MERN スタック アプリケーションの構築 - ベスト プラクティスとヒント
1. MERN スタックを理解する
MERN スタックは、MongoDB、Express.js、React.js、Node.js を組み合わせた、最新の Web アプリケーションを構築するための最も人気のある選択肢の 1 つとなっています。 MERN スタックは、フルスタック JavaScript フレームワークとして、フロントエンド開発者とバックエンド開発者の両方にシームレスな開発エクスペリエンスを提供します。ただし、MERN スタック アプリケーションを構築するには、最適なパフォーマンスとスケーラビリティを確保するために慎重な計画と実装が必要です。このブログでは、MERN スタック アプリケーションを構築するためのベスト プラクティスとヒントを探り、API 統合、データベース管理から認証、デプロイメントまですべてをカバーします。フルスタック開発が初めての場合でも、既存の MERN スタック プロジェクトの改善を検討している場合でも、このブログは堅牢で効率的な Web アプリケーションの構築に役立つ貴重な洞察を提供します。
2. MERN スタック アプリケーションを構築するためのベスト プラクティス
MERN スタック アプリケーションを確実に成功させるには、効率、拡張性、保守性を促進するベスト プラクティスに従うことが不可欠です。以下に、留意すべき主要なベスト プラクティスをいくつか示します:
- コードをモジュール化します:
コードを再利用可能なコンポーネントに分割して、コードの構成と再利用性を強化します。
// Example: Modularizing a React Component function Header() { return <h1>My MERN Stack App</h1>; } function App() { return ( <div> <Header /> {/* Other components */} </div> ); }
- 適切なエラー処理を実装します:
エラーを適切に処理して、ユーザー エクスペリエンスを向上させ、デバッグを容易にします。
// Example: Express.js Error Handling Middleware app.use((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something broke!'); });
- パフォーマンスの最適化:
効率的なコードを作成し、キャッシュ メカニズムを利用し、データベース クエリを最適化してパフォーマンスを向上させます。
// Example: Using Redis for Caching const redis = require('redis'); const client = redis.createClient(); app.get('/data', (req, res) => { client.get('data', (err, data) => { if (data) { res.send(JSON.parse(data)); } else { // Fetch data from database and cache it } }); });
- 徹底的なテストを実施します:
アプリケーションを厳密にテストして、開発プロセスの早い段階で問題を特定して修正します。
// Example: Jest Unit Test for a Function test('adds 1 + 2 to equal 3', () => { expect(1 + 2).toBe(3); });
- セキュリティのベストプラクティスに従ってください:
安全な認証方法を実装し、ユーザー入力をサニタイズし、一般的なセキュリティ脆弱性から保護します。
// Example: Sanitizing User Input const sanitizeHtml = require('sanitize-html'); const cleanInput = sanitizeHtml(userInput);
これらのベスト プラクティスに従うことで、最高の品質基準を満たす、信頼性が高くスケーラブルな MERN スタック アプリケーションを構築できます。
a.適切なツールとテクノロジーの選択
MERN スタック アプリケーションを構築する場合、適切なツールとテクノロジを選択することが重要です。適切なライブラリ、フレームワーク、開発ツールを選択すると、プロジェクトの品質と効率に大きな影響を与える可能性があります。これらの決定を行う際には、コミュニティ サポート、アプリケーション要件との互換性、統合の容易さなどの要素を考慮してください。たとえば、信頼性の高いパフォーマンスを実現するには、Express.js や React.js などの確立されたライブラリを選択します。さらに、API テストには Postman、データベース管理には MongoDB Compass などのツールを活用して、開発プロセスを合理化します。プロジェクトのニーズに合ったツールとテクノロジーを慎重に選択することで、生産性を向上させ、一流の MERN スタック アプリケーションを提供できます。
b.クリーンでスケーラブルなコードを維持する
MERN スタック アプリケーションの持続性とスケーラビリティを確保するには、開発プロセス全体を通じてクリーンで効率的なコードを維持することが最も重要です。一貫したコーディング スタイルに従う、モジュール化を利用する、設計パターンを実装するなどのベスト プラクティスに従うことで、コードの可読性と管理性が向上します。コードベースを定期的にリファクタリングして、冗長性を排除し、パフォーマンスを向上させます。コードベースの理解と保守を支援するために、意味のあるコメントやドキュメントを書くことの重要性を強調します。クリーンでスケーラブルなコードを優先することで、MERN スタック アプリケーションの長期的な成功のための強固な基盤を築くことができます。
// Example: Using ES6 Modules for Clean Code import express from 'express'; import { connectToDB } from './db'; import routes from './routes'; const app = express(); connectToDB(); app.use('/api', routes);
c.セキュリティ対策の実施
MERN スタック アプリケーションを潜在的なサイバー脅威から保護するには、堅牢なセキュリティ対策を実装することが重要です。ユーザーの認可と認証には、JSON Web Token (JWT) などの安全な認証方法を利用します。 SQL インジェクションやクロスサイト スクリプティング攻撃などの一般的なセキュリティ脆弱性を防ぐために入力検証を実装します。依存関係を定期的に更新して、アプリケーションで使用されているパッケージで報告されたセキュリティの脆弱性に対処します。セキュリティ監査と侵入テストを実施して、潜在的なセキュリティの抜け穴を特定して修正します。 MERN スタック アプリケーションのセキュリティを確保することは、ユーザーの信頼を維持し、機密データを保護するために不可欠であることを忘れないでください。
// Example: Implementing JWT Authentication import jwt from 'jsonwebtoken'; function authenticateToken(req, res, next) { const token = req.header('Authorization'); if (!token) return res.status(401).send('Access Denied'); try { const verified = jwt.verify(token, process.env.JWT_SECRET); req.user = verified; next(); } catch (err) { res.status(400).send('Invalid Token'); } }
3. パフォーマンスを最適化するためのヒント
To optimize performance in your MERN stack application, consider the following tips:
- Implement server-side rendering to enhance page load times and improve SEO rankings.
// Example: Implementing SSR with React and Node.js import ReactDOMServer from 'react-dom/server'; import App from './App'; app.get('*', (req, res) => { const html = ReactDOMServer.renderToString(<App />); res.send(`<!DOCTYPE html><html><body>${html}</body></html>`); });
Utilize caching techniques like Redis to store frequently accessed data and reduce latency.
Minify and compress assets to reduce the size of files sent to the client, improving loading speed.
// Example: Using Gzip Compression in Express import compression from 'compression'; app.use(compression());
- Optimize database queries to efficiently retrieve and manipulate data.
// Example: MongoDB Query Optimization db.collection('users').find({ age: { $gte: 21 } }).sort({ age: 1 }).limit(10);
Use performance monitoring tools like New Relic or Datadog to identify bottlenecks and optimize performance.
Employ lazy loading for images and components to decrease initial load times.
// Example: Implementing Lazy Loading in React const LazyComponent = React.lazy(() => import('./LazyComponent')); function App() { return ( <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> ); }
By implementing these performance optimization tips, you can ensure your MERN stack application runs smoothly and efficiently for users.
4. Testing and debugging your MERN stack application
Ensuring the stability and functionality of your MERN stack application is crucial for delivering an exceptional user experience. Prioritize testing by incorporating unit tests, integration tests, and end-to-end testing using tools like Jest, Enzyme, and Mocha. Implement continuous integration and deployment (CI/CD) pipelines to automate testing processes and catch errors early on. Utilize debugging tools like Chrome DevTools to troubleshoot issues and optimize code performance. By dedicating time to testing and debugging, you can identify and resolve potential issues before they impact your users, leading to a more robust and reliable application.
5. Continuous integration and deployment
Implementing a robust continuous integration and deployment (CI/CD) pipeline is essential for streamlining development processes in your MERN stack application. By automating testing, builds, and deployments with tools such as Jenkins or GitLab CI/CD, teams can ensure quicker delivery of features and updates while maintaining code integrity. Integrate version control systems like Git to manage code changes effectively and facilitate collaboration among team members. A well-structured CI/CD pipeline not only enhances productivity but also helps in maintaining the overall quality and reliability of your application. Stay tuned for more insights on optimizing the CI/CD process in our upcoming blogs.
# Example: GitLab CI/CD Pipeline Configuration stages: - build - test - deploy build: script: - npm install - npm run build test: script: - npm run test deploy: script: - npm run deploy
6. Conclusion: Embracing best practices for a successful MERN stack application
In conclusion, implementing a well-structured continuous integration and deployment (CI/CD) pipeline, along with leveraging version control systems like Git, is crucial for ensuring the efficiency and quality of your MERN stack application development. By following best practices and incorporating automation tools such as Jenkins or GitLab CI/CD, teams can accelerate delivery timelines and enhance collaboration among team members. Stay committed to optimizing your CI/CD process and integrating the latest industry standards to achieve a successful MERN stack application that meets user expectations and maintains code integrity. Keep exploring new techniques and stay updated with upcoming blogs for more insightful tips on building robust MERN stack applications.
Congratulations if you've made it to this point of the article.
If you found this article useful, let me know in the comments.?
以上がMERN スタック アプリケーションの構築 - ベスト プラクティスとヒントの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










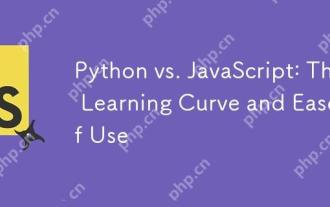
Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。
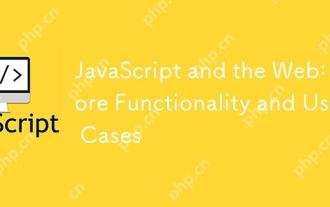
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
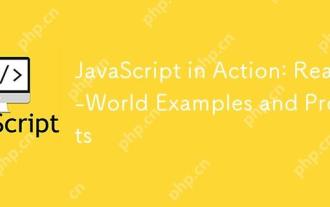
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
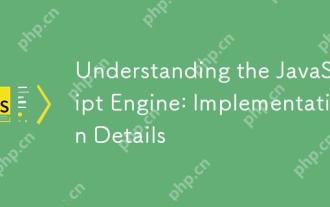
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。
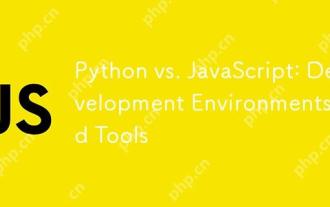
開発環境におけるPythonとJavaScriptの両方の選択が重要です。 1)Pythonの開発環境には、Pycharm、Jupyternotebook、Anacondaが含まれます。これらは、データサイエンスと迅速なプロトタイピングに適しています。 2)JavaScriptの開発環境には、フロントエンドおよびバックエンド開発に適したnode.js、vscode、およびwebpackが含まれます。プロジェクトのニーズに応じて適切なツールを選択すると、開発効率とプロジェクトの成功率が向上する可能性があります。
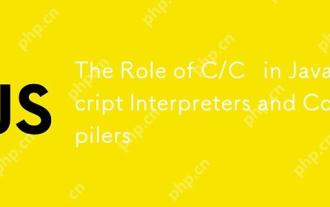
CとCは、主に通訳者とJITコンパイラを実装するために使用されるJavaScriptエンジンで重要な役割を果たします。 1)cは、JavaScriptソースコードを解析し、抽象的な構文ツリーを生成するために使用されます。 2)Cは、Bytecodeの生成と実行を担当します。 3)Cは、JITコンパイラを実装し、実行時にホットスポットコードを最適化およびコンパイルし、JavaScriptの実行効率を大幅に改善します。

Pythonはデータサイエンスと自動化により適していますが、JavaScriptはフロントエンドとフルスタックの開発により適しています。 1. Pythonは、データ処理とモデリングのためにNumpyやPandasなどのライブラリを使用して、データサイエンスと機械学習でうまく機能します。 2。Pythonは、自動化とスクリプトにおいて簡潔で効率的です。 3. JavaScriptはフロントエンド開発に不可欠であり、動的なWebページと単一ページアプリケーションの構築に使用されます。 4. JavaScriptは、node.jsを通じてバックエンド開発において役割を果たし、フルスタック開発をサポートします。
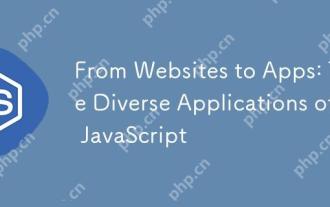
JavaScriptは、Webサイト、モバイルアプリケーション、デスクトップアプリケーション、サーバー側のプログラミングで広く使用されています。 1)Webサイト開発では、JavaScriptはHTMLおよびCSSと一緒にDOMを運用して、JQueryやReactなどのフレームワークをサポートします。 2)ReactNativeおよびIonicを通じて、JavaScriptはクロスプラットフォームモバイルアプリケーションを開発するために使用されます。 3)電子フレームワークにより、JavaScriptはデスクトップアプリケーションを構築できます。 4)node.jsを使用すると、JavaScriptがサーバー側で実行され、高い並行リクエストをサポートします。
