Comment choisir le framework Golang
Lorsque vous choisissez le framework Web approprié dans Go, vous pouvez choisir en fonction de vos besoins : Gin : framework Web RESTful léger et performant ; Echo : framework Web RESTful évolutif et robuste ; Gorilla : package de création d'API REST modulaire ; Beego ; stack web framework, fournissant l'API RESTful, MVC et ORM Hugo : générateur de sites Web statiques, construit sur Go, rapide et flexible.
Guide de sélection du framework Go
Choisir le bon framework dans Go peut être une tâche ardue car il existe de nombreuses options parmi lesquelles choisir. Cet article vise à aider les développeurs à prendre une décision éclairée en fournissant une comparaison complète et un exemple pratique.
Le framework le plus populaire de Go
- Gin : Un framework Web orienté REST connu pour sa simplicité, ses performances et sa richesse en fonctionnalités.
- Echo : Un autre framework Web RESTful axé sur l'évolutivité et la robustesse.
- Gorilla : Un ensemble de packages modulaires pour la création d'API RESTful, la validation, le routage et la gestion de session.
- Beego : Un framework Web full-stack qui fournit une API RESTful, une architecture MVC et une intégration ORM.
- Hugo : Un générateur de sites Web statiques construit sur Go, connu pour sa rapidité et sa flexibilité.
Comparaison des cadres
Caractéristiques | Gin | Echo | Gorilla | Beego | Hugo |
---|---|---|---|---|---|
routage | Supporte le routage imbriqué | Supporte le routage imbriqué | Supporte le routage imbriqué | Supporte le routage imbriqué | Aucun |
Validation | Validateur intégré | Validateur intégré | Aucun | Validateur intégré | Aucun |
ORM | Aucun | Aucun | Aucun | ORM intégré | Aucun |
Support RESTful | Support complet | Support complet | Support partiel | Support complet | Aucun |
Traitement des fichiers statiques | support | Support | Support | Support | Support |
Évolutivité | Très évolutive | Très évolutive | Évolutivité moyenne | Faible évolutivité | Aucun |
Documentation | Excellent | Bon | Bon | Bon | Excellent |
Pratique cas : Utiliser Gin pour créer une API RESTful
Créons maintenant une API RESTful simple pour démontrer le framework Gin.
package main import ( "github.com/gin-gonic/gin" ) // 定义一个用于存储 JSON 数据的结构体 type Person struct { Name string `json:"name"` Age int `json:"age"` Address string `json:"address"` } // 主函数 func main() { // 创建 Gin 路由器 router := gin.Default() // 定义一个路由来获取所有人员 router.GET("/persons", getAllPersons) // 定义一个路由来获取特定人员 router.GET("/persons/:id", getPersonByID) // 定义一个路由来创建新的人员 router.POST("/persons", createPerson) // 定义一个路由来更新人员 router.PUT("/persons/:id", updatePerson) // 定义一个路由来删除人员 router.DELETE("/persons/:id", deletePerson) // 启动服务器 router.Run(":8080") } // 实现控制器函数 // getAllPersons 获取所有人员 func getAllPersons(c *gin.Context) { // 从数据库获取所有人员 persons := []Person{} // 将人员数据返回给客户端 c.JSON(200, persons) } // getPersonByID 通过 ID 获取人员 func getPersonByID(c *gin.Context) { // 从 URL 参数获取 ID id := c.Param("id") // 从数据库获取与 ID 匹配的人员 person := Person{} // 如果人员不存在,返回 404 错误 if person.ID == 0 { c.JSON(404, gin.H{"error": "Person not found"}) return } // 将人员数据返回给客户端 c.JSON(200, person) } // createPerson 创建新的人员 func createPerson(c *gin.Context) { // 从请求正文中解析 Person 数据 var person Person if err := c.BindJSON(&person); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } // 将新的人员保存到数据库 if err := person.Save(); err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } // 返回创建的 Person 数据 c.JSON(201, person) } // updatePerson 更新人员 func updatePerson(c *gin.Context) { // 从 URL 参数获取 ID id := c.Param("id") // 从数据库获取与 ID 匹配的人员 person := Person{} // 如果人员不存在,返回 404 错误 if person.ID == 0 { c.JSON(404, gin.H{"error": "Person not found"}) return } // 从请求正文中解析更新后的 Person 数据 var updatedPerson Person if err := c.BindJSON(&updatedPerson); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } // 更新人员数据 if err := person.Update(updatedPerson); err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } // 返回更新后的 Person 数据 c.JSON(200, person) } // deletePerson 删除人员 func deletePerson(c *gin.Context) { // 从 URL 参数获取 ID id := c.Param("id") // 从数据库获取与 ID 匹配的人员 person := Person{} // 如果人员不存在,返回 404 错误 if person.ID == 0 { c.JSON(404, gin.H{"error": "Person not found"}) return } // 删除人员 if err := person.Delete(); err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } // 返回成功消息 c.JSON(200, gin.H{"message": "Person deleted successfully"}) }
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

AI Hentai Generator
Générez AI Hentai gratuitement.

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)
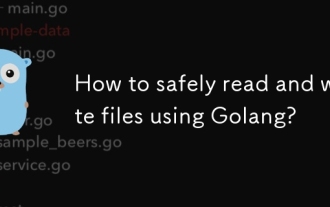
Lire et écrire des fichiers en toute sécurité dans Go est crucial. Les directives incluent : Vérification des autorisations de fichiers Fermeture de fichiers à l'aide de reports Validation des chemins de fichiers Utilisation de délais d'attente contextuels Le respect de ces directives garantit la sécurité de vos données et la robustesse de vos applications.
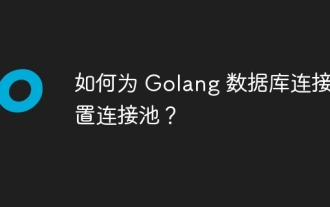
Comment configurer le pool de connexions pour les connexions à la base de données Go ? Utilisez le type DB dans le package base de données/sql pour créer une connexion à la base de données ; définissez MaxOpenConns pour contrôler le nombre maximum de connexions simultanées ; définissez MaxIdleConns pour définir le nombre maximum de connexions inactives ; définissez ConnMaxLifetime pour contrôler le cycle de vie maximum de la connexion ;
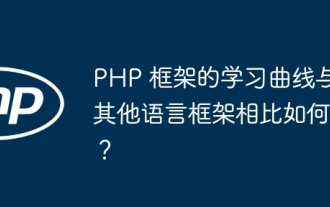
La courbe d'apprentissage d'un framework PHP dépend de la maîtrise du langage, de la complexité du framework, de la qualité de la documentation et du support de la communauté. La courbe d'apprentissage des frameworks PHP est plus élevée par rapport aux frameworks Python et inférieure par rapport aux frameworks Ruby. Par rapport aux frameworks Java, les frameworks PHP ont une courbe d'apprentissage modérée mais un temps de démarrage plus court.

Le framework PHP léger améliore les performances des applications grâce à une petite taille et une faible consommation de ressources. Ses fonctionnalités incluent : une petite taille, un démarrage rapide, une faible utilisation de la mémoire, une vitesse de réponse et un débit améliorés et une consommation de ressources réduite. Cas pratique : SlimFramework crée une API REST, seulement 500 Ko, une réactivité élevée et un débit élevé.
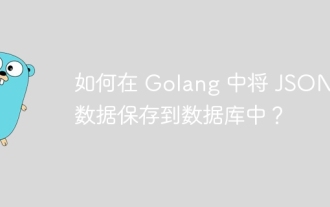
Les données JSON peuvent être enregistrées dans une base de données MySQL à l'aide de la bibliothèque gjson ou de la fonction json.Unmarshal. La bibliothèque gjson fournit des méthodes pratiques pour analyser les champs JSON, et la fonction json.Unmarshal nécessite un pointeur de type cible pour désorganiser les données JSON. Les deux méthodes nécessitent la préparation d'instructions SQL et l'exécution d'opérations d'insertion pour conserver les données dans la base de données.
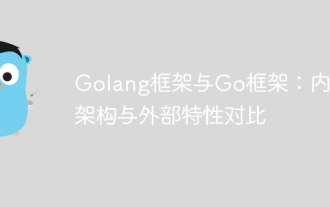
La différence entre le framework GoLang et le framework Go se reflète dans l'architecture interne et les fonctionnalités externes. Le framework GoLang est basé sur la bibliothèque standard Go et étend ses fonctionnalités, tandis que le framework Go se compose de bibliothèques indépendantes pour atteindre des objectifs spécifiques. Le framework GoLang est plus flexible et le framework Go est plus facile à utiliser. Le framework GoLang présente un léger avantage en termes de performances et le framework Go est plus évolutif. Cas : gin-gonic (framework Go) est utilisé pour créer l'API REST, tandis qu'Echo (framework GoLang) est utilisé pour créer des applications Web.
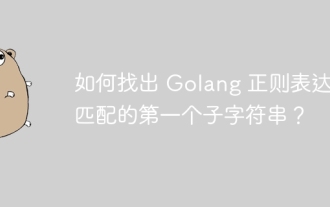
La fonction FindStringSubmatch recherche la première sous-chaîne correspondant à une expression régulière : la fonction renvoie une tranche contenant la sous-chaîne correspondante, le premier élément étant la chaîne entière correspondante et les éléments suivants étant des sous-chaînes individuelles. Exemple de code : regexp.FindStringSubmatch(text,pattern) renvoie une tranche de sous-chaînes correspondantes. Cas pratique : Il peut être utilisé pour faire correspondre le nom de domaine dans l'adresse email, par exemple : email:="user@example.com", pattern:=@([^\s]+)$ pour obtenir la correspondance du nom de domaine [1].
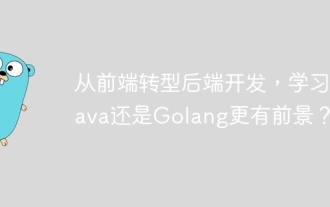
Chemin d'apprentissage du backend: le parcours d'exploration du front-end à l'arrière-end en tant que débutant back-end qui se transforme du développement frontal, vous avez déjà la base de Nodejs, ...
