


Cookie encapsulation and usage guide made by javascript_javascript skills
1. Foreword
Previously, when using cookies, they were all operated in the form of document.cookie. Although the compatibility was good, it was troublesome. I personally like to make wheels, so I encapsulated a tool class for cookies. For a long time, I have liked writing code, but I don't really like text summaries, nor do I like writing fragmentary things. It seems that I have to change it.
2. Ideas
(1) How to encapsulate and what to encapsulate
How to encapsulate: Use native js to encapsulate it into a tool, so that it can be used anywhere. Encapsulating document.cookie is the best way, and all operations are based on document.cookie.
How to encapsulate it: It can exist in the form of an object and can be implemented using getter & setter methods.
(2) Which methods are encapsulated
get(), set(name, value, opts), remove(name), clear(), getCookies(), etc. I personally think that encapsulating so many methods is enough to use cookies.
3. Action
(1) Understand cookies. The essence of cookies is HTTP cookies. The object displayed on the client is document.cookie. For more information, you can read my code below and comment
(2) Above code: These codes should be very intuitive and can be compressed together with the project code. I think the opening comment below is the key point.
/*
* HTTP Cookie: stores session information
* Names and values must be RUL encoded when transmitted
* Cookies are bound to the specified domain name. Cookies cannot be shared with non-local domains, but cookies can be shared from the main site to sub-sites
* Cookies have some restrictions: for example, IE6 & IE6- are limited to 20; IE7 50; Opear 30... so cookies are generally set according to [must] needs
* The name of the cookie is not case-sensitive; it is also recommended to encode the cookie URL; the path is a good way to distinguish the cookie delivery under different circumstances; with a security mark cookie
* In the case of SSL, it is sent to the server, but not in http. It is recommended to set expires, domain, and path for cookies; each cookie is less than 4KB
* */
//Encapsulate cookies using getter and setter methods
(function(global){
//Get the cookie object, expressed as an object
Function getCookiesObj(){
var cookies = {};
If(document.cookie){
var objs = document.cookie.split('; ');
for(var i in objs){
var index = objs[i].indexOf('='),
name = objs[i].substr(0, index),
value = objs[i].substr(index 1, objs[i].length);
cookies[name] = value;
}
}
return cookies;
}
//Set cookie
Function set(name, value, opts){
//opts maxAge, path, domain, secure
If(name && value){
var cookie = encodeURIComponent(name) '=' encodeURIComponent(value);
//Optional parameters
if(opts){
If(opts.maxAge){
Cookie = '; max-age=' opts.maxAge;
}
If(opts.path){
Cookie = '; path=' opts.path;
}
If(opts.domain){
Cookie = '; domain=' opts.domain;
}
If(opts.secure){
cookie = '; secure';
}
}
document.cookie = cookie;
return cookie;
}else{
return '';
}
}
//Get cookie
Function get(name){
return decodeURIComponent(getCookiesObj()[name]) || null;
}
//Clear a cookie
Function remove(name){
if(getCookiesObj()[name]){
document.cookie = name '=; max-age=0';
}
}
//清除所有cookie
function clear(){
var cookies = getCookiesObj();
for(var key in cookies){
document.cookie = key '=; max-age=0';
}
}
//获取所有cookies
function getCookies(name){
return getCookiesObj();
}
//解决冲突
function noConflict(name){
if(name && typeof name === 'string'){
if(name && window['cookie']){
window[name] = window['cookie'];
delete window['cookie'];
return window[name];
}
}else{
return window['cookie'];
delete window['cookie'];
}
}
global['cookie'] = {
'getCookies': getCookies,
'set': set,
'get': get,
'remove': remove,
'clear': clear,
'noConflict': noConflict
};
})(window);
(3)example
(4)代码地址:https://github.com/vczero/cookie

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
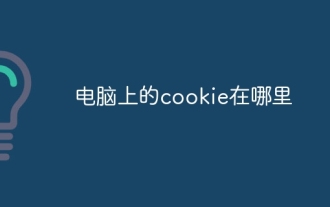
Cookies on your computer are stored in specific locations on your browser, depending on the browser and operating system used: 1. Google Chrome, stored in C:\Users\YourUsername\AppData\Local\Google\Chrome\User Data\Default \Cookies etc.
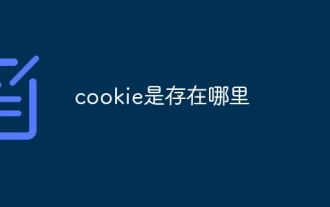
Cookies are usually stored in the cookie folder of the browser. Cookie files in the browser are usually stored in binary or SQLite format. If you open the cookie file directly, you may see some garbled or unreadable content, so it is best to use Use the cookie management interface provided by your browser to view and manage cookies.
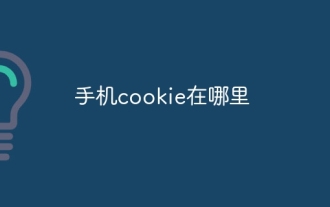
Cookies on the mobile phone are stored in the browser application of the mobile device: 1. On iOS devices, Cookies are stored in Settings -> Safari -> Advanced -> Website Data of the Safari browser; 2. On Android devices, Cookies Stored in Settings -> Site settings -> Cookies of Chrome browser, etc.
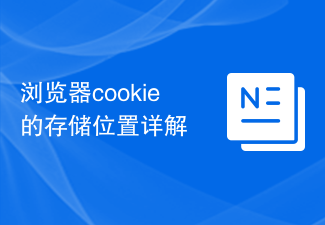
With the popularity of the Internet, we use browsers to surf the Internet have become a way of life. In the daily use of browsers, we often encounter situations where we need to enter account passwords, such as online shopping, social networking, emails, etc. This information needs to be recorded by the browser so that it does not need to be entered again the next time you visit. This is when cookies come in handy. What are cookies? Cookie refers to a small data file sent by the server to the user's browser and stored locally. It contains user behavior of some websites.
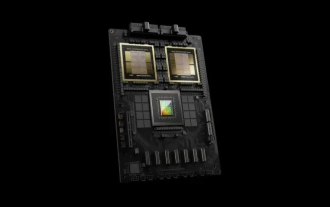
According to news from this site on April 17, TrendForce recently released a report, believing that demand for Nvidia's new Blackwell platform products is bullish, and is expected to drive TSMC's total CoWoS packaging production capacity to increase by more than 150% in 2024. NVIDIA Blackwell's new platform products include B-series GPUs and GB200 accelerator cards integrating NVIDIA's own GraceArm CPU. TrendForce confirms that the supply chain is currently very optimistic about GB200. It is estimated that shipments in 2025 are expected to exceed one million units, accounting for 40-50% of Nvidia's high-end GPUs. Nvidia plans to deliver products such as GB200 and B100 in the second half of the year, but upstream wafer packaging must further adopt more complex products.
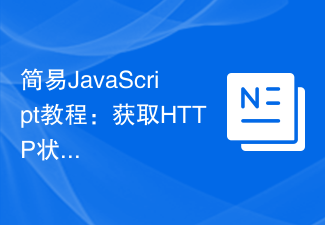
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
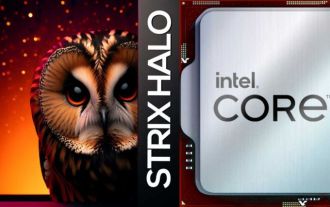
This website reported on July 9 that the AMD Zen5 architecture "Strix" series processors will have two packaging solutions. The smaller StrixPoint will use the FP8 package, while the StrixHalo will use the FP11 package. Source: videocardz source @Olrak29_ The latest revelation is that StrixHalo’s FP11 package size is 37.5mm*45mm (1687 square millimeters), which is the same as the LGA-1700 package size of Intel’s AlderLake and RaptorLake CPUs. AMD’s latest Phoenix APU uses an FP8 packaging solution with a size of 25*40mm, which means that StrixHalo’s F
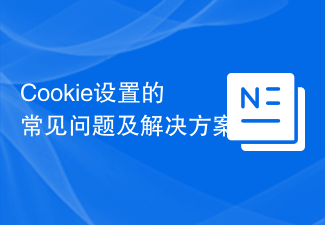
Common problems and solutions for cookie settings, specific code examples are required. With the development of the Internet, cookies, as one of the most common conventional technologies, have been widely used in websites and applications. Cookie, simply put, is a data file stored on the user's computer that can be used to store the user's information on the website, including login name, shopping cart contents, website preferences, etc. Cookies are an essential tool for developers, but at the same time, cookie settings are often encountered
