collection. This is equivalent to calling Map from an iterator and returning a single attribute.
var song = new Backbone.Collection([
{name: "Flatts"},
{name: "OMC"},
{name: "Jackson"}
]);
var names = songs.pluck("name");
alert(JSON.stringify(names));
9. Where
where: Returns an array of all models in the collection that match the passed attribute, using a filter.
var song = new Backbone.Collection([
{name: "Yes I Do", artist: "Flatts"},
{name: "How Bizarre", artist: "How Bizarre"},
{name: "What Hurts the Most", artist: "Flatts"},
]);
var artists = song.where({artist: "Flatts"});
alert(artists.length);
10. URL
Set the URL attribute in the collection, which will reference the location of the server. Models within the collection will use this URL to construct their own URLs.
var Songs = Backbone.Collection.extend({
url: '/songs'
});
var Songs = Backbone.Collection.extend({
url: function() {
Return this.document.url() '/songs';
}
});
11. Analysis
Parse: Called by Backbone when fetching, regardless of whether the server returns the collection's model. This function is passed the original response object and it should return an array of model properties that are added to the collection. The default implementation is no-op. Simply pass in a JSON response, override this operation with a pre-existing API, or better yet namespace the response.
var songs = Backbone.Collection.extend({
Parse: function(response) {
Return response.results;
}
});
12. Extraction
Fetch: Fetch the default model set of the collection from the server, and set them in the collection after retrieval. This option hash accepts a success or error callback, and it passes three parameters (collection, response, options). The model data is then returned from the server. It is used to set up merged extracted models.
Backbone.sync = function(method, model) {
alert(method ": " model.url);
};
var songs = new Backbone.Collection;
songs.url = '/songs';
songs.fetch();
As you can see above, there are so many methods in Backbone's collection alone, and mastering them can improve the quality of your code.