PHP 13: 类
Notice: This article is working on progress!
本章将介绍PHP类.
现在,基本上每种语言都支持面向对象,当然PHP也不列外。
PHP在以前的版本不支持面向对象,但自从PHP4,包括PHP4之后开始支持了。本系列将以PHP5为例来描述面向对象的知识。在后面也将提及PHP4的知识。
PHP对面向对象的支持的能力,以我个人观点,没有C++,C#,Java等语言那么深入,即使如此,也算得上是比较全面的。
你问问现在的每一个人面向对象的特点是什么?他们肯定回答封装,多态,继承,没错,就是这些。
类
好了,我们从类开始说起吧。类的概念不说了,世人都知道。
PHP中的类可以这样写:
php
class Page
{
}
?>
你也可以定义属性和方法,如下:
php
class Page
{
// 2 attributes
var $attribute1 ;
var $attribute2 ;
// 2 functions
function Operation1()
{
}
function Operation2( $param1 , $param2 )
{
}
}
?>
构造用__construct();析构用__destruct();( 注意:前面是2个下划线)
代码如下:
1 php
2 class Page
3 {
4 // 2 attributes
5 var $attribute1 ;
6 var $attribute2 ;
7
8 // 2 functions
9 function Operation1()
10 {
11 }
12
13 function Operation2( $param1 , $param2 )
14 {
15 }
16
17 // construction function
18 function __construct( $param )
19 {
20 $this -> attribute1 = $param ;
21 }
22
23 // destruction function
24 function __destruct()
25 {
26 $this -> attribute1 = 0 ;
27 }
28 }
29 ?>
类的实例化
构建了类,我们就需要应用它,如何使用?实例化,这个和其他语言一样,所以只给出代码:
1 php
2 class Page
3 {
4 // 2 attributes
5 var $attribute1 ;
6 var $attribute2 ;
7
8 // 2 functions
9 function Operation1()
10 {
11 }
12
13 function Operation2( $param1 , $param2 )
14 {
15 }
16
17 // construction function
18 function __construct( $param )
19 {
20 $this -> attribute1 = $param ;
21 echo " It will construct the attribute: {$this->attribute1}
" ;
22 }
23
24 // destruction function
25 function __destruct()
26 {
27 $this -> attribute1 = 0 ;
28 }
29 }
30 $instance1 = new Page( " attribute1 in instance 1 " );
31 $instance2 = new Page( " attribute2 in instance 2 " );
32 $instance1 = new Page();
33 ?>
1 It will construct the attribute : attribute1 in instance 1
2 It will construct the attribute : attribute2 in instance 2
3 It will construct the attribute :
设置或得到属性
这个问题很有意思。
拿上面的例子来说, $attribute1 就是一个属性,我们可以利用 $this -> attribute1 来获取或设置其值。
但是为了更好的体现类的封装性,还是采用PHP提供的get或set方法,这个和C#里的功能这样。但是在PHP中,提供2个方法:__get($name),__set($name,$value)函数来设置属性。
这样做的另外一个好处就是根据实际需要,加入自己的逻辑,如果直接赋值的话就不能办到。比如说属性一定要是1-100之间的数,怎么办?还是采用__set吧。看看代码吧:
1 2 class Page
3 {
4 //2 attributes
5 var $attribute1;
6 var $attribute2;
7
8 //2 functions
9 function Operation1()
10 {
11 }
12
13 function Operation2($param1,$param2)
14 {
15 }
16
17 //construction function
18 function __construct($param)
19 {
20 $this->attribute1=$param;
21 echo "It will construct the attribute: {$this->attribute1}
";
22 }
23
24 //destruction function
25 function __destruct()
26 {
27 $this->attribute1=0;
28 }
29 function __set($name,$value)
30 {
31 if($name==="attribute2"&&$value>=2&&attribute232 {
33 $this->attribute2=$value;
34 }
35 }
36 function __get($name)
37 {
38 return $this->$name;
39 }
40 }
41 $instance1=new Page("attribute1 in instance 1");
42 $instance2=new Page("attribute1 in instance 1");
43 $instance3=new Page();
44 $instance3->attribute2=99;
45 echo $instance3->attribute2;
46 ?>
类成员和方法的可视性
还是public,private以及protected。和C#的作用一样。但是需要注意的是,PHP默认的是public,而不是大多数语言默认的private。基于此,上述的例子才可以使用。还需要注意的一点是,如果使用了这3个关键字,var就省略了。看个例子吧。
1 2 class Page
3 {
4 //2 attributes
5 public $attribute1;
6 public $attribute2;
7
8 //2 functions
9 public function Operation1()
10 {
11 }
12
13 public function Operation2($param1,$param2)
14 {
15 }
16
17 //construction function
18 public function __construct($param)
19 {
20 $this->attribute1=$param;
21 echo "It will construct the attribute: {$this->attribute1}
";
22 }
23
24 //destruction function
25 public function __destruct()
26 {
27 $this->attribute1=0;
28 }
29 public function __set($name,$value)
30 {
31 if($name==="attribute2"&&$value>=2&&attribute232 {
33 $this->attribute2=$value;
34 }
35 }
36 public function __get($name)
37 {
38 return $this->$name;
39 }
40 }
41 $instance1=new Page("attribute1 in instance 1");
42 $instance2=new Page("attribute1 in instance 1");
43 $instance3=new Page();
44 $instance3->attribute2=99;
45 echo $instance3->attribute2;
46 ?>
类的继承
类的继承和C#,Java语言一样,不支持多重继承。PHP实用extends来继承一个类,和Java有些类似。
在Java里有final这个词,PHP里也有,并且作用相似,就是为了阻止继承或重载,重写方法。
提到继承,那么PHP支持接口吗?支持。例如:
1 php
2 interface IDispose
3 {
4 function Dispose();
5 }
6
7 class page extends IDispose
8 {
9 function Dispose()
10 {
11 // Your code here.
12 }
13 }
14 ?>
1. Per-class常量
它是在PHP5引入的。这个常理不需要再初始化。例如:
1 2 class Math
3 {
4 const pi=3.1415927;
5 }
6 echo "Math::pi='.Math::pi."\n";
7 ?>
2. 静态函数
既然说到了静态变量,当然也要说静态函数了。它和上面的思想一样,实现时需要加static关键字,好多语言都是这样的。C#,Java等。
代码可以如下:
1 2 class Shape
3 {
4 static function SquredSize($length)
5 {
6 return $length*$length;
7 }
8 }
9 echo "Squre:".Shape::SquredSize(10);
10 ?>
你要想判断一个类是不是某个类的类型。非常简单,就是instanceof,如果你使用过java,那么你非常熟悉了,因为和Java一样。如果你是用C#的,类似于C# 里的is。
4. 克隆对象
PHP提供一个关键字,clone,就是这个功能。我看PHP是我见到语言中克隆对象比较简单的语言之一。代码如下:
$c = clone $b
如果你想改变$c的某一特性,怎么办?这个时候你需要重写__clone方法。5. 抽象类
PHP也支持抽象类,并且和C#等语言非常类似。给个例子吧。
1 2 abstract class Shape
3 {
4 abstract protected function getSquared();
5 abstract protected function getLineCount();
6 public function printShapeValue()
7 {
8 echo "The squared size is :{$this->getSquared()}
";
9 echo "And the number of lines is :{$this->getLineCount()}
";
10 }
11 }
12
13 class Rectange extends Shape
14 {
15 protected function getSquared()
16 {
17 return 12*12;
18 }
19 protected function getLineCount()
20 {
21 return 4;
22 }
23 }
24
25 class Triangle extends Shape
26 {
27 protected function getSquared()
28 {
29 return 10*12;
30 }
31 protected function getLineCount()
32 {
33 return 3;
34 }
35 }
36
37 $rect=new Rectange();
38 $rect->printShapeValue();
39
40 $tri=new Triangle();
41 $tri->printShapeValue();
42 ?>
The squared size is : 144
And the number of lines is : 4
The squared size is : 120
And the number of lines is : 3
6. 使用__call重载
这是我认为PHP提供的一个非常cool的功能,非常的佩服。这个方法用来监视一个对象中的其它方法。如果你试着调用一个对象中不存在的方法,__call 方法将会被自动调用。
举个例子吧。代码如下:
1 2 class Caller
3 {
4 private $x = array(1, 2, 3);
5
6 public function __call($m, $a)
7 {
8 print "Method $m called:\n";
9 var_dump($a);
10 return $this->x;
11 }
12 }
13
14 $foo = new Caller();
15 $a = $foo->test1(1, "2", 3.4, true);
16 var_dump($a);
17 ?>
Method test1 called : array ( 4 ) { [ 0 ] => int( 1 ) [ 1 ] => string ( 1 ) " 2 " [ 2 ] => float ( 3.4 ) [ 3 ] => bool( true ) } array ( 3 ) { [ 0 ] => int( 1 ) [ 1 ] => int( 2 ) [ 2 ] => int( 3 ) }
$foo->test1这个方法在foo类里不存在的,但是定义了__call之后,就可以了。7. 使用__autoload()方法。
这个功能仍然很cool。很多开发者写面向象的应用程序时对对每个类的定义建立一个 PHP 源文件。一个很大的痛苦是不得不在每个脚本(每个类一个文件)开头写一个长长的包含文件列表。在 PHP 5 中,我们再也不需要这样了。 __autoload 函数就能轻松搞定,当代码视图使用未定义的类时,此方法自动调用。通过调用此函数,脚本引擎在出错失败之前就有了最后一个补救的机会来加载所需的类。但是需要注意的是 __autoload 函数抛出的异常不能被 catch 语句块捕获并导致致命错误。
一个例子:
1 php
2 function __autoload( $class_name ) {
3 require_once $class_name . ' .php ' ;
4 }
5
6 $obj1 = new MyClass1();
7 $obj2 = new MyClass2();
8 ?>
它将迭代类的所有可见的属性以及值等
9 . 将类型转化为字符串(__toString()).
这是一个魔术函数的应用,__toString().它有点像C#里的ToString()函数一样,但是对于类本身来说还是有区别的。如果你在PHP的类里实现了该函数,它们载打印这个类时就会调用此函数,例如:
1 php
2 class Page
3 {
4 public function __toString()
5 {
6 return ' Hello ' ;
7 }
8 }
9 $page = new Page();
10 echo $page ;
11 ?>
Hello
10. 反射。没想到PHP也支持反射的。如果你对C#的反射熟悉的话,它们我就不用说了。如果不是,请继续往下看。
反射是通过类或对象查找它包含的内容的信息的能力。当此类的文档信息不详或没有提供时,可以通过反射得到类的一些属性,方法等信息。
反射继承了Zend的一些类。具体实用请参看PHP反射的类的文档。

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

AI Hentai Generator
Générez AI Hentai gratuitement.

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)
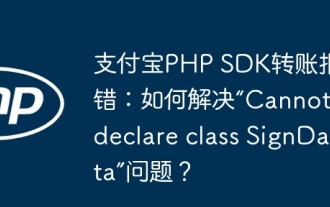
Alipay Php ...
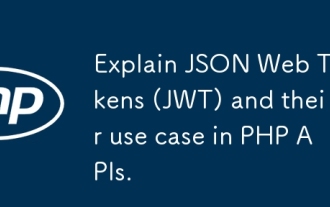
JWT est une norme ouverte basée sur JSON, utilisée pour transmettre en toute sécurité des informations entre les parties, principalement pour l'authentification de l'identité et l'échange d'informations. 1. JWT se compose de trois parties: en-tête, charge utile et signature. 2. Le principe de travail de JWT comprend trois étapes: la génération de JWT, la vérification de la charge utile JWT et l'analyse. 3. Lorsque vous utilisez JWT pour l'authentification en PHP, JWT peut être généré et vérifié, et les informations sur le rôle et l'autorisation des utilisateurs peuvent être incluses dans l'utilisation avancée. 4. Les erreurs courantes incluent une défaillance de vérification de signature, l'expiration des jetons et la charge utile surdimensionnée. Les compétences de débogage incluent l'utilisation des outils de débogage et de l'exploitation forestière. 5. L'optimisation des performances et les meilleures pratiques incluent l'utilisation des algorithmes de signature appropriés, la définition des périodes de validité raisonnablement,
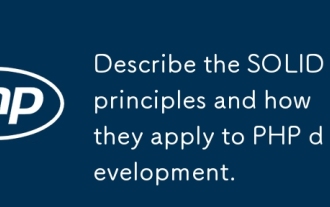
L'application du principe solide dans le développement de PHP comprend: 1. Principe de responsabilité unique (SRP): Chaque classe n'est responsable d'une seule fonction. 2. Principe ouvert et ferme (OCP): les changements sont réalisés par extension plutôt que par modification. 3. Principe de substitution de Lisch (LSP): les sous-classes peuvent remplacer les classes de base sans affecter la précision du programme. 4. Principe d'isolement d'interface (ISP): utilisez des interfaces à grain fin pour éviter les dépendances et les méthodes inutilisées. 5. Principe d'inversion de dépendance (DIP): les modules élevés et de bas niveau reposent sur l'abstraction et sont mis en œuvre par injection de dépendance.
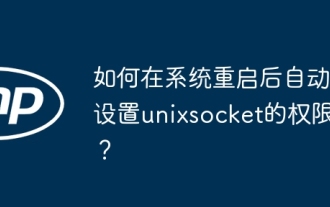
Comment définir automatiquement les autorisations d'UnixSocket après le redémarrage du système. Chaque fois que le système redémarre, nous devons exécuter la commande suivante pour modifier les autorisations d'UnixSocket: sudo ...
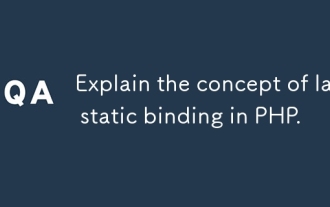
L'article traite de la liaison statique tardive (LSB) dans PHP, introduite dans PHP 5.3, permettant une résolution d'exécution de la méthode statique nécessite un héritage plus flexible. Problème main: LSB vs polymorphisme traditionnel; Applications pratiques de LSB et perfo potentiel
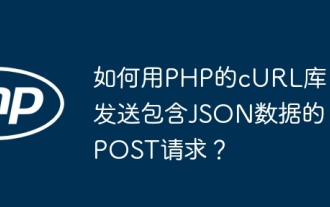
Envoyant des données JSON à l'aide de la bibliothèque Curl de PHP dans le développement de PHP, il est souvent nécessaire d'interagir avec les API externes. L'une des façons courantes consiste à utiliser la bibliothèque Curl pour envoyer le post� ...
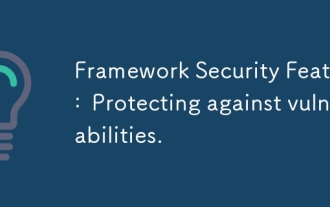
L'article traite des fonctionnalités de sécurité essentielles dans les cadres pour se protéger contre les vulnérabilités, notamment la validation des entrées, l'authentification et les mises à jour régulières.
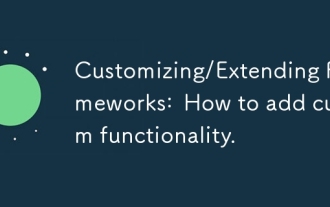
L'article examine l'ajout de fonctionnalités personnalisées aux cadres, en se concentrant sur la compréhension de l'architecture, l'identification des points d'extension et les meilleures pratiques pour l'intégration et le débogage.
