この記事では、PHP の文字列の反転について学びます。文字列は裏側から呼び出される文字の集合です。これらの文字のコレクションは、PHP 関数 strrev() を逆にするか、単純な PHP プログラミング コードを使用して使用できます。たとえば、リバースすると、PAVANKUMAR は RAMUKNAVAP
になります。
広告
このカテゴリーの人気コース
PHP 開発者 - 専門分野 | 8コースシリーズ | 3 つの模擬テスト
無料ソフトウェア開発コースを始めましょう
Web 開発、プログラミング言語、ソフトウェア テスト、その他
ロジック:
- まず、文字列を変数に代入します。
- 次に、文字列の長さを計算します。
- 次に、反転した文字列を保存するために新しい変数を作成します。
- その後、for ループ、while ループ、do while ループなどのループを進めます。
- ループ内で文字列を連結します。
- 次に、途中で作成した新しい変数に格納されている反転した文字列を表示/印刷します。
さまざまなループを使用して PHP で文字列を反転する
PHP プログラミング言語で文字列を反転するための、FOR LOOP、WHILE LOOP、DO WHILE LOOP、RECURSIVE METHOD などのさまざまなループ。
1. strrev() 関数の使用
- 以下の例/構文は、文字列を反転するために、すでに定義されている関数 strrev() を使用して元の文字列を反転します。この例では、文字列 (つまり元の文字列) を保存するために $string1 変数が作成され、strtev() 関数の助けを借りて元の文字列と反転された文字列を出力するために echo ステートメントが使用され、次に次のように出力されます。両方を連結します。
- 以下のプログラムの出力を確認して、文字列が反転されているかどうかを確認できます。
コード:
<?php
$string1 = "PAVANKUMAR";
echo "Reversed string of the $string1 is " .strrev ( $string1 );
?>
ログイン後にコピー
出力:

2. For ループの使用
- 以下の例では、string1 変数の値を「PAVANSAKE」として割り当てることにより、「$string1」変数が作成されます。次に、strlen() 関数を使用して、$string1 変数の長さを保存するために、$length 変数が作成されます。ここで、for ループは、初期化、条件、および増分値を「$length1-1」、「$i1>=0」、「$i1 – -」として使用されます。次に、$string1 変数のインデックス値は、$i1 を $length-1 に等しい初期化を使用して裏側から呼び出します。
- 最初は「元の文字列 – 1」の長さの値からFOR LOOPが始まります。次に、条件「i1>=0」をチェックしてループの実行が開始され、元の文字列のインデックスが逆方向に呼び出され、同様にループは条件が FALSE になるまで各反復で後ろから各インデックス値を出力します。最後に、FOR ループを使用して文字列の逆を取得します。
コード:
<?php
$string1 = "PAVANSAKE";
$length1 = strlen($string1);
for ($i1=($length1-1) ; $i1 >= 0 ; $i1--)
{
echo $string1[$i1];
}
?>
ログイン後にコピー
出力:
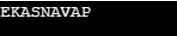
3. While ループの使用
- 以下の例では、元の文字列「PAVANSAKEkumar」を使用して反転された文字列を出力するために while ループが使用されています。
- 以下の syntax/php プログラムでは、最初に $string1 変数に文字列値「PAVANSAKEkumar」が割り当てられ、次に $string1 変数の値の長さを計算し、$length1 変数に格納されます。常に整数になります。 $i1 変数は、文字列変数の値 -1($length1-1) の長さになります。
- ここで条件「$i1>=0」を使用して WHILE ループから開始し、その後、$i1 値が元の文字列のインデックス値の最後であるため、文字列のインデックス値を後ろから出力します。 。この後、元の文字列($i1=$i1-1)を逆にするためにデクリメントが行われます。
コード:
<?php
$string1 = "PAVANSAKEkumar";
$length1 = strlen($string1);
$i1=$length1-1;
while($i1>=0){
echo $string1[$i1];
$i1=$i1-1;
}
?>
ログイン後にコピー
出力:
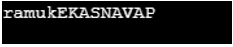
4. do while ループを使用する
- PHP で DO while ループを使用して文字列を反転するプログラムを以下に示します。以下の例では、WHILE LOOP と同じです。すべての論理項は WHILE LOOP と同じですが、その後の部分が少し異なり、最初に条件をチェックするのではなく、最初に出力を出力します。
- つまり、条件の結果が FALSE であっても出力を印刷したとしてもです。
コード:
<?php
$string1 = "PAVANSAKEkumaran";
$length1 = strlen($string1);
$i1=$length1-1;
do{
echo $string1[$i1];
$i1=$i1-1;
}
while($i1>=0)
?>
ログイン後にコピー
出力:
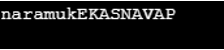
5. Using the Recursion Technique and the substr()
- Here in the below example reversing the string is done using the recursion technique and the substr() function. Substr() function will help in getting the original string’s substring. Here a new function called Reverse() also defined which is passed as an argument with the string.
- At each and every recursive call, substr() method is used in order to extract the argument’s string of the first character and it is called as Reverse () function again just bypassing the argument’s remaining part and then the first character concatenated at the string’s end from the current call. Check the below to know more.
- The reverse () function is created to reverse a string using some code in it with the recursion technique. $len1 is created to store the length of the string specified. Then if the length of the variable equals to 1 i.e. one letter then it will return the same.
- If the string is not one letter then the else condition works by calling the letters from behind one by one using “length – -“ and also calling the function back in order to make a recursive loop using the function (calling the function in function using the library function of PHP). $str1 variable is storing the original string as a variable. Now printing the function result which is the reverse of the string.
Code:
<?php
function Reverse($str1){
$len1 = strlen($str1);
if($len1 == 1){
return $str1;
}
else{
$len1--;
return Reverse(substr($str1,1, $len1))
. substr($str1, 0, 1);
}
}
$str1 = "PavanKumarSake";
print_r(Reverse($str1));
?>
ログイン後にコピー
Output:
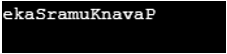
6. Reversing String without using any library functions of PHP
- The below syntax/ program example is done by swapping the index’s characters using the for loop like first character’s index is replaced by the last character’s index then the second character is replaced by the second from the last and so on until we reach the middle index of the string.
- The below example is done without using any of the library functions. Here in the below example, $i2 variable is assigned with the length of the original string-1 and $j2 value is stored with value as “0” then in the loop will continue by swapping the indexes by checking the condition “$j2
Code:
Output:
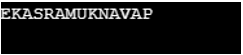
Conclusion
I hope you understood the concept of logic of reversing the input string and how to reverse a string using various techniques using an example for each one with an explanation.
以上がPHP で文字列を反転するの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。