Comment créer un serveur proxy Scratch à l'aide de Node.js
Vous pourriez être curieux de savoir comment fonctionnent les serveurs proxy et comment ils diffusent des données sur Internet. Dans ce blog, je vais implémenter un serveur proxy utilisant NodeJs de base. J'y suis parvenu en utilisant un package NodeJs de base appelé net qui est déjà fourni avec NodeJs.
Comment fonctionne le proxy
un serveur proxy est un agent entre le client et le serveur. Quand un
le client envoie une requête à un serveur, elle est transmise à un proxy cible
serveur. Le serveur proxy ciblé traite la requête et l'envoie
au serveur principal et le serveur principal envoie la demande de retour au
serveur proxy et le serveur proxy envoie la demande au client.
Configurer le serveur proxy
Avant de commencer la programmation, vous savez peu de choses sur socket et NodeJs. Vous savez ce qu'est un socket et comment ils fonctionnent.
Dans NodeJs, il existe deux méthodes pour implémenter un serveur proxy. D'abord, j'ai une méthode personnalisée et ensuite une méthode intégrée. Les deux sont faciles à comprendre.
Pour tester votre serveur proxy, vous pouvez exécuter votre service HTTP local sur votre machine locale, puis cibler la machine hôte.
- Maintenant, je vais définir le package net, puis écrire le serveur cible et le numéro de port.
const net = require('net'); // Define the target host and port const targetHost = 'localhost'; // Specify the hostname of the target server. For Ex: (12.568.45.25) const targetPort = 80; // Specify the port of the target server
- Création et écoute du serveur TCP.
const server = net.createServer((clientSocket) => { }); // Start listening for incoming connections on the specified port const proxyPort = 3000; // Specify the port for the proxy server server.listen(proxyPort, () => { console.log(`Reverse proxy server is listening on port ${proxyPort}`); });
- nous avons créé un serveur en utilisant le package net. c'est un serveur TCP qui exécute le numéro de port 3000 comme nous le définissons en utilisant la variable proxyPort. si le serveur démarre, il affichera le message Le serveur proxy inverse écoute sur le port 3000 sur la console.
- Lorsque le client essaie de se connecter au serveur proxy, il exécute la fonction de rappel qui se trouve à l'intérieur de createServer.
Dans le rappel de la fonction serveur, nous avons un paramètre clientSocket, c'est la connexion utilisateur que nous avons reçue pour la connexion.
accepter et recevoir les données de l'utilisateur
const targetHost = 'localhost'; // Specify the hostname of the target server. For Ex: (12.568.45.25) const targetPort = 80; // Specify the port of the target server // Create a TCP server const server = net.createServer((clientSocket) => { // Establish a connection to the target host net.createConnection({host: targetHost, port: targetPort}, () => { // When data is received from the client, write it to the target server clientSocket.on("data", (data) => { targetSocket.write(data); }); // When data is received from the target server, write it back to the client targetSocket.on("data", (data) => { clientSocket.write(data); }); }); });
- Lorsqu'un client essaie de se connecter au serveur proxy, nous créerons une connexion temporaire au serveur en utilisant create createConnection
-
createServer a 2 arguments
- 1er hôte où nous voulons nous connecter dans ce cas, nous devons nous connecter à notre serveur hôte qui est défini dans la variable targetHost.
- 2ème port où nous voulons nous connecter dans ce cas nous devons nous connecter au port de notre serveur qui est défini dans la variable targetPort.
- Maintenant, lorsque l'utilisateur envoie des données, je transmets ces données au serveur. en utilisant ce code
clientSocket.on("data", (data) => { targetSocket.write(data); });
- Ensuite, le serveur envoie des données. J'enverrai ces données à l'utilisateur, en utilisant ce code
targetSocket.on("data", (data) => { clientSocket.write(data); });
- Félicitations ! vous avez créé avec succès votre serveur proxy.
Gestion des erreurs
Bien qu'explorer les fonctionnalités du serveur proxy soit passionnant, garantir la fiabilité nécessite des méthodes robustes de gestion des erreurs pour gérer les problèmes inattendus avec élégance. Pour gérer ces types d’erreurs, nous avons un événement appelé erreur. C'est très simple à mettre en œuvre.
const server = net.createServer((clientSocket) => { // Establish a connection to the target host const targetSocket = net.createConnection({host: targetHost,port: targetPort}, () => { // Handle errors when connecting to the target server targetSocket.on('error', (err) => { console.error('Error connecting to target:', err); clientSocket.end(); // close connection }); // Handle errors related to the client socket clientSocket.on('error', (err) => { console.error('Client socket error:', err); targetSocket.end(); // close connection }); }); });
- Lorsqu'une erreur se produit, nous consolerons l'erreur puis fermerons la connexion.
TOUS les codes
- remplacez le nom d'hôte et le numéro de port selon vos préférences.
const net = require('net'); // Define the target host and port const targetHost = 'localhost'; // Specify the hostname of the target server const targetPort = 80; // Specify the port of the target server // Create a TCP server const server = net.createServer((clientSocket) => { // Establish a connection to the target host const targetSocket = net.createConnection({ host: targetHost, port: targetPort }, () => { // When data is received from the target server, write it back to the client targetSocket.on("data", (data) => { clientSocket.write(data); }); // When data is received from the client, write it to the target server clientSocket.on("data", (data) => { targetSocket.write(data); }); }); // Handle errors when connecting to the target server targetSocket.on('error', (err) => { console.error('Error connecting to target:', err); clientSocket.end(); }); // Handle errors related to the client socket clientSocket.on('error', (err) => { console.error('Client socket error:', err); targetSocket.end(); }); }); // Start listening for incoming connections on the specified port const proxyPort = 3000; // Specify the port for the proxy server server.listen(proxyPort, () => { console.log(`Reverse proxy server is listening on port ${proxyPort}`); });
Méthode construite
Pour réduire la complexité des connexions client et serveur, nous avons un canal de méthode intégré. Je remplacerai cette syntaxe
// Handle errors when connecting to the target server targetSocket.on("data", (data) => { clientSocket.write(data); }); // When data is received from the client, write it to the target server clientSocket.on("data", (data) => { targetSocket.write(data); });
Dans cette syntaxe
// Pipe data from the client to the target clientSocket.pipe(targetSocket); // When data is received from the client, write it to the target server targetSocket.pipe(clientSocket);
Voici tout le code
const net = require('net'); // Define the target host and port const targetHost = 'localhost'; const targetPort = 80; // Create a TCP server const server = net.createServer((clientSocket) => { // Establish a connection to the target host const targetSocket = net.createConnection({ host: targetHost, port: targetPort }, () => { // Pipe data from the client to the target clientSocket.pipe(targetSocket); // Pipe data from the target to the client targetSocket.pipe(clientSocket); }); // Handle errors targetSocket.on('error', (err) => { console.error('Error connecting to target:', err); clientSocket.end(); }); clientSocket.on('error', (err) => { console.error('Client socket error:', err); targetSocket.end(); }); }); // Start listening for incoming connections const proxyPort = 3000; server.listen(proxyPort, () => { console.log(`Reverse proxy server is listening on port ${proxyPort}`); });
Un serveur proxy fonctionnel !
Suivez-moi sur GitHub avinashtare, merci.
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

Video Face Swap
Échangez les visages dans n'importe quelle vidéo sans effort grâce à notre outil d'échange de visage AI entièrement gratuit !

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)

Sujets chauds










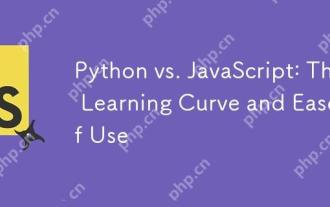
Python convient plus aux débutants, avec une courbe d'apprentissage en douceur et une syntaxe concise; JavaScript convient au développement frontal, avec une courbe d'apprentissage abrupte et une syntaxe flexible. 1. La syntaxe Python est intuitive et adaptée à la science des données et au développement back-end. 2. JavaScript est flexible et largement utilisé dans la programmation frontale et côté serveur.
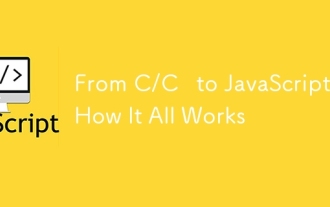
Le passage de C / C à JavaScript nécessite de s'adapter à la frappe dynamique, à la collecte des ordures et à la programmation asynchrone. 1) C / C est un langage dactylographié statiquement qui nécessite une gestion manuelle de la mémoire, tandis que JavaScript est dynamiquement typé et que la collecte des déchets est automatiquement traitée. 2) C / C doit être compilé en code machine, tandis que JavaScript est une langue interprétée. 3) JavaScript introduit des concepts tels que les fermetures, les chaînes de prototypes et la promesse, ce qui améliore la flexibilité et les capacités de programmation asynchrones.
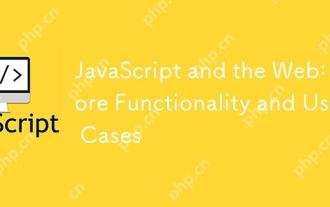
Les principales utilisations de JavaScript dans le développement Web incluent l'interaction client, la vérification du formulaire et la communication asynchrone. 1) Mise à jour du contenu dynamique et interaction utilisateur via les opérations DOM; 2) La vérification du client est effectuée avant que l'utilisateur ne soumette les données pour améliorer l'expérience utilisateur; 3) La communication de rafraîchissement avec le serveur est réalisée via la technologie AJAX.
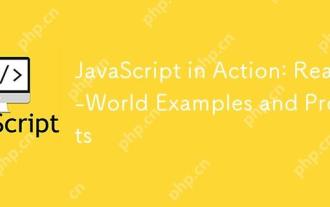
L'application de JavaScript dans le monde réel comprend un développement frontal et back-end. 1) Afficher les applications frontales en créant une application de liste TODO, impliquant les opérations DOM et le traitement des événements. 2) Construisez RestulAPI via Node.js et Express pour démontrer les applications back-end.
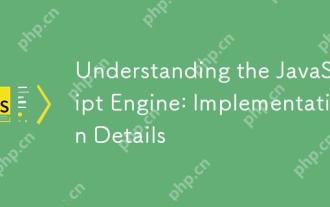
Comprendre le fonctionnement du moteur JavaScript en interne est important pour les développeurs car il aide à écrire du code plus efficace et à comprendre les goulots d'étranglement des performances et les stratégies d'optimisation. 1) Le flux de travail du moteur comprend trois étapes: analyse, compilation et exécution; 2) Pendant le processus d'exécution, le moteur effectuera une optimisation dynamique, comme le cache en ligne et les classes cachées; 3) Les meilleures pratiques comprennent l'évitement des variables globales, l'optimisation des boucles, l'utilisation de const et de locations et d'éviter une utilisation excessive des fermetures.
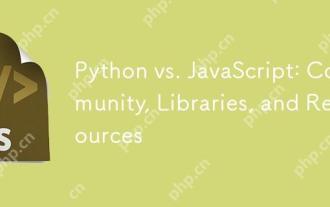
Python et JavaScript ont leurs propres avantages et inconvénients en termes de communauté, de bibliothèques et de ressources. 1) La communauté Python est amicale et adaptée aux débutants, mais les ressources de développement frontal ne sont pas aussi riches que JavaScript. 2) Python est puissant dans les bibliothèques de science des données et d'apprentissage automatique, tandis que JavaScript est meilleur dans les bibliothèques et les cadres de développement frontaux. 3) Les deux ont des ressources d'apprentissage riches, mais Python convient pour commencer par des documents officiels, tandis que JavaScript est meilleur avec MDNWEBDOCS. Le choix doit être basé sur les besoins du projet et les intérêts personnels.
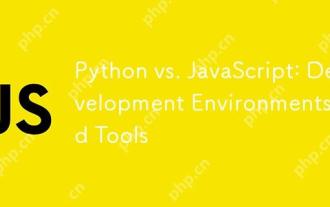
Les choix de Python et JavaScript dans les environnements de développement sont importants. 1) L'environnement de développement de Python comprend Pycharm, Jupyternotebook et Anaconda, qui conviennent à la science des données et au prototypage rapide. 2) L'environnement de développement de JavaScript comprend Node.js, VScode et WebPack, qui conviennent au développement frontal et back-end. Le choix des bons outils en fonction des besoins du projet peut améliorer l'efficacité du développement et le taux de réussite du projet.
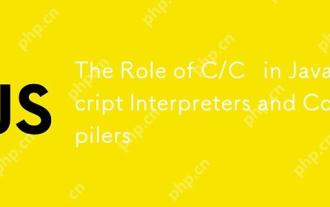
C et C jouent un rôle essentiel dans le moteur JavaScript, principalement utilisé pour implémenter des interprètes et des compilateurs JIT. 1) C est utilisé pour analyser le code source JavaScript et générer une arborescence de syntaxe abstraite. 2) C est responsable de la génération et de l'exécution de bytecode. 3) C met en œuvre le compilateur JIT, optimise et compile le code de point chaud à l'exécution et améliore considérablement l'efficacité d'exécution de JavaScript.
