


Description détaillée du modèle d'adaptateur PHP (avec exemples de code)
Cet article vous apporte des connaissances pertinentes sur PHP. Il parle principalement du mode adaptateur PHP et des exemples de code. Les amis intéressés peuvent jeter un œil ci-dessous.
Explication du modèle d'adaptateur PHP et exemples de code
Adapter est un modèle de conception structurelle qui permet à des objets incompatibles de coopérer les uns avec les autres.
L'adaptateur peut agir comme un wrapper entre deux objets. Il recevra un appel à un objet et le convertira en un format et une interface reconnus par l'autre objet.
Complexité : ******
Popularité : ******
Exemple d'utilisation : Le modèle Adapter est très courant dans le code PHP. Les systèmes basés sur du code existant utilisent souvent ce modèle. Dans ce cas, les adaptateurs permettent au code existant de fonctionner avec les classes modernes.
Méthode d'identification : Les adaptateurs peuvent être identifiés via des constructeurs qui prennent des instances de différents types abstraits ou d'interface comme paramètres. Lorsqu'une méthode de l'adaptateur est appelée, elle convertit les paramètres dans le format approprié, puis dirige l'appel vers une ou plusieurs méthodes dans son objet d'encapsulation.
Exemples du monde réel
Les adaptateurs vous permettent d'utiliser des classes de systèmes tiers ou existants, même si elles sont incompatibles avec votre code. Par exemple, vous pouvez créer une série de wrappers spéciaux pour adapter les appels effectués par l'application à l'interface et au format requis par la classe tierce, sans avoir à réécrire l'interface de notification de l'application pour prendre en charge chaque service tiers (tel que DingTalk, WeChat, SMS ou tout autre service).
index.php : Exemple réel
<?php namespace RefactoringGuru\Adapter\RealWorld; /** * The Target interface represents the interface that your application's classes * already follow. */ interface Notification { public function send(string $title, string $message); } /** * Here's an example of the existing class that follows the Target interface. * * The truth is that many real apps may not have this interface clearly defined. * If you're in that boat, your best bet would be to extend the Adapter from one * of your application's existing classes. If that's awkward (for instance, * SlackNotification doesn't feel like a subclass of EmailNotification), then * extracting an interface should be your first step. */ class EmailNotification implements Notification { private $adminEmail; public function __construct(string $adminEmail) { $this->adminEmail = $adminEmail; } public function send(string $title, string $message): void { mail($this->adminEmail, $title, $message); echo "Sent email with title '$title' to '{$this->adminEmail}' that says '$message'."; } } /** * The Adaptee is some useful class, incompatible with the Target interface. You * can't just go in and change the code of the class to follow the Target * interface, since the code might be provided by a 3rd-party library. */ class SlackApi { private $login; private $apiKey; public function __construct(string $login, string $apiKey) { $this->login = $login; $this->apiKey = $apiKey; } public function logIn(): void { // Send authentication request to Slack web service. echo "Logged in to a slack account '{$this->login}'.\n"; } public function sendMessage(string $chatId, string $message): void { // Send message post request to Slack web service. echo "Posted following message into the '$chatId' chat: '$message'.\n"; } } /** * The Adapter is a class that links the Target interface and the Adaptee class. * In this case, it allows the application to send notifications using Slack * API. */ class SlackNotification implements Notification { private $slack; private $chatId; public function __construct(SlackApi $slack, string $chatId) { $this->slack = $slack; $this->chatId = $chatId; } /** * An Adapter is not only capable of adapting interfaces, but it can also * convert incoming data to the format required by the Adaptee. */ public function send(string $title, string $message): void { $slackMessage = "#" . $title . "# " . strip_tags($message); $this->slack->logIn(); $this->slack->sendMessage($this->chatId, $slackMessage); } } /** * The client code can work with any class that follows the Target interface. */ function clientCode(Notification $notification) { // ... echo $notification->send("Website is down!", "<strong style='color:red;font-size: 50px;'>Alert!</strong> " . "Our website is not responding. Call admins and bring it up!"); // ... } echo "Client code is designed correctly and works with email notifications:\n"; $notification = new EmailNotification("developers@example.com"); clientCode($notification); echo "\n\n"; echo "The same client code can work with other classes via adapter:\n"; $slackApi = new SlackApi("example.com", "XXXXXXXX"); $notification = new SlackNotification($slackApi, "Example.com Developers"); clientCode($notification);
Output.txt : Résultat d'exécution
Client code is designed correctly and works with email notifications: Sent email with title 'Website is down!' to 'developers@example.com' that says '<strong style='color:red;font-size: 50px;'>Alert!</strong> Our website is not responding. Call admins and bring it up!'. The same client code can work with other classes via adapter: Logged in to a slack account 'example.com'. Posted following message into the 'Example.com Developers' chat: '#Website is down!# Alert! Our website is not responding. Call admins and bring it up!'.
Exemple conceptuel
Cet exemple illustre la structure du modèle de conception Adapter et se concentre sur la réponse aux questions suivantes :
- De quels cours s'agit-il ?
- Quels rôles jouent ces classes ?
- Comment les différents éléments du motif seront-ils liés les uns aux autres ?
Après avoir compris la structure de ce modèle, vous pouvez plus facilement comprendre les cas d'application PHP réels suivants.
index.php: Exemple de concept
<?php namespace RefactoringGuru\Adapter\Conceptual; /** * The Target defines the domain-specific interface used by the client code. */ class Target { public function request(): string { return "Target: The default target's behavior."; } } /** * The Adaptee contains some useful behavior, but its interface is incompatible * with the existing client code. The Adaptee needs some adaptation before the * client code can use it. */ class Adaptee { public function specificRequest(): string { return ".eetpadA eht fo roivaheb laicepS"; } } /** * The Adapter makes the Adaptee's interface compatible with the Target's * interface. */ class Adapter extends Target { private $adaptee; public function __construct(Adaptee $adaptee) { $this->adaptee = $adaptee; } public function request(): string { return "Adapter: (TRANSLATED) " . strrev($this->adaptee->specificRequest()); } } /** * The client code supports all classes that follow the Target interface. */ function clientCode(Target $target) { echo $target->request(); } echo "Client: I can work just fine with the Target objects:\n"; $target = new Target(); clientCode($target); echo "\n\n"; $adaptee = new Adaptee(); echo "Client: The Adaptee class has a weird interface. See, I don't understand it:\n"; echo "Adaptee: " . $adaptee->specificRequest(); echo "\n\n"; echo "Client: But I can work with it via the Adapter:\n"; $adapter = new Adapter($adaptee); clientCode($adapter);
Output.txt: Résultats d'exécution
Client: I can work just fine with the Target objects: Target: The default target's behavior. Client: The Adaptee class has a weird interface. See, I don't understand it: Adaptee: .eetpadA eht fo roivaheb laicepS Client: But I can work with it via the Adapter: Adapter: (TRANSLATED) Special behavior of the Adaptee.
Apprentissage recommandé : "Tutoriel vidéo PHP"
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!

Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

AI Hentai Generator
Générez AI Hentai gratuitement.

Article chaud

Outils chauds

Bloc-notes++7.3.1
Éditeur de code facile à utiliser et gratuit

SublimeText3 version chinoise
Version chinoise, très simple à utiliser

Envoyer Studio 13.0.1
Puissant environnement de développement intégré PHP

Dreamweaver CS6
Outils de développement Web visuel

SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)
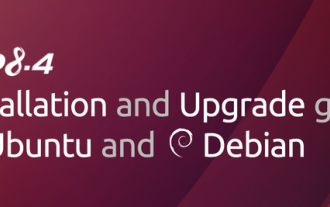
PHP 8.4 apporte plusieurs nouvelles fonctionnalités, améliorations de sécurité et de performances avec une bonne quantité de dépréciations et de suppressions de fonctionnalités. Ce guide explique comment installer PHP 8.4 ou mettre à niveau vers PHP 8.4 sur Ubuntu, Debian ou leurs dérivés. Bien qu'il soit possible de compiler PHP à partir des sources, son installation à partir d'un référentiel APT comme expliqué ci-dessous est souvent plus rapide et plus sécurisée car ces référentiels fourniront les dernières corrections de bogues et mises à jour de sécurité à l'avenir.
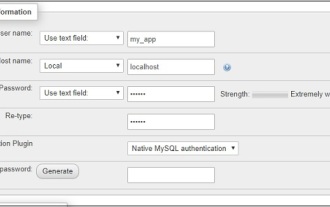
Travailler avec la base de données dans CakePHP est très simple. Nous comprendrons les opérations CRUD (Créer, Lire, Mettre à jour, Supprimer) dans ce chapitre.
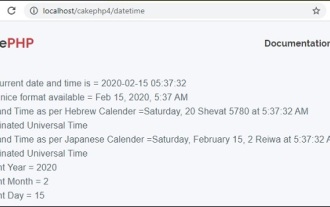
Pour travailler avec la date et l'heure dans cakephp4, nous allons utiliser la classe FrozenTime disponible.
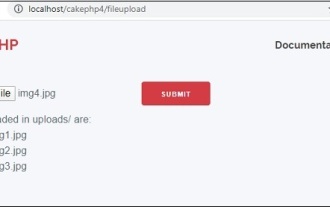
Pour travailler sur le téléchargement de fichiers, nous allons utiliser l'assistant de formulaire. Voici un exemple de téléchargement de fichiers.
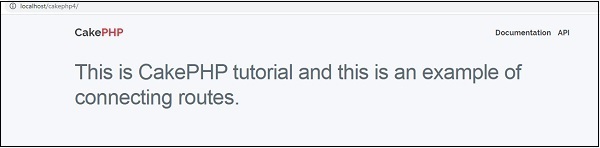
Dans ce chapitre, nous allons apprendre les sujets suivants liés au routage ?
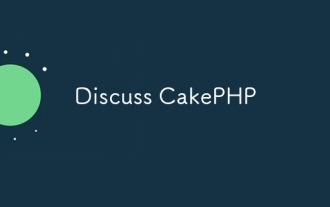
CakePHP est un framework open source pour PHP. Il vise à faciliter grandement le développement, le déploiement et la maintenance d'applications. CakePHP est basé sur une architecture de type MVC à la fois puissante et facile à appréhender. Modèles, vues et contrôleurs gu
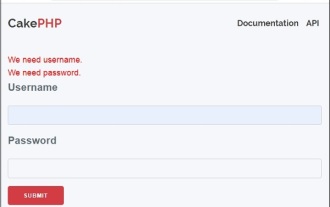
Le validateur peut être créé en ajoutant les deux lignes suivantes dans le contrôleur.
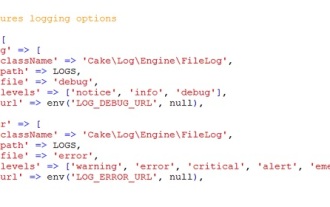
Se connecter à CakePHP est une tâche très simple. Il vous suffit d'utiliser une seule fonction. Vous pouvez enregistrer les erreurs, les exceptions, les activités des utilisateurs, les actions entreprises par les utilisateurs, pour tout processus en arrière-plan comme cronjob. La journalisation des données dans CakePHP est facile. La fonction log() est fournie
