Golang を使用して、異なるタイムゾーンのコルーチンの時間を同期するにはどうすればよいですか?
Go コルーチンで異なるタイム ゾーンを同期するメソッド: time.LoadLocation() 関数を使用して、タイム ゾーン データベースからタイム ゾーン情報をロードし、タイム ゾーンを表す *time.Location インスタンスを返します。コルーチンでコンテキストを使用して、*time.Location コンテキストを各コルーチンに渡し、同じ時間情報にアクセスできるようにします。実際のアプリケーションでは、注文のタイムゾーンに基づいてタイムスタンプや注文処理のロジックを印刷できます。
Goroutine で異なるタイムゾーンを同期する方法
コルーチンは、Go の同時プログラミングによく使用される軽量のスレッドです。異なるタイムゾーンのデータを扱う場合、時刻を手動で同期するのは難しい場合があります。このチュートリアルでは、Go 標準ライブラリを使用して、さまざまなタイムゾーンを処理し、時刻の同期を保つ方法を説明します。
time.LoadLocation()
time.LoadLocation()
time.LoadLocation()
函数用于从时区数据库中加载时区信息。通过提供时区的名称,可以获取一个代表该时区的 *time.Location
实例。
import ( "fmt" "time" ) func main() { // 加载东京时区 tokyo, err := time.LoadLocation("Asia/Tokyo") if err != nil { log.Fatal(err) } // 加载纽约时区 newYork, err := time.LoadLocation("America/New_York") if err != nil { log.Fatal(err) } // 创建一个 Tokyo 时间的时刻 tokyoTime := time.Now().In(tokyo) fmt.Println("东京时间:", tokyoTime.Format("2006-01-02 15:04:05")) // 创建一个纽约时间的一个时刻 newYorkTime := time.Now().In(newYork) fmt.Println("纽约时间:", newYorkTime.Format("2006-01-02 15:04:05")) }
在协程中使用上下文
当使用协程处理数据时,可以在将 *time.Location
time.LoadLocation()
関数を使用して、タイム ゾーン データベースからタイム ゾーン情報を読み込みます。タイム ゾーンの名前を指定すると、そのタイム ゾーンを表す *time.Location
のインスタンスを取得できます。 package main
import (
"context"
"fmt"
"time"
)
func main() {
ctx := context.Background()
// 加载东京时区
tokyo, err := time.LoadLocation("Asia/Tokyo")
if err != nil {
log.Fatal(err)
}
// 使用 Tokyo 时区创建上下文
ctx = context.WithValue(ctx, "timeZone", tokyo)
go func() {
// 从上下文中获取时区
timeZone := ctx.Value("timeZone").(*time.Location)
// 创建东京时间的一个时刻
tokyoTime := time.Now().In(timeZone)
fmt.Println("东京时间:", tokyoTime.Format("2006-01-02 15:04:05"))
}()
// 加载纽约时区
newYork, err := time.LoadLocation("America/New_York")
if err != nil {
log.Fatal(err)
}
// 使用纽约时区创建上下文
ctx = context.WithValue(ctx, "timeZone", newYork)
go func() {
// 从上下文中获取时区
timeZone := ctx.Value("timeZone").(*time.Location)
// 创建纽约时间的一个时刻
newYorkTime := time.Now().In(timeZone)
fmt.Println("纽约时间:", newYorkTime.Format("2006-01-02 15:04:05"))
}()
time.Sleep(time.Second)
}
*time.Location
コンテキストを各コルーチンに渡すことで、すべてのコルーチンが同じ時間情報にアクセスできるようになります。 🎜package main import ( "context" "fmt" "time" ) type Order struct { Timestamp time.Time Location string } func main() { ctx := context.Background() // 加载东京时区的订单 tokyoOrder := Order{ Timestamp: time.Now().In(time.LoadLocation("Asia/Tokyo")), Location: "Tokyo", } // 加载纽约时区的订单 newYorkOrder := Order{ Timestamp: time.Now().In(time.LoadLocation("America/New_York")), Location: "New York", } // 使用东京时区创建上下文 ctxTokyo := context.WithValue(ctx, "order", tokyoOrder) // 使用纽约时区创建上下文 ctxNewYork := context.WithValue(ctx, "order", newYorkOrder) go processOrder(ctxTokyo) go processOrder(ctxNewYork) time.Sleep(time.Second) } func processOrder(ctx context.Context) { // 从上下文中获取订单 order := ctx.Value("order").(Order) // 根据订单的时区打印时间戳 fmt.Printf("订单来自 %s,时间戳为:%s\n", order.Location, order.Timestamp.Format("2006-01-02 15:04:05")) }
以上がGolang を使用して、異なるタイムゾーンのコルーチンの時間を同期するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










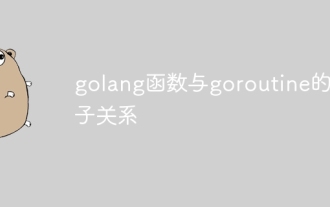
Go では関数とゴルーチンの間に親子関係があり、親ゴルーチンは子ゴルーチンを作成し、子ゴルーチンは親ゴルーチンの変数にアクセスできますが、その逆はできません。 go キーワードを使用して子ゴルーチンを作成すると、子ゴルーチンは匿名関数または名前付き関数を通じて実行されます。親ゴルーチンは、すべての子ゴルーチンが完了する前にプログラムが終了しないように、sync.WaitGroup を介して子ゴルーチンが完了するのを待つことができます。
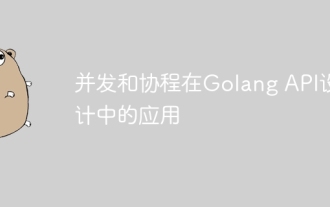
同時実行性とコルーチンは、GoAPI 設計で次の目的で使用されます。 高パフォーマンス処理: 複数のリクエストを同時に処理してパフォーマンスを向上させます。非同期処理: コルーチンを使用してタスク (電子メールの送信など) を非同期に処理し、メインスレッドを解放します。ストリーム処理: コルーチンを使用して、データ ストリーム (データベース読み取りなど) を効率的に処理します。
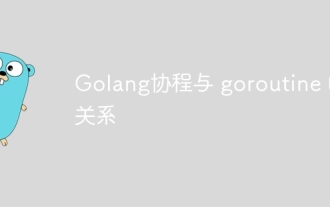
コルーチンはタスクを同時に実行するための抽象的な概念であり、ゴルーチンはコルーチンの概念を実装する Go 言語の軽量スレッド関数です。この 2 つは密接に関連していますが、Goroutine のリソース消費量は少なく、Go スケジューラによって管理されます。 GoroutineはWebリクエストの同時処理やプログラムのパフォーマンス向上など、実戦で広く活用されています。
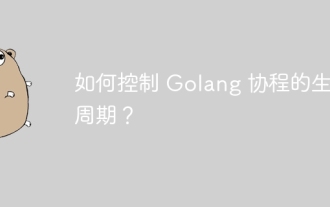
Go コルーチンのライフ サイクルは、次の方法で制御できます。 コルーチンを作成します。 go キーワードを使用して、新しいタスクを開始します。コルーチンを終了する: すべてのコルーチンが完了するまで待ち、sync.WaitGroup を使用します。チャネル終了信号を使用します。コンテキスト context.Context を使用します。
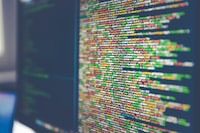
同時プログラミングと非同期プログラミング 同時プログラミングは、同時に実行される複数のタスクを扱います。非同期プログラミングは、タスクがスレッドをブロックしない同時プログラミングの一種です。 asyncio は Python の非同期プログラミング用のライブラリで、プログラムがメイン スレッドをブロックせずに I/O 操作を実行できるようにします。イベント ループ asyncio の中核は、I/O イベントを監視し、対応するタスクをスケジュールするイベント ループです。コルーチンの準備が完了すると、イベント ループは I/O 操作を待つまでそのコルーチンを実行します。その後、コルーチンを一時停止し、他のコルーチンの実行を継続します。コルーチン コルーチンは、実行を一時停止および再開できる関数です。 asyncdef キーワードは、コルーチンの作成に使用されます。コルーチンは await キーワードを使用して、I/O 操作が完了するのを待ちます。 asyncio の次の基本
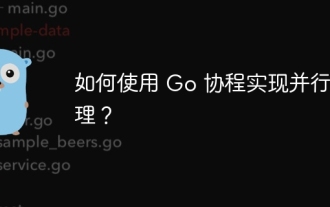
Go コルーチンを使用して並列処理を実装するにはどうすればよいですか?フィボナッチ数列を並列計算するコルーチンを作成します。コルーチンはチャネルを通じてデータを転送し、並列コンピューティングを実現します。メイン コルーチンは、並列計算の結果を受け取って処理します。
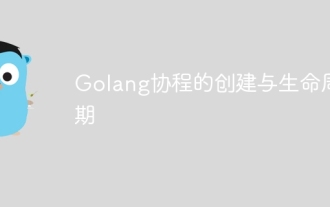
コルーチンは、明示的に切り替えることで同じコール スタック内の実行ユニットを再利用する軽量のスレッドです。そのライフサイクルには、作成、実行、一時停止、回復、完了が含まれます。 go キーワードを使用してコルーチンを作成します。これは、実際の並列計算 (フィボナッチ数の計算など) に使用できます。
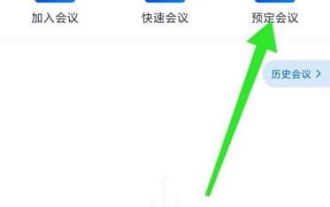
皆さん、テンセントの会議を予約するときにタイムゾーンを選択する方法を知っていますか?今日は、編集者がテンセントの会議を予約するときにタイムゾーンを選択する方法を説明します。興味があれば、編集者と一緒に見に来てください。お役に立てれば幸いです。ステップ 1: Tencent Meeting APP に入り、クリックして会議を予約します (図を参照)。ステップ 2: 定例ミーティングなどのミーティング タイプを選択し、[次へ] をクリックします (図を参照)。ステップ 3: 会議予約ページで、タイムゾーンをクリックします (図を参照)。ステップ 4: タイムゾーンを選択します (図を参照)。ステップ 5: 設定が成功したら、「完了」をクリックします (図を参照)。
