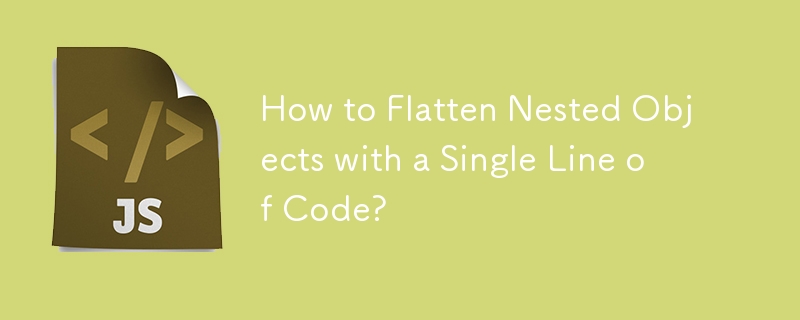
Flattening Nested Objects with a One-Liner
To flatten nested objects, you can employ the following one-line solution:
Object.assign({}, ...function _flatten(o) { return [].concat(...Object.keys(o).map(k => typeof o[k] === 'object' ? _flatten(o[k]) : ({[k]: o[k]})))}(yourObject))
Copier après la connexion
This one-liner can flatten objects with nested properties, converting them into flat objects with one-level properties.
How It Works:
-
Recursive Function _flatten: The function recursively traverses the object, flattening any nested properties.
-
Spread Operator (ES6): Inside the _flatten function, the spread operator ... is used to create an array of one-property objects from each property in the object ({[k]: o[k]}).
-
Concatenation: The Object.keys(o) function returns an array of property names, and the [].concat function is used to concatenate the arrays of one-property objects into a single array.
-
Object.assign Function (ES6): The Object.assign function is used to combine the flattened arrays of one-property objects into a single, flattened object.
Example:
Using the example object:
{
a: 2,
b: {
c: 3
}
}
Copier après la connexion
The one-line solution will produce the flattened object:
{
a: 2,
c: 3
}
Copier après la connexion
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!