JavaScript、HTML、CSS を使用した Ollama の Llama odel によるチャット アプリケーションの構築
導入
このブログ投稿では、Ollama の Llama 3 モデルと対話する単純なチャット アプリケーションを作成するプロセスを順を追って説明します。フロントエンドには JavaScript、HTML、CSS を使用し、バックエンドには Express を備えた Node.js を使用します。最終的には、ユーザー メッセージを AI モデルに送信し、その応答をリアルタイムで表示する、機能するチャット アプリケーションが完成します。
前提条件
始める前に、次のものがマシンにインストールされていることを確認してください:
- Node.js
- npm (ノードパッケージマネージャー)
ステップ 1: フロントエンドのセットアップ
HTML
まず、チャット アプリケーションの構造を定義する、index.html という名前の HTML ファイルを作成します。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Chat with Ollama's Llama 3</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div id="chat-container"> <div id="chat-window"> <div id="messages"></div> </div> <input type="text" id="user-input" placeholder="Type your message here..."> <button id="send-button">Send</button> </div> <script src="script.js"></script> </body> </html>
この HTML ファイルには、チャット メッセージのコンテナ、ユーザー メッセージの入力フィールド、および送信ボタンが含まれています。
CSS
次に、チャット アプリケーションのスタイルを設定するために、styles.css という名前の CSS ファイルを作成します。
body { font-family: Arial, sans-serif; display: flex; justify-content: center; align-items: center; height: 100vh; background-color: #f0f0f0; margin: 0; } #chat-container { width: 400px; border: 1px solid #ccc; background-color: #fff; border-radius: 8px; box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1); overflow: hidden; } #chat-window { height: 300px; padding: 10px; overflow-y: auto; border-bottom: 1px solid #ccc; } #messages { display: flex; flex-direction: column; } .message { padding: 8px; margin: 4px 0; border-radius: 4px; } .user-message { align-self: flex-end; background-color: #007bff; color: #fff; } .ai-message { align-self: flex-start; background-color: #e0e0e0; color: #000; } #user-input { width: calc(100% - 60px); padding: 10px; border: none; border-radius: 0; outline: none; } #send-button { width: 60px; padding: 10px; border: none; background-color: #007bff; color: #fff; cursor: pointer; }
この CSS ファイルにより、チャット アプリケーションがクリーンでモダンに見えるようになります。
JavaScript
フロントエンド機能を処理するために、script.js という名前の JavaScript ファイルを作成します。
document.getElementById('send-button').addEventListener('click', sendMessage); document.getElementById('user-input').addEventListener('keypress', function (e) { if (e.key === 'Enter') { sendMessage(); } }); function sendMessage() { const userInput = document.getElementById('user-input'); const messageText = userInput.value.trim(); if (messageText === '') return; displayMessage(messageText, 'user-message'); userInput.value = ''; // Send the message to the local AI and get the response getAIResponse(messageText).then(aiResponse => { displayMessage(aiResponse, 'ai-message'); }).catch(error => { console.error('Error:', error); displayMessage('Sorry, something went wrong.', 'ai-message'); }); } function displayMessage(text, className) { const messageElement = document.createElement('div'); messageElement.textContent = text; messageElement.className = `message ${className}`; document.getElementById('messages').appendChild(messageElement); document.getElementById('messages').scrollTop = document.getElementById('messages').scrollHeight; } async function getAIResponse(userMessage) { // Example AJAX call to a local server interacting with Ollama Llama 3 const response = await fetch('http://localhost:5000/ollama', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ message: userMessage }), }); if (!response.ok) { throw new Error('Network response was not ok'); } const data = await response.json(); return data.response; // Adjust this based on your server's response structure }
この JavaScript ファイルは、送信ボタンと入力フィールドにイベント リスナーを追加し、ユーザー メッセージをバックエンドに送信し、ユーザーと AI の両方の応答を表示します。
ステップ 2: バックエンドのセットアップ
Node.js と Express
Node.js がインストールされていることを確認してください。次に、バックエンド用のserver.jsファイルを作成します。
-
Express のインストール:
npm install express body-parser
ログイン後にコピー -
server.js ファイルを作成します:
const express = require('express'); const bodyParser = require('body-parser'); const app = express(); const port = 5000; app.use(bodyParser.json()); app.post('/ollama', async (req, res) => { const userMessage = req.body.message; // Replace this with actual interaction with Ollama's Llama 3 // This is a placeholder for demonstration purposes const aiResponse = await getLlama3Response(userMessage); res.json({ response: aiResponse }); }); // Placeholder function to simulate AI response async function getLlama3Response(userMessage) { // Replace this with actual API call to Ollama's Llama 3 return `Llama 3 says: ${userMessage}`; } app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); });
ログイン後にコピー -
サーバーを実行します:
node server.js
ログイン後にコピー
このセットアップでは、Node.js サーバーは受信リクエストを処理し、Ollama の Llama 3 モデルと対話して、応答を返します。
結論
これらの手順に従うことで、ユーザー メッセージを Ollama の Llama 3 モデルに送信し、応答を表示するチャット アプリケーションが作成されました。このセットアップは、特定の要件と Llama 3 モデルが提供する機能に基づいて拡張およびカスタマイズできます。
チャット アプリケーションの機能を自由に探索して拡張してください。コーディングを楽しんでください!
以上がJavaScript、HTML、CSS を使用した Ollama の Llama odel によるチャット アプリケーションの構築の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










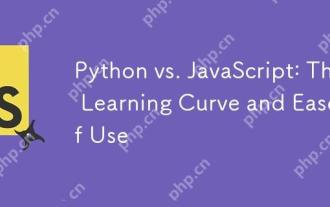
Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。
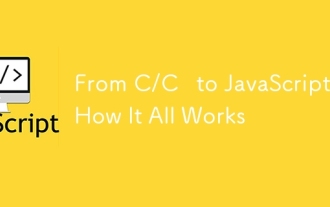
C/CからJavaScriptへのシフトには、動的なタイピング、ゴミ収集、非同期プログラミングへの適応が必要です。 1)C/Cは、手動メモリ管理を必要とする静的に型付けられた言語であり、JavaScriptは動的に型付けされ、ごみ収集が自動的に処理されます。 2)C/Cはマシンコードにコンパイルする必要がありますが、JavaScriptは解釈言語です。 3)JavaScriptは、閉鎖、プロトタイプチェーン、約束などの概念を導入します。これにより、柔軟性と非同期プログラミング機能が向上します。
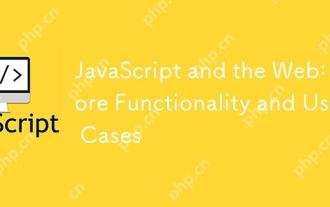
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
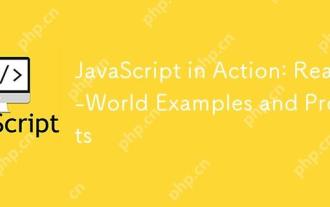
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
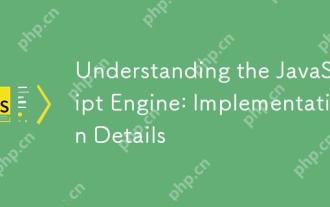
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。
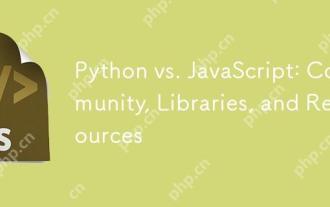
PythonとJavaScriptには、コミュニティ、ライブラリ、リソースの観点から、独自の利点と短所があります。 1)Pythonコミュニティはフレンドリーで初心者に適していますが、フロントエンドの開発リソースはJavaScriptほど豊富ではありません。 2)Pythonはデータサイエンスおよび機械学習ライブラリで強力ですが、JavaScriptはフロントエンド開発ライブラリとフレームワークで優れています。 3)どちらも豊富な学習リソースを持っていますが、Pythonは公式文書から始めるのに適していますが、JavaScriptはMDNWebDocsにより優れています。選択は、プロジェクトのニーズと個人的な関心に基づいている必要があります。
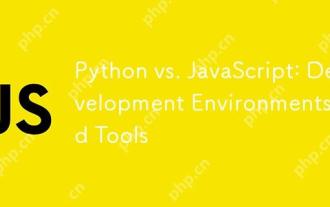
開発環境におけるPythonとJavaScriptの両方の選択が重要です。 1)Pythonの開発環境には、Pycharm、Jupyternotebook、Anacondaが含まれます。これらは、データサイエンスと迅速なプロトタイピングに適しています。 2)JavaScriptの開発環境には、フロントエンドおよびバックエンド開発に適したnode.js、vscode、およびwebpackが含まれます。プロジェクトのニーズに応じて適切なツールを選択すると、開発効率とプロジェクトの成功率が向上する可能性があります。
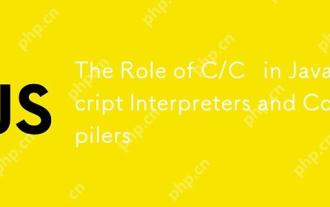
CとCは、主に通訳者とJITコンパイラを実装するために使用されるJavaScriptエンジンで重要な役割を果たします。 1)cは、JavaScriptソースコードを解析し、抽象的な構文ツリーを生成するために使用されます。 2)Cは、Bytecodeの生成と実行を担当します。 3)Cは、JITコンパイラを実装し、実行時にホットスポットコードを最適化およびコンパイルし、JavaScriptの実行効率を大幅に改善します。
