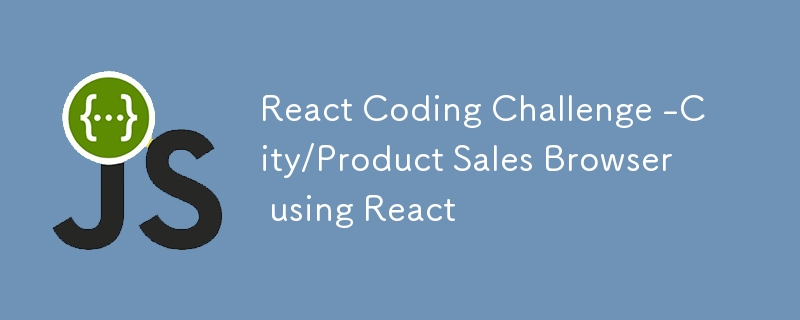
課題: React を使用した都市/物販ブラウザ
会社名: Cytel
結果 - 次のラウンドに選出
客観的
ルーティングと動的なデータ取得を使用して都市と製品の販売情報を表示するシンプルな React アプリケーションを開発します。このアプリでは、ユーザーが異なるページ間を移動できるようにする必要があり、訪問した都市と商品の数を追跡する必要があります。データはユーザーのリクエストに応じて更新する必要があります。
要件
-
アプリケーションの概要:
- ページの上部に 2 つのカウンターを表示します。
- カウンターをリセットしてデータを再ロードする 更新 ボタンを提供します。
-
ホームページ:
-
URL: /
- 2 つのリストを表示します:
- API から取得した都市名のリスト。
- API から取得した製品名のリスト。
- アプリの起動時に、訪問都市 と 訪問製品 カウンターを 0 に初期化します。
-
API エンドポイント:
-
すべての都市を取得するには: https://assessments.reliscore.com/api/cities
-
すべての製品を取得するには: https://assessments.reliscore.com/api/sales/products
-
都市の詳細ページ:
-
URL: /api/sales//
- ホームページで都市名をクリックすると、都市固有のページに移動します。
- 次のエンドポイントを使用して、選択した都市で販売された製品名と数量のリストを取得して表示します。
https://assessments.reliscore.com/api/sales/<都市名>/
( を実際の都市名に置き換えます。)
- 都市ページが訪問されるたびに、訪問都市カウンターを 1 つ増やします。
-
商品詳細ページ:
-
URL: /sales/product//
- 任意のページで製品名をクリックすると、製品固有のページに移動します。
- 次のエンドポイントを使用して、製品が販売されたすべての都市名と各都市での販売金額のリストを取得して表示します。
https://assessments.reliscore.com/api/sales/product/<製品名>/
( を実際の製品名に置き換えます。)
- 商品ページが訪問されるたびに、Products Visited カウンタを 1 増やします。
-
更新ボタン:
- 任意のページで 更新 ボタンをクリックすると、次のことが行われます。
- それぞれの API からすべてのデータを再読み込みします。
-
訪問都市 と 訪問製品 カウンターを 0 にリセットします。
-
ルーティング付きシングル ページ アプリケーション (SPA):
- React を使用してアプリケーションをシングルページ アプリケーション (SPA) として実装します。
- ルーティングを利用して、ブラウザの「戻る」ボタンと「進む」ボタンが正しく機能することを確認しながら、異なるページ (都市の詳細、製品の詳細など) 間のナビゲーションを処理します。
- ユーザーが適切な URL を使用して任意のページに直接移動できるようにします。
-
評価基準:
- 再利用可能な React コンポーネントの適切な使用。
- フックや状態管理などの React 機能の効率的な使用。
- さまざまなページをシミュレートするためのルーティングの適切な実装。
- コード構造とモジュール性におけるベスト プラクティスの遵守。
成果物
- 上記の要件を満たす完全に機能する React アプリケーション。
- プロジェクトは構造化され、十分なコメントが付けられ、ナビゲートしやすいものである必要があります。
- データの欠落や不正な URL などの特殊なケースにアプリケーションが対処できるようにします。
注意事項
- アプリケーションを徹底的にテストして、すべての機能が期待どおりに動作することを確認します。
- アプリの応答性が高く、さまざまな画面サイズでも適切に動作することを確認してください。
API レスポンスの概要
-
都市 API 応答:
-
エンドポイント: https://assessments.reliscore.com/api/cities
-
応答形式:
{
"status": "success",
"data": [
"Bombay",
"Bangalore",
"Pune",
"Kolkata",
"Chennai",
"New Delhi"
]
}
ログイン後にコピー
説明: この API は、販売データが利用可能な都市名のリストを返します。データ配列にはこれらの都市の名前が含まれています。
-
特定の都市の売上データ:
-
Endpoint: https://assessments.reliscore.com/api/sales/pune (Replace pune with any other city name as needed)
-
Response Format:
{
"status": "success",
"data": {
"product1": 137,
"product2": 23,
"product3": 77
}
}
ログイン後にコピー
ログイン後にコピー
ログイン後にコピー
Description: This API returns sales data for a specific city. The data object contains key-value pairs where the key is the product name and the value is the number of items sold in that city.
-
Products List API Response:
-
Endpoint: https://assessments.reliscore.com/api/sales/products
-
Response Format:
{
"status": "success",
"data": {
"product1": 137,
"product2": 23,
"product3": 77
}
}
ログイン後にコピー
ログイン後にコピー
ログイン後にコピー
Description: This API returns a list of all products with their total sales figures. The data object contains key-value pairs where the key is the product name and the value is the total number of items sold across all cities.
-
Product Detail API Response:
-
Endpoint: https://assessments.reliscore.com/api/sales/product/product1 (Replace product1 with any other product name as needed)
-
Response Format:
{
"status": "success",
"data": {
"product1": 137,
"product2": 23,
"product3": 77
}
}
ログイン後にコピー
ログイン後にコピー
ログイン後にコピー
Description: This API returns the sales data for a specific product across different cities. The data object contains key-value pairs where the key is the city name and the value is the number of items sold for that product in that city.
Note:
Please ensure that you fully understand the requirements before starting the implementation. There’s a minor issue with the API response for the product/ endpoint, but it can be worked around with the provided data. Adding the API responses for reference above.
You are encouraged to implement the solution and make any necessary modifications to the APIs as needed to meet the requirements. If you need more details or are interested in similar assignments, you can refer to my E-Commerce Project.
以上がReact コーディング チャレンジ - React を使用した都市/物販ブラウザの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。