PHP書き込みファイル
PHP には、ファイルに対してさまざまなアクションを実行するために使用されるさまざまな組み込み関数があります。ファイルの作成、開く、読み取り、書き込み、その他の操作を行う可能性があります。
無料ソフトウェア開発コースを始めましょう
Web 開発、プログラミング言語、ソフトウェア テスト、その他
PHP書き込みファイルの機能
以下は、PHP でデフォルトで利用できる主な関数です。
1. fopen()
まず、ファイルに書き込むためには、そのファイルの作成方法を知る必要があります。これは、open() 関数の助けを借りて行われます。ファイルを開くという名前は誤解を招くかもしれませんが、PHP では、Linux の vi 関数と同じように、ファイルの作成と開くために同じ関数が使用されます。この関数は、実行されるとすぐにファイルが存在するかどうかを確認し、ファイルを作成するだけです。以下の例は同じことを示しています:
コード:
<?php // Creating a file for test $myfile = fopen("test.txt", "w") ?>
出力:
2. fwrite()
ファイルを作成した後、必要な内容をファイルに書き込む必要があるため、この関数を同じ目的で使用します。この関数は、ファイルの終わり (EOF) または最初に来る順序に従って指定した長さに達した場合にのみ停止します。
構文:
fwrite(file, string, length)
- ファイルは、書き込むファイルを説明する必須フィールドです
- 文字列は、開いているファイルに書き込むように文字列に指示するもう 1 つの必須パラメータです
- length はオプションのパラメータであり、書き込まれる最大バイト数を与えます
コード:
<?php // Your code here! $f = fopen("testfile.txt", "w"); $text = "Random data here\n"; fwrite($f, $text); ?>
出力:
ここで、testfile.txt は作成されたファイルであり、$text に割り当てられた文字列値がそのファイルに書き込まれます。
3. file_put_contents()
これは、PHP でファイルにコンテンツを書き込むために使用できる別の関数です。ファイルにアクセスするときに従うべき、前述したのと同じ順序で一定数のルールがあります:
- FILE_USE_INCLUDE_PATH というプロパティがあり、設定時にファイル名のコピーがパスに含まれているかどうかをチェックします。
- ファイルの存在を確認してからファイルを作成します
- 次に、ファイルを開きます
- プロパティ LOCK_EX が設定されている場合、ファイルはロックされます
- プロパティ FILE_APPEND が設定されている場合、ファイルの末尾に移動し、設定されていない場合はファイルの内容をクリアします。
- これで、必要なデータがファイルに書き込まれます。
- ファイルを閉じ、ロックが存在する場合は解放します
構文:
file_put_contents(filename, text, mode, context)
- ここで、filename は必須パラメータであり、書き込む必要があるファイルのパス全体を示します。したがって、この関数はファイルをチェックして作成します。
- text は、ファイルに書き込む必要があるデータであるもう 1 つの必須フィールドです。単純な文字列、文字列の配列、またはデータ ストリームの形式にすることができます。
- mode は、ファイルを操作するためのさまざまな方法を提供するオプションのフィールドです。可能な値は次のとおりです:
-
- FILE_USE_INCLUDE_PATH: インクルード ディレクトリ パスで指定されたファイル名を検索します。
-
- FILE_APPEND: データを上書きするのではなく、ファイルに追加します。
-
- LOCK_EX: これにより、書き込み時にファイルに明示的なロックが設定されます。
- context は、ファイルのコンテキストを与えるオプションのパラメータです。これは基本的に、ストリームの動作を変更できる一連のオプションです。
戻り値: この関数は、成功の場合はファイルに書き込まれた合計バイト数を返し、失敗した場合は値 FALSE を返します。
以下は例です:
コード:
<?php echo file_put_contents("filetest.txt","Testing for file write"); ?>
出力:
ここでは、作成しているファイルが最初のパラメータとして指定されており、次のパラメータはそのファイルに書き込まれるテキストです。
4.上書き
上記の既にデータが書き込まれているファイルに上書きすることができます。ファイル内にすでに存在するデータはすべて消去され、まったく新しい空のファイルとして開始されます。以下の例では、既存のファイルを開いて、そこに新しいデータを書き込んでみます。
以下は例です:
コード:
<?php $f = fopen("testfile.txt", "w"); $text = "Random data here\n"; $filetext = "Over writing the data\n"; fwrite($f, $filetext); $filetext = "File Dummy Data\n"; fwrite($f, $filetext); fclose($f); ?>
出力:
Here we are overwriting data in testfile.txt, so whatever is mentioned in the $filetext string value, the same will be written to the file. And when we use the same write command again by changing data given to the $filetext, then the old data is cleaned up and occupied by the latest text value that is provided.
At the end of the file, we always should close it by using the close() function which we have opened using the fwrite() function. As shown in the above example, we also use the \n, which represents the newline equivalent to pressing the enter button on our keyboard.
Now let us take an example and see how to include more data in our file.
Examples to Implement of PHP Write File
Below are the examples of PHP Write File:
Example #1
First, we add 2 lines of data using the below code:
Code:
<?php $testf = "TestFile.txt"; $filehandle = fopen($testf, 'w'); $fdata = "Julian Caesar\n"; fwrite($filehandle, $fdata); $fdata = "Harry James\n"; fwrite($filehandle, $fdata); print "Data writing completed"; fclose($filehandle); ?>
Output:
This creates a file TestFile.txt having 2 lines of data as mentioned.
Example #2
We will append another 2 names in the same file as shown below:
Code:
<?php $testf = "TestFile.txt"; $filehandle = fopen($testf, 'a'); $fdata = "Lilly Potter\n"; fwrite($filehandle, $fdata); $fdata = "Edward Cullen\n"; fwrite($filehandle, $fdata); print "Data has been appended"; fclose($filehandle); ?>
Output:
This example appends the given names to the same file as in the first example.
Conclusion
As shown above, there are various methods and steps that need to be followed when we want to write to a file in PHP. fwrite() is one of the main functions to do this and is used majorly for writing data to a file. They can do basic writing of data to our file but should be used in combination with other mandatory functions like open() and close(), without which it is not possible to perform operations on the file if it does not exist.
Recommended Article
This is a guide to the PHP Write File. Here we discuss the Introduction and its Functions of PHP Write File along with examples and Code Implementation. You can also go through our other suggested articles to learn more-
- Overview of Abstract Class in Python
- What is Abstract Class in PHP?
- Socket Programming in PHP with Methods
- PHP chop() | How to Work?
以上がPHP書き込みファイルの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










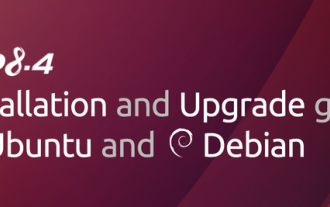
Ubuntu および Debian 用の PHP 8.4 インストールおよびアップグレード ガイド
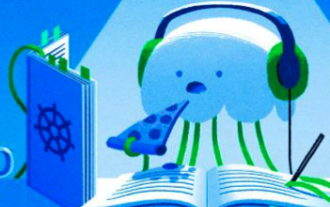
PHP 開発用に Visual Studio Code (VS Code) をセットアップする方法
