Vueアンプとの違いVue3
Vue.js は、ユーザー インターフェイスを構築するための人気のある JavaScript フレームワークです。 Vue 3 のリリースでは、Vue 2 と比較して大幅な改善と新機能が追加されました。この投稿では、Vue 2 と Vue 3 の詳細な比較を提供し、主な違いと強化点を強調し、これらの変更を説明するコード スニペットを示します。
1. 反応性システム
Vue 2:
実装:
Vue 2 の反応性システムは Object.defineProperty に基づいています。このメソッドは、各プロパティのゲッターとセッターを定義することにより、プロパティのアクセスと変更をインターセプトします。
// Vue 2 reactivity using Object.defineProperty const data = { message: 'Hello Vue 2' }; Object.defineProperty(data, 'message', { get() { // getter logic }, set(newValue) { // setter logic console.log('Message changed to:', newValue); } }); data.message = 'Hello World'; // Console: Message changed to: Hello World
制限事項:
- プロパティの追加/削除: Vue 2 はプロパティの追加または削除を動的に検出できません。
- 配列の変更: Vue 2 では、変更を追跡するために特定の配列の変更メソッド (プッシュ、ポップ、スプライスなど) が必要ですが、制限があり直感的ではない可能性があります。
Vue 3:
実装:
Vue 3 は反応性システムに ES6 プロキシを使用します。これにより、フレームワークは、より包括的かつ煩わしくない方法でオブジェクトと配列への変更を傍受して監視できます。
// Vue 3 reactivity using Proxy const data = Vue.reactive({ message: 'Hello Vue 3' }); Vue.watchEffect(() => { console.log('Message changed to:', data.message); }); data.message = 'Hello World'; // Console: Message changed to: Hello World
利点:
動的変更: Vue 3 はプロパティの追加と削除を反応的に検出できます。
パフォーマンスの向上: プロキシベースのシステムは、パフォーマンスが向上し、オーバーヘッドが少なくなります。
2. 構成API
Vue 2:
可用性:
Composition API は、Vue Comboposition API プラグイン経由で利用できます。
// Vue 2 component using Options API Vue.component('my-component', { data() { return { count: 0 }; }, methods: { increment() { this.count++; } }, template: `<button @click="increment">{{ count }}</button>` });
使用法:
開発者は主に、コンポーネント コードをデータ、メソッド、計算などのセクションに編成するオプション API を使用します。
Vue 3:
内蔵:
Composition API は Vue 3 にネイティブに組み込まれており、Options API の代替手段を提供します。
// Vue 3 component using Composition API import { defineComponent, ref } from 'vue'; export default defineComponent({ setup() { const count = ref(0); const increment = () => count.value++; return { count, increment }; }, template: `<button @click="increment">{{ count }}</button>` });
利点:
- ロジックの再利用: ロジックの再利用と合成を容易にします。
- コード構成: 関連するロジックをグループ化して、コードをよりモジュール化して保守しやすくします。
3. パフォーマンス
Vue 2:
レンダリング:
差分アルゴリズムを備えた従来の仮想 DOM を使用します。
最適化: 特に大規模なアプリケーションでは、最適化の範囲が限られています。
Vue 3:
レンダリング:
仮想 DOM が改善され、差分アルゴリズムが最適化されました。
木の揺れ:
ツリーシェーキング機能が強化され、未使用のコードが削除されることでバンドルサイズが小さくなります。
メモリ管理:
より効率的なデータ構造と最適化により、メモリ使用量が向上します。
4. TypeScript のサポート
Vue 2:
基本サポート:
Vue 2 は TypeScript をサポートしていますが、追加の構成が必要であり、シームレスではない可能性があります。
ツーリング:
TypeScript のツールとサポートはそれほど統合されていません。
// Vue 2 with TypeScript import Vue from 'vue'; import Component from 'vue-class-component'; @Component export default class MyComponent extends Vue { message: string = 'Hello'; greet() { console.log(this.message); } }
Vue 3:
ファーストクラスのサポート:
Vue 3 は、より優れた型推論とツールを備えたファーストクラスの TypeScript サポートを提供します。
統合:
TypeScript を念頭に置いて設計されており、使いやすく、より良い開発エクスペリエンスを提供します。
// Vue 3 with TypeScript import { defineComponent, ref } from 'vue'; export default defineComponent({ setup() { const message = ref<string>('Hello'); const greet = () => { console.log(message.value); }; return { message, greet }; } });
5. 新機能と機能強化
Vue 3 では、Vue 2 では利用できないいくつかの新機能が導入されています。
- Teleport: DOM ツリーの親コンポーネントとは異なる部分でコンポーネントをレンダリングできるようにします。モーダル、ツールチップ、および同様の UI 要素に役立ちます。
<!-- Vue 3 Teleport feature --> <template> <div> <h1>Main Content</h1> <teleport to="#modals"> <div class="modal"> <p>This is a modal</p> </div> </teleport> </div> </template> <script> export default { name: 'App' }; </script> <!-- In your HTML --> <div id="app"></div> <div id="modals"></div>
- フラグメント: コンポーネントのテンプレートで複数のルート ノードをサポートし、単一のルート要素が不要になります。
<!-- Vue 2 requires a single root element --> <template> <div> <h1>Title</h1> <p>Content</p> </div> </template>
<!-- Vue 3 supports fragments with multiple root elements --> <template> <h1>Title</h1> <p>Content</p> </template>
- Suspense: コンポーネントの非同期依存関係を処理するメカニズム。非同期操作が完了するのを待機している間にフォールバック コンテンツを表示する方法を提供します。
<!-- Vue 3 Suspense feature --> <template> <Suspense> <template #default> <AsyncComponent /> </template> <template #fallback> <div>Loading...</div> </template> </Suspense> </template> <script> import { defineComponent, h } from 'vue'; const AsyncComponent = defineComponent({ async setup() { const data = await fetchData(); return () => h('div', data); } }); export default { components: { AsyncComponent } }; </script>
- 複数のルート要素: コンポーネントのテンプレートに複数のルート要素を含めることができるため、テンプレート設計の柔軟性が高まります。
6. 生態系
Vue 2:
成熟したエコシステム:
Vue 2 には、幅広い安定したライブラリ、プラグイン、ツールを備えた確立されたエコシステムがあります。
コミュニティサポート:
広範なコミュニティ サポートとリソースが利用可能です。
Vue 3:
成長するエコシステム:
Vue 3 エコシステムは急速に成長しており、Vue 3 の機能を活用するために多くのライブラリやツールが更新または新規作成されています。
互換性:
一部の Vue 2 ライブラリはまだ完全には互換性がありませんが、コミュニティは更新と新しいリリースに積極的に取り組んでいます。
7. 移行
Vue 2 から Vue 3 への移行:
- 移行ガイド: Vue チームは、開発者が Vue 2 から Vue 3 に移行できるように、詳細な移行ガイドを提供しています。このガイドでは、必要な手順と重大な変更の概要が説明されています。
- 互換性ビルド: Vue 3 は、ほとんどの Vue 2 API との下位互換性を提供する互換性ビルドを提供し、段階的な移行プロセスを可能にします。
まとめ:
- 反応性システム: Vue 3 のプロキシベースの反応性システムは、Vue 2 の Object.defineProperty システムよりも効率的かつ柔軟です。
- Composition API: Vue 3 に組み込まれ、より強力になり、コードの編成とロジックの再利用が強化されます。
- パフォーマンス: レンダリング、ツリーシェイキング、メモリ管理が改善され、Vue 3 が大幅に改善されました。
- TypeScript サポート: Vue 3 は最上級の TypeScript サポートを提供し、統合と使用が容易になります。
- 新機能: Vue 3 では、テレポート、フラグメント、サスペンス、および複数のルート要素のサポートが導入され、より柔軟で強力な機能が提供されます。
- エコシステム: Vue 2 には成熟したエコシステムがありますが、Vue 3 のエコシステムはコミュニティの積極的なサポートにより急速に成長しています。
- 移行: Vue 3 は、Vue 2 からの移行を促進するためのツールとガイドを提供し、よりスムーズな移行を保証します。
Vue 3 では、より効率的な反応性システム、組み込みのコンポジション API、強化されたパフォーマンス、最上級の TypeScript サポート、テレポート、フラグメント、サスペンスなどの新機能など、Vue 2 に比べていくつかの改善点と新機能が追加されています。これらの変更により、最新の Web アプリケーションを構築するための柔軟性、パフォーマンスが向上し、より強力なフレームワークが提供されます。
新しいプロジェクトを開始する場合は、高度な機能と将来のサポートのため、Vue 3 が推奨されます。既存のプロジェクトについては、Vue 2 には依然として成熟したエコシステムと強力なサポートがあり、Vue 3 への明確な移行パスが用意されています。
Vue 2 または Vue 3 の特定の機能について、さらに例や説明が必要ですか?コメント欄でお知らせください!
以上がVueアンプとの違いVue3の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










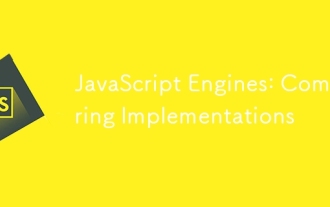
さまざまなJavaScriptエンジンは、各エンジンの実装原則と最適化戦略が異なるため、JavaScriptコードを解析および実行するときに異なる効果をもたらします。 1。語彙分析:ソースコードを語彙ユニットに変換します。 2。文法分析:抽象的な構文ツリーを生成します。 3。最適化とコンパイル:JITコンパイラを介してマシンコードを生成します。 4。実行:マシンコードを実行します。 V8エンジンはインスタントコンピレーションと非表示クラスを通じて最適化され、Spidermonkeyはタイプ推論システムを使用して、同じコードで異なるパフォーマンスパフォーマンスをもたらします。
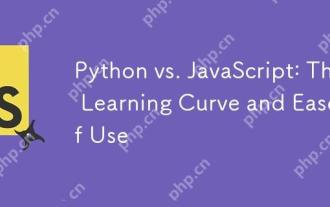
Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。
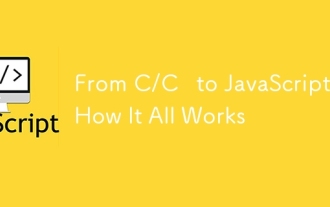
C/CからJavaScriptへのシフトには、動的なタイピング、ゴミ収集、非同期プログラミングへの適応が必要です。 1)C/Cは、手動メモリ管理を必要とする静的に型付けられた言語であり、JavaScriptは動的に型付けされ、ごみ収集が自動的に処理されます。 2)C/Cはマシンコードにコンパイルする必要がありますが、JavaScriptは解釈言語です。 3)JavaScriptは、閉鎖、プロトタイプチェーン、約束などの概念を導入します。これにより、柔軟性と非同期プログラミング機能が向上します。
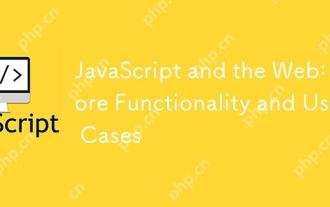
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
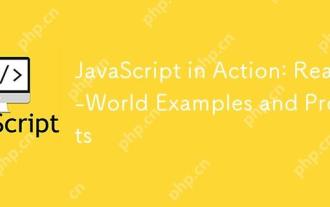
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
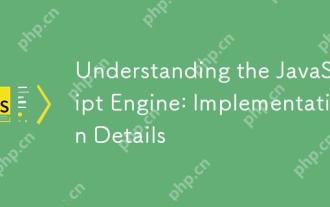
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。
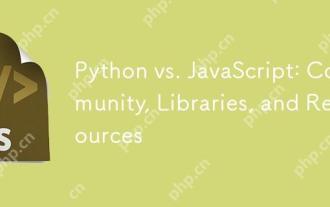
PythonとJavaScriptには、コミュニティ、ライブラリ、リソースの観点から、独自の利点と短所があります。 1)Pythonコミュニティはフレンドリーで初心者に適していますが、フロントエンドの開発リソースはJavaScriptほど豊富ではありません。 2)Pythonはデータサイエンスおよび機械学習ライブラリで強力ですが、JavaScriptはフロントエンド開発ライブラリとフレームワークで優れています。 3)どちらも豊富な学習リソースを持っていますが、Pythonは公式文書から始めるのに適していますが、JavaScriptはMDNWebDocsにより優れています。選択は、プロジェクトのニーズと個人的な関心に基づいている必要があります。
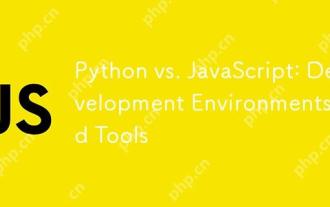
開発環境におけるPythonとJavaScriptの両方の選択が重要です。 1)Pythonの開発環境には、Pycharm、Jupyternotebook、Anacondaが含まれます。これらは、データサイエンスと迅速なプロトタイピングに適しています。 2)JavaScriptの開発環境には、フロントエンドおよびバックエンド開発に適したnode.js、vscode、およびwebpackが含まれます。プロジェクトのニーズに応じて適切なツールを選択すると、開発効率とプロジェクトの成功率が向上する可能性があります。
