JavaScript で大規模言語モデルの力を解き放つ: 現実世界のアプリケーション
近年、大規模言語モデル (LLM) により、テクノロジーとの対話方法に革命が起こり、機械が人間のようなテキストを理解して生成できるようになりました。 JavaScript は Web 開発用の多用途言語であるため、LLM をアプリケーションに統合すると、可能性の世界が広がります。このブログでは、JavaScript を使用した LLM のエキサイティングな実用的な使用例をいくつか探り、開始するための例を示します。
1. インテリジェントなチャットボットによる顧客サポートの強化
顧客の問い合わせを 24 時間年中無休で処理し、即座に正確な応答を提供できる仮想アシスタントがあることを想像してください。 LLM を使用すると、顧客の質問を効果的に理解し、それに応答するチャットボットを構築できます。
例: カスタマー サポート チャットボット
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function getSupportResponse(query) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Customer query: "${query}". How should I respond?`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error generating response:', error); return 'Sorry, I am unable to help with that request.'; } } // Example usage const customerQuery = 'How do I reset my password?'; getSupportResponse(customerQuery).then(response => { console.log('Support Response:', response); });
この例を使用すると、一般的な顧客の質問に役立つ応答を提供し、ユーザー エクスペリエンスを向上させ、人間のサポート エージェントの作業負荷を軽減するチャットボットを構築できます。
2. 自動化されたブログアウトラインでコンテンツ作成を促進
魅力的なコンテンツの作成には時間がかかる場合があります。 LLM はブログ投稿のアウトラインの生成を支援し、コンテンツ作成をより効率的にします。
例: ブログ投稿アウトライン ジェネレーター
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function generateBlogOutline(topic) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Create a detailed blog post outline for the topic: "${topic}".`, max_tokens: 150, temperature: 0.7 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error generating outline:', error); return 'Unable to generate the blog outline.'; } } // Example usage const topic = 'The Future of Artificial Intelligence'; generateBlogOutline(topic).then(response => { console.log('Blog Outline:', response); });
このスクリプトは、次のブログ投稿の構造化されたアウトラインを迅速に生成するのに役立ち、確実な開始点を提供し、コンテンツ作成プロセスの時間を節約します。
3. リアルタイム翻訳で言語の壁を打ち破る
言語翻訳は、LLM が得意とするもう 1 つの分野です。 LLM を活用して、異なる言語を話すユーザーに即時翻訳を提供できます。
例: テキスト翻訳
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function translateText(text, targetLanguage) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Translate the following English text to ${targetLanguage}: "${text}"`, max_tokens: 60, temperature: 0.3 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error translating text:', error); return 'Translation error.'; } } // Example usage const text = 'Hello, how are you?'; translateText(text, 'French').then(response => { console.log('Translated Text:', response); });
この例を使用すると、アプリに翻訳機能を統合して、世界中のユーザーがアクセスできるようにすることができます。
4. 複雑な文章を読みやすいように要約する
長い記事を読んで理解するのは難しい場合があります。 LLM は、これらのテキストを要約して理解しやすくするのに役立ちます。
例: テキストの要約
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function summarizeText(text) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Summarize the following text: "${text}"`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error summarizing text:', error); return 'Unable to summarize the text.'; } } // Example usage const article = 'The quick brown fox jumps over the lazy dog. This sentence contains every letter of the English alphabet at least once.'; summarizeText(article).then(response => { console.log('Summary:', response); });
このコード スニペットは、長い記事やドキュメントの要約を作成するのに役立ちます。これは、コンテンツのキュレーションや情報の配布に役立ちます。
5. コード生成による開発者の支援
開発者は LLM を使用してコード スニペットを生成し、コーディング タスクを支援し、定型コードの作成に費やす時間を短縮できます。
例: コード生成
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function generateCodeSnippet(description) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Write a JavaScript function that ${description}.`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error generating code:', error); return 'Unable to generate the code.'; } } // Example usage const description = 'calculates the factorial of a number'; generateCodeSnippet(description).then(response => { console.log('Generated Code:', response); });
この例では、説明に基づいてコード スニペットを生成し、開発タスクをより効率的にすることができます。
6. パーソナライズされた推奨事項の提供
LLM は、ユーザーの興味に基づいてパーソナライズされた推奨事項を提供し、さまざまなアプリケーションでのユーザー エクスペリエンスを向上させるのに役立ちます。
例: 本の推薦
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function recommendBook(interest) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Recommend a book for someone interested in ${interest}.`, max_tokens: 60, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error recommending book:', error); return 'Unable to recommend a book.'; } } // Example usage const interest = 'science fiction'; recommendBook(interest).then(response => { console.log('Book Recommendation:', response); });
このスクリプトは、ユーザーの興味に基づいてパーソナライズされた書籍の推奨を提供します。これは、カスタマイズされたコンテンツの提案を作成するのに役立ちます。
7. 概念説明による教育支援
LLM は、複雑な概念の詳細な説明を提供することで教育を支援し、学習をよりアクセスしやすくします。
例:コンセプトの説明
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function explainConcept(concept) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Explain the concept of ${concept} in detail.`, max_tokens: 150, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error explaining concept:', error); return 'Unable to explain the concept.'; } } // Example usage const concept = 'quantum computing'; explainConcept(concept).then(response => { console.log('Concept Explanation:', response); });
この例は、複雑な概念の詳細な説明を生成するのに役立ち、教育現場で役立ちます。
8. パーソナライズされた電子メール応答の作成
パーソナライズされた応答を作成するには時間がかかる場合があります。 LLM は、コンテキストとユーザー入力に基づいてカスタマイズされた電子メール応答を生成するのに役立ちます。
例: 電子メール応答の下書き
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function draftEmailResponse(emailContent) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Draft a response to the following email: "${emailContent}"`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error drafting email response:', error); return 'Unable to draft the email response.'; } } // Example usage const emailContent = 'I am interested in your product and would like more information.'; draftEmailResponse(emailContent).then(response => { console.log('Drafted Email Response:', response); });
このスクリプトは、電子メールの返信の下書きプロセスを自動化し、時間を節約し、一貫したコミュニケーションを確保します。
9. 法的文書の要約
法的文書は密度が高く、解析が難しい場合があります。 LLM は、これらのドキュメントを要約してアクセスしやすくするのに役立ちます。
例: 法的文書の概要
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function summarizeLegalDocument(document) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Summarize the following legal document: "${document}"`, max_tokens: 150, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error summarizing document:', error); return 'Unable to summarize the document.'; } } // Example usage const document = 'This agreement governs the terms under which the parties agree to collaborate...'; summarizeLegalDocument(document).then(response => { console.log('Document Summary:', response); });
この例では、複雑な法的文書を要約して理解しやすくする方法を示します。
10. 病状の説明
医療情報は複雑で把握するのが難しい場合があります。 LLM は病状について明確かつ簡潔な説明を提供できます。
例:病状説明
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function explainMedicalCondition(condition) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Explain the medical condition ${condition} in simple terms.`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error explaining condition:', error); return 'Unable to explain the condition.'; } } // Example usage const condition = 'Type 2 Diabetes'; explainMedicalCondition(condition).then(response => { console.log('Condition Explanation:', response); });
このスクリプトは、病状の簡略化された説明を提供し、患者の教育と理解を助けます。
LLM を JavaScript アプリケーションに組み込むと、機能とユーザー エクスペリエンスが大幅に向上します。チャットボットの構築、コンテンツの生成、教育支援のいずれの場合でも、LLM はさまざまなプロセスを合理化および改善するための強力な機能を提供します。これらの例をプロジェクトに統合することで、AI の力を活用して、よりインテリジェントで応答性の高いアプリケーションを作成できます。
特定のニーズやユースケースに基づいて、これらの例を自由に調整および拡張してください。コーディングを楽しんでください!
以上がJavaScript で大規模言語モデルの力を解き放つ: 現実世界のアプリケーションの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










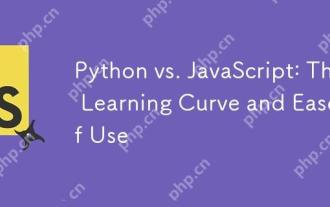
Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。
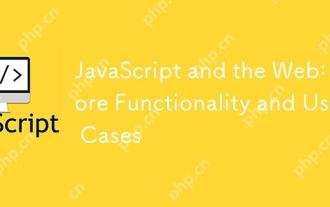
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
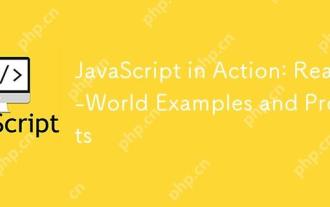
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
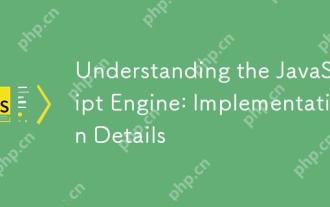
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。
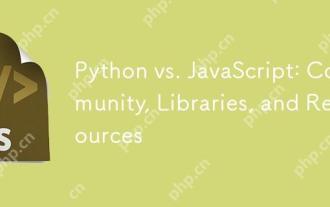
PythonとJavaScriptには、コミュニティ、ライブラリ、リソースの観点から、独自の利点と短所があります。 1)Pythonコミュニティはフレンドリーで初心者に適していますが、フロントエンドの開発リソースはJavaScriptほど豊富ではありません。 2)Pythonはデータサイエンスおよび機械学習ライブラリで強力ですが、JavaScriptはフロントエンド開発ライブラリとフレームワークで優れています。 3)どちらも豊富な学習リソースを持っていますが、Pythonは公式文書から始めるのに適していますが、JavaScriptはMDNWebDocsにより優れています。選択は、プロジェクトのニーズと個人的な関心に基づいている必要があります。
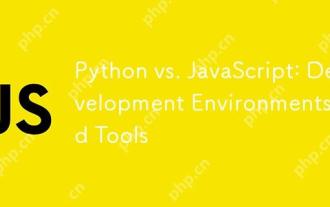
開発環境におけるPythonとJavaScriptの両方の選択が重要です。 1)Pythonの開発環境には、Pycharm、Jupyternotebook、Anacondaが含まれます。これらは、データサイエンスと迅速なプロトタイピングに適しています。 2)JavaScriptの開発環境には、フロントエンドおよびバックエンド開発に適したnode.js、vscode、およびwebpackが含まれます。プロジェクトのニーズに応じて適切なツールを選択すると、開発効率とプロジェクトの成功率が向上する可能性があります。
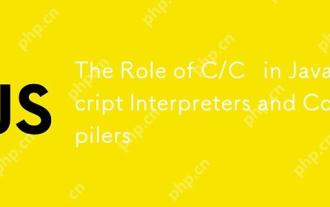
CとCは、主に通訳者とJITコンパイラを実装するために使用されるJavaScriptエンジンで重要な役割を果たします。 1)cは、JavaScriptソースコードを解析し、抽象的な構文ツリーを生成するために使用されます。 2)Cは、Bytecodeの生成と実行を担当します。 3)Cは、JITコンパイラを実装し、実行時にホットスポットコードを最適化およびコンパイルし、JavaScriptの実行効率を大幅に改善します。

Pythonはデータサイエンスと自動化により適していますが、JavaScriptはフロントエンドとフルスタックの開発により適しています。 1. Pythonは、データ処理とモデリングのためにNumpyやPandasなどのライブラリを使用して、データサイエンスと機械学習でうまく機能します。 2。Pythonは、自動化とスクリプトにおいて簡潔で効率的です。 3. JavaScriptはフロントエンド開発に不可欠であり、動的なWebページと単一ページアプリケーションの構築に使用されます。 4. JavaScriptは、node.jsを通じてバックエンド開発において役割を果たし、フルスタック開発をサポートします。
