DéplacementMNIST dans PyTorch
Achetez-moi un café☕
*Mon message explique Moving MNIST.
MovingMNIST() peut utiliser l'ensemble de données Moving MNIST comme indiqué ci-dessous :
*Mémos :
- Le 1er argument est root (Required-Type:str ou pathlib.Path). *Un chemin absolu ou relatif est possible.
- Le 2ème argument est split(Optional-Default:None-Type:str) :
*Mémos :
- Aucun, "train" ou "test" ne peut y être défini.
- Si ce n'est aucun, les 20 images (images) de chaque vidéo sont renvoyées, en ignorant split_ratio.
- Le 3ème argument est split_ratio(Optional-Default:10-Type:int) :
*Mémos :
- Si split est "train", data[:, :split_ratio] est renvoyé.
- Si split est "test", data[:, split_ratio:] est renvoyé.
- Si split est Aucun, il est ignoré. en ignorant split_ratio.
- Le 4ème argument est transform(Optional-Default:None-Type:callable).
- Le 5ème argument est download(Optional-Default:False-Type:bool) :
*Mémos :
- Si c'est vrai, l'ensemble de données est téléchargé depuis Internet vers la racine.
- Si c'est vrai et que l'ensemble de données est déjà téléchargé, il est extrait.
- Si c'est vrai et que l'ensemble de données est déjà téléchargé, rien ne se passe.
- Il devrait être faux si l'ensemble de données est déjà téléchargé car il est plus rapide.
- Vous pouvez télécharger et extraire manuellement l'ensemble de données à partir d'ici, par exemple. data/MovingMNIST/.
from torchvision.datasets import MovingMNIST all_data = MovingMNIST( root="data" ) all_data = MovingMNIST( root="data", split=None, split_ratio=10, download=False, transform=None ) train_data = MovingMNIST( root="data", split="train" ) test_data = MovingMNIST( root="data", split="test" ) len(all_data), len(train_data), len(test_data) # (10000, 10000, 10000) len(all_data[0]), len(train_data[0]), len(test_data[0]) # (20, 10, 10) all_data # Dataset MovingMNIST # Number of datapoints: 10000 # Root location: data all_data.root # 'data' print(all_data.split) # None all_data.split_ratio # 10 all_data.download # <bound method MovingMNIST.download of Dataset MovingMNIST # Number of datapoints: 10000 # Root location: data> print(all_data.transform) # None from torchvision.datasets import MovingMNIST import matplotlib.pyplot as plt plt.figure(figsize=(10, 3)) plt.subplot(1, 3, 1) plt.title("all_data") plt.imshow(all_data[0].squeeze()[0]) plt.subplot(1, 3, 2) plt.title("train_data") plt.imshow(train_data[0].squeeze()[0]) plt.subplot(1, 3, 3) plt.title("test_data") plt.imshow(test_data[0].squeeze()[0]) plt.show()
from torchvision.datasets import MovingMNIST all_data = MovingMNIST( root="data", split=None ) train_data = MovingMNIST( root="data", split="train" ) test_data = MovingMNIST( root="data", split="test" ) def show_images(data, main_title=None): plt.figure(figsize=(10, 8)) plt.suptitle(t=main_title, y=1.0, fontsize=14) for i, image in enumerate(data, start=1): plt.subplot(4, 5, i) plt.tight_layout(pad=1.0) plt.title(i) plt.imshow(image) plt.show() show_images(data=all_data[0].squeeze(), main_title="all_data") show_images(data=train_data[0].squeeze(), main_title="train_data") show_images(data=test_data[0].squeeze(), main_title="test_data")
from torchvision.datasets import MovingMNIST all_data = MovingMNIST( root="data", split=None ) train_data = MovingMNIST( root="data", split="train" ) test_data = MovingMNIST( root="data", split="test" ) import matplotlib.pyplot as plt def show_images(data, main_title=None): plt.figure(figsize=(10, 8)) plt.suptitle(t=main_title, y=1.0, fontsize=14) col = 5 for i, image in enumerate(data, start=1): plt.subplot(4, 5, i) plt.tight_layout(pad=1.0) plt.title(i) plt.imshow(image.squeeze()[0]) if i == col: break plt.show() show_images(data=all_data, main_title="all_data") show_images(data=train_data, main_title="train_data") show_images(data=test_data, main_title="test_data")
from torchvision.datasets import MovingMNIST import matplotlib.animation as animation all_data = MovingMNIST( root="data" ) import matplotlib.pyplot as plt from IPython.display import HTML figure, axis = plt.subplots() # ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ `ArtistAnimation()` ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ images = [] for image in all_data[0].squeeze(): images.append([axis.imshow(image)]) ani = animation.ArtistAnimation(fig=figure, artists=images, interval=100) # ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ `ArtistAnimation()` ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ # ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ `FuncAnimation()` ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ # def animate(i): # axis.imshow(all_data[0].squeeze()[i]) # # ani = animation.FuncAnimation(fig=figure, func=animate, # frames=20, interval=100) # ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ `FuncAnimation()` ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ # ani.save('result.gif') # Save the animation as a `.gif` file plt.ioff() # Hide a useless image # ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ Show animation ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ HTML(ani.to_jshtml()) # Animation operator # HTML(ani.to_html5_video()) # Animation video # ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ Show animation ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ # ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ Show animation ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ # plt.rcParams["animation.html"] = "jshtml" # Animation operator # plt.rcParams["animation.html"] = "html5" # Animation video # ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ Show animation ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑
from torchvision.datasets import MovingMNIST from ipywidgets import interact, IntSlider all_data = MovingMNIST( root="data" ) import matplotlib.pyplot as plt from IPython.display import HTML def func(i): plt.imshow(all_data[0].squeeze()[i]) interact(func, i=(0, 19, 1)) # interact(func, i=IntSlider(min=0, max=19, step=1, value=0)) # ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ Set the start value ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ ↑ plt.show()
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

Video Face Swap
Échangez les visages dans n'importe quelle vidéo sans effort grâce à notre outil d'échange de visage AI entièrement gratuit !

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)

Sujets chauds










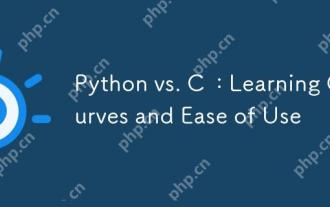
Python est plus facile à apprendre et à utiliser, tandis que C est plus puissant mais complexe. 1. La syntaxe Python est concise et adaptée aux débutants. Le typage dynamique et la gestion automatique de la mémoire le rendent facile à utiliser, mais peuvent entraîner des erreurs d'exécution. 2.C fournit des fonctionnalités de contrôle de bas niveau et avancées, adaptées aux applications haute performance, mais a un seuil d'apprentissage élevé et nécessite une gestion manuelle de la mémoire et de la sécurité.
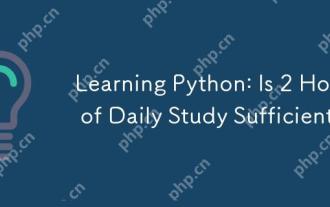
Est-ce suffisant pour apprendre Python pendant deux heures par jour? Cela dépend de vos objectifs et de vos méthodes d'apprentissage. 1) Élaborer un plan d'apprentissage clair, 2) Sélectionnez les ressources et méthodes d'apprentissage appropriées, 3) la pratique et l'examen et la consolidation de la pratique pratique et de l'examen et de la consolidation, et vous pouvez progressivement maîtriser les connaissances de base et les fonctions avancées de Python au cours de cette période.
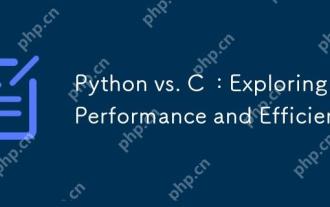
Python est meilleur que C dans l'efficacité du développement, mais C est plus élevé dans les performances d'exécution. 1. La syntaxe concise de Python et les bibliothèques riches améliorent l'efficacité du développement. Les caractéristiques de type compilation et le contrôle du matériel de CC améliorent les performances d'exécution. Lorsque vous faites un choix, vous devez peser la vitesse de développement et l'efficacité de l'exécution en fonction des besoins du projet.
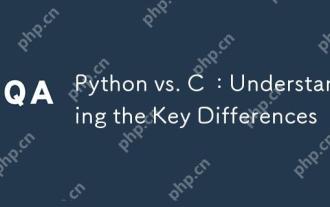
Python et C ont chacun leurs propres avantages, et le choix doit être basé sur les exigences du projet. 1) Python convient au développement rapide et au traitement des données en raison de sa syntaxe concise et de son typage dynamique. 2) C convient à des performances élevées et à une programmation système en raison de son typage statique et de sa gestion de la mémoire manuelle.
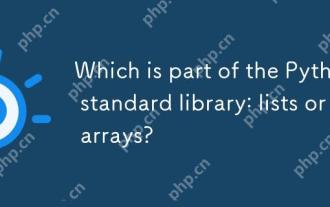
PythonlistSaReparmentofthestandardLibrary, tandis que les coloccules de colocède, tandis que les colocculations pour la base de la Parlementaire, des coloments de forage polyvalent, tandis que la fonctionnalité de la fonctionnalité nettement adressée.
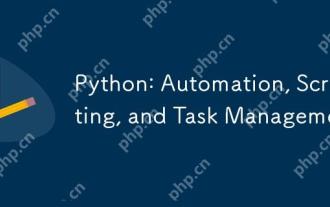
Python excelle dans l'automatisation, les scripts et la gestion des tâches. 1) Automatisation: La sauvegarde du fichier est réalisée via des bibliothèques standard telles que le système d'exploitation et la fermeture. 2) Écriture de script: utilisez la bibliothèque PSUTIL pour surveiller les ressources système. 3) Gestion des tâches: utilisez la bibliothèque de planification pour planifier les tâches. La facilité d'utilisation de Python et la prise en charge de la bibliothèque riche en font l'outil préféré dans ces domaines.
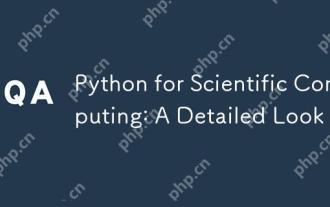
Les applications de Python en informatique scientifique comprennent l'analyse des données, l'apprentissage automatique, la simulation numérique et la visualisation. 1.Numpy fournit des tableaux multidimensionnels et des fonctions mathématiques efficaces. 2. Scipy étend la fonctionnalité Numpy et fournit des outils d'optimisation et d'algèbre linéaire. 3. Pandas est utilisé pour le traitement et l'analyse des données. 4.Matplotlib est utilisé pour générer divers graphiques et résultats visuels.
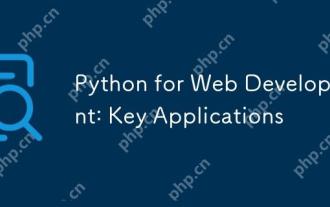
Les applications clés de Python dans le développement Web incluent l'utilisation des cadres Django et Flask, le développement de l'API, l'analyse et la visualisation des données, l'apprentissage automatique et l'IA et l'optimisation des performances. 1. Framework Django et Flask: Django convient au développement rapide d'applications complexes, et Flask convient aux projets petits ou hautement personnalisés. 2. Développement de l'API: Utilisez Flask ou DjangorestFramework pour construire RestulAPI. 3. Analyse et visualisation des données: utilisez Python pour traiter les données et les afficher via l'interface Web. 4. Apprentissage automatique et AI: Python est utilisé pour créer des applications Web intelligentes. 5. Optimisation des performances: optimisée par la programmation, la mise en cache et le code asynchrones
