Streamlit を使用した Web アプリとしての機械学習モデルのデプロイメント
導入
機械学習モデルは本質的に、予測を行ったり、データ内のパターンを見つけたりするために使用される一連のルールまたはメカニズムです。非常に簡単に言うと (単純化しすぎることを恐れずに)、Excel の最小二乗法を使用して計算された傾向線もモデルです。ただし、実際のアプリケーションで使用されるモデルはそれほど単純ではありません。多くの場合、単純な方程式だけでなく、より複雑な方程式やアルゴリズムが含まれます。
この投稿では、プロセスの雰囲気をつかむために、非常に単純な機械学習モデルを構築し、それを非常に単純な Web アプリとしてリリースすることから始めます。
ここでは、ML モデル自体ではなく、プロセスのみに焦点を当てます。また、Streamlit と Streamlit Community Cloud を使用して、Python Web アプリケーションを簡単にリリースします。
TL;DR:
機械学習用の人気のある Python ライブラリである scikit-learn を使用すると、データを迅速にトレーニングし、単純なタスク用のわずか数行のコードでモデルを作成できます。その後、モデルは joblib を使用して再利用可能なファイルとして保存できます。この保存されたモデルは、Web アプリケーションの通常の Python ライブラリと同様にインポート/ロードできるため、アプリはトレーニングされたモデルを使用して予測を行うことができます!
アプリの URL: https://yh-machine-learning.streamlit.app/
GitHub: https://github.com/yoshan0921/yh-machine-learning.git
テクノロジースタック
- パイソン
- Streamlit: Web アプリケーション インターフェイスの作成用。
- scikit-learn: 事前トレーニングされたランダム フォレスト モデルをロードして使用します。
- NumPy と Pandas: データの操作と処理用。
- Matplotlib と Seaborn: 視覚化の生成用。
私が作ったもの
このアプリを使用すると、パーマー ペンギン データセットでトレーニングされたランダム フォレスト モデルによって行われた予測を調べることができます。 (トレーニング データの詳細については、この記事の最後を参照してください。)
具体的には、このモデルは、種、島、くちばしの長さ、足ひれの長さ、体の大きさ、性別などのさまざまな特徴に基づいてペンギンの種を予測します。ユーザーはアプリを操作して、さまざまな特徴がモデルの予測にどのような影響を与えるかを確認できます。
予測画面
学習データ・可視化画面
開発ステップ 1 - モデルの作成
ステップ1.1 ライブラリをインポートする
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score import joblib
pandas は、データ操作と分析に特化した Python ライブラリです。 DataFrame を使用したデータの読み込み、前処理、構造化をサポートし、機械学習モデル用のデータを準備します。
sklearn は、トレーニングと評価のためのツールを提供する機械学習用の包括的な Python ライブラリです。この投稿では、ランダム フォレストと呼ばれる学習手法を使用してモデルを構築します。
joblib は、機械学習モデルなどの Python オブジェクトを非常に効率的な方法で保存およびロードするのに役立つ Python ライブラリです。
Step1.2 データの読み込み
df = pd.read_csv("./dataset/penguins_cleaned.csv") X_raw = df.drop("species", axis=1) y_raw = df.species
データセット (トレーニング データ) をロードし、それを特徴 (X) とターゲット変数 (y) に分離します。
Step1.3 カテゴリ変数をエンコードする
encode = ["island", "sex"] X_encoded = pd.get_dummies(X_raw, columns=encode) target_mapper = {"Adelie": 0, "Chinstrap": 1, "Gentoo": 2} y_encoded = y_raw.apply(lambda x: target_mapper[x])
カテゴリ変数は、ワンホット エンコード (X_encoded) を使用して数値形式に変換されます。たとえば、「island」にカテゴリ「Biscoe」、「Dream」、および「Torgersen」が含まれている場合、それぞれに新しい列が作成されます (island_Biscoe、island_Dream、island_Torgersen)。セックスでも同じことが行われます。元のデータが「Biscoe」の場合、island_Biscoe 列は 1 に設定され、その他の列は 0 に設定されます。
ターゲット変数の種は数値 (y_encoded) にマッピングされます。
Step1.4 データセットを分割する
x_train, x_test, y_train, y_test = train_test_split( X_encoded, y_encoded, test_size=0.3, random_state=1 )
モデルを評価するには、トレーニングに使用されていないデータに対するモデルのパフォーマンスを測定する必要があります。 7:3 は、機械学習の一般的な手法として広く使用されています。
Step1.5 ランダムフォレストモデルをトレーニングする
clf = RandomForestClassifier() clf.fit(x_train, y_train)
モデルのトレーニングには Fit メソッドが使用されます。
x_train は説明変数の学習データを表し、y_train はターゲット変数を表します。
このメソッドを呼び出すと、学習データに基づいて学習されたモデルが clf.
Step1.6 モデルを保存する
joblib.dump(clf, "penguin_classifier_model.pkl")
joblib.dump() は、Python オブジェクトをバイナリ形式で保存する関数です。モデルをこの形式で保存すると、モデルをファイルからロードして、再度トレーニングすることなくそのまま使用できます。
サンプルコード
Development Step2 - Building the Web App and Integrating the Model
Step2.1 Import Libraries
import streamlit as st import numpy as np import pandas as pd import joblib
stremlit is a Python library that makes it easy to create and share custom web applications for machine learning and data science projects.
numpy is a fundamental Python library for numerical computing. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently.
Step2.2 Retrieve and encode input data
data = { "island": island, "bill_length_mm": bill_length_mm, "bill_depth_mm": bill_depth_mm, "flipper_length_mm": flipper_length_mm, "body_mass_g": body_mass_g, "sex": sex, } input_df = pd.DataFrame(data, index=[0]) encode = ["island", "sex"] input_encoded_df = pd.get_dummies(input_df, prefix=encode)
Input values are retrieved from the input form created by Stremlit, and categorical variables are encoded using the same rules as when the model was created. Note that the order of each data must also be the same as when the model was created. If the order is different, an error will occur when executing a forecast using the model.
Step2.3 Load the Model
clf = joblib.load("penguin_classifier_model.pkl")
"penguin_classifier_model.pkl" is the file where the previously saved model is stored. This file contains a trained RandomForestClassifier in binary format. Running this code loads the model into clf, allowing you to use it for predictions and evaluations on new data.
Step2.4 Perform prediction
prediction = clf.predict(input_encoded_df) prediction_proba = clf.predict_proba(input_encoded_df)
clf.predict(input_encoded_df): Uses the trained model to predict the class for the new encoded input data, storing the result in prediction.
clf.predict_proba(input_encoded_df): Calculates the probability for each class, storing the results in prediction_proba.
Sample Code
Step3. Deploy
You can publish your developed application on the Internet by accessing the Stremlit Community Cloud (https://streamlit.io/cloud) and specifying the URL of the GitHub repository.
About Data Set
Artwork by @allison_horst (https://github.com/allisonhorst)
The model is trained using the Palmer Penguins dataset, a widely recognized dataset for practicing machine learning techniques. This dataset provides information on three penguin species (Adelie, Chinstrap, and Gentoo) from the Palmer Archipelago in Antarctica. Key features include:
- Species: The species of the penguin (Adelie, Chinstrap, Gentoo).
- Island: The specific island where the penguin was observed (Biscoe, Dream, Torgersen).
- Bill Length: The length of the penguin's bill (mm).
- Bill Depth: The depth of the penguin's bill (mm).
- Flipper Length: The length of the penguin's flipper (mm).
- Body Mass: The mass of the penguin (g).
- Sex: The sex of the penguin (male or female).
This dataset is sourced from Kaggle, and it can be accessed here. The diversity in features makes it an excellent choice for building a classification model and understanding the importance of each feature in species prediction.
以上がStreamlit を使用した Web アプリとしての機械学習モデルのデプロイメントの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










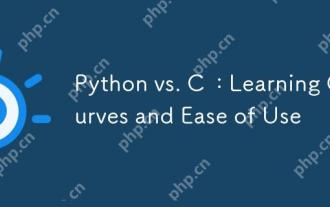
Pythonは学習と使用が簡単ですが、Cはより強力ですが複雑です。 1。Python構文は簡潔で初心者に適しています。動的なタイピングと自動メモリ管理により、使いやすくなりますが、ランタイムエラーを引き起こす可能性があります。 2.Cは、高性能アプリケーションに適した低レベルの制御と高度な機能を提供しますが、学習しきい値が高く、手動メモリとタイプの安全管理が必要です。
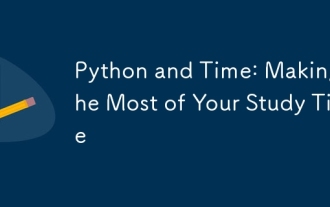
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
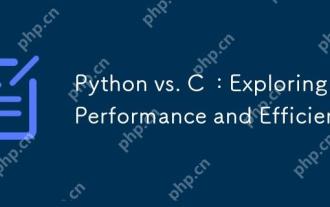
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
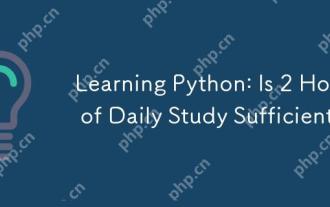
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
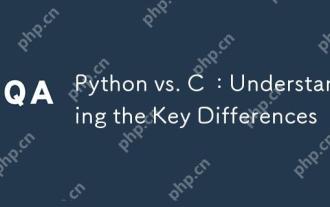
PythonとCにはそれぞれ独自の利点があり、選択はプロジェクトの要件に基づいている必要があります。 1)Pythonは、簡潔な構文と動的タイピングのため、迅速な開発とデータ処理に適しています。 2)Cは、静的なタイピングと手動メモリ管理により、高性能およびシステムプログラミングに適しています。
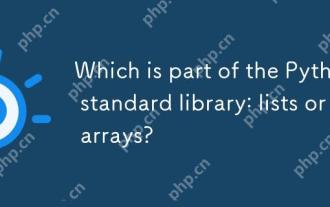
PythonListSarePartOfThestAndardarenot.liestareBuilting-in、versatile、forStoringCollectionsのpythonlistarepart。
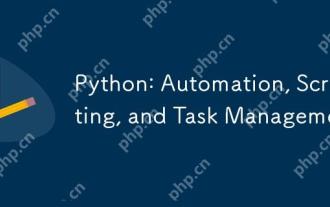
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
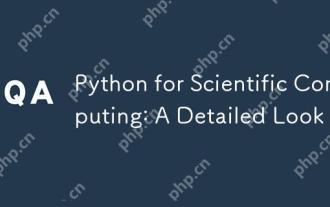
科学コンピューティングにおけるPythonのアプリケーションには、データ分析、機械学習、数値シミュレーション、視覚化が含まれます。 1.numpyは、効率的な多次元配列と数学的関数を提供します。 2。ScipyはNumpy機能を拡張し、最適化と線形代数ツールを提供します。 3. Pandasは、データ処理と分析に使用されます。 4.matplotlibは、さまざまなグラフと視覚的な結果を生成するために使用されます。
