Detailed analysis of the use of HTML5 FormData object
XMLHttpRequest Level 2 adds a new interface - FormData.
Using the FormData object,
we can use some key-value pairs to simulate a series of form controls through JavaScript. We can also use the send() method of XMLHttpRequest to submit the form asynchronously . Compared with ordinary Ajax, the biggest advantage of using FormData is that we can upload binary files asynchronously.
Create a FormData object
You can first create an empty FormData
object, and then use the append()
method to add fields to the object , as follows:
var oMyForm = new FormData(); oMyForm.append("username", "Groucho"); oMyForm.append("accountnum", 123456); // 数字123456被立即转换成字符串"123456" // fileInputElement中已经包含了用户所选择的文件 oMyForm.append("userfile", fileInputElement.files[0]); var oFileBody = "<a id="a"><b id="b">hey!</b></a>"; // Blob对象包含的文件内容 var oBlob = new Blob([oFileBody], { type: "text/xml"}); oMyForm.append("webmasterfile", oBlob); var oReq = new XMLHttpRequest(); oReq.open("POST", "http://foo.com/submitform.php"); oReq.send(oMyForm);
Note: The values of the fields "userfile" and "webmasterfile" both contain a file. Numbers assigned to the field "accountnum" through the FormData.append()
method are automatically converted into characters (the value of the field can be a Blob
object, File
object Or string, the rest of the other types of values will be automatically converted into strings).
In this example, we create a FormData object named oMyForm, which contains field names named "username", "accountnum", "userfile" and "webmasterfile", and then use The send()
method of XMLHttpRequest
sends these data. The value of the "webmasterfile" field is not a string, but a Blob
object.
Use HTML form to initialize a FormData object
You can use an existing form element to initialize the FormData object,
just need to change this form
Elements are passed in as parameters FormData
Constructor Just like:
var newFormData = new FormData(someFormElement);
For example:
var formElement = document.getElementById("myFormElement"); var oReq = new XMLHttpRequest(); oReq.open("POST", "submitform.php"); oReq.send(new FormData(formElement));
You can also Based on the form data, continue to add new key-value pairs, as follows:
var formElement = document.getElementById("myFormElement"); formData = new FormData(formElement); formData.append("serialnumber", serialNumber++); oReq.send(formData);
You can add some fixed fields that you do not want users to edit in this way, and then send them.
Use the FormData object to send files
You can also use FormData
to send binary files. First, there must be a form element in HTML that contains a file input box:
<form enctype="multipart/form-data" method="post" name="fileinfo"> <label>Your email address:</label> <input type="email" autocomplete="on" autofocus name="userid" placeholder="email" required size="32" maxlength="64" /><br /> <label>Custom file label:</label> <input type="text" name="filelabel" size="12" maxlength="32" /><br /> <label>File to stash:</label> <input type="file" name="file" required /> </form> <p id="output"></p> <a href="javascript:sendForm()">Stash the file!</a>
Then you can use the following code to asynchronously upload the file selected by the user:
function sendForm() { var oOutput = document.getElementById("output"); var oData = new FormData(document.forms.namedItem("fileinfo")); oData.append("CustomField", "This is some extra data"); var oReq = new XMLHttpRequest(); oReq.open("POST", "stash.php", true); oReq.onload = function(oEvent) { if (oReq.status == 200) { oOutput.innerHTML = "Uploaded!"; } else { oOutput.innerHTML = "Error " + oReq.status + " occurred uploading your file.<br \/>"; } }; oReq.send(oData); }
You can also add a File directly to the
FormData object without using an HTML form
Object or a Blob
Object:
data.append("myfile", myBlob);
If a field value in the FormData object is a Blob
object, when sending an HTTP request, it represents The Blob
object contains the file name of the file . The value of the "Content-Disposition" request header is different in different browsers. Firefox uses The fixed string "blob" is used, while Chrome uses a random string.
You can also use jQuery to send FormData, but you must set the relevant options correctly:
var fd = new FormData(document.getElementById("fileinfo")); fd.append("CustomField", "This is some extra data"); $.ajax({ url: "stash.php", type: "POST", data: fd, processData: false, // 告诉jQuery不要去处理发送的数据 contentType: false // 告诉jQuery不要去设置Content-Type请求头 });
Browser compatibility
Desktop:
Feature | Chrome | Firefox (Gecko) | Internet Explorer | Opera | Safari |
---|---|---|---|---|---|
Basic support | 7+ | 4.0 (2.0) | 10+ | 12+ | 5+ |
Support filename parameter |
(Yes) | 22.0 (22.0 ) | ? | ? | ? |
Android | Chrome for AndroidFirefox Mobile (Gecko) | IE Mobile | Opera Mobile | Safari Mobile | ||
---|---|---|---|---|---|---|
3.0 | ? | 4.0 (2.0 ) | ? | 12+ | ?||
filename parameter
| ?? | 22.0 (22.0) | ? | ? | ? |
The above is the detailed content of Detailed analysis of the use of HTML5 FormData object. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










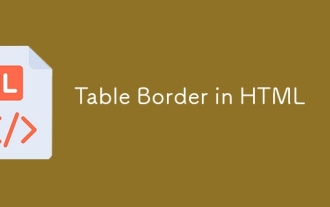
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
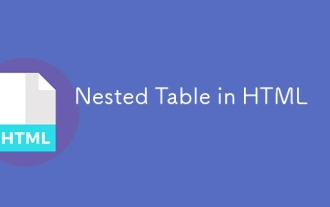
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
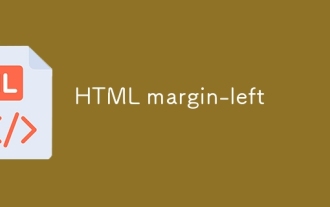
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
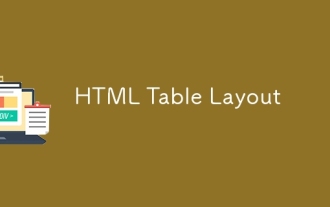
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
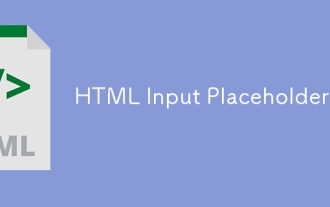
Guide to HTML Input Placeholder. Here we discuss the Examples of HTML Input Placeholder along with the codes and outputs.
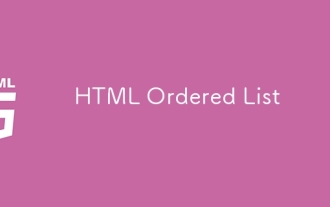
Guide to the HTML Ordered List. Here we also discuss introduction of HTML Ordered list and types along with their example respectively
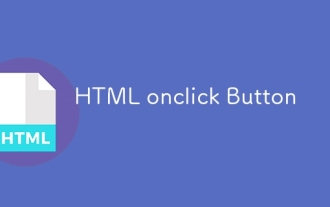
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.
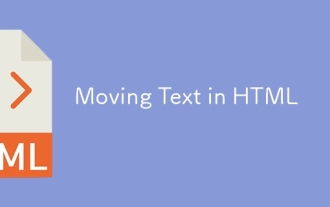
Guide to Moving Text in HTML. Here we discuss an introduction, how marquee tag work with syntax and examples to implement.
