Django リクエストのライフサイクルの説明
Web 開発の世界では、リクエストのライフサイクルを理解することは、パフォーマンスの最適化、問題のデバッグ、および堅牢なアプリケーションの構築にとって非常に重要です。人気の Python Web フレームワークである Django では、リクエストのライフサイクルは、サーバーがリクエストを受信した瞬間から、レスポンスがクライアントに送り返されるまで、リクエストが通過する一連のステップを明確に定義したものです。
このブログ記事では、Django リクエストのライフサイクルの広範な調査が提供されています。手順の各段階を説明し、コード サンプルを提供し、Django アプリのパフォーマンスを調整および改善する方法に関するヒントとアドバイスを提供します。この投稿を最後まで読むと、Django のリクエストとレスポンスの処理について完全に理解できるようになります。
- Django リクエストのライフサイクルの概要
リクエストのライフサイクルの詳細に入る前に、Web 開発のコンテキストにおけるリクエストが何であるかを理解することが重要です。リクエストは、クライアント (通常は Web ブラウザ) からサーバーに送信され、特定のリソースまたはアクションを要求する HTTP メッセージです。サーバーはリクエストを処理し、Web ページ、画像、JSON 形式のデータなどの HTTP レスポンスを送り返します。
Django は高レベルの Python Web フレームワークであり、HTTP リクエストとレスポンスの処理の複雑さの多くを抽象化します。ただし、Django がこれらのリクエストを処理する方法の基本的な仕組みを理解することは、フレームワークの能力を最大限に活用したい開発者にとって非常に貴重です。
- Django リクエストの構造
本質的に、Django リクエストは HttpRequest クラスのインスタンスです。サーバーがリクエストを受信すると、Django はリクエストに関するメタデータを含む HttpRequest オブジェクトを作成します。
メソッド: 使用される HTTP メソッド (GET、POST、PUT、DELETE など)。
パス: リクエストの URL パス。
ヘッダー: User-Agent、Host などの HTTP ヘッダーを含む辞書
本文: リクエストの本文。フォーム データ、JSON ペイロードなどが含まれる場合があります。
Django ビューでこれらのプロパティの一部にアクセスする簡単な例を次に示します。
from django.http import HttpResponse def example_view(request): method = request.method path = request.path user_agent = request.headers.get('User-Agent', '') response_content = f"Method: {method}, Path: {path}, User-Agent: {user_agent}" return HttpResponse(response_content)
この例では、example_view は、リクエストから HTTP メソッド、パス、ユーザー エージェントを抽出し、それらをレスポンスで返す基本的な Django ビューです。
- Django リクエストのライフサイクルの段階的な内訳
Django リクエストのライフサイクルの各ステップを詳しく見てみましょう:
ステップ 1: URL ルーティング
リクエストが Django サーバーに到着すると、最初のステップは URL ルーティングです。 Django は、URL ディスパッチャを使用して、受信リクエストのパスを urls.py ファイルで定義された事前定義された URL パターンのリストと照合します。
# urls.py from django.urls import path from .views import example_view urlpatterns = [ path('example/', example_view, name='example'), ]
この例では、パス /example/ を持つリクエストは example_view 関数にルーティングされます。
Django は一致する URL パターンを見つけると、関連付けられたビュー関数を呼び出します。一致するものが見つからない場合、Django は 404 Not Found 応答を返します。
ステップ 2: ミドルウェア処理
ビューが実行される前に、Django は一連のミドルウェアを通じてリクエストを処理します。ミドルウェアは、開発者がリクエストと応答をグローバルに処理できるようにするフックです。これらは、認証、ログ記録、リクエスト/レスポンスの変更など、さまざまな目的に使用できます。
リクエストのメソッドとパスを記録するカスタム ミドルウェアの例を次に示します。
# middleware.py class LogRequestMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): # Process the request print(f"Request Method: {request.method}, Path: {request.path}") response = self.get_response(request) # Process the response return response
このミドルウェアを使用するには、settings.py ファイルの MIDDLEWARE リストに追加します。
# settings.py MIDDLEWARE = [ 'django.middleware.security.SecurityMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', # Add your custom middleware here 'myapp.middleware.LogRequestMiddleware', 'django.middleware.common.CommonMiddleware', 'django.middleware.csrf.CsrfViewMiddleware', 'django.contrib.auth.middleware.AuthenticationMiddleware', 'django.contrib.messages.middleware.MessageMiddleware', 'django.middleware.clickjacking.XFrameOptionsMiddleware', ]
ミドルウェアは、MIDDLEWARE リストにリストされている順序で処理されます。リクエストはビューに到達するまで、リスト内の各ミドルウェアを通過します。
ステップ 3: 実行の表示
リクエストがすべてのミドルウェアを通過すると、Django は一致した URL パターンに関連付けられたビューを呼び出します。ビューは、アプリケーションのコア ロジックが存在する場所です。リクエストを処理し、モデルやデータベースと対話し、応答を返す責任があります。
データベースと対話する Django ビューの例を次に示します。
# views.py from django.shortcuts import render from .models import Product def product_list(request): products = Product.objects.all() return render(request, 'product_list.html', {'products': products})
この例では、product_list ビューが Product モデルにクエリを実行してデータベースからすべての製品を取得し、それらをレンダリングのために product_list.html テンプレートに渡します。
ステップ 4: テンプレートのレンダリング
ビューが HttpResponse オブジェクトを直接返す場合、Django はテンプレートのレンダリング手順をスキップします。ただし、ビューがコンテキスト データの辞書を返す場合、Django はテンプレート エンジンを使用して HTML 応答をレンダリングします。
簡単な Django テンプレートの例を次に示します:
<!-- templates/product_list.html --> <!DOCTYPE html> <html> <head> <title>Product List</title> </head> <body> <h1>Products</h1> <ul> {% for product in products %} <li>{{ product.name }} - ${{ product.price }}</li> {% endfor %} </ul> </body> </html>
この例では、product_list.html テンプレートが products コンテキスト変数をループし、各製品の名前と価格を順序なしリストでレンダリングします。
ステップ 5: 応答の生成
After the view has processed the request and rendered the template (if applicable), Django generates an HttpResponse object. This object contains the HTTP status code, headers, and content of the response.
Here's an example of manually creating an HttpResponse object:
from django.http import HttpResponse def custom_response_view(request): response = HttpResponse("Hello, Django!") response.status_code = 200 response['Content-Type'] = 'text/plain' return response
In this example, the custom_response_view function returns a plain text response with a status code of 200 (OK).
Step 6: Middleware Response Processing
Before the response is sent back to the client, it passes through the middleware again. This time, Django processes the response through any middleware that has a process_response method.
This is useful for tasks such as setting cookies, compressing content, or adding custom headers. Here’s an example of a middleware that adds a custom header to the response:
# middleware.py class CustomHeaderMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): response = self.get_response(request) response['X-Custom-Header'] = 'MyCustomHeaderValue' return response
Step 7: Sending the Response
Finally, after all middleware processing is complete, Django sends the HttpResponse object back to the client. The client receives the response and renders the content (if it’s a web page) or processes it further (if it’s an API response).
- Advanced Topics in Django Request Handling
Now that we’ve covered the basics of the Django request life cycle, let's explore some advanced topics:
4.1 Custom Middleware
Creating custom middleware allows you to hook into the request/response life cycle and add custom functionality globally. Here’s an example of a middleware that checks for a custom header and rejects requests that do not include it:
# middleware.py from django.http import HttpResponseForbidden class RequireCustomHeaderMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): if 'X-Required-Header' not in request.headers: return HttpResponseForbidden("Forbidden: Missing required header") response = self.get_response(request) return response
4.2 Request and Response Objects
Django's HttpRequest and HttpResponse objects are highly customizable. You can subclass these objects to add custom behavior. Here’s an example of a custom request class that adds a method for checking if the request is coming from a mobile device:
# custom_request.py from django.http import HttpRequest class CustomHttpRequest(HttpRequest): def is_mobile(self): user_agent = self.headers.get('User-Agent', '').lower() return 'mobile' in user_agent
To use this custom request class, you need to set it in the settings.py file:
# settings.py MIDDLEWARE = [ 'django.middleware.security.SecurityMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', 'django.middleware.common.Common Middleware', # Use your custom request class 'myapp.custom_request.CustomHttpRequest', 'django.middleware.csrf.CsrfViewMiddleware', 'django.contrib.auth.middleware.AuthenticationMiddleware', 'django.contrib.messages.middleware.MessageMiddleware', 'django.middleware.clickjacking.XFrameOptionsMiddleware', ]
4.3 Optimizing the Request Life Cycle
Optimizing the request life cycle can significantly improve your Django application's performance. Here are some tips:
Use Caching: Caching can drastically reduce the load on your server by storing frequently accessed data in memory. Django provides a robust caching framework that supports multiple backends, such as Memcached and Redis.
# views.py from django.views.decorators.cache import cache_page @cache_page(60 * 15) # Cache the view for 15 minutes def my_view(request): # View logic here return HttpResponse("Hello, Django!")
Minimize Database Queries: Use Django’s select_related and prefetch_related methods to minimize the number of database queries.
# views.py from django.shortcuts import render from .models import Author def author_list(request): # Use select_related to reduce database queries authors = Author.objects.select_related('profile').all() return render(request, 'author_list.html', {'authors': authors})
Leverage Middleware for Global Changes: Instead of modifying each view individually, use middleware to make global changes. This can include setting security headers, handling exceptions, or modifying the request/response.
Asynchronous Views: Starting with Django 3.1, you can write asynchronous views to handle requests asynchronously. This can improve performance for I/O-bound tasks such as making external API calls or processing large files.
# views.py from django.http import JsonResponse import asyncio async def async_view(request): await asyncio.sleep(1) # Simulate a long-running task return JsonResponse({'message': 'Hello, Django!'})
- Conclusion
Understanding the Django request life cycle is fundamental for any Django developer. By knowing how requests are processed, you can write more efficient, maintainable, and scalable applications. This guide has walked you through each step of the request life cycle, from URL routing to sending the response, and provided code examples and tips for optimizing your Django applications.
By leveraging the power of Django’s middleware, request and response objects, and caching framework, you can build robust web applications that perform well under load and provide a great user experience.
References
Django Documentation: https://docs.djangoproject.com/en/stable/
Django Middleware: https://docs.djangoproject.com/en/stable/topics/http/middleware/
Django Views: https://docs.djangoproject.com/en/stable/topics/http/views/
Django Templates: https://docs.djangoproject.com/en/stable/topics/templates/
Django Caching: https://docs.djangoproject.com/en/stable/topics/cache/
以上がDjango リクエストのライフサイクルの説明の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










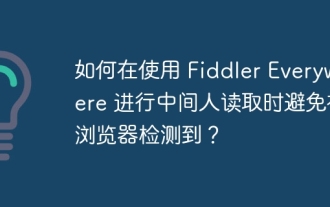
fiddlereveryversings for the-middleの測定値を使用するときに検出されないようにする方法
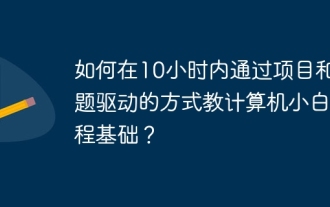
10時間以内にコンピューター初心者プログラミングの基本を教える方法は?コンピューター初心者にプログラミングの知識を教えるのに10時間しかない場合、何を教えることを選びますか...
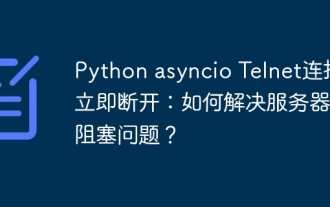
Pythonasyncioについて...
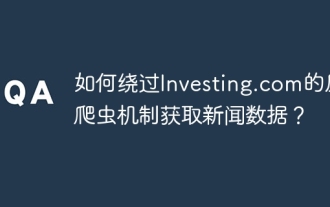
Investing.comの反クラウリング戦略を理解する多くの人々は、Investing.com(https://cn.investing.com/news/latest-news)からのニュースデータをクロールしようとします。
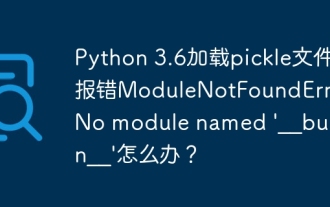
Python 3.6のピクルスファイルの読み込みエラー:modulenotfounderror:nomodulenamed ...
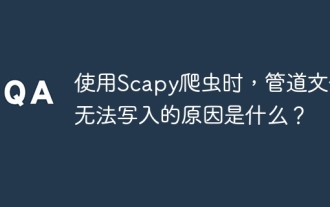
SCAPYクローラーを使用するときにパイプラインファイルを作成できない理由についての議論は、SCAPYクローラーを学習して永続的なデータストレージに使用するときに、パイプラインファイルに遭遇する可能性があります...
