すべての開発者が知っておくべき重要な Web API
Mastering various Web APIs can significantly enhance your web application's functionality and user experience. These APIs provide developers with tools to interact with browsers in ways that were previously impossible. Here, we’ll explore 12 essential Web APIs, explain their functionality, and provide code examples to help you implement them in your projects.
1. Storage API
The Web Storage API (including localStorage and sessionStorage) allows you to store key-value pairs in a web browser. It's useful for saving user preferences or persisting data between sessions.
Code Example:
// Save data to localStorage localStorage.setItem('userName', 'Vishal'); // Retrieve data from localStorage const user = localStorage.getItem('userName'); // Clear localStorage localStorage.removeItem('userName');
Learn More about Storage API
2. Payment Request API
The Payment Request API simplifies the process of accepting payments on the web by providing a consistent user experience across various payment methods.
Code Example:
if (window.PaymentRequest) { const payment = new PaymentRequest([{ supportedMethods: 'basic-card' }], { total: { label: 'Total', amount: { currency: 'USD', value: '10.00' } } }); payment.show().then(result => { // Process payment result console.log(result); }).catch(error => { console.error('Payment failed:', error); }); }
Learn More about Payment Request API
3. DOM API
The DOM (Document Object Model) API allows you to manipulate the structure, style, and content of the document. This is one of the most widely used APIs in web development.
Code Example:
// Select and update an element const element = document.querySelector('#myElement'); element.textContent = 'Hello, World!';
Learn More about DOM API
4. HTML Sanitizer API
The HTML Sanitizer API helps clean up untrusted HTML content to avoid security risks like XSS (Cross-Site Scripting) attacks.
Code Example:
const dirtyHTML = '<img src="javascript:alert(1)">'; const cleanHTML = sanitizer.sanitize(dirtyHTML); console.log(cleanHTML); // Safe HTML output
Learn More about HTML Sanitizer API
5. Canvas API
The Canvas API allows you to draw graphics and animations on a web page using the
Code Example:
const canvas = document.getElementById('myCanvas'); const context = canvas.getContext('2d'); context.fillStyle = 'blue'; context.fillRect(10, 10, 150, 100);
Learn More about Canvas API
6. History API
The History API lets you interact with the browser’s session history, allowing you to manipulate the browser's history stack (e.g., pushState, replaceState).
Code Example:
history.pushState({ page: 1 }, 'title', '/page1'); history.replaceState({ page: 2 }, 'title', '/page2');
Learn More about History API
7. Clipboard API
The Clipboard API allows you to read from and write to the clipboard, enabling features like copy-paste functionality.
Code Example:
navigator.clipboard.writeText('Hello, Clipboard!').then(() => { console.log('Text copied to clipboard'); }).catch(err => { console.error('Failed to copy text:', err); });
Learn More about Clipboard API
8. Fullscreen API
The Fullscreen API allows you to present a specific element or the entire webpage in fullscreen mode, useful for videos or immersive experiences like games.
Code Example:
document.getElementById('myElement').requestFullscreen().catch(err => { console.error(`Error attempting to enable full-screen mode: ${err.message}`); });
Learn More about Fullscreen API
9. FormData API
The FormData API simplifies the process of constructing key/value pairs representing form fields and their values for easier form data submission via XHR or Fetch.
Code Example:
const form = document.querySelector('form'); const formData = new FormData(form); fetch('/submit', { method: 'POST', body: formData }).then(response => { if (response.ok) { console.log('Form submitted successfully!'); } });
Learn More about FormData API
10. Fetch API
The Fetch API provides a modern and flexible way to make asynchronous network requests, offering a simpler, promise-based alternative to XMLHttpRequest.
Code Example:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error fetching data:', error));
Learn More about Fetch API
11. Drag and Drop API
The Drag and Drop API allows you to implement drag-and-drop functionality in your web applications, enhancing user interactions with intuitive UI elements.
Code Example:
const item = document.getElementById('item'); item.addEventListener('dragstart', (e) => { e.dataTransfer.setData('text/plain', item.id); });
Learn More about Drag and Drop API
12. Geolocation API
The Geolocation API provides access to geographical location information from the user’s device, enabling location-based services and features.
Code Example:
navigator.geolocation.getCurrentPosition((position) => { console.log(`Latitude: ${position.coords.latitude}, Longitude: ${position.coords.longitude}`); }, (error) => { console.error(`Error getting location: ${error.message}`); });
Learn More about Geolocation API
Conclusion
These Web APIs open up a world of possibilities for creating highly interactive, user-friendly web applications. From storage and payments to geolocation and graphics, mastering these APIs can take your web development skills to the next level.
By understanding how to effectively implement these APIs in your projects, you can significantly enhance both functionality and user experience.
References:
- MDN Web Docs - Introduction to Web APIs
- W3Schools - Web APIs Introduction
- Mozilla Developer Network - Web APIs
- Web APIs - Microsoft Learn
If you found this guide useful, please consider sharing it with others! ?
이 블로그에서는 각 API에 대한 명확한 설명과 실용적인 코드 예제를 통합하면서 모든 개발자가 알아야 할 필수 웹 API에 대한 업데이트된 개요를 제공합니다.
以上がすべての開発者が知っておくべき重要な Web APIの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










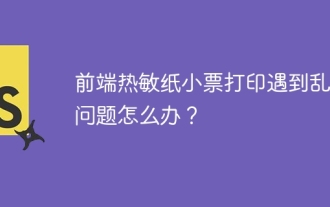
フロントエンドのサーマルペーパーチケット印刷のためのよくある質問とソリューションフロントエンド開発におけるチケット印刷は、一般的な要件です。しかし、多くの開発者が実装しています...
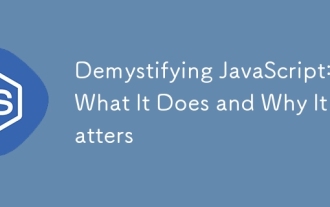
JavaScriptは現代のWeb開発の基礎であり、その主な機能には、イベント駆動型のプログラミング、動的コンテンツ生成、非同期プログラミングが含まれます。 1)イベント駆動型プログラミングにより、Webページはユーザー操作に応じて動的に変更できます。 2)動的コンテンツ生成により、条件に応じてページコンテンツを調整できます。 3)非同期プログラミングにより、ユーザーインターフェイスがブロックされないようにします。 JavaScriptは、Webインタラクション、シングルページアプリケーション、サーバー側の開発で広く使用されており、ユーザーエクスペリエンスとクロスプラットフォーム開発の柔軟性を大幅に改善しています。
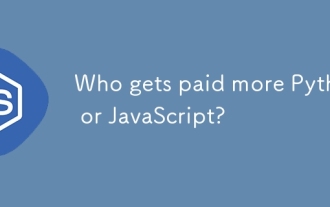
スキルや業界のニーズに応じて、PythonおよびJavaScript開発者には絶対的な給与はありません。 1. Pythonは、データサイエンスと機械学習でさらに支払われる場合があります。 2。JavaScriptは、フロントエンドとフルスタックの開発に大きな需要があり、その給与もかなりです。 3。影響要因には、経験、地理的位置、会社の規模、特定のスキルが含まれます。
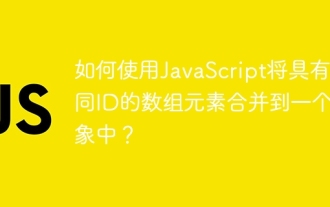
同じIDを持つ配列要素をJavaScriptの1つのオブジェクトにマージする方法は?データを処理するとき、私たちはしばしば同じIDを持つ必要性に遭遇します...
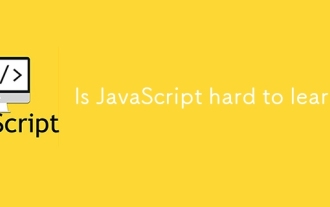
JavaScriptを学ぶことは難しくありませんが、挑戦的です。 1)変数、データ型、関数などの基本概念を理解します。2)非同期プログラミングをマスターし、イベントループを通じて実装します。 3)DOM操作を使用し、非同期リクエストを処理することを約束します。 4)一般的な間違いを避け、デバッグテクニックを使用します。 5)パフォーマンスを最適化し、ベストプラクティスに従ってください。
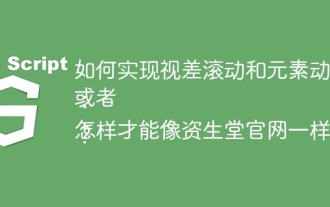
この記事の視差スクロールと要素のアニメーション効果の実現に関する議論では、Shiseidoの公式ウェブサイト(https://www.shisido.co.co.jp/sb/wonderland/)と同様の達成方法について説明します。
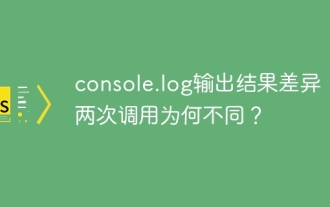
Console.log出力の違いの根本原因に関する詳細な議論。この記事では、Console.log関数の出力結果の違いをコードの一部で分析し、その背後にある理由を説明します。 �...
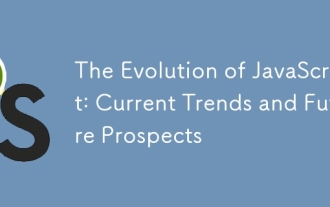
JavaScriptの最新トレンドには、TypeScriptの台頭、最新のフレームワークとライブラリの人気、WebAssemblyの適用が含まれます。将来の見通しは、より強力なタイプシステム、サーバー側のJavaScriptの開発、人工知能と機械学習の拡大、およびIoTおよびEDGEコンピューティングの可能性をカバーしています。
