


Diminution de la charge du serveur en utilisant la technique anti-rebond/accélérateur dans Reactjs
Anti-rebond et limitation dans React.js
L'anti-rebond et la limitation sont des techniques utilisées pour optimiser les performances en contrôlant la fréquence d'exécution des fonctions en réponse à des événements fréquents (par exemple, saisie, défilement, redimensionnement).
1. Qu’est-ce que l’anti-rebond ?
L'anti-rebond retarde l'exécution d'une fonction jusqu'à ce qu'un certain laps de temps se soit écoulé depuis la dernière fois qu'elle a été invoquée.
Exemple de cas d'utilisation :
- Champ de saisie de recherche : évitez de déclencher un appel API à chaque frappe.
Exemple anti-rebond dans React
import React, { useState, useEffect } from 'react'; const DebouncedSearch = () => { const [searchTerm, setSearchTerm] = useState(''); const [debouncedValue, setDebouncedValue] = useState(''); useEffect(() => { const handler = setTimeout(() => { setDebouncedValue(searchTerm); }, 500); // Delay of 500ms return () => { clearTimeout(handler); // Cleanup on input change }; }, [searchTerm]); useEffect(() => { if (debouncedValue) { console.log('API Call with:', debouncedValue); // Make API call here } }, [debouncedValue]); return ( <input type="text" placeholder="Search..." value={searchTerm} onChange={(e) => setSearchTerm(e.target.value)} /> ); }; export default DebouncedSearch;
2. Qu’est-ce que la limitation ?
La limitation garantit qu'une fonction est exécutée au plus une fois dans un intervalle de temps spécifié, quel que soit le nombre de fois où elle est déclenchée pendant cet intervalle.
Exemple de cas d'utilisation :
- Événement de défilement : Limitez la fréquence d'une fonction déclenchée lors d'un événement de défilement pour améliorer les performances.
Exemple de limitation dans React
import React, { useEffect } from 'react'; const ThrottledScroll = () => { useEffect(() => { const handleScroll = throttle(() => { console.log('Scroll event fired'); }, 1000); // Execute at most once every 1000ms window.addEventListener('scroll', handleScroll); return () => { window.removeEventListener('scroll', handleScroll); }; }, []); return <div> <hr> <h3> <strong>3. Using Libraries (Optional)</strong> </h3> <p>Instead of implementing custom debounce/throttle, you can use popular libraries like:</p> <h4> <strong>Lodash</strong> </h4> <p>Install:<br> </p> <pre class="brush:php;toolbar:false">npm install lodash
Utilisation :
import { debounce, throttle } from 'lodash'; // Debounce Example const debouncedFunc = debounce(() => console.log('Debounced!'), 500); // Throttle Example const throttledFunc = throttle(() => console.log('Throttled!'), 1000);
Réagir-utiliser
Installer :
npm install react-use
Utilisation :
import { useDebounce, useThrottle } from 'react-use'; const Demo = () => { const [value, setValue] = useState(''); const debouncedValue = useDebounce(value, 500); const throttledValue = useThrottle(value, 1000); useEffect(() => { console.log('Debounced:', debouncedValue); console.log('Throttled:', throttledValue); }, [debouncedValue, throttledValue]); return ( <input value={value} onChange={(e) => setValue(e.target.value)} placeholder="Type something..." /> ); }; export default Demo;
Différences clés
Feature | Debouncing | Throttling |
---|---|---|
Execution | Executes once after the user stops firing events for a specified time. | Executes at regular intervals during the event. |
Use Cases | Search input, resizing, form validation. | Scroll events, button clicks, API polling. |
Performance | Reduces the number of function calls. | Limits execution to once per interval. |
Anti-rebond
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

Video Face Swap
Échangez les visages dans n'importe quelle vidéo sans effort grâce à notre outil d'échange de visage AI entièrement gratuit !

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)

Sujets chauds










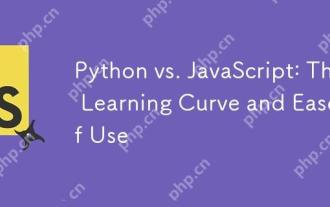
Python convient plus aux débutants, avec une courbe d'apprentissage en douceur et une syntaxe concise; JavaScript convient au développement frontal, avec une courbe d'apprentissage abrupte et une syntaxe flexible. 1. La syntaxe Python est intuitive et adaptée à la science des données et au développement back-end. 2. JavaScript est flexible et largement utilisé dans la programmation frontale et côté serveur.
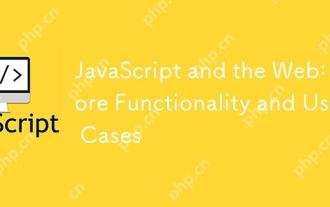
Les principales utilisations de JavaScript dans le développement Web incluent l'interaction client, la vérification du formulaire et la communication asynchrone. 1) Mise à jour du contenu dynamique et interaction utilisateur via les opérations DOM; 2) La vérification du client est effectuée avant que l'utilisateur ne soumette les données pour améliorer l'expérience utilisateur; 3) La communication de rafraîchissement avec le serveur est réalisée via la technologie AJAX.
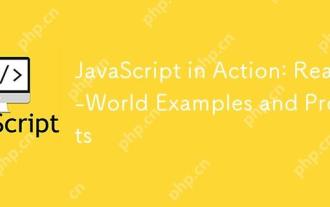
L'application de JavaScript dans le monde réel comprend un développement frontal et back-end. 1) Afficher les applications frontales en créant une application de liste TODO, impliquant les opérations DOM et le traitement des événements. 2) Construisez RestulAPI via Node.js et Express pour démontrer les applications back-end.
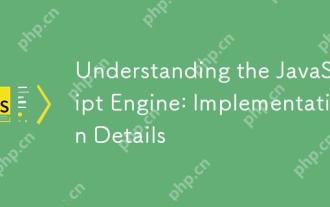
Comprendre le fonctionnement du moteur JavaScript en interne est important pour les développeurs car il aide à écrire du code plus efficace et à comprendre les goulots d'étranglement des performances et les stratégies d'optimisation. 1) Le flux de travail du moteur comprend trois étapes: analyse, compilation et exécution; 2) Pendant le processus d'exécution, le moteur effectuera une optimisation dynamique, comme le cache en ligne et les classes cachées; 3) Les meilleures pratiques comprennent l'évitement des variables globales, l'optimisation des boucles, l'utilisation de const et de locations et d'éviter une utilisation excessive des fermetures.
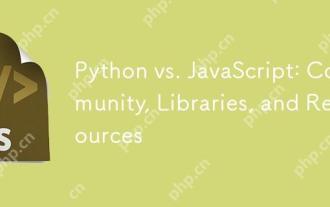
Python et JavaScript ont leurs propres avantages et inconvénients en termes de communauté, de bibliothèques et de ressources. 1) La communauté Python est amicale et adaptée aux débutants, mais les ressources de développement frontal ne sont pas aussi riches que JavaScript. 2) Python est puissant dans les bibliothèques de science des données et d'apprentissage automatique, tandis que JavaScript est meilleur dans les bibliothèques et les cadres de développement frontaux. 3) Les deux ont des ressources d'apprentissage riches, mais Python convient pour commencer par des documents officiels, tandis que JavaScript est meilleur avec MDNWEBDOCS. Le choix doit être basé sur les besoins du projet et les intérêts personnels.
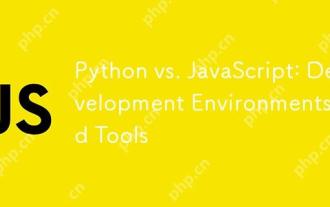
Les choix de Python et JavaScript dans les environnements de développement sont importants. 1) L'environnement de développement de Python comprend Pycharm, Jupyternotebook et Anaconda, qui conviennent à la science des données et au prototypage rapide. 2) L'environnement de développement de JavaScript comprend Node.js, VScode et WebPack, qui conviennent au développement frontal et back-end. Le choix des bons outils en fonction des besoins du projet peut améliorer l'efficacité du développement et le taux de réussite du projet.
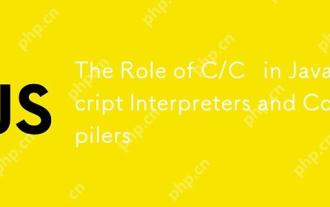
C et C jouent un rôle essentiel dans le moteur JavaScript, principalement utilisé pour implémenter des interprètes et des compilateurs JIT. 1) C est utilisé pour analyser le code source JavaScript et générer une arborescence de syntaxe abstraite. 2) C est responsable de la génération et de l'exécution de bytecode. 3) C met en œuvre le compilateur JIT, optimise et compile le code de point chaud à l'exécution et améliore considérablement l'efficacité d'exécution de JavaScript.

Python est plus adapté à la science et à l'automatisation des données, tandis que JavaScript est plus adapté au développement frontal et complet. 1. Python fonctionne bien dans la science des données et l'apprentissage automatique, en utilisant des bibliothèques telles que Numpy et Pandas pour le traitement et la modélisation des données. 2. Python est concis et efficace dans l'automatisation et les scripts. 3. JavaScript est indispensable dans le développement frontal et est utilisé pour créer des pages Web dynamiques et des applications à une seule page. 4. JavaScript joue un rôle dans le développement back-end via Node.js et prend en charge le développement complet de la pile.
