Java の国際化
次の記事では、Java における国際化の概要を説明します。国際化とは、アプリケーションに変更を加えることなく、複数の国、言語、通貨を自動的にサポートするような Web アプリケーションを作成するプロセスです。文字 I と N の間に 18 文字があるため、I18N とも呼ばれます。今日、ソフトウェアや Web サイトを設計する場合、世界的な市場は重要な要素です。世界市場向けのソフトウェア アプリケーションの拡大が続く中、企業は現地の地域や言語のユーザーを魅了する製品を作成する必要があります。
無料ソフトウェア開発コースを始めましょう
Web 開発、プログラミング言語、ソフトウェア テスト、その他
国際市場向けのソフトウェアに取り組んでいる開発者は、それぞれの文化の習慣と違いを認識する必要があります。言語、句読点、通貨、日付、時刻、数字、タイムゾーンはすべて違いの例です。最初の文字「L」と最後の文字「N」の間に 10 文字があるため、ローカリゼーションは I10N とも短縮されます。ローカリゼーションとは、アプリケーションを特定の言語と場所に合わせて調整できるように、ロケール固有のテキストとコンポーネントをアプリケーションに追加するプロセスです。
Java における国際化の構文
次のクラスを使用して国際化を実装できます:
- ロケール
- 数値形式
- 日付形式
1.ロケール
Locale オブジェクトは、地理的位置または言語を表すために使用できます。 java.util パッケージには Locale クラスが含まれています。
Locale クラスのコンストラクター:
Locale l = new Locale(String language);
Locale l = new Locale(String language, String country);
ロケールクラスの定数:
一部の Locale 定数は Locale クラスですでに宣言されています。
これらの定数は直接使用できます。いくつかの定数を以下に示します。
- ロケール。イギリス
- ロケール.ITALY
- ロケール.US
ロケールクラスの関数:
- pubic static Locale getDefault(): このメソッドは、現在のロケールのインスタンスを取得するために使用されます。
- public static Locale[] getAvailableLocales(): このメソッドは、現在のロケールの配列を取得するために使用されます。
- public String getDisplayLanguage(Locale l): このメソッドは、渡されたロケール オブジェクトの言語名を取得するために使用されます。
- public String getDisplayVariant(Locale l): このメソッドは、渡されたロケール オブジェクトのバリアント コードを取得するために使用されます。
- public String getDisplay Country(Locale l): このメソッドは、渡されたロケール オブジェクトの国名を取得するために使用されます。
- public static getISO3Language(): このメソッドは、ロケールの現在の言語コードの 3 文字の省略形を取得するために使用されます。
- public static getISO3 Country(): このメソッドは、ロケールの現在の国の 3 文字の略称を取得するために使用されます。
2.数値形式
NumberFormat クラスを使用すると、特定のロケールに従って数値を書式設定できます。 NumberFormat クラスは java.Text パッケージに存在し、抽象クラスであるため、そのコンストラクターを使用してオブジェクトを作成できません。
ロケールクラスの関数:
- public static NumberFormat getInstance(): このメソッドは、デフォルトの Locale のオブジェクトを取得するために使用されます。
- public static NumberFormat getInstance(Locale l): このメソッドは、渡された Locale オブジェクトのオブジェクトを取得するために使用されます。
- public static NumberFormat getCurrencyInstance(): このメソッドは、特定の通貨で表示するデフォルト ロケールのオブジェクトを取得するために使用されます。
- public static format(long l):このメソッドは、渡された数値をロケール オブジェクトに変換するために使用されます。
3.日付形式
日付の形式は場所によって異なるため、日付形式を国際化しています。 DateFromat クラスを使用すると、指定されたロケールに従って日付をフォーマットできます。 DateFormat は java.text パッケージの抽象クラスです。
ロケールクラスの定数:
一部の DateFormat 定数は、DateFormat クラスですでに宣言されています。
これらの定数は直接使用できます。いくつかの定数を以下に示します。
- DateFormat.LONG
- DateFormat.MINUTE_FIELD
- DateFormat.MINUTE_FIELD
DateFormat クラスの関数:
- final String format(Date date): This method converts and returns the specified Date object into a string.
- static final DateFormat getInstance(): This method is used to gets a date-time formatter with a short formatting style for date and time.
- static Locale[] getAvailableLocales(): This method is used to gets an array of present locales.
- static final DateFormat getTimeInstance(): This method is used to gets time formatter with default formatting style for the default locale.
- static final DateFormat getTimeInstance(int style): This method is used to gets time formatter with the specified formatting style for the default locale.
- static final DateFormat getTimeInstance(int style, Locale locale): This method is used to gets time formatter with the specified formatting style for the given locale.
- TimeZone getTimeZone(): This method is used to gets an object of TimeZone for this DateFormat instance.
- static final DateFormat getDateInstance(): This method is used to gets date formatter with default formatting style for the default locale.
- static final DateFormat getDateInstance(int style): This method is used to gets date formatter with the specified formatting style for the default locale.
- static final DateFormat getDateInstance(int style, Locale locale): This method is used to gets date formatter with the specified formatting style for the given locale.
- static final DateFormat getDateTimeInstance(): This method is used to gets date or time formatter with default formatting style for the default locale.
- NumberFormat getNumberFormat(): This method is used to gets an object of NumberFormat for this DateFormat instance.
- Calendar getCalendar(): This method is used to gets an object of the Calendar for this DateFormat instance.
Examples of Internationalization in Java
Given below are the examples mentioned:
Example #1
Example for the internationalization in Java to create different country locale.
Code:
// The program can be tested in Eclipse IDE, JAVA 11 package jex; import java.util.Locale; public class ex { public static void main(String[] args) { Locale[] locales = { new Locale("en", "US"), new Locale("it", "IT"), new Locale("es", "ES") }; for (int l=0; l< locales.length; l++) { String Language = locales[l].getDisplayLanguage(locales[l]); System.out.println(locales[l].toString() + ": " + Language); } } }
Output:
As in the above program, the Locale class objects are created and store in the array. Next, used the for loop to iterate each locale object and display its name and its language, as we can see in the above output.
Example #2
Example for the internationalization in Java to show the number in different formats for the different countries.
Code:
// The program can be tested in Eclipse IDE, JAVA 11 package jex; import java.util.*; import java.text.*; public class ex { public static void main (String[]args) { double n = 45273.8956; NumberFormat f1 = NumberFormat.getInstance (Locale.US); NumberFormat f2 = NumberFormat.getInstance (Locale.ITALY); NumberFormat f3 = NumberFormat.getInstance (Locale.CHINA); System.out.println ("The number format in US is :" + f1.format (n)); System.out.println ("The number format in ITALY is:" + f2.format (n)); System.out.println ("The number format in CHINA is :" + f3.format (n)); } }
Output:
As in the above program, three different NumberFormat class objects are created using the Locale class. Next, using the format() method of the NumberFormat class, the given number is printing in the specific format of the country, as we can see in the above output.
Example #3
Example for the internationalization in Java to show the date in different formats for the different countries.
Code:
// The program can be tested in Eclipse IDE, JAVA 11 package jex; import java.text.DateFormat; import java.util.Date; import java.util.Locale; public class ex { public static void main (String[]args) { DateFormat d1 = DateFormat.getDateInstance (0, Locale.US); DateFormat d2 = DateFormat.getDateInstance (0, Locale.ITALY); DateFormat d3 = DateFormat.getDateInstance (0, Locale.CHINA); System.out.println ("The date format in US is :" + d1.format (new Date ())); System.out.println ("The date format in ITALY is : " + d2.format (new Date ())); System.out.println ("The date format in CHINA is : " + d3.format (new Date ())); } }
Output:
As in the above program, three different DateFormat class objects are created using the Locale class. Next, using the format() method of the DateFormat class, the return date of the Date() method is printing in the specific format of the country, as we can see in the above output.
Conclusion
Internationalization is also called I18N because there are 18 characters between the letters I and N. It is the process of creating web applications in such a way that they automatically support several countries, languages, and currencies without requiring any changes to the application.
以上がJava の国際化の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










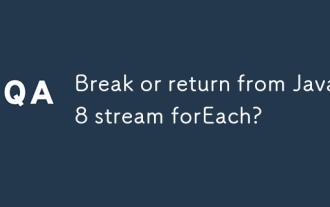
Java 8は、Stream APIを導入し、データ収集を処理する強力で表現力のある方法を提供します。ただし、ストリームを使用する際の一般的な質問は次のとおりです。 従来のループにより、早期の中断やリターンが可能になりますが、StreamのForeachメソッドはこの方法を直接サポートしていません。この記事では、理由を説明し、ストリーム処理システムに早期終了を実装するための代替方法を調査します。 さらに読み取り:JavaストリームAPIの改善 ストリームを理解してください Foreachメソッドは、ストリーム内の各要素で1つの操作を実行する端末操作です。その設計意図はです
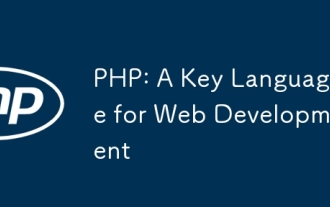
PHPは、サーバー側で広く使用されているスクリプト言語で、特にWeb開発に適しています。 1.PHPは、HTMLを埋め込み、HTTP要求と応答を処理し、さまざまなデータベースをサポートできます。 2.PHPは、ダイナミックWebコンテンツ、プロセスフォームデータ、アクセスデータベースなどを生成するために使用され、強力なコミュニティサポートとオープンソースリソースを備えています。 3。PHPは解釈された言語であり、実行プロセスには語彙分析、文法分析、編集、実行が含まれます。 4.PHPは、ユーザー登録システムなどの高度なアプリケーションについてMySQLと組み合わせることができます。 5。PHPをデバッグするときは、error_reporting()やvar_dump()などの関数を使用できます。 6. PHPコードを最適化して、キャッシュメカニズムを使用し、データベースクエリを最適化し、組み込み関数を使用します。 7
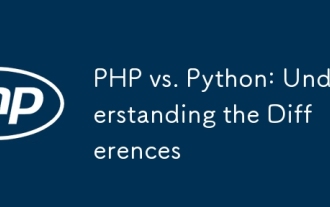
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHPは、シンプルな構文と高い実行効率を備えたWeb開発に適しています。 2。Pythonは、簡潔な構文とリッチライブラリを備えたデータサイエンスと機械学習に適しています。
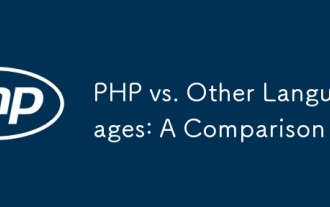
PHPは、特に迅速な開発や動的なコンテンツの処理に適していますが、データサイエンスとエンタープライズレベルのアプリケーションには良くありません。 Pythonと比較して、PHPはWeb開発においてより多くの利点がありますが、データサイエンスの分野ではPythonほど良くありません。 Javaと比較して、PHPはエンタープライズレベルのアプリケーションでより悪化しますが、Web開発により柔軟性があります。 JavaScriptと比較して、PHPはバックエンド開発により簡潔ですが、フロントエンド開発のJavaScriptほど良くありません。
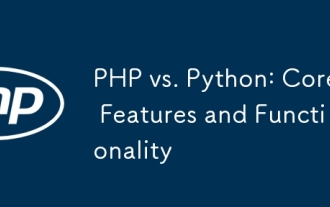
PHPとPythonにはそれぞれ独自の利点があり、さまざまなシナリオに適しています。 1.PHPはWeb開発に適しており、組み込みのWebサーバーとRich Functionライブラリを提供します。 2。Pythonは、簡潔な構文と強力な標準ライブラリを備えたデータサイエンスと機械学習に適しています。選択するときは、プロジェクトの要件に基づいて決定する必要があります。
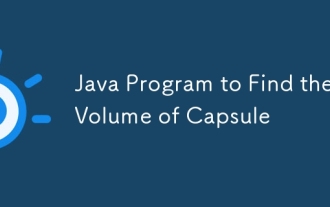
カプセルは3次元の幾何学的図形で、両端にシリンダーと半球で構成されています。カプセルの体積は、シリンダーの体積と両端に半球の体積を追加することで計算できます。このチュートリアルでは、さまざまな方法を使用して、Javaの特定のカプセルの体積を計算する方法について説明します。 カプセルボリュームフォーミュラ カプセルボリュームの式は次のとおりです。 カプセル体積=円筒形の体積2つの半球体積 で、 R:半球の半径。 H:シリンダーの高さ(半球を除く)。 例1 入力 RADIUS = 5ユニット 高さ= 10単位 出力 ボリューム= 1570.8立方ユニット 説明する 式を使用してボリュームを計算します。 ボリューム=π×R2×H(4
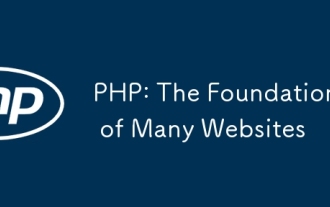
PHPが多くのWebサイトよりも優先テクノロジースタックである理由には、その使いやすさ、強力なコミュニティサポート、広範な使用が含まれます。 1)初心者に適した学習と使用が簡単です。 2)巨大な開発者コミュニティと豊富なリソースを持っています。 3)WordPress、Drupal、その他のプラットフォームで広く使用されています。 4)Webサーバーとしっかりと統合して、開発の展開を簡素化します。
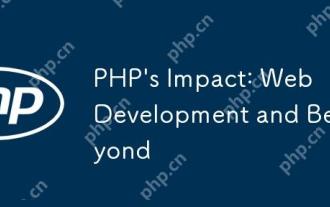
phphassiblasifly-impactedwebdevevermentandsbeyondit.1)itpowersmajorplatformslikewordpratsandexcelsindatabase interactions.2)php'sadaptableability allowsitale forlargeapplicationsusingframeworkslikelavel.3)
