


Comment analyser une chaîne de requête URI dans une collection nom-valeur en Java ?
Analyser une chaîne URI dans une collection nom-valeur
Vue d'ensemble
Lorsque vous traitez des URI (Uniform Resource Identifiers), il est souvent utile d'analyser le chaîne de requête dans une collection de paires nom-valeur. En Java, il n'existe pas de méthode intégrée équivalente à la méthode C#/.NET HttpUtility.ParseQueryString. Cependant, il existe différentes manières d'y parvenir à l'aide d'un code personnalisé.
Utilisation d'une méthode personnalisée
Une façon d'analyser une chaîne URI dans une carte consiste à créer une méthode personnalisée. Voici une version simplifiée :
public static Map<String, String> splitQuery(URL url) throws UnsupportedEncodingException { Map<String, String> queryPairs = new LinkedHashMap<>(); String query = url.getQuery(); String[] pairs = query.split("&"); for (String pair : pairs) { int idx = pair.indexOf("="); queryPairs.put(URLDecoder.decode(pair.substring(0, idx), "UTF-8"), URLDecoder.decode(pair.substring(idx + 1), "UTF-8")); } return queryPairs; }
Méthode améliorée avec décodage d'URL et gestion de plusieurs paramètres
La méthode ci-dessus a été mise à jour pour gérer plusieurs paramètres avec la même clé et des paramètres sans valeur. Voici la version améliorée :
public static Map<String, List<String>> splitQuery(URL url) throws UnsupportedEncodingException { final Map<String, List<String>> queryPairs = new LinkedHashMap<>(); final String[] pairs = url.getQuery().split("&"); for (String pair : pairs) { final int idx = pair.indexOf("="); final String key = idx > 0 ? URLDecoder.decode(pair.substring(0, idx), "UTF-8") : pair; if (!queryPairs.containsKey(key)) { queryPairs.put(key, new LinkedList<>()); } final String value = idx > 0 && pair.length() > idx + 1 ? URLDecoder.decode(pair.substring(idx + 1), "UTF-8") : null; queryPairs.get(key).add(value); } return queryPairs; }
Version Java 8
Voici une version Java 8 de la méthode :
public Map<String, List<String>> splitQuery(URL url) { if (Strings.isNullOrEmpty(url.getQuery())) { return Collections.emptyMap(); } return Arrays.stream(url.getQuery().split("&")) .map(this::splitQueryParameter) .collect(Collectors.groupingBy(SimpleImmutableEntry::getKey, LinkedHashMap::new, mapping(Map.Entry::getValue, toList()))); } public SimpleImmutableEntry<String, String> splitQueryParameter(String it) { final int idx = it.indexOf("="); final String key = idx > 0 ? it.substring(0, idx) : it; final String value = idx > 0 && it.length() > idx + 1 ? it.substring(idx + 1) : null; return new SimpleImmutableEntry<>(URLDecoder.decode(key, StandardCharsets.UTF_8), URLDecoder.decode(value, StandardCharsets.UTF_8)); }
Exemple d'utilisation
Pour utilisez la méthode splitQuery, transmettez simplement un objet URL et il renverra une carte contenant la chaîne de requête analysée paramètres :
URL url = new URL("https://google.com.ua/oauth/authorize?client_id=SS&response_type=code&scope=N_FULL&access_type=offline&redirect_uri=http://localhost/Callback"); Map<String, String> queryParameters = splitQuery(url);
L'accès aux valeurs de la carte est simple :
String clientId = queryParameters.get("client_id"); // SS
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

Video Face Swap
Échangez les visages dans n'importe quelle vidéo sans effort grâce à notre outil d'échange de visage AI entièrement gratuit !

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)

Sujets chauds
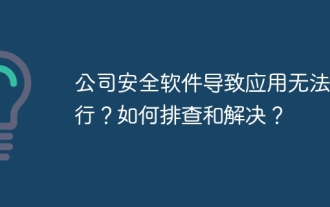
Dépannage et solutions au logiciel de sécurité de l'entreprise qui fait que certaines applications ne fonctionnent pas correctement. De nombreuses entreprises déploieront des logiciels de sécurité afin d'assurer la sécurité des réseaux internes. ...
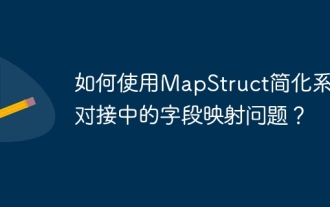
Le traitement de la cartographie des champs dans l'amarrage du système rencontre souvent un problème difficile lors de l'exécution d'amarrage du système: comment cartographier efficacement les champs d'interface du système a ...
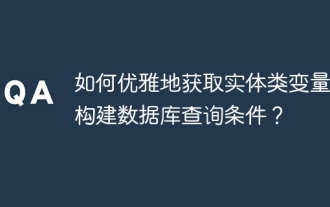
Lorsque vous utilisez MyBatis-Plus ou d'autres cadres ORM pour les opérations de base de données, il est souvent nécessaire de construire des conditions de requête en fonction du nom d'attribut de la classe d'entité. Si vous manuellement à chaque fois ...
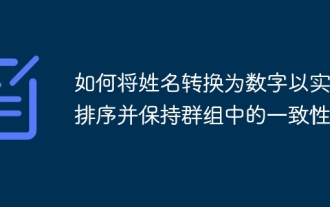
Solutions pour convertir les noms en nombres pour implémenter le tri dans de nombreux scénarios d'applications, les utilisateurs peuvent avoir besoin de trier en groupe, en particulier en un ...
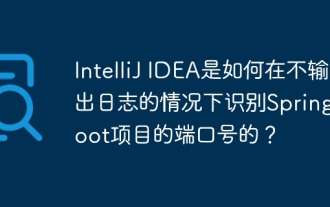
Commencez le printemps à l'aide de la version IntelliJideaultimate ...
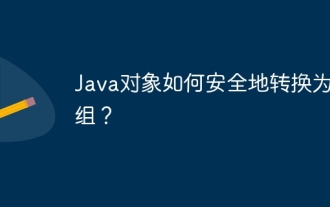
Conversion des objets et des tableaux Java: Discussion approfondie des risques et des méthodes correctes de la conversion de type de distribution De nombreux débutants Java rencontreront la conversion d'un objet en un tableau ...
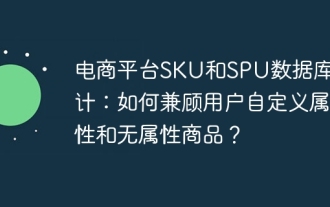
Explication détaillée de la conception des tables SKU et SPU sur les plates-formes de commerce électronique Cet article discutera des problèmes de conception de la base de données de SKU et SPU dans les plateformes de commerce électronique, en particulier comment gérer les ventes définies par l'utilisateur ...
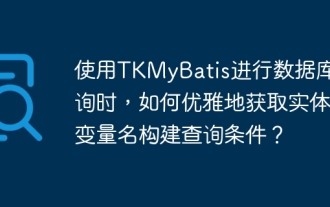
Lorsque vous utilisez TkMyBatis pour les requêtes de base de données, comment obtenir gracieusement les noms de variables de classe d'entité pour créer des conditions de requête est un problème courant. Cet article épinglera ...
