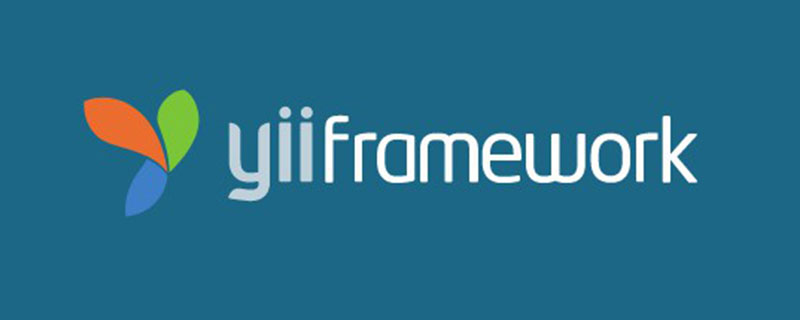
1. Requête simple :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | one(): 根据查询结果返回查询的第一条记录。
all(): 根据查询结果返回所有记录。
count (): 返回记录的数量。
sum(): 返回指定列的总数。
average(): 返回指定列的平均值。
min(): 返回指定列的最小值。
max(): 返回指定列的最大值。
scalar(): 返回查询结果的第一行中的第一列的值。
column(): 返回查询结果中的第一列的值。
exists(): 返回一个值,该值指示查询结果是否有数据。
where(): 添加查询条件
with(): 该查询应执行的关系列表。
indexBy(): 根据索引的列的名称查询结果。
asArray(): 以数组的形式返回每条记录。
|
Copier après la connexion
Exemple d'application :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | Customer::find()->one(); 此方法返回一条数据;
Customer::find()->all(); 此方法返回所有数据;
Customer::find()-> count (); 此方法返回记录的数量;
Customer::find()->average(); 此方法返回指定列的平均值;
Customer::find()->min(); 此方法返回指定列的最小值 ;
Customer::find()->max(); 此方法返回指定列的最大值 ;
Customer::find()->scalar(); 此方法返回值的第一行第一列的查询结果;
Customer::find()->column(); 此方法返回查询结果中的第一列的值;
Customer::find()->exists(); 此方法返回一个值指示是否包含查询结果的数据行;
Customer::find()->asArray()->one(); 以数组形式返回一条数据;
Customer::find()->asArray()->all(); 以数组形式返回所有数据;
Customer::find()->where( $condition )->asArray()->one(); 根据条件以数组形式返回一条数据;
Customer::find()->where( $condition )->asArray()->all(); 根据条件以数组形式返回所有数据;
Customer::find()->where( $condition )->asArray()->orderBy('id DESC')->all(); 根据条件以数组形式返回所有数据,并根据ID倒序;
|
Copier après la connexion
2. >
1 2 | ActiveRecord::hasOne():返回对应关系的单条记录
ActiveRecord::hasMany():返回对应关系的多条记录
|
Copier après la connexion
Exemple d'application :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
Class CustomerModel extends yiidbActiveRecord
{
...
public function getOrders()
{
return $this ->hasMany(OrdersModel::className(), ['id'=>'order_id']);
}
public function getCountry()
{
return $this ->hasOne(CountrysModel::className(), ['id'=>'Country_id']);
}
....
}
CustomerModel::find()->with('orders', 'country')->all();
CustomerModel::find()->with('orders.address')->all();
CustomerModel::find()->with([
'orders' => function ( $query ) {
$query ->andWhere('status = 1');
},
'country',
])->all();
|
Copier après la connexion
Remarque : les commandes avec correspondent à getOrders
FAQ : ajoutée - lors de l'interrogation >select ();Comme suit, order_id doit être ajouté, c'est-à-dire que le champ associé (par exemple : order_id) doit être dans le select, sinon une erreur sera signalée : index non défini order_id1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | CustomerModel::find()->select('order_id')->with('orders', 'country')->all();
findOne()和findAll():
$customer = Customer::findOne(10);
$customer = Customer::find()->where(['id' => 10])->one();
$customer = Customer::findOne(['age' => 30, 'status' => 1]);
$customer = Customer::find()->where(['age' => 30, 'status' => 1])->one();
$customers = Customer::findAll(10);
$customers = Customer::find()->where(['id' => 10])->all();
$customers = Customer::findAll([10, 11, 12]);
$customers = Customer::find()->where(['id' => [10, 11, 12]])->all();
$customers = Customer::findAll(['age' => 30, 'status' => 1]);
$customers = Customer::find()->where(['age' => 30, 'status' => 1])->all();
|
Copier après la connexion
where( )Condition :
1 2 3 4 5 6 7 8 9 10 11 12 | $customers = Customer::find()->where( $cond )->all();
$cond 写法举例:
$cond = ['type' => 1, 'status' => 2]
$cond = ['id' => [1, 2, 3], 'status' => 2]
$cond = ['status' => null]
|
Copier après la connexion
[[et]] : Combinez différentes conditions ensemble, exemple d'utilisation :
1 2 3 4 5 | $cond = [' and ', 'id=1', 'id=2']
$cond = [' and ', 'type=1', [' or ', 'id=1', 'id=2']]
|
Copier après la connexion
[[ou ] ]:
1 2 | $cond = [' or ', ['type' => [7, 8, 9]], ['id' => [1, 2, 3]]
|
Copier après la connexion
[[not]]:
1 2 | $cond = ['not', ['attribute' => null]]
|
Copier après la connexion
[[entre]] : pas entre a le même usage que
1 2 | $cond = ['between', 'id', 1, 10]
|
Copier après la connexion
[[in]] : pas dans l'usage comme
1 2 3 4 5 6 7 8 | $cond = ['in', 'id', [1, 2, 3]]
$cond = ['in', ['id', 'name'], [['id' => 1, 'name' => 'foo'], ['id' => 2, 'name' => 'bar']]]
$cond = ['in', 'user_id', ( new Query())->select('id')->from('users')->where(['active' => 1])]
|
Copier après la connexion
[[like]] :
1 2 3 4 5 6 7 8 | $cond = ['like', 'name', 'tester']
$cond = ['like', 'name', ['test', 'sample']]
$cond = ['like', 'name', '%tester', false]
|
Copier après la connexion
[ [existe]] : n'existe pas, l'utilisation est similaire à
1 2 3 4 5 6 7 8 9 | $cond = ['exists', ( new Query())->select('id')->from('users')->where(['active' => 1])]
此外,您可以指定任意运算符如下
$cond = ['>=', 'id', 10]
$cond = ['!=', 'id', 10]
|
Copier après la connexion
Requêtes courantes :
1 2 3 4 5 6 7 | User::find()->select('*')->where(['>=', 'admin_id', 10])->offset(0)->limit(10)->all()
$subQuery = ( new Query())->select(' COUNT (*)')->from('user');
$query = ( new Query())->select(['id', ' count ' => $subQuery ])->from('post');
User::find()->select('user_id')->distinct();
|
Copier après la connexion
Mise à jour :
1 2 3 4 5 6 7 8 9 10 11 | $model ->update( $runValidation , $attributeNames );
Customer::updateAll(['status' => 1], 'status = 2');
Customer::updateAll(['status' => 1], ['status'=> '2','uid'=>'1']);
|
Copier après la connexion
Supprimer :
1 2 3 | $model = Customer::findOne( $id );
$model -> delete ();
$model ->deleteAll(['id'=>1]);
|
Copier après la connexion
Insérer par lots :
1 2 3 4 5 | Yii:: $app ->db->createCommand()->batchInsert(UserModel::tableName(), ['user_id','username'], [
['1','test1'],
['2','test2'],
['3','test3'],
])->execute();
|
Copier après la connexion
Voir l'exécution sql
1 2 3 | $query = UserModel::find()->where(['status'=>1]);
echo $query ->createCommand()->getRawSql();
|
Copier après la connexion
Tutoriel recommandé :
Tutoriel YII
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!