Laravel のデータベースシーダーを使用してデータを簡単に生成する方法
アプリケーションを構築するときは、小規模、中規模、または大規模であっても構いません。アプリケーション内でテスト データを使用できるようにすることは避けられず、重要です。
それでは、2 つの異なるシナリオを使って、簡単なものから高度なものまで始めてみましょう。
私。すべてのアプリケーションまたは大部分のアプリケーションにはユーザーが必要です。場合によっては、ユーザーを 管理者 または通常の ユーザー として分類/タグ付けしたい場合があります。そこで、以下にリストされているテーブル仕様を使用して、100 人のユーザーを含む単純なシーダーを生成してみましょう。
- ID、名、姓、メールアドレス、パスワード
- ユーザー タイプ: 管理者 または通常の ユーザー
それでは、行きましょう。
users テーブルにシードするには、もちろん、Laravel によって作成されたデフォルトのユーザー移行テーブルが必要です。
このデモンストレーションのために、テーブルの移行は次のようになります。
public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('first_name'); $table->string('last_name'); $table->string('email'); $table->string('password'); $table->enum('user_type', ['user','admin'])->default('user'); $table->rememberToken(); $table->timestamps(); }); }
Users テーブルのシーダー クラスを作成します。
php artisan make:seeder UserSeeder
したがって、作成したばかりのシーダーの値を作成するには、すべての Laravel アプリケーションに付属するデフォルトの Faker パッケージを使用する必要があります。
一度に 100 人のユーザーを生成するには、まず for ループを実行し、次のように偽/疑似データを各列に渡します。
use Faker\Factory as Faker; public function run() { $faker = Faker::create(); for(i = 0; i <= 99; i++) { User::create([ 'first_name' => $faker->firstName(), 'last_name' => $faker->lastName(), 'email' => $faker->email(), 'password' => bcrypt('hello1234'), // we might want to set this to a value we can easily remember for testing sake 'user_type' => $faker->randomElement(['admin', 'user']) ]); } }
これをデータベースにシードするには、以下のコマンドを実行する必要があります:
php artisan db:seed --class=UserSeeder
これにより 100 人のユーザーが作成され、データベースに挿入されます。
それはとても簡単です。
それでは、より高度なソリューションに移ります。
次のものを作成する必要があります:
- 10 の新しい部門を含む部門テーブル。
- 新しい 100 ユーザーを作成します。
- 同じ詳細で users テーブル を参照する Staff テーブルと、Departments テーブル (つまり、Department_id) を参照するための追加の列を参照します。
ユーザーテーブルがどのようになるかはすでにわかっています。スタッフテーブルと部門テーブルがどのようになるかを見てみましょう。
スタッフテーブル
Schema::create('staff', function (Blueprint $table) { $table->id(); $table->string('first_name'); $table->string('last_name'); $table->string('email'); $table->foreignId('department_id')->references('id')->on('departments'); $table->foreignId('user_id')->references('id')->on('users'); $table->timestamps(); });
部門テーブル:
Schema::create('departments', function (Blueprint $table) { $table->id(); $table->string('name'); $table->timestamps(); });
したがって、これらのテーブルをデータベースに含めるために移行を実行する必要があります。
次に、UserSeeder クラスについて、前に述べた箇条書きを実装します。
- 10 の新しい部門を含む部門テーブル。
$now = now(); $count = 0; $departmentNames = ['Electronics', 'Home & Garden', 'Toys & Games', 'Health & Beauty', 'Automotive', 'Sports & Outdoors','Clothing & Accessories', 'Books', 'Music', 'Movies & TV', 'Grocery' ]; for ($j=0; $j <= 9; $j++){ Department::create([ 'name' => $departmentNames[$j], 'created_at' => $now, 'updated_at' => $now, ]); $count++; }
最後の 2 つの箇条書きは一緒に実装する必要があります
- 新しい 100 ユーザーを作成します
- 同じ詳細で users テーブル を参照する Staff テーブルと、Departments テーブル (つまり、Department_id) を参照する追加の列を参照します。
if ($count == 10) { // we need to make sure we have all 10 departments $departments = Department::pluck('id')->toArray(); for ($i=0; $i <= 99; $i++) { $user = User::create([ 'first_name' => $faker->firstName(), 'last_name' => $faker->lastName(), 'email' => $faker->email(), 'password' => bcrypt('hello1234'), 'user_type' => $faker->randomElement(['admin', 'user']), 'created_at' => $now, 'updated_at' => $now ]); Staff::create([ 'first_name' => $user->first_name, 'last_name' => $user->last_name, 'email' => $user->email, 'user_id' => $user->id, 'department_id' => $faker->randomElement($departments), // pick random departments from DB 'created_at' => $now, 'updated_at' => $now ]); } }
説明:
最初に 10 個の部門を作成しました。
次に、10 部門すべてが作成されたかどうかを確認するカウンターを設定します。
カウンター条件が true の場合、100 人のユーザーを作成します。
これら 100 人のユーザーごとに、staffs テーブルと呼ばれる別のテーブルで詳細を参照する必要があります。
各スタッフはユーザーに属している必要があり、また部門にも属している必要があります。そのため、これを行うには、前に作成したすべての部門を取得して、部門 ID 列にランダムに挿入する必要があります。
完全な UserSeeder 実装
$now = now(); $count = 0; $departmentNames = ['Electronics', 'Home & Garden', 'Toys & Games', 'Health & Beauty', 'Automotive', 'Sports & Outdoors','Clothing & Accessories', 'Books', 'Music', 'Movies & TV', 'Grocery' ]; for ($j=0; $j <= 9; $j++){ Department::create([ 'name' => $departmentNames[$j], 'created_at' => $now, 'updated_at' => $now, ]); $count++; } $faker = Faker::create(); if ($count == 10) { $departments = Department::pluck('id')->toArray(); for ($i=0; $i <= 99; $i++) { $user = User::create([ 'first_name' => $faker->firstName(), 'last_name' => $faker->lastName(), 'email' => $faker->email(), 'password' => bcrypt('hello1234'), 'user_type' => $faker->randomElement(['admin', 'user']), 'created_at' => $now, 'updated_at' => $now ]); Staff::create([ 'first_name' => $user->first_name, 'last_name' => $user->last_name, 'email' => $user->email, 'user_id' => $user->id, 'department_id' => $faker->randomElement($departments), // pick random departments from DB 'created_at' => $now, 'updated_at' => $now ]); } }
次に以下を実行します:
php artisan make:seeder --class=UserSeeder
ご質問がございましたら、お気軽にお問い合わせください。コーディングを楽しんでください!
以上がLaravel のデータベースシーダーを使用してデータを簡単に生成する方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










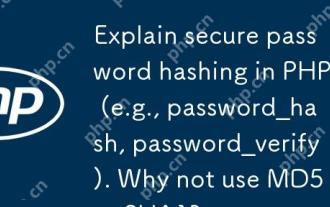
PHPでは、Password_hashとpassword_verify関数を使用して安全なパスワードハッシュを実装する必要があり、MD5またはSHA1を使用しないでください。 1)password_hashセキュリティを強化するために、塩値を含むハッシュを生成します。 2)password_verifyハッシュ値を比較して、パスワードを確認し、セキュリティを確保します。 3)MD5とSHA1は脆弱であり、塩の値が不足しており、最新のパスワードセキュリティには適していません。
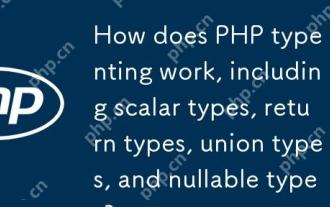
PHPタイプは、コードの品質と読みやすさを向上させるためのプロンプトがあります。 1)スカラータイプのヒント:php7.0であるため、基本データ型は、int、floatなどの関数パラメーターで指定できます。 3)ユニオンタイプのプロンプト:PHP8.0であるため、関数パラメーターまたは戻り値で複数のタイプを指定することができます。 4)Nullable Typeプロンプト:null値を含めることができ、null値を返す可能性のある機能を処理できます。
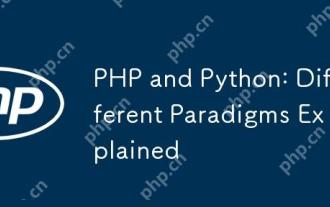
PHPは主に手順プログラミングですが、オブジェクト指向プログラミング(OOP)もサポートしています。 Pythonは、OOP、機能、手続き上のプログラミングなど、さまざまなパラダイムをサポートしています。 PHPはWeb開発に適しており、Pythonはデータ分析や機械学習などのさまざまなアプリケーションに適しています。
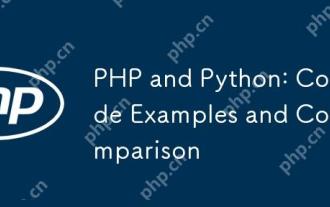
PHPとPythonには独自の利点と短所があり、選択はプロジェクトのニーズと個人的な好みに依存します。 1.PHPは、大規模なWebアプリケーションの迅速な開発とメンテナンスに適しています。 2。Pythonは、データサイエンスと機械学習の分野を支配しています。
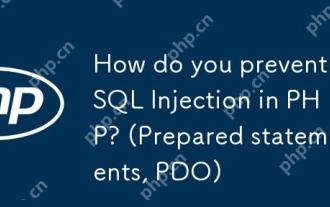
PHPで前処理ステートメントとPDOを使用すると、SQL注入攻撃を効果的に防ぐことができます。 1)PDOを使用してデータベースに接続し、エラーモードを設定します。 2)準備方法を使用して前処理ステートメントを作成し、プレースホルダーを使用してデータを渡し、メソッドを実行します。 3)結果のクエリを処理し、コードのセキュリティとパフォーマンスを確保します。
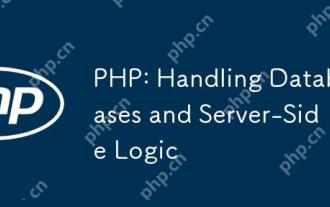
PHPはMySQLIおよびPDO拡張機能を使用して、データベース操作とサーバー側のロジック処理で対話し、セッション管理などの関数を介してサーバー側のロジックを処理します。 1)MySQLIまたはPDOを使用してデータベースに接続し、SQLクエリを実行します。 2)セッション管理およびその他の機能を通じて、HTTPリクエストとユーザーステータスを処理します。 3)トランザクションを使用して、データベース操作の原子性を確保します。 4)SQLインジェクションを防ぎ、例外処理とデバッグの閉鎖接続を使用します。 5)インデックスとキャッシュを通じてパフォーマンスを最適化し、読みやすいコードを書き、エラー処理を実行します。
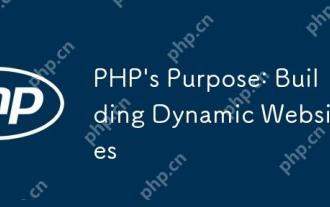
PHPは動的なWebサイトを構築するために使用され、そのコア関数には次のものが含まれます。1。データベースに接続することにより、動的コンテンツを生成し、リアルタイムでWebページを生成します。 2。ユーザーのインタラクションを処理し、提出をフォームし、入力を確認し、操作に応答します。 3.セッションとユーザー認証を管理して、パーソナライズされたエクスペリエンスを提供します。 4.パフォーマンスを最適化し、ベストプラクティスに従って、ウェブサイトの効率とセキュリティを改善します。
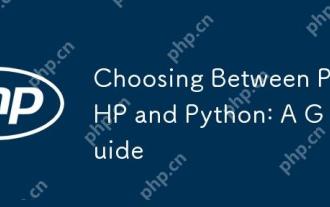
PHPはWeb開発と迅速なプロトタイピングに適しており、Pythonはデータサイエンスと機械学習に適しています。 1.PHPは、単純な構文と迅速な開発に適した動的なWeb開発に使用されます。 2。Pythonには簡潔な構文があり、複数のフィールドに適しており、強力なライブラリエコシステムがあります。
