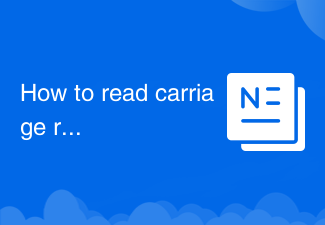
How to read carriage return in java
In Java, a carriage return is usually represented by a newline character. "\r\n" is used in Windows systems to represent carriage return and line feed, while "\n" is used in Unix/Linux systems. When reading text that contains carriage returns, Java treats these characters as normal characters. You can use the BufferedReader or Scanner classes to read text from the input stream until a carriage return or line feed is encountered.


How to read carriage return in java
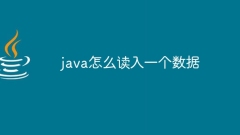
How to read data in java
In Java, the way data is read depends on the data source and format. Common methods include: - **Reading data from the console:** Use the Scanner class to read data entered by the user. - **Reading data from files:** Use the BufferedReader and FileReader classes to read text files. For binary files, you can use the Files and Paths classes (Java 8 and above). - **Read data from the database: **Use JDBC (Java Database Connectivity) to connect to the relational database and execute queries. - **Read data from other sources:
Mar 22, 2024 pm 04:10 PM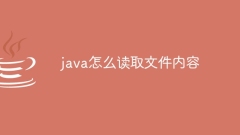
How to read file content in java
Java provides a variety of ways to read file contents, including: * **Files.readAllLines/Files.readAllBytes (Java 8 and above)**: Using the java.nio.file.Files class, you can easily read all lines or the entire content of a file. * **BufferedReader**: For older versions of Java, you can use the BufferedReader class to read a file line by line. * **Scanner**: The Scanner class provides another way to read files, which can read the content line by line or by delimiter.
Mar 22, 2024 pm 03:39 PM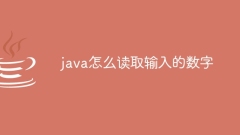
How to read input numbers in java
Obtaining method: 1. Create a Scanner object to read the user's input; 2. Get the integer number entered by the user by calling the nextInt() method; 3. Output the obtained number.
Mar 22, 2024 pm 03:26 PM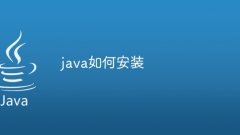
How to install java
Installation steps: 1. Download JDK; 2. Install JDK; 3. Verify installation; 4. Set environment variables. Detailed introduction: 1. Download JDK: Go to Oracle official website or OpenJDK official website. Select the latest version of JDK suitable for the operating system to download; 2. Install JDK: Open the downloaded installation file and install it according to the instructions of the installation wizard; 3. Verify the installation: Open the command line and enter the "java -version" command to check whether Java is successful. Installation, if the installation is successful, the Java version, etc. will be displayed.
Dec 27, 2023 pm 04:13 PM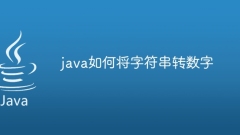
How to convert string to number in java
How to convert a string to a number in java: 1. Use the Integer.parseInt() method to convert the string to an integer; 2. Use the Double.parseDouble() method to convert the string to a floating point number; 3. Use Float.parseFloat( ) method to convert a string to a floating point number; 4. Use the Long.parseLong() method to convert a string to a long integer; 5. Use the Short.parseShort() method to convert a string to a short integer.
Aug 11, 2023 am 11:34 AM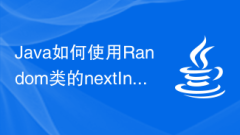
How to generate random integers in Java using nextInt() function of Random class
How does Java use the nextInt() function of the Random class to generate random integers? Introduction: In programming, we often encounter the need to generate random integers. Java provides the Random class to help us implement this function. The nextInt() function can generate a random integer within a specified range. This article will introduce readers to how to use the nextInt() function of the Random class to generate random integers and give corresponding code examples. Ran
Jul 24, 2023 pm 02:03 PM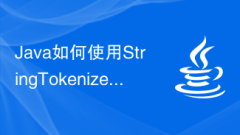
How to split a string into multiple substrings using StringTokenizer class in Java
How to use the StringTokenizer class in Java to split a string into multiple substrings Introduction: In Java development, it is often necessary to split a string into multiple substrings for further processing. Java provides many methods to split strings, one of the commonly used tools is the StringTokenizer class. This article will introduce the basic usage of the StringTokenizer class and provide code examples to help readers better understand. StringToknizer
Jul 24, 2023 pm 03:24 PM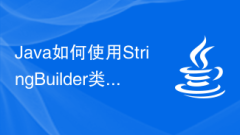
How does Java use the insert() function of the StringBuilder class to insert a string at a specified position?
How does Java use the insert() function of the StringBuilder class to insert a string at a specified position? In Java programming, the String class is an immutable class, which means that once a string object is created, its value cannot be changed. However, in actual development, we sometimes need to insert another string into one string. In order to achieve this function, Java provides the StringBuilder class. StringBuilder is a mutable class that allows us to
Jul 25, 2023 am 09:31 AM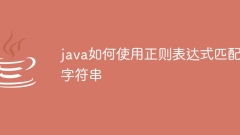
How to use regular expressions to match strings in java
Steps for using regular expressions to match strings in java: 1. Create a Pattern object and use the "Pattern.compile()" method to compile a regular expression; 2. Use the Matcher object to perform matching operations, which can be done by calling "Pattern. matcher()" method to create; 3. Call various methods of the Matcher object to perform matching operations. You can use "matches()", "find()", and "group()".
Aug 11, 2023 am 11:19 AM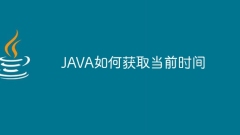
How to get the current time in JAVA
How to get the current time in JAVA: 1. Using the Date class, you can use the no-argument constructor of the Date class to create a Date object representing the current time, and then use the "toString()" method of the object to convert it into a string representation; 2. Use the Calendar class to obtain the values of the current time's fields such as year, month, day, hour, minute, and second. 3. Use the new time and date API to provide a more concise, easy-to-use, and thread-safe way. Process dates and times.
Sep 28, 2023 pm 01:42 PM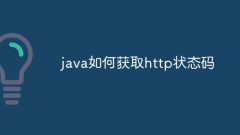
How to get http status code in java
The http status code can be obtained by using the HttpURLConnection class. The specific operations are as follows: 1. Create a URL object; 2. Use the object to open a connection; 3. Set the request method to GET; 4. Use the `getResponseCode()` method to obtain the HTTP status code; 5. Print Get the status code and close the connection.
Oct 07, 2023 pm 02:39 PM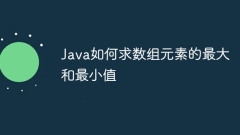
How to find the maximum and minimum value of array elements in Java
Use the `Arrays.stream()` function in Java to convert an array into a stream, and then use the `min()` and `max()` functions to calculate the minimum and maximum values.
Oct 08, 2023 am 09:44 AM
Hot Article

Hot Tools

Kits AI
Transform your voice with AI artist voices. Create and train your own AI voice model.

SOUNDRAW - AI Music Generator
Create music easily for videos, films, and more with SOUNDRAW's AI music generator.

Web ChatGPT.ai
Free Chrome extension with OpenAI chatbot for efficient browsing.

Sweetless
AI-powered app to monitor and reduce sugar intake.

Notte.ai
AI meeting assistant for note-taking and organizing ideas.
