


Explication détaillée de JavaScript cet exemple de code de pointeur
La signification de ceci en JavaScript est très riche. Il peut s'agir de l'objet global, de l'objet courant ou de n'importe quel objet, selon la manière dont la fonction est appelée. Les fonctions peuvent être appelées des manières suivantes : en tant que méthode objet, en tant que fonction, en tant que constructeur, appliquer ou appeler.
Appel de méthode objet
Lorsqu'il est appelé en tant que méthode objet, celui-ci sera lié à l'objet.
var point = { x : 0, y : 0, moveTo : function(x, y) { this.x = this.x + x; this.y = this.y + y; } }; point.moveTo(1, 1)//this 绑定到当前对象,即 point 对象
Je voudrais souligner une chose ici, c'est-à-dire que this obtient la valeur correspondante lorsque la fonction est exécutée, pas lorsque la fonction est définie. Même s'il s'agit d'un appel de méthode objet, si l'attribut de fonction de la méthode est passé dans une autre portée sous la forme d'un nom de fonction, le pointeur de celle-ci sera modifié. Laissez-moi vous donner un exemple :
var a = { aa : 0, bb : 0, fun : function(x,y){ this.aa = this.aa + x; this.bb = this.bb + y; } }; var aa = 1; var b = { aa:0, bb:0, fun : function(){return this.aa;} } a.fun(3,2); document.write(a.aa);//3,this指向对象本身 document.write(b.fun());//0,this指向对象本身 (function(aa){//注意传入的是函数,而不是函数执行的结果 var c = aa(); document.write(c);//1 , 由于fun在该处执行,导致this不再指向对象本身,而是这里的window })(b.fun);
C'est tout. Cela peut être un endroit déroutant.
Appel de fonction
La fonction peut également être appelée directement à ce stade, celle-ci est liée à l'objet global.
var x = 1; function test(){ this.x = 0; } test(); alert(x); //0
Mais cela posera quelques problèmes, c'est-à-dire que pour les fonctions définies à l'intérieur de la fonction, cela pointera également vers le monde global, ce qui est exactement le contraire de ce que nous voulons. Le code est le suivant :
var point = { x : 0, y : 0, moveTo : function(x, y) { // 内部函数 var moveX = function(x) { this.x = x;//this 绑定到了全局 }; // 内部函数 var moveY = function(y) { this.y = y;//this 绑定到了全局 }; moveX(x); moveY(y); } }; point.moveTo(1, 1); point.x; //==>0 point.y; //==>0 x; //==>1 y; //==>1
Nous constaterons que non seulement l'effet de mouvement que nous souhaitons n'est pas terminé, mais qu'il y aura deux autres variables globales. Alors comment le résoudre ? Enregistrez-le simplement dans une variable lors de la saisie d'une fonction dans une fonction, puis utilisez la variable. Le code est le suivant :
var point = { x : 0, y : 0, moveTo : function(x, y) { var that = this; // 内部函数 var moveX = function(x) { that.x = x; }; // 内部函数 var moveY = function(y) { that.y = y; } moveX(x); moveY(y); } }; point.moveTo(1, 1); point.x; //==>1 point.y; //==>1
Appel du constructeur
Lorsque vous créez vous-même le constructeur en JavaScript, vous pouvez l'utiliser pour pointer vers l'objet nouvellement créé. Cela empêchera la fonction de pointer vers le monde global.
var x = 2; function test(){ this.x = 1; } var o = new test(); alert(x); //2
appliquer ou appeler
Ces deux méthodes peuvent changer le contexte d'exécution de la fonction, c'est-à-dire modifier la liaison de cet objet . Apply et call sont similaires. La différence est que lors de la transmission des paramètres, une exigence est un tableau et l'autre exigence est qu'ils soient transmis séparément. Prenons donc apply comme exemple :
<pre name="code" class="html">var name = "window"; var someone = { name: "Bob", showName: function(){ alert(this.name); } }; var other = { name: "Tom" }; someone.showName(); //Bob someone.showName.apply(); //window someone.showName.apply(other); //Tom
Vous pouvez voir que lorsque vous accédez normalement à la méthode dans l'objet, cela pointe vers l'objet. Après avoir utilisé apply, lorsque apply n'a pas de paramètres, l'objet actuel de this est l'objet global. Lorsque apply a des paramètres, l'objet actuel de this est le paramètre.
Appel de la fonction flèche
Une chose qui doit être ajoutée ici est que dans la norme javascript ES6 de nouvelle génération, la fonction flèche ceci pointe toujours vers this lorsque la fonction est définie, et non vers lorsqu'elle est exécutée. Comprenons à travers un exemple :
var o = { x : 1, func : function() { console.log(this.x) }, test : function() { setTimeout(function() { this.func(); }, 100); } }; o.test(); // TypeError : this.func is not a function
Le code ci-dessus provoquera une erreur car le pointeur de ceci passe de o au global. Nous devons modifier le code ci-dessus comme suit :
var o = { x : 1, func : function() { console.log(this.x) }, test : function() { var _this = this; setTimeout(function() { _this.func(); }, 100); } }; o.test();
Utilisez simplement celui-ci enregistré en externe à l'avance. Les fonctions fléchées peuvent être utilisées ici. Comme nous venons de le dire, le ceci de la fonction flèche pointe toujours vers le ceci< lorsque le la fonction est définie 🎜>, pas lorsqu'elle est exécutée. Nous modifions donc le code ci-dessus comme suit :
var o = { x : 1, func : function() { console.log(this.x) }, test : function() { setTimeout(() => { this.func() }, 100); } }; o.test();
var x = 1, o = { x : 10, test : () => this.x }; o.test(); // 1 o.test.call(o); // 依然是1
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

AI Hentai Generator
Générez AI Hentai gratuitement.

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)

Sujets chauds
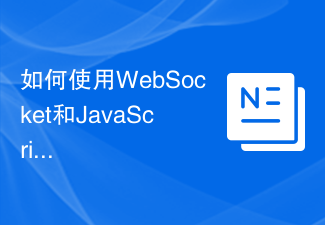
Comment utiliser WebSocket et JavaScript pour mettre en œuvre un système de reconnaissance vocale en ligne Introduction : Avec le développement continu de la technologie, la technologie de reconnaissance vocale est devenue une partie importante du domaine de l'intelligence artificielle. Le système de reconnaissance vocale en ligne basé sur WebSocket et JavaScript présente les caractéristiques d'une faible latence, d'un temps réel et d'une multiplateforme, et est devenu une solution largement utilisée. Cet article explique comment utiliser WebSocket et JavaScript pour implémenter un système de reconnaissance vocale en ligne.
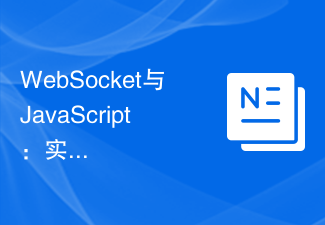
WebSocket et JavaScript : technologies clés pour réaliser des systèmes de surveillance en temps réel Introduction : Avec le développement rapide de la technologie Internet, les systèmes de surveillance en temps réel ont été largement utilisés dans divers domaines. L'une des technologies clés pour réaliser une surveillance en temps réel est la combinaison de WebSocket et de JavaScript. Cet article présentera l'application de WebSocket et JavaScript dans les systèmes de surveillance en temps réel, donnera des exemples de code et expliquera leurs principes de mise en œuvre en détail. 1. Technologie WebSocket
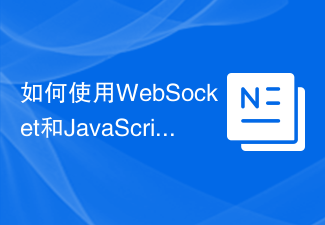
Comment utiliser WebSocket et JavaScript pour mettre en œuvre un système de réservation en ligne. À l'ère numérique d'aujourd'hui, de plus en plus d'entreprises et de services doivent fournir des fonctions de réservation en ligne. Il est crucial de mettre en place un système de réservation en ligne efficace et en temps réel. Cet article explique comment utiliser WebSocket et JavaScript pour implémenter un système de réservation en ligne et fournit des exemples de code spécifiques. 1. Qu'est-ce que WebSocket ? WebSocket est une méthode full-duplex sur une seule connexion TCP.
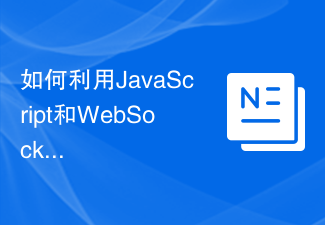
Introduction à l'utilisation de JavaScript et de WebSocket pour mettre en œuvre un système de commande en ligne en temps réel : avec la popularité d'Internet et les progrès de la technologie, de plus en plus de restaurants ont commencé à proposer des services de commande en ligne. Afin de mettre en œuvre un système de commande en ligne en temps réel, nous pouvons utiliser les technologies JavaScript et WebSocket. WebSocket est un protocole de communication full-duplex basé sur le protocole TCP, qui peut réaliser une communication bidirectionnelle en temps réel entre le client et le serveur. Dans le système de commande en ligne en temps réel, lorsque l'utilisateur sélectionne des plats et passe une commande
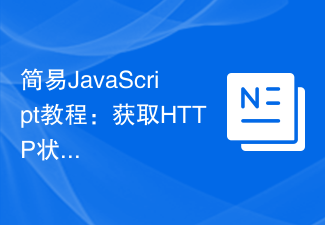
Tutoriel JavaScript : Comment obtenir le code d'état HTTP, des exemples de code spécifiques sont requis Préface : Dans le développement Web, l'interaction des données avec le serveur est souvent impliquée. Lors de la communication avec le serveur, nous devons souvent obtenir le code d'état HTTP renvoyé pour déterminer si l'opération a réussi et effectuer le traitement correspondant en fonction de différents codes d'état. Cet article vous apprendra comment utiliser JavaScript pour obtenir des codes d'état HTTP et fournira quelques exemples de codes pratiques. Utilisation de XMLHttpRequest
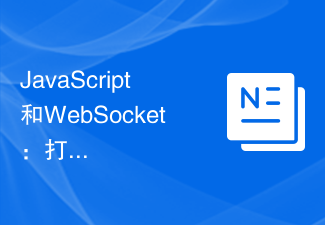
JavaScript et WebSocket : Construire un système efficace de prévisions météorologiques en temps réel Introduction : Aujourd'hui, la précision des prévisions météorologiques revêt une grande importance pour la vie quotidienne et la prise de décision. À mesure que la technologie évolue, nous pouvons fournir des prévisions météorologiques plus précises et plus fiables en obtenant des données météorologiques en temps réel. Dans cet article, nous apprendrons comment utiliser la technologie JavaScript et WebSocket pour créer un système efficace de prévisions météorologiques en temps réel. Cet article démontrera le processus de mise en œuvre à travers des exemples de code spécifiques. Nous
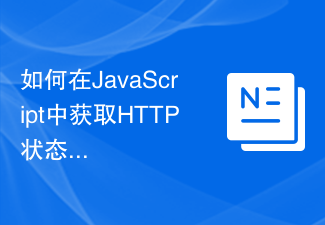
Introduction à la méthode d'obtention du code d'état HTTP en JavaScript : Dans le développement front-end, nous devons souvent gérer l'interaction avec l'interface back-end, et le code d'état HTTP en est une partie très importante. Comprendre et obtenir les codes d'état HTTP nous aide à mieux gérer les données renvoyées par l'interface. Cet article explique comment utiliser JavaScript pour obtenir des codes d'état HTTP et fournit des exemples de code spécifiques. 1. Qu'est-ce que le code d'état HTTP ? Le code d'état HTTP signifie que lorsque le navigateur lance une requête au serveur, le service

Utilisation : En JavaScript, la méthode insertBefore() est utilisée pour insérer un nouveau nœud dans l'arborescence DOM. Cette méthode nécessite deux paramètres : le nouveau nœud à insérer et le nœud de référence (c'est-à-dire le nœud où le nouveau nœud sera inséré).
