React でのイベント処理
Event handling in React allows you to respond to user interactions like clicks, form submissions, and other events. Here’s a basic overview and example:
Basic Concepts
- Event Binding: In React, you typically use camelCase for event names (e.g., onClick, onChange).
- Event Handling: You can pass a function as an event handler directly in JSX.
- Synthetic Events: React wraps the native events in a synthetic event to ensure cross-browser compatibility.
Example
Here's a simple example of handling a button click and an input change:
import React, { useState } from 'react'; const EventHandlingExample = () => { const [inputValue, setInputValue] = useState(''); const handleClick = () => { alert(`Button clicked! Input value: ${inputValue}`); }; const handleChange = (event) => { setInputValue(event.target.value); }; return ( <div> <input type="text" value={inputValue} onChange={handleChange} placeholder="Type something..." /> <button onClick={handleClick}>Click Me</button> </div> ); }; export default EventHandlingExample;
Key Points
- State Management: Use useState to manage the state in functional components.
- Event Object: The event handler receives an event object that contains information about the event.
- Prevent Default: Use event.preventDefault() to prevent the default behavior of events (like form submissions).
Feel free to ask if you need specific examples or further explanations!
以上がReact でのイベント処理の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック








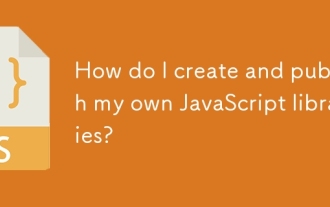
記事では、JavaScriptライブラリの作成、公開、および維持について説明し、計画、開発、テスト、ドキュメント、およびプロモーション戦略に焦点を当てています。
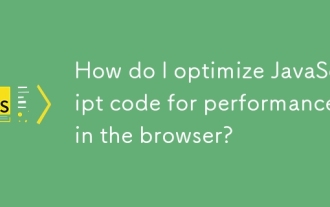
この記事では、ブラウザでJavaScriptのパフォーマンスを最適化するための戦略について説明し、実行時間の短縮、ページの負荷速度への影響を最小限に抑えることに焦点を当てています。
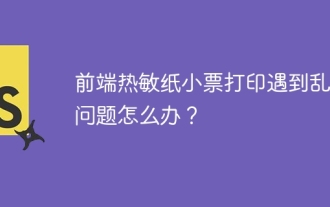
フロントエンドのサーマルペーパーチケット印刷のためのよくある質問とソリューションフロントエンド開発におけるチケット印刷は、一般的な要件です。しかし、多くの開発者が実装しています...
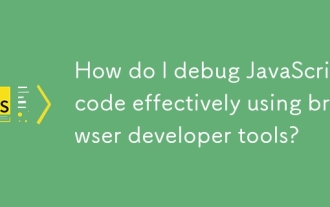
この記事では、ブラウザ開発者ツールを使用した効果的なJavaScriptデバッグについて説明し、ブレークポイントの設定、コンソールの使用、パフォーマンスの分析に焦点を当てています。
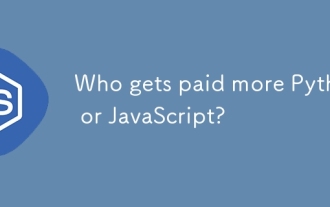
スキルや業界のニーズに応じて、PythonおよびJavaScript開発者には絶対的な給与はありません。 1. Pythonは、データサイエンスと機械学習でさらに支払われる場合があります。 2。JavaScriptは、フロントエンドとフルスタックの開発に大きな需要があり、その給与もかなりです。 3。影響要因には、経験、地理的位置、会社の規模、特定のスキルが含まれます。
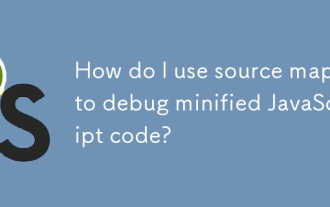
この記事では、ソースマップを使用して、元のコードにマッピングすることにより、Minified JavaScriptをデバッグする方法について説明します。ソースマップの有効化、ブレークポイントの設定、Chrome DevtoolsやWebpackなどのツールの使用について説明します。
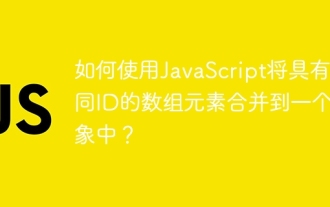
同じIDを持つ配列要素をJavaScriptの1つのオブジェクトにマージする方法は?データを処理するとき、私たちはしばしば同じIDを持つ必要性に遭遇します...
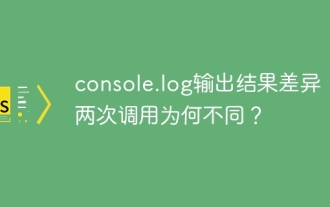
Console.log出力の違いの根本原因に関する詳細な議論。この記事では、Console.log関数の出力結果の違いをコードの一部で分析し、その背後にある理由を説明します。 �...
