Firebase を使用した Chrome 拡張機能での Google 認証
Google の公式ガイドにはいくつかの重要な手順が欠けているため、このガイドを作成しています。以下にリンクしています。
Chrome 拡張機能で Firebase を使用して認証する
これはどのオペレーティング システムでも動作します。このガイドでは Mac OS
を使用します。前提条件
- Google Chrome ブラウザ
- Google アカウント
- Chrome ウェブストア デベロッパー アカウント (1 回限りの料金 5 ドル)
- Node.js と npm がインストールされています
ステップ 1: プロジェクト構造を作成する
a) プロジェクトの新しいディレクトリを作成します。
mkdir firebase-chrome-auth cd firebase-chrome-auth
b) 2 つのサブディレクトリを作成します:
mkdir chrome-extension mkdir firebase-project
ステップ 2: Firebase プロジェクトをセットアップする
a) Firebase コンソールに移動します。
b) [プロジェクトを追加] をクリックし、手順に従って新しいプロジェクトを作成します。
c) 作成したら、[Web] をクリックして Web アプリをプロジェクトに追加します。
d) ニックネーム (例: 「Chrome Extension Auth」) を使用してアプリを登録します。
e) Firebase 構成オブジェクトをコピーします。これは後で必要になります。
const firebaseConfig = { apiKey: "example", authDomain: "example.firebaseapp.com", projectId: "example", storageBucket: "example", messagingSenderId: "example", appId: "example" };
f) firebase-project ディレクトリに移動します
cd firebase-プロジェクト
g) 新しい npm プロジェクトを初期化します
npm init -y
h) Firebase をインストールします:
npm install firebase
i) firebase-project/index.html
にindex.html ファイルを作成します。
<!DOCTYPE html> <html> <head> <title>Firebase Auth for Chrome Extension</title> </head> <body> <h1>Firebase Auth for Chrome Extension</h1> <script type="module" src="signInWithPopup.js"></script> </body> </html>
j) firebase-project/signInWithPopup.js
にsignInWithPopup.js ファイルを作成します。
import { initializeApp } from 'firebase/app'; import { getAuth, signInWithPopup, GoogleAuthProvider } from 'firebase/auth'; const firebaseConfig = { // Your web app's Firebase configuration // Replace with the config you copied from Firebase Console }; const app = initializeApp(firebaseConfig); const auth = getAuth(); // This gives you a reference to the parent frame, i.e. the offscreen document. const PARENT_FRAME = document.location.ancestorOrigins[0]; const PROVIDER = new GoogleAuthProvider(); function sendResponse(result) { window.parent.postMessage(JSON.stringify(result), PARENT_FRAME); } window.addEventListener('message', function({data}) { if (data.initAuth) { signInWithPopup(auth, PROVIDER) .then(sendResponse) .catch(sendResponse); } });
k) Firebase プロジェクトをデプロイします
npm install -g firebase-tools firebase login firebase init hosting firebase deploy
展開後に提供されるホスティング URL に注目してください。これは Chrome 拡張機能に必要です。
ステップ 3: Chrome 拡張機能をセットアップする
a) chrome-extension ディレクトリに移動します
cd ../chrome-extension
b) chrome-extension/manifest.json
にmanifest.json ファイルを作成します。
{ "manifest_version": 3, "name": "Firebase Auth Extension", "version": "1.0", "description": "Chrome extension with Firebase Authentication", "permissions": [ "identity", "storage", "offscreen" ], "host_permissions": [ "https://*.firebaseapp.com/*" ], "background": { "service_worker": "background.js", "type": "module" }, "action": { "default_popup": "popup.html" }, "web_accessible_resources": [ { "resources": ["offscreen.html"], "matches": ["<all_urls>"] } ], "oauth2": { "client_id": "YOUR-ID.apps.googleusercontent.com", "scopes": [ "openid", "email", "profile" ] }, "key": "-----BEGIN PUBLIC KEY-----\nYOURPUBLICKEY\n-----END PUBLIC KEY-----" }
c) chrome-extension/popup.html
に Popup.html ファイルを作成します。
<!DOCTYPE html> <html> <head> <title>Firebase Auth Extension</title> </head> <body> <h1>Firebase Auth Extension</h1> <div id="userInfo"></div> <button id="signInButton">Sign In</button> <button id="signOutButton" style="display:none;">Sign Out</button> <script src="popup.js"></script> </body> </html>
d) chrome-extension/popup.js
に Popup.js ファイルを作成します。
document.addEventListener('DOMContentLoaded', function() { const signInButton = document.getElementById('signInButton'); const signOutButton = document.getElementById('signOutButton'); const userInfo = document.getElementById('userInfo'); function updateUI(user) { if (user) { userInfo.textContent = `Signed in as: ${user.email}`; signInButton.style.display = 'none'; signOutButton.style.display = 'block'; } else { userInfo.textContent = 'Not signed in'; signInButton.style.display = 'block'; signOutButton.style.display = 'none'; } } chrome.storage.local.get(['user'], function(result) { updateUI(result.user); }); signInButton.addEventListener('click', function() { chrome.runtime.sendMessage({action: 'signIn'}, function(response) { if (response.user) { updateUI(response.user); } }); }); signOutButton.addEventListener('click', function() { chrome.runtime.sendMessage({action: 'signOut'}, function() { updateUI(null); }); }); });
e) chrome-extension/background.js
にbackground.js ファイルを作成します。
const OFFSCREEN_DOCUMENT_PATH = 'offscreen.html'; const FIREBASE_HOSTING_URL = 'https://your-project-id.web.app'; // Replace with your Firebase hosting URL let creatingOffscreenDocument; async function hasOffscreenDocument() { const matchedClients = await clients.matchAll(); return matchedClients.some((client) => client.url.endsWith(OFFSCREEN_DOCUMENT_PATH)); } async function setupOffscreenDocument() { if (await hasOffscreenDocument()) return; if (creatingOffscreenDocument) { await creatingOffscreenDocument; } else { creatingOffscreenDocument = chrome.offscreen.createDocument({ url: OFFSCREEN_DOCUMENT_PATH, reasons: [chrome.offscreen.Reason.DOM_SCRAPING], justification: 'Firebase Authentication' }); await creatingOffscreenDocument; creatingOffscreenDocument = null; } } async function getAuthFromOffscreen() { await setupOffscreenDocument(); return new Promise((resolve, reject) => { chrome.runtime.sendMessage({action: 'getAuth', target: 'offscreen'}, (response) => { if (chrome.runtime.lastError) { reject(chrome.runtime.lastError); } else { resolve(response); } }); }); } chrome.runtime.onMessage.addListener((message, sender, sendResponse) => { if (message.action === 'signIn') { getAuthFromOffscreen() .then(user => { chrome.storage.local.set({user: user}, () => { sendResponse({user: user}); }); }) .catch(error => { console.error('Authentication error:', error); sendResponse({error: error.message}); }); return true; // Indicates we will send a response asynchronously } else if (message.action === 'signOut') { chrome.storage.local.remove('user', () => { sendResponse(); }); return true; } });
f) chrome-extension/offscreen.html
に offscreen.html ファイルを作成します。
<!DOCTYPE html> <html> <head> <title>Offscreen Document</title> </head> <body> <script src="offscreen.js"></script> </body> </html>
g) _chrome-extension/offscreen.js
に offscreen.js ファイルを作成します。
_
const FIREBASE_HOSTING_URL = 'https://your-project-id.web.app'; // Replace with your Firebase hosting URL const iframe = document.createElement('iframe'); iframe.src = FIREBASE_HOSTING_URL; document.body.appendChild(iframe); chrome.runtime.onMessage.addListener((message, sender, sendResponse) => { if (message.action === 'getAuth' && message.target === 'offscreen') { function handleIframeMessage({data}) { try { const parsedData = JSON.parse(data); window.removeEventListener('message', handleIframeMessage); sendResponse(parsedData.user); } catch (e) { console.error('Error parsing iframe message:', e); } } window.addEventListener('message', handleIframeMessage); iframe.contentWindow.postMessage({initAuth: true}, FIREBASE_HOSTING_URL); return true; // Indicates we will send a response asynchronously } });
ステップ 4: Firebase 認証を構成する
a) Firebase コンソールで、[認証] > [認証] に移動します。サインイン方法。
b) Google をサインイン プロバイダーとして有効にします。
c) Chrome 拡張機能の ID を承認済みドメイン リストに追加します:
形式は次のとおりです: chrome-extension://YOUR_EXTENSION_ID
拡張機能 ID は、解凍された拡張機能として読み込んだ後、Chrome の拡張機能管理ページで確認できます。
ステップ 5: 拡張機能をロードしてテストする
a) Google Chrome を開き、chrome://extensions/ に移動します。
b) 右上隅の「開発者モード」を有効にします。
c) [解凍してロード] をクリックし、Chrome 拡張機能のディレクトリを選択します。
d) Chrome のツールバーの拡張機能アイコンをクリックしてポップアップを開きます。
e) [サインイン] ボタンをクリックし、認証フローをテストします。
トラブルシューティング
CORS の問題が発生した場合は、Firebase ホスティング URL がbackground.js と offscreen.js の両方で正しく設定されていることを確認してください。
Chrome 拡張機能の ID が Firebase の承認済みドメインに正しく追加されていることを確認してください。
ポップアップ、バックグラウンド スクリプト、オフスクリーン ドキュメントのコンソール ログでエラー メッセージがないか確認してください。
結論
これで、オフスクリーン ドキュメントで Firebase Authentication を使用してサインイン プロセスを処理する Chrome 拡張機能が完成しました。この設定により、機密性の高い Firebase 設定の詳細を拡張コード内で直接公開することなく、安全な認証が可能になります。
拡張機能を公開する前に、プレースホルダーの値 (YOUR_EXTENSION_ID、YOUR-CLIENT-ID、YOUR_PUBLIC_KEY、your-project-id など) を必ず実際の値に置き換えてください。
以上がFirebase を使用した Chrome 拡張機能での Google 認証の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










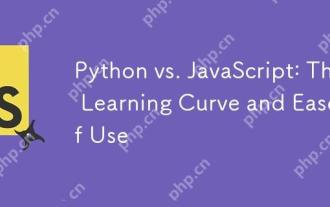
Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。
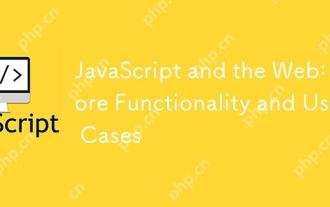
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
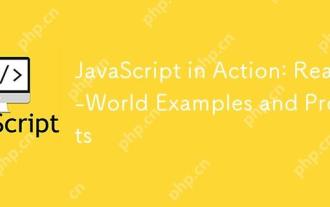
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
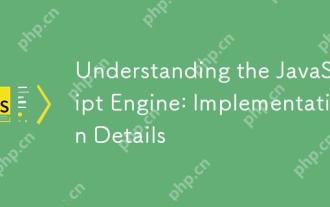
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。
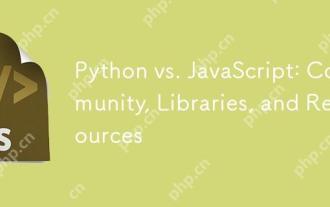
PythonとJavaScriptには、コミュニティ、ライブラリ、リソースの観点から、独自の利点と短所があります。 1)Pythonコミュニティはフレンドリーで初心者に適していますが、フロントエンドの開発リソースはJavaScriptほど豊富ではありません。 2)Pythonはデータサイエンスおよび機械学習ライブラリで強力ですが、JavaScriptはフロントエンド開発ライブラリとフレームワークで優れています。 3)どちらも豊富な学習リソースを持っていますが、Pythonは公式文書から始めるのに適していますが、JavaScriptはMDNWebDocsにより優れています。選択は、プロジェクトのニーズと個人的な関心に基づいている必要があります。
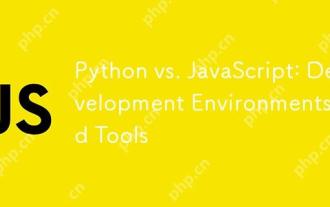
開発環境におけるPythonとJavaScriptの両方の選択が重要です。 1)Pythonの開発環境には、Pycharm、Jupyternotebook、Anacondaが含まれます。これらは、データサイエンスと迅速なプロトタイピングに適しています。 2)JavaScriptの開発環境には、フロントエンドおよびバックエンド開発に適したnode.js、vscode、およびwebpackが含まれます。プロジェクトのニーズに応じて適切なツールを選択すると、開発効率とプロジェクトの成功率が向上する可能性があります。
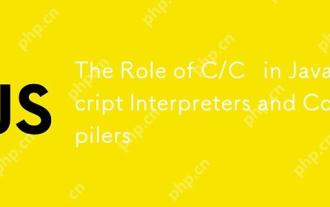
CとCは、主に通訳者とJITコンパイラを実装するために使用されるJavaScriptエンジンで重要な役割を果たします。 1)cは、JavaScriptソースコードを解析し、抽象的な構文ツリーを生成するために使用されます。 2)Cは、Bytecodeの生成と実行を担当します。 3)Cは、JITコンパイラを実装し、実行時にホットスポットコードを最適化およびコンパイルし、JavaScriptの実行効率を大幅に改善します。

Pythonはデータサイエンスと自動化により適していますが、JavaScriptはフロントエンドとフルスタックの開発により適しています。 1. Pythonは、データ処理とモデリングのためにNumpyやPandasなどのライブラリを使用して、データサイエンスと機械学習でうまく機能します。 2。Pythonは、自動化とスクリプトにおいて簡潔で効率的です。 3. JavaScriptはフロントエンド開発に不可欠であり、動的なWebページと単一ページアプリケーションの構築に使用されます。 4. JavaScriptは、node.jsを通じてバックエンド開発において役割を果たし、フルスタック開発をサポートします。
