


node.js solves the problem of obtaining the real file type of images_node.js
Encountered a requirement: Suppose there is an image file, the real type is jpg, and someone is too lazy to copy the jpg directly and save it as a png file with the same name, so that there will be no problem when AS3 reads the file. But mobile phone C encountered problems when reading files - -!
Now you need to write a program to traverse the files in all folders and find files with "abnormal" file formats. Our resources are mainly gif, png, and jpg. At first, I found an article on the Internet: Obtain the file type mime-type based on the binary stream and file header, and then read the binary header information of the file to obtain its true file type. , compare with the file type obtained through the suffix name.
var fd = fs.openSync(new_file_path, 'r');
var buffer = new Buffer(8);
var mineType = mime.lookup(new_file_path);
var fileType = mime.extension(mineType);
fs.readSync(fd, buffer, 0, 8, 0);
var newBuf = buffer.slice(0, 4);
var head_1 = newBuf[0].toString(16);
var head_2 = newBuf[1].toString(16);
var head_3 = newBuf[2].toString(16);
var head_4 = newBuf[3].toString(16);
var head_iden = head_1 head_2;
var tempFileType = FILE_TYPE_CONFIG[head_iden];
if (!tempFileType) {
head_iden = head_3;
tempFileType = FILE_TYPE_CONFIG[head_iden];
if (!tempFileType) {
var msg = "Unknow fileType " new_file_path '-' fileType;
showLog(msg);
Continue;
}
}
if (tempFileType != fileType) {
var msg = "Error fileType" new_file_path '-' fileType '|' tempFileType '--correct image file format';
ShowLog(msg);
g_errorFileTypArr.push(msg);
}
Later, when I searched for information related to node image, I found this article: node.js module ranking>> (images)
Then I filtered out a module "node-imageinfo" and wrote an example for testing (deliberately directly changing the suffix of the jpg file to png):
If you are interested, you can study its source code:
function readUInt32(buffer, offset, bigEndian) {
if (buffer.readUInt32) {
return buffer.readUInt32(offset, bigEndian);
}
var value;
if (bigEndian) {
if (buffer.readUInt32BE) {
return buffer.readUInt32BE(offset);
}
value = (buffer[offset] << 24) (buffer[offset 1] << 16) (buffer[offset 2] << 8) buffer[offset 3];
}
else {
if (buffer.readUInt32LE) {
return buffer.readUInt32LE(offset);
}
value = buffer[offset] (buffer[offset 1] << 8) (buffer[offset 2] << 16) (buffer[offset 3] << 24);
}
return value;
}
function readUInt16(buffer, offset, bigEndian) {
if (buffer.readUInt16) {
return buffer.readUInt16(offset, bigEndian);
}
var value;
if (bigEndian) {
if (buffer.readUInt16BE) {
return buffer.readUInt16BE(offset);
}
value = (buffer[offset] << 8) buffer[offset 1];
}
else {
if (buffer.readUInt16LE) {
return buffer.readUInt16LE(offset);
}
value = buffer[offset] (buffer[offset 1] << 8);
}
return value;
}
function readBit(buffer, offset, bitOffset) {
if (bitOffset > 7) {
offset = Math.floor(bitOffset / 8);
bitOffset = bitOffset % 8;
}
var b = buffer[offset];
if (bitOffset < 7) {
b >>>= (7 - bitOffset);
}
var val = b & 0x01;
return val;
}
function readBits(buffer, offset, bitOffset, bitLen, signed) {
var val = 0;
var neg = false;
if (signed) {
if (readBit(buffer, offset, bitOffset) > 0) {
neg = true;
}
bitLen--;
bitOffset ;
}
var bytes = [];
for (var i = 0; i < bitLen; i ) {
var b = readBit(buffer, offset, bitOffset i);
if (i>0 && (bitLen - i) % 8 == 0) {
bytes.push(val);
val = 0;
}
val <<= 1;
val |= b;
}
bytes.push(val);
val = new Buffer(bytes);
val.negative = neg?true:false;
return val;
}
function imageInfoPng(buffer) {
var imageHeader = [0x49, 0x48, 0x44, 0x52],
pos = 12;
if (!checkSig(buffer, pos, imageHeader)) {
return false;
}
pos = 4;
return {
type: 'image',
format: 'PNG',
mimeType: 'image/png',
width: readUInt32(buffer, pos, true),
height: readUInt32(buffer, pos 4, true),
};
}
function imageInfoJpg(buffer) {
var pos = 2,
len = buffer.length,
sizeSig = [0xff, [0xc0, 0xc2]];
while (pos < len) {
if (checkSig(buffer, pos, sizeSig)) {
pos = 5;
return {
type: 'image',
format: 'JPG',
mimeType: 'image/jpeg',
width: readUInt16(buffer, pos 2, true),
height: readUInt16(buffer, pos, true),
};
}
pos = 2;
var size = readUInt16(buffer, pos, true);
pos = size;
}
}
function imageInfoGif(buffer) {
var pos = 6;
return {
type: 'image',
format: 'GIF',
mimeType: 'image/gif',
width: readUInt16(buffer, pos, false),
height: readUInt16(buffer, pos 2, false),
};
}
function imageInfoSwf(buffer) {
var pos = 8,
bitPos = 0,
val;
if (buffer[0] === 0x43) {
try {
// If you have zlib available ( npm install zlib ) then we can read compressed flash files
buffer = require('zlib').inflate(buffer.slice(8, 100));
pos = 0;
}
catch (ex) {
// Can't get width/height of compressed flash files... yet (need zlib)
return {
type: 'flash',
format: 'SWF',
mimeType: 'application/x-shockwave-flash',
width: null,
height: null,
}
}
}
var numBits = readBits(buffer, pos, bitPos, 5)[0];
bitPos = 5;
val = readBits(buffer, pos, bitPos, numBits, true);
var xMin = (numBits > 9 ? readUInt16(val, 0, true) : val[0]) * (val.negative ? -1 : 1);
bitPos = numBits;
val = readBits(buffer, pos, bitPos, numBits, true);
var xMax = (numBits > 9 ? readUInt16(val, 0, true) : val[0]) * (val.negative ? -1 : 1);
bitPos = numBits;
val = readBits(buffer, pos, bitPos, numBits, true);
var yMin = (numBits > 9 ? readUInt16(val, 0, true) : val[0]) * (val.negative ? -1 : 1);
bitPos = numBits;
val = readBits(buffer, pos, bitPos, numBits, true);
var yMax = (numBits > 9 ? readUInt16(val, 0, true) : val[0]) * (val.negative ? -1 : 1);
return {
type: 'flash',
format: 'SWF',
mimeType: 'application/x-shockwave-flash',
width: Math.ceil((xMax - xMin) / 20),
height: Math.ceil((yMax - yMin) / 20),
};
}
function checkSig(buffer, offset, sig) {
var len = sig.length;
for (var i = 0; i < len; i ) {
var b = buffer[i offset],
s = sig[i],
m = false;
if ('number' == typeof s) {
m = s === b;
}
else {
for (var k in s) {
var o = s[k];
if (o === b) {
m = true;
}
}
}
if (!m) {
return false;
}
}
return true;
}
module.exports = function imageInfo(buffer, path) {
var pngSig = [0x89, 0x50, 0x4e, 0x47, 0x0d, 0x0a, 0x1a, 0x0a];
var jpgSig = [0xff, 0xd8, 0xff];
var gifSig = [0x47, 0x49, 0x46, 0x38, [0x37, 0x39], 0x61];
var swfSig = [[0x46, 0x43], 0x57, 0x53];
if (checkSig(buffer, 0, pngSig)) return imageInfoPng(buffer);
if (checkSig(buffer, 0, jpgSig)) return imageInfoJpg(buffer);
if (checkSig(buffer, 0, gifSig)) return imageInfoGif(buffer);
if (checkSig(buffer, 0, swfSig)) return imageInfoSwf(buffer);
return false;
};

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


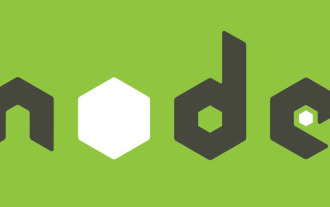
This article will give you an in-depth understanding of the memory and garbage collector (GC) of the NodeJS V8 engine. I hope it will be helpful to you!
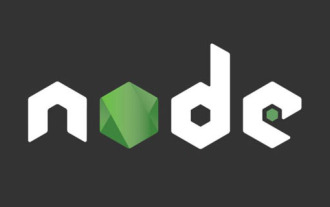
The Node service built based on non-blocking and event-driven has the advantage of low memory consumption and is very suitable for handling massive network requests. Under the premise of massive requests, issues related to "memory control" need to be considered. 1. V8’s garbage collection mechanism and memory limitations Js is controlled by the garbage collection machine

Choosing a Docker image for Node may seem like a trivial matter, but the size and potential vulnerabilities of the image can have a significant impact on your CI/CD process and security. So how do we choose the best Node.js Docker image?
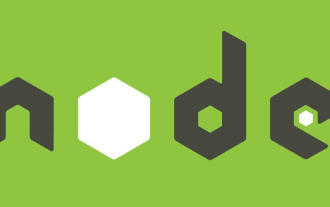
The file module is an encapsulation of underlying file operations, such as file reading/writing/opening/closing/delete adding, etc. The biggest feature of the file module is that all methods provide two versions of **synchronous** and **asynchronous**, with Methods with the sync suffix are all synchronization methods, and those without are all heterogeneous methods.
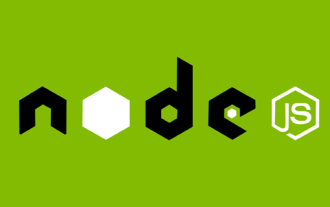
Node 19 has been officially released. This article will give you a detailed explanation of the 6 major features of Node.js 19. I hope it will be helpful to you!
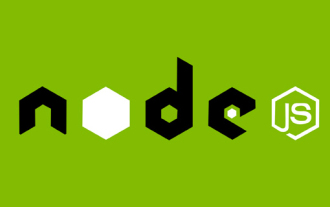
How does Node.js do GC (garbage collection)? The following article will take you through it.
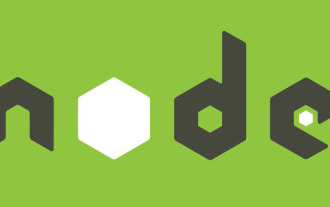
The event loop is a fundamental part of Node.js and enables asynchronous programming by ensuring that the main thread is not blocked. Understanding the event loop is crucial to building efficient applications. The following article will give you an in-depth understanding of the event loop in Node. I hope it will be helpful to you!
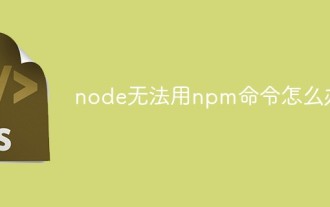
The reason why node cannot use the npm command is because the environment variables are not configured correctly. The solution is: 1. Open "System Properties"; 2. Find "Environment Variables" -> "System Variables", and then edit the environment variables; 3. Find the location of nodejs folder; 4. Click "OK".
