PHP中将数组转成XML格式的实现代码_php技巧
May 17, 2016 am 09:16 AM下面是网上的
class ArrayToXML
{
/**
* The main function for converting to an XML document.
* Pass in a multi dimensional array and this recrusively loops through and builds up an XML document.
*
* @param array $data
* @param string $rootNodeName - what you want the root node to be - defaultsto data.
* @param SimpleXMLElement $xml - should only be used recursively
* @return string XML
*/
public static function toXml($data, $rootNodeName = 'data', $xml=null)
{
// turn off compatibility mode as simple xml throws a wobbly if you don't.
if (ini_get('zend.ze1_compatibility_mode') == 1)
{
ini_set ('zend.ze1_compatibility_mode', 0);
}
if ($xml == null)
{
$xml = simplexml_load_string("");
}
// loop through the data passed in.
foreach($data as $key => $value)
{
// no numeric keys in our xml please!
if (is_numeric($key))
{
// make string key...
$key = "unknownNode_". (string) $key;
}
// replace anything not alpha numeric
$key = preg_replace('/[^a-z]/i', '', $key);
// if there is another array found recrusively call this function
if (is_array($value))
{
$node = $xml->addChild($key);
// recrusive call.
ArrayToXML::toXml($value, $rootNodeName, $node);
}
else
{
// add single node.
$value = htmlentities($value);
$xml->addChild($key,$value);
}
}
// pass back as string. or simple xml object if you want!
return $xml->asXML();
}
}
下面是我编辑过的代码
function arrtoxml($arr,$dom=0,$item=0){
if (!$dom){
$dom = new DOMDocument("1.0");
}
if(!$item){
$item = $dom->createElement("root");
$dom->appendChild($item);
}
foreach ($arr as $key=>$val){
$itemx = $dom->createElement(is_string($key)?$key:"item");
$item->appendChild($itemx);
if (!is_array($val)){
$text = $dom->createTextNode($val);
$itemx->appendChild($text);
}else {
arrtoxml($val,$dom,$itemx);
}
}
return $dom->saveXML();
}
数组转换成XML格式
$elementLevel = 0 ;
function array_Xml($array, $keys = '')
{
global $elementLevel;
if(!is_array($array))
{
if($keys == ''){
return $array;
}else{
return "\n" . $array . "$keys>";
}
}else{
foreach ($array as $key => $value)
{
$haveTag = true;
if (is_numeric($key))
{
$key = $keys;
$haveTag = false;
}
/**
* The first element
*/
if($elementLevel == 0 )
{
$startElement = "";
$endElement = "$key>";
}
$text .= $startElement."\n";
/**
* Other elements
*/
if(!$haveTag)
{
$elementLevel++;
$text .= "" . array_Xml($value, $key). "$key>\n";
}else{
$elementLevel++;
$text .= array_Xml($value, $key);
}
$text .= $endElement."\n";
}
}
return $text;
}
?>
函数描述及例子
$array = array(
"employees" => array(
"employee" => array(
array(
"name" => "name one",
"position" => "position one"
),
array(
"name" => "name two",
"position" => "position two"
),
array(
"name" => "name three",
"position" => "position three"
)
)
)
);
echo array_Xml($array);
?>

Article chaud

Outils chauds Tags

Article chaud

Tags d'article chaud

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)

Sujets chauds
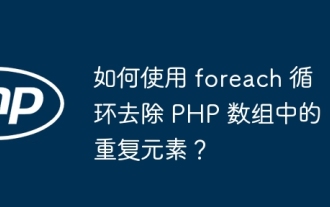
Comment supprimer les éléments en double du tableau PHP à l'aide de la boucle foreach ?

Inversion des valeurs clés du tableau PHP : analyse comparative des performances de différentes méthodes
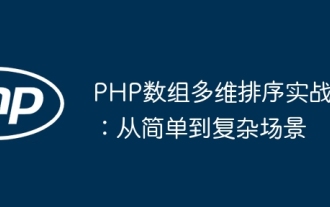
Pratique du tri multidimensionnel des tableaux PHP : des scénarios simples aux scénarios complexes
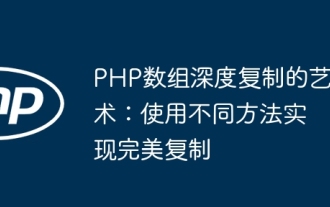
L'art de PHP Array Deep Copy : utiliser différentes méthodes pour obtenir une copie parfaite
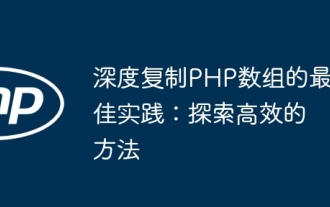
Meilleures pratiques pour la copie approfondie des tableaux PHP : découvrez des méthodes efficaces
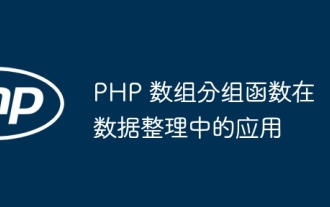
Application de la fonction de regroupement de tableaux PHP dans le tri des données
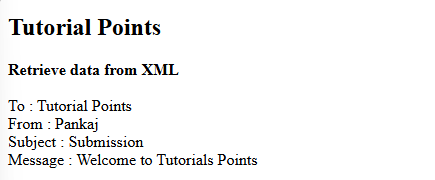
Comment analysez-vous et traitez-vous HTML / XML dans PHP?
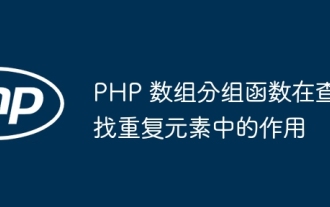
Le rôle de la fonction de regroupement de tableaux PHP dans la recherche d'éléments en double
