Python - 辞書、セット、タプル
これら 3 つはすべて、Python の異なるタイプのデータ構造です。これは、さまざまなデータ コレクションを保存するために使用されます。要件のユースケースに基づいて、これらの中から選択する必要があります。
辞書 (dict):
- 辞書はキーと値のペアのコレクションであり、各キーは値に関連付けられています
- キーは一意である必要があるため、キー値に基づいてデータを取得できます (キーベースの検索)。
- 3.7 までは辞書に順序はありませんが、値は変更できます。キー名を直接変更することはできません
構文:
在庫 = {'リンゴ':20, 'バナナ':30 , 'ニンジン':15, 'ミルク':15}
print('t1.在庫アイテム', 在庫)
以下の構文を使用して、辞書に別の値を追加したり、既存のキーの値を変更したりできます
在庫['卵'] = 20
在庫['パン'] = 25
print('t2.更新された在庫アイテム', 在庫)
在庫['卵']= 在庫['卵']+5
print('t3.再入荷後'、在庫)
- dict からのデータの削除は、del キーワードを使用して実行できます。
- データの存在を確認するには、in キーワードを使用します。結果はブール値になります。
在庫削除['キャロット']
在庫を削除['パン']
print('t4.削除後に更新されたインベントリ', inventory)
is_bananas_in_inventory = 在庫に「バナナ」があります
print('t5a. バナナは在庫にあります', is_bananas_in_inventory)
is_oranges_in_inventory = 在庫に「オレンジ」があります
print('t5b. オレンジは在庫にあります', is_oranges_in_inventory)
メモ:
さらに、dict.items() は、辞書内の各項目をタプル (キーと値のペアのような) として与えます。 list(dict.items()) を使用すると、データをリストとして取得することもできます。 for ループと if 条件を使用すると、特定のキーにアクセスし、そのデータに対して目的の操作を実行できます
for product, product_count in inventory.items(): print('\t\t6. Product:', product, 'count is:', product_count) print ('\t7. Iterating inventory gives tuple:', inventory.items()) #Printing only egg count(Value of key 'egg') by itearting dict for product, product_count in inventory.items(): if product is 'egg': print('\t8. Product:', product, ' its count is:', product_count) #Printing egg count (value of key 'egg') print('\t9. Count of apple',inventory['egg'])
Output: 1. Inventory items {'apple': 20, 'Banana': 30, 'carrot': 15, 'milk': 15} 2. Updated Inventory items {'apple': 20, 'Banana': 30, 'carrot': 15, 'milk': 15, 'egg': 20, 'bread': 25} 3. After restocking {'apple': 30, 'Banana': 30, 'carrot': 15, 'milk': 15, 'egg': 25, 'bread': 25} 4. Updated Inventory after delete {'apple': 30, 'Banana': 30, 'milk': 15, 'egg': 25} 5a. Is banana in inventory True 5b. Is Orange in inventory False 6. Product: apple count is: 30 6. Product: Banana count is: 30 6. Product: milk count is: 15 6. Product: egg count is: 25 7. Iterating inventory gives tuple: dict_items([('apple', 30), ('Banana', 30), ('milk', 15), ('egg', 25)]) 8. Product: egg its count is: 25 9. Count of apple 25
設定:
セットは、順序付けされていない一意の要素のコレクションです。セットは変更可能ですが、要素の重複は許可されません。
構文:
botanical_garden = {'バラ'、'蓮'、'ユリ'}
botanical_garden.add('ジャスミン')
botanical_garden.remove('ローズ')
is_present_Jasmine = botanical_garden の「ジャスミン」
上記では、セットを定義し、値を追加して削除することがわかりました。同じ要素をセットに追加すると、エラーが発生します。
ベン図と同様に 2 つのセットを比較することもできます。 2 つのデータセットの結合、差分、共通部分と同様です。
botanical_garden = {'Tuple', 'rose', 'Lily', 'Jasmine', 'lotus'} rose_garden = {'rose', 'lotus', 'Hybiscus'} common_flower= botanical_garden.intersection(rose_garden) flowers_only_in_bg = botanical_garden.difference(rose_garden) flowers_in_both_set = botanical_garden.union(rose_garden) Output will be a set by default. If needed we can typecase into list using list(expression)
タプル:
タプルは、要素の順序付けされたコレクションであり、不変です。つまり、作成後に変更することはできません。
構文:
ooty_trip = ('Ooty', '2024-1-1', 'Botanical_Garden') munnar_trip = ('Munar', '2024-06-06', 'Eravikulam National Park') germany_trip = ('Germany', '2025-1-1', 'Lueneburg') print('\t1. Trip details', ooty_trip, germany_trip) #Accessing tuple using index location = ooty_trip[0] date = ooty_trip[1] place = ooty_trip[2] print(f'\t2a. Location: {location} Date: {date} Place: {place} ') location, date, place =germany_trip # Assinging a tuple to 3 different variables print(f'\t2b. Location: {location} Date: {date} Place: {place} ') print('\t3. The count of ooty_trip is ',ooty_trip.count) Output: 1. Trip details ('Ooty', '2024-1-1', 'Botanical_Garden') ('Germany', '2025-1-1', 'Lueneburg') 2a. Location: Ooty Date: 2024-1-1 Place: Botanical_Garden 2b. Location: Germany Date: 2025-1-1 Place: Lueneburg 3. The count of ooty_trip is <built-in method count of tuple object at 0x0000011634B3DBC0>
タプルにはインデックスを使用してアクセスできます。タプルの値は複数の変数に簡単に割り当てることができます。 2 つのタプルを結合して、別のタプルを作成できます。ただし、タプルは変更できません。
以上がPython - 辞書、セット、タプルの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










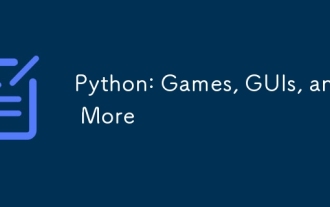
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。
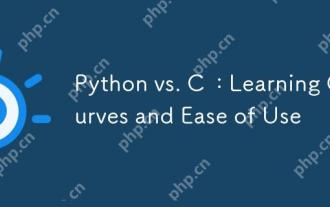
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
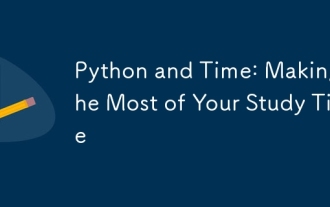
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
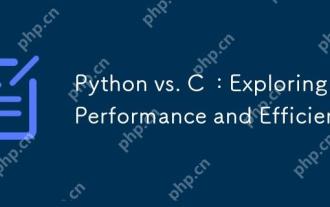
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
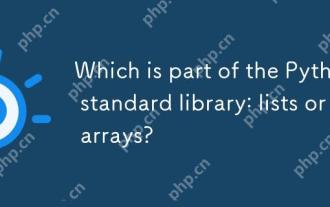
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
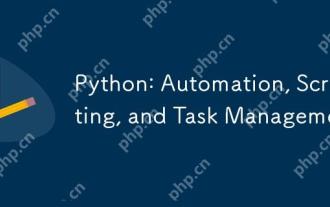
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
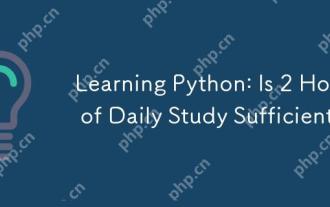
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
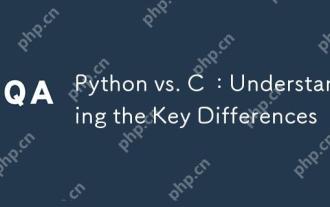
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
