


Structure des données de la pile | Dernier entré, premier sorti (LIFO)
- - Push (Ajouter un élément) : Ajoutez un élément en haut de la pile.
- - Pop (Supprimer un élément) : Supprimez l'élément du haut.
- - isFull : Vérifiez si la pile a atteint sa limite (dans ce cas, 10).
- - isEmpty : Vérifiez si la pile est vide.
- - Affichage : Afficher les éléments de la pile.
1.Exemple :
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Stack | Last In First Out (LIFO) or First in Last out | - By Sudhanshu Gaikwad (FILO)</title> </head> <body> <h3>Stack in Javascript</h3> <script> let Data = []; // Add an element to the array function AddEle(Ele) { if (isFull()) { console.log("Array is Full, Element can't be added!"); } else { console.log("Element Added!"); Data.push(Ele); } } // Check if the array is full function isFull() { return Data.length >= 10; } // Remove an element from the array function Remove() { if (isEmpty()) { console.log("Array is Empty, can't remove element!"); } else { Data.pop(); console.log("Element Removed!"); } } // Check if the array is empty function isEmpty() { return Data.length === 0; } // Display the array elements function Display() { console.log("Updated Array >> ", Data); } // Example usage AddEle(55); AddEle(85); AddEle(25); Remove(); Display(); // [55, 85] </script> </body> </html>
2.Exemple :
index2.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>What is Stack in JavaScript | By Sudhanshu Gaikwad</title> <style> * { box-sizing: border-box; } body { font-family: "Roboto Condensed", sans-serif; background-color: #f4f4f4; margin: 0; padding: 0; display: flex; flex-direction: column; justify-content: center; align-items: center; height: 100vh; } .container { background-color: white; padding: 20px; border-radius: 10px; box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1); max-width: 400px; width: 100%; margin-bottom: 20px; } h3 { color: #333; text-align: center; margin-bottom: 20px; } input { padding: 10px; width: calc(100% - 20px); margin-bottom: 10px; border: 1px solid #ccc; border-radius: 5px; } button { padding: 10px; margin: 10px 0; border: none; border-radius: 5px; background-color: #292f31; color: white; cursor: pointer; width: 100%; } button:hover { background-color: #e9e9ea; color: #292f31; } .message { margin-top: 15px; color: #333; font-size: 16px; text-align: center; } .footer { text-align: center; margin-top: 20px; font-size: 14px; color: #555; } /* Responsive design */ @media (max-width: 768px) { .container { padding: 15px; max-width: 90%; } button { font-size: 14px; } input { font-size: 14px; } } </style> </head> <body> <div class="container"> <!-- Title --> <h3>Stack in JavaScript</h3> <!-- Input section --> <input type="text" id="AddEle" placeholder="Enter an element" /> <!-- Buttons section --> <button onclick="AddData()">Add Element</button> <button onclick="RemoveEle()">Remove Element</button> <button onclick="Display()">Show Array</button> <!-- Message sections --> <div id="Add" class="message"></div> <div id="Remove" class="message"></div> <div id="Display" class="message"></div> </div> <!-- Footer with copyright symbol --> <div class="footer"> © 2024 Sudhanshu Developer | All Rights Reserved </div> <script> let Data = []; // Function to add an element to the stack function AddData() { let NewEle = document.getElementById("AddEle").value; if (isFull()) { document.getElementById("Add").innerHTML = "Array is full, element cannot be added!"; } else if (NewEle.trim() === "") { document.getElementById("Add").innerHTML = "Please enter a valid element!"; } else { Data.push(NewEle); document.getElementById("Add").innerHTML = `Element "${NewEle}" added!`; document.getElementById("AddEle").value = ""; console.log("Current Array: ", Data); Display(); } } function isFull() { return Data.length >= 10; } function RemoveEle() { if (isEmpty()) { document.getElementById("Remove").innerHTML = "Array is empty!"; } else { let removedElement = Data.pop(); document.getElementById("Remove").innerHTML = `Element "${removedElement}" removed!`; console.log("Current Array: ", Data); Display(); } } function isEmpty() { return Data.length === 0; } function Display() { let displayArea = document.getElementById("Display"); displayArea.innerHTML = ""; if (Data.length === 0) { displayArea.innerHTML = "No elements in the Array!"; console.log("Array is empty."); } else { for (let i = 0; i < Data.length; i++) { displayArea.innerHTML += `Element ${i + 1}: ${Data[i]}<br>`; } console.log("Displaying Array: ", Data); } } </script> </body> </html>
Sortie :
Pile en langage C avec saisie utilisateur
#include <stdio.h> #include <stdbool.h> #define MAX 10 int Data[MAX]; int top = -1; // Function to check if the stack is full bool isFull() { return top >= MAX - 1; } // Function to check if the stack is empty bool isEmpty() { return top == -1; } // Function to add an element to the stack (Push operation) void AddEle() { int Ele; if (isFull()) { printf("Array is Full, Element can't be added!\n"); } else { printf("Enter an element to add: "); scanf("%d", &Ele); // Read user input Data[++top] = Ele; // Increment top and add element printf("Element %d Added!\n", Ele); } } // Function to remove an element from the stack (Pop operation) void Remove() { if (isEmpty()) { printf("Array is Empty, can't remove element!\n"); } else { printf("Element %d Removed!\n", Data[top--]); // Remove element and decrement top } } // Function to display all elements in the stack void Display() { if (isEmpty()) { printf("Array is Empty!\n"); } else { printf("Updated Array >> "); for (int i = 0; i <= top; i++) { printf("%d ", Data[i]); } printf("\n"); } } int main() { int choice; do { printf("\n1. Add Element\n2. Remove Element\n3. Display Stack\n4. Exit\n"); printf("Enter your choice: "); scanf("%d", &choice); // Read the user's choice switch (choice) { case 1: AddEle(); break; case 2: Remove(); break; case 3: Display(); break; case 4: printf("Exiting...\n"); break; default: printf("Invalid choice! Please select a valid option.\n"); } } while (choice != 4); return 0; }
Exemple de sortie :
1. Add Element 2. Remove Element 3. Display Stack 4. Exit Enter your choice: 1 Enter an element to add: 55 Element 55 Added! 1. Add Element 2. Remove Element 3. Display Stack 4. Exit Enter your choice: 3 Updated Array >> 55 1. Add Element 2. Remove Element 3. Display Stack 4. Exit Enter your choice: 4 Exiting...
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

Video Face Swap
Échangez les visages dans n'importe quelle vidéo sans effort grâce à notre outil d'échange de visage AI entièrement gratuit !

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)

Sujets chauds










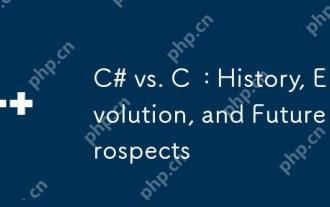
L'histoire et l'évolution de C # et C sont uniques, et les perspectives d'avenir sont également différentes. 1.C a été inventé par Bjarnestrousstrup en 1983 pour introduire une programmation orientée objet dans le langage C. Son processus d'évolution comprend plusieurs normalisations, telles que C 11, introduisant des mots clés automobiles et des expressions de lambda, C 20 introduisant les concepts et les coroutines, et se concentrera sur les performances et la programmation au niveau du système à l'avenir. 2.C # a été publié par Microsoft en 2000. Combinant les avantages de C et Java, son évolution se concentre sur la simplicité et la productivité. Par exemple, C # 2.0 a introduit les génériques et C # 5.0 a introduit la programmation asynchrone, qui se concentrera sur la productivité et le cloud computing des développeurs à l'avenir.
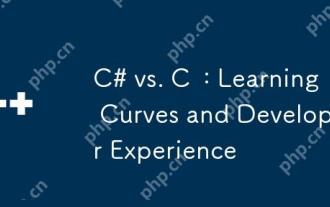
Il existe des différences significatives dans les courbes d'apprentissage de l'expérience C # et C et du développeur. 1) La courbe d'apprentissage de C # est relativement plate et convient au développement rapide et aux applications au niveau de l'entreprise. 2) La courbe d'apprentissage de C est raide et convient aux scénarios de contrôle haute performance et de bas niveau.
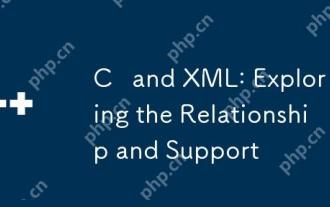
C interagit avec XML via des bibliothèques tierces (telles que TinyXML, PUGIXML, XERCES-C). 1) Utilisez la bibliothèque pour analyser les fichiers XML et les convertir en structures de données propices à C. 2) Lors de la génération de XML, convertissez la structure des données C au format XML. 3) Dans les applications pratiques, le XML est souvent utilisé pour les fichiers de configuration et l'échange de données afin d'améliorer l'efficacité du développement.
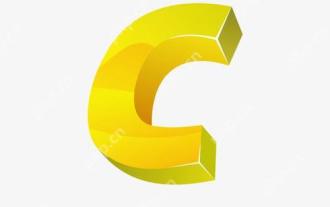
L'application de l'analyse statique en C comprend principalement la découverte de problèmes de gestion de la mémoire, la vérification des erreurs de logique de code et l'amélioration de la sécurité du code. 1) L'analyse statique peut identifier des problèmes tels que les fuites de mémoire, les doubles versions et les pointeurs non initialisés. 2) Il peut détecter les variables inutilisées, le code mort et les contradictions logiques. 3) Les outils d'analyse statique tels que la couverture peuvent détecter le débordement de tampon, le débordement entier et les appels API dangereux pour améliorer la sécurité du code.
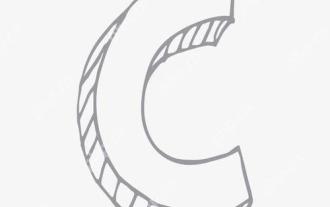
L'utilisation de la bibliothèque Chrono en C peut vous permettre de contrôler plus précisément les intervalles de temps et de temps. Explorons le charme de cette bibliothèque. La bibliothèque Chrono de C fait partie de la bibliothèque standard, qui fournit une façon moderne de gérer les intervalles de temps et de temps. Pour les programmeurs qui ont souffert de temps et ctime, Chrono est sans aucun doute une aubaine. Il améliore non seulement la lisibilité et la maintenabilité du code, mais offre également une précision et une flexibilité plus élevées. Commençons par les bases. La bibliothèque Chrono comprend principalement les composants clés suivants: std :: chrono :: system_clock: représente l'horloge système, utilisée pour obtenir l'heure actuelle. std :: chron
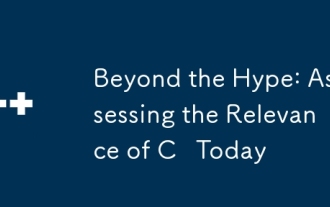
C a toujours une pertinence importante dans la programmation moderne. 1) Les capacités de fonctionnement matériel et directes en font le premier choix dans les domaines du développement de jeux, des systèmes intégrés et de l'informatique haute performance. 2) Les paradigmes de programmation riches et les fonctionnalités modernes telles que les pointeurs intelligents et la programmation de modèles améliorent sa flexibilité et son efficacité. Bien que la courbe d'apprentissage soit raide, ses capacités puissantes le rendent toujours important dans l'écosystème de programmation d'aujourd'hui.
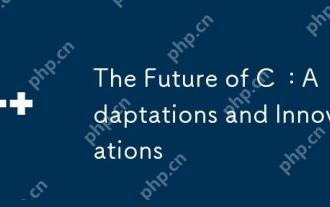
L'avenir de C se concentrera sur l'informatique parallèle, la sécurité, la modularisation et l'apprentissage AI / Machine: 1) L'informatique parallèle sera améliorée par des fonctionnalités telles que les coroutines; 2) La sécurité sera améliorée par le biais de mécanismes de vérification et de gestion de la mémoire plus stricts; 3) La modulation simplifiera l'organisation et la compilation du code; 4) L'IA et l'apprentissage automatique inviteront C à s'adapter à de nouveaux besoins, tels que l'informatique numérique et le support de programmation GPU.
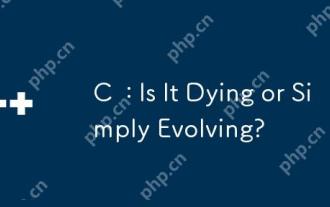
C isnotdying; il se révolte.1) C reste réévèreurtoitSversatity et effecciation en termes
