CakePHP ルーティング
この章では、ルーティングに関連する次のトピックについて学習します -
- ルーティングの概要
- 接続ルート
- 引数をルートに渡す
- URL を生成しています
- リダイレクト URL
ルーティングの概要
このセクションでは、ルートを実装する方法、URL からコントローラーのアクションに引数を渡す方法、URL を生成する方法、特定の URL にリダイレクトする方法について説明します。通常、ルートはファイル config/routes.php に実装されます。ルーティングは 2 つの方法で実装できます -
- 静的メソッド
- スコープ付きルートビルダー
ここでは、両方のタイプを示す例を示します。
// Using the scoped route builder. Router::scope('/', function ($routes) { $routes->connect('/', ['controller' => 'Articles', 'action' => 'index']); }); // Using the static method. Router::connect('/', ['controller' => 'Articles', 'action' => 'index']);
どちらのメソッドも ArticlesController のインデックス メソッドを実行します。 2 つの方法のうち、スコープ ルート ビルダー の方がパフォーマンスが優れています。
接続ルート
Router::connect() メソッドはルートの接続に使用されます。以下はメソッドの構文です -
static Cake\Routing\Router::connect($route, $defaults =[], $options =[])
Router::connect() メソッドには 3 つの引数があります -
最初の引数は、照合する URL テンプレートです。
2 番目の引数には、ルート要素のデフォルト値が含まれます。
3 番目の引数にはルートのオプションが含まれており、通常は正規表現ルールが含まれます。
これはルートの基本的な形式です -
$routes->connect( 'URL template', ['default' => 'defaultValue'], ['option' => 'matchingRegex'] );
例
以下に示すように、config/routes.php ファイルを変更します。
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('/', ['controller' => 'Tests', 'action' => 'show']); $builder->connect('/pages/*', ['controller' => 'Pages', 'action' => 'display']); $builder->fallbacks(); });
src/Controller/TestsController.php に TestsController.php ファイルを作成します。 コントローラー ファイルに次のコードをコピーします。
src/Controller/TestsController.php
<?php declare(strict_types=1); namespace App\Controller; use Cake\Core\Configure; use Cake\Http\Exception\ForbiddenException; use Cake\Http\Exception\NotFoundException; use Cake\Http\Response; use Cake\View\Exception\MissingTemplateException; class TestsController extends AppController { public function show() { } }
src/Template の下にフォルダー Tests を作成し、そのフォルダーの下に show.php という名前の ビュー ファイル を作成します。そのファイルに次のコードをコピーします。
src/Template/Tests/show.php
<h1>This is CakePHP tutorial and this is an example of connecting routes.</h1>
http://localhost/cakephp4/ にある次の URL にアクセスして、上記の例を実行します
出力
上記の URL は次の出力を生成します。
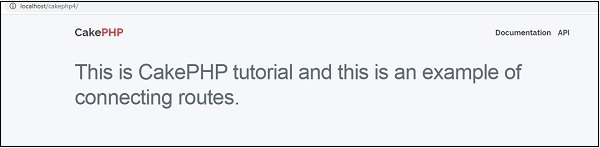
渡された引数
渡された引数は、URL で渡される引数です。これらの引数はコントローラーのアクションに渡すことができます。これらの渡された引数は、3 つの方法でコントローラーに与えられます。
アクションメソッドの引数として
次の例は、コントローラーのアクションに引数を渡す方法を示しています。次の URL (http://localhost/cakephp4/tests/value1/value2
) にアクセスしてください。これは次のルート行と一致します。
$builder->connect('tests/:arg1/:arg2', ['controller' => 'Tests', 'action' => 'show'],['pass' => ['arg1', 'arg2']]);
ここでは、URL の value1 が arg1 に割り当てられ、value2 が arg2 に割り当てられます。
数値的にインデックス付けされた配列として
引数がコントローラーのアクションに渡されたら、次のステートメントで引数を取得できます。
$args = $this->request->params[‘pass’]
コントローラーのアクションに渡される引数は $args 変数に格納されます。
ルーティングアレイの使用
次のステートメントによって引数をアクションに渡すこともできます -
$routes->connect('/', ['controller' => 'Tests', 'action' => 'show',5,6]);
上記のステートメントは、2 つの引数 5 と 6 を TestController の show() メソッドに渡します。
例
次のプログラムに示すように、config/routes.php ファイルを変更します。
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('tests/:arg1/:arg2', ['controller' => 'Tests', 'action' => 'show'],['pass' => ['arg1', 'arg2']]); $builder->connect('/pages/*', ['controller' => 'Pages', 'action' => 'display']); $builder->fallbacks(); });
src/Controller/TestsController.php に TestsController.php ファイルを作成します。 コントローラー ファイルに次のコードをコピーします。
src/Controller/TestsController.php
<?php declare(strict_types=1); namespace App\Controller; use Cake\Core\Configure; use Cake\Http\Exception\ForbiddenException; use Cake\Http\Exception\NotFoundException; use Cake\Http\Response; use Cake\View\Exception\MissingTemplateException; class TestsController extends AppController { public function show($arg1, $arg2) { $this->set('argument1',$arg1); $this->set('argument2',$arg2); } }
src/Template にフォルダー Tests を作成し、そのフォルダーの下に show.php という名前の View ファイルを作成します。そのファイルに次のコードをコピーします。
src/Template/Tests/show.php.
<h1>This is CakePHP tutorial and this is an example of Passed arguments.</h1> <?php echo "Argument-1:".$argument1."<br/>"; echo "Argument-2:".$argument2."<br/>"; ?>
次の URL http://localhost/cakephp4/tests/Virat/Kunal にアクセスして、上記の例を実行します
出力
実行すると、上記の URL は次の出力を生成します。
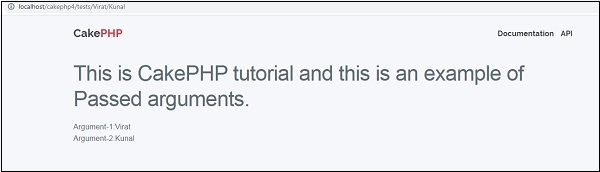
URL の生成
これは CakePHP の優れた機能です。生成された URL を使用すると、コード全体を変更することなく、アプリケーション内の URL の構造を簡単に変更できます。
url( string|array|null $url null , boolean $full false )
上記の関数は 2 つの引数を取ります -
最初の引数は、'controller'、'action'、'plugin' のいずれかを指定する配列です。さらに、ルーティングされた要素またはクエリ文字列パラメーターを提供できます。文字列の場合は、任意の有効な URL 文字列の名前を指定できます。
true の場合、完全なベース URL が結果の先頭に追加されます。デフォルトは false です。
例
次のプログラムに示すように、config/routes.php ファイルを変更します。
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('/generate',['controller'=>'Generates','action'=>'show']); $builder->fallbacks(); });
Create a GeneratesController.php file at src/Controller/GeneratesController.php. Copy the following code in the controller file.
src/Controller/GeneratesController.php
<?php declare(strict_types=1); namespace App\Controller; 21 use Cake\Core\Configure; use Cake\Http\Exception\ForbiddenException; use Cake\Http\Exception\NotFoundException; use Cake\Http\Response; use Cake\View\Exception\MissingTemplateException; class GeneratesController extends AppController { public function show() { } }
Create a folder Generates at src/Template and under that folder, create a View file called show.php. Copy the following code in that file.
src/Template/Generates/show.php
<h1>This is CakePHP tutorial and this is an example of Generating URLs<h1>
Execute the above example by visiting the following URL −
http://localhost/cakephp4/generate
Output
The above URL will produce the following output −
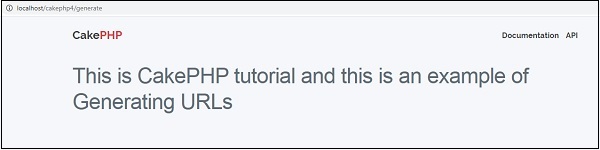
Redirect Routing
Redirect routing is useful, when we want to inform client applications that, this URL has been moved. The URL can be redirected using the following function −
static Cake\Routing\Router::redirect($route, $url, $options =[])
There are three arguments to the above function as follows −
A string describing the template of the route.
A URL to redirect to.
An array matching the named elements in the route to regular expressions which that element should match.
Example
Make Changes in the config/routes.php file as shown below. Here, we have used controllers that were created previously.
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('/generate',['controller'=>'Generates','action'=>'show']); $builder->redirect('/redirect','https://tutorialspoint.com/'); $builder->fallbacks(); });
Execute the above example by visiting the following URLs.
URL 1 − http://localhost/cakephp4/generate
Output for URL 1
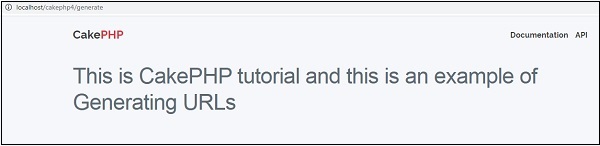
URL 2 − http://localhost/cakephp4/redirect
Output for URL 2
You will be redirected to https://tutorialspoint.com
以上がCakePHP ルーティングの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










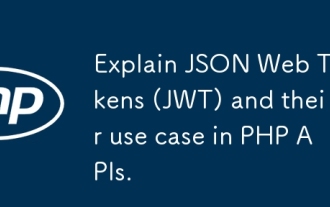
JWTは、JSONに基づくオープン標準であり、主にアイデンティティ認証と情報交換のために、当事者間で情報を安全に送信するために使用されます。 1。JWTは、ヘッダー、ペイロード、署名の3つの部分で構成されています。 2。JWTの実用的な原則には、JWTの生成、JWTの検証、ペイロードの解析という3つのステップが含まれます。 3. PHPでの認証にJWTを使用する場合、JWTを生成および検証でき、ユーザーの役割と許可情報を高度な使用に含めることができます。 4.一般的なエラーには、署名検証障害、トークンの有効期限、およびペイロードが大きくなります。デバッグスキルには、デバッグツールの使用とロギングが含まれます。 5.パフォーマンスの最適化とベストプラクティスには、適切な署名アルゴリズムの使用、有効期間を合理的に設定することが含まれます。
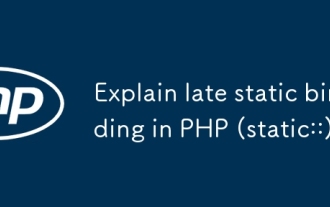
静的結合(静的::) PHPで後期静的結合(LSB)を実装し、クラスを定義するのではなく、静的コンテキストで呼び出しクラスを参照できるようにします。 1)解析プロセスは実行時に実行されます。2)継承関係のコールクラスを検索します。3)パフォーマンスオーバーヘッドをもたらす可能性があります。
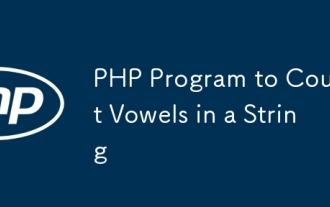
文字列は、文字、数字、シンボルを含む一連の文字です。このチュートリアルでは、さまざまな方法を使用してPHPの特定の文字列内の母音の数を計算する方法を学びます。英語の母音は、a、e、i、o、u、そしてそれらは大文字または小文字である可能性があります。 母音とは何ですか? 母音は、特定の発音を表すアルファベットのある文字です。大文字と小文字など、英語には5つの母音があります。 a、e、i、o、u 例1 入力:string = "tutorialspoint" 出力:6 説明する 文字列「TutorialSpoint」の母音は、u、o、i、a、o、iです。合計で6元があります

PHPの魔法の方法は何ですか? PHPの魔法の方法には次のものが含まれます。1。\ _ \ _コンストラクト、オブジェクトの初期化に使用されます。 2。\ _ \ _リソースのクリーンアップに使用される破壊。 3。\ _ \ _呼び出し、存在しないメソッド呼び出しを処理します。 4。\ _ \ _ get、dynamic属性アクセスを実装します。 5。\ _ \ _セット、動的属性設定を実装します。これらの方法は、特定の状況で自動的に呼び出され、コードの柔軟性と効率を向上させます。
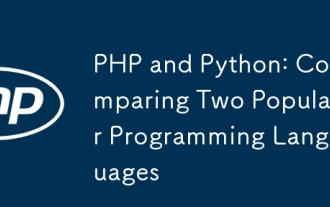
PHPとPythonにはそれぞれ独自の利点があり、プロジェクトの要件に従って選択します。 1.PHPは、特にWebサイトの迅速な開発とメンテナンスに適しています。 2。Pythonは、データサイエンス、機械学習、人工知能に適しており、簡潔な構文を備えており、初心者に適しています。
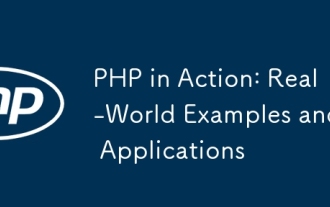
PHPは、電子商取引、コンテンツ管理システム、API開発で広く使用されています。 1)eコマース:ショッピングカート機能と支払い処理に使用。 2)コンテンツ管理システム:動的コンテンツの生成とユーザー管理に使用されます。 3)API開発:RESTFUL API開発とAPIセキュリティに使用されます。パフォーマンスの最適化とベストプラクティスを通じて、PHPアプリケーションの効率と保守性が向上します。
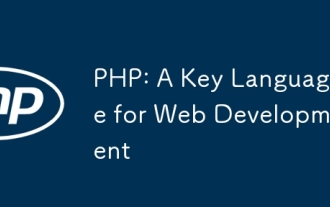
PHPは、サーバー側で広く使用されているスクリプト言語で、特にWeb開発に適しています。 1.PHPは、HTMLを埋め込み、HTTP要求と応答を処理し、さまざまなデータベースをサポートできます。 2.PHPは、ダイナミックWebコンテンツ、プロセスフォームデータ、アクセスデータベースなどを生成するために使用され、強力なコミュニティサポートとオープンソースリソースを備えています。 3。PHPは解釈された言語であり、実行プロセスには語彙分析、文法分析、編集、実行が含まれます。 4.PHPは、ユーザー登録システムなどの高度なアプリケーションについてMySQLと組み合わせることができます。 5。PHPをデバッグするときは、error_reporting()やvar_dump()などの関数を使用できます。 6. PHPコードを最適化して、キャッシュメカニズムを使用し、データベースクエリを最適化し、組み込み関数を使用します。 7
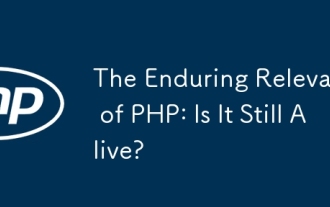
PHPは依然として動的であり、現代のプログラミングの分野で重要な位置を占めています。 1)PHPのシンプルさと強力なコミュニティサポートにより、Web開発で広く使用されています。 2)その柔軟性と安定性により、Webフォーム、データベース操作、ファイル処理の処理において顕著になります。 3)PHPは、初心者や経験豊富な開発者に適した、常に進化し、最適化しています。
